Embed:
(wiki syntax)
Show/hide line numbers
USBBusInterface.h
00001 /* USBBusInterface.h */ 00002 /* USB Bus Interface */ 00003 /* Copyright (c) 2011 ARM Limited. All rights reserved. */ 00004 00005 #ifndef _USB_BUS_INTERFACE_ 00006 #define _USB_BUS_INTERFACE_ 00007 00008 #include "mbed.h" 00009 #include "USBEndpoints.h" 00010 00011 /* Configuration */ 00012 bool USBBusInterface_init(void); 00013 void USBBusInterface_uninit(void); 00014 void USBBusInterface_connect(void); 00015 void USBBusInterface_disconnect(void); 00016 void USBBusInterface_configureDevice(void); 00017 void USBBusInterface_unconfigureDevice(void); 00018 void USBBusInterface_setAddress(uint8_t address); 00019 void USBBusInterface_remoteWakeup(void); 00020 00021 /* Endpoint 0 */ 00022 void USBBusInterface_EP0setup(uint8_t *buffer); 00023 void USBBusInterface_EP0read(void); 00024 uint32_t USBBusInterface_EP0getReadResult(uint8_t *buffer); 00025 void USBBusInterface_EP0write(uint8_t *buffer, uint32_t size); 00026 void USBBusInterface_EP0getWriteResult(void); 00027 void USBBusInterface_EP0stall(void); 00028 00029 /* Other endpoints */ 00030 EP_STATUS USBBusInterface_endpointRead(uint8_t endpoint, uint8_t *data, uint32_t maximumSize); 00031 EP_STATUS USBBusInterface_endpointReadResult(uint8_t endpoint, uint32_t *bytesRead); 00032 EP_STATUS USBBusInterface_endpointWrite(uint8_t endpoint, uint8_t *data, uint32_t size); 00033 EP_STATUS USBBusInterface_endpointWriteResult(uint8_t endpoint); 00034 void USBBusInterface_stallEndpoint(uint8_t endpoint); 00035 void USBBusInterface_unstallEndpoint(uint8_t endpoint); 00036 bool USBBusInterface_realiseEndpoint(uint8_t endpoint, uint32_t maxPacket, uint32_t options); 00037 bool USBBusInterface_getEndpointStallState(unsigned char endpoint); 00038 00039 #endif 00040 00041
Generated on Fri Jul 15 2022 02:22:27 by
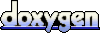