
This example positions a lit LED in the I2C LED array using the rotary encoder
Embed:
(wiki syntax)
Show/hide line numbers
RotaryEncode.cpp
00001 /* 00002 RotaryEncode 00003 (c) 2009, cstyles 00004 */ 00005 00006 #include "RotaryEncode.h" 00007 #include "mbed.h" 00008 00009 /* 00010 Constructor, pin names for I2C and the I2C addrss of the device 00011 */ 00012 00013 00014 RotaryEncode::RotaryEncode(PinName A, PinName B) 00015 : _rotary_in(A,B) { 00016 00017 _position = 0; 00018 00019 // Attach ticker 00020 _ticker.attach(this, &RotaryEncode::_ticker_handler, 0.010); 00021 } 00022 00023 00024 00025 int RotaryEncode::read(void) { 00026 int rval = _position; 00027 _position = 0; 00028 return (rval); 00029 } 00030 00031 00032 00033 00034 void RotaryEncode::_ticker_handler(void) { 00035 00036 _state = _rotary_in; 00037 _e = R_W; 00038 00039 // we're in the no-change state 00040 if (_state == 0x03) { 00041 return; 00042 } 00043 00044 while (_state != 0x03) { 00045 switch (_e) { 00046 case R_W: 00047 if (_state == 0x02) 00048 _e = R_R1; 00049 else if (_state == 0x01) 00050 _e = R_L1; 00051 break; 00052 00053 case R_L1: 00054 if (_state == 0x00) 00055 _e = R_R2; 00056 break; 00057 case R_L2: 00058 if (_state == 0x01) { 00059 _e = R_R3; 00060 _position++; 00061 } 00062 break; 00063 case R_R1: 00064 if (_state == 0x00) 00065 _e = R_L2; 00066 break; 00067 case R_R2: 00068 if (_state == 0x02) { 00069 _e = R_L3; 00070 _position--; 00071 } 00072 break; 00073 } 00074 _state = _rotary_in; 00075 } 00076 00077 } 00078 00079 00080 00081
Generated on Fri Jul 15 2022 02:53:23 by
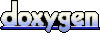