
This example positions a lit LED in the I2C LED array using the rotary encoder
Embed:
(wiki syntax)
Show/hide line numbers
PCA9532.cpp
00001 /* 00002 PCA9532 00003 (c) 2009, cstyles 00004 */ 00005 00006 #include "PCA9532.h" 00007 #include "mbed.h" 00008 00009 /* 00010 Constructor, pin names for I2C and the I2C addrss of the device 00011 */ 00012 00013 00014 PCA9532::PCA9532(PinName scl, PinName sda, int addr) 00015 : _i2c(scl, sda) { 00016 00017 _addr = addr; 00018 00019 } 00020 00021 00022 00023 00024 00025 /* 00026 force the LEDs on or off according to thier corresponding bits 00027 in the vector 00028 */ 00029 00030 void PCA9532::write (int leds) { 00031 00032 // cycle through the array 00033 for (int i=0; i < 16; i++) { 00034 00035 // if the ith bit is '1' 00036 if (leds & (0x1 << i)) { 00037 _rmw(i,PCA9532_MODE_SET); 00038 } 00039 00040 else { 00041 _rmw(i,PCA9532_MODE_CLEAR); 00042 } 00043 } 00044 } 00045 00046 00047 00048 /* 00049 this is one hot encoding for the LED array 00050 any bit set will have it's corresponding LED switched on 00051 */ 00052 00053 void PCA9532::set (int leds) { 00054 for (int i=0; i < 16; i++) { 00055 if (leds & (0x1 << i)) { 00056 _rmw(i,PCA9532_MODE_SET); 00057 } 00058 } 00059 } 00060 00061 00062 void PCA9532::clear (int leds) { 00063 for (int i=0; i < 16; i++) { 00064 if (leds & (0x1 << i)) { 00065 _rmw(i,PCA9532_MODE_CLEAR); 00066 } 00067 } 00068 } 00069 00070 00071 void PCA9532::pwm0 (int leds) { 00072 for (int i=0; i < 16; i++) { 00073 if (leds & (0x1 << i)) { 00074 _rmw(i,PCA9532_MODE_PWM0); 00075 } 00076 } 00077 } 00078 00079 00080 void PCA9532::pwm1 (int leds) { 00081 for (int i=0; i < 16; i++) { 00082 if (leds & (0x1 << i)) { 00083 _rmw(i,PCA9532_MODE_PWM1); 00084 } 00085 } 00086 } 00087 00088 00089 void PCA9532::duty0 (float d) { 00090 00091 char duty = 0; 00092 00093 if (d > 1.0) { duty = 255; } 00094 else if ( d < 0.0 ) { duty = 0; } 00095 else { duty = 256 * d; } 00096 00097 _write(PCA9532_REG_PWM0,duty); 00098 00099 } 00100 00101 00102 void PCA9532::duty1 (float d) { 00103 00104 char duty = 0; 00105 00106 if (d > 1.0) { duty = 255; } 00107 else if ( d < 0.0 ) { duty = 0; } 00108 else { duty = 256 * d; } 00109 00110 _write(PCA9532_REG_PWM1,duty); 00111 00112 } 00113 00114 00115 00116 00117 00118 00119 00120 /* 00121 led is in the range 0-15 00122 mode is inthe range 0-3 00123 */ 00124 00125 void PCA9532::_rmw(int led, int mode) { 00126 00127 int reg = 0; 00128 int offset = (led % 4); 00129 00130 // makesure mode is within bounds 00131 if ( (mode < 0) || (mode > 3) ) { 00132 return; 00133 } 00134 00135 00136 // determine which register this is, 00137 if (led < 4) { 00138 reg = PCA9532_REG_LS0;} 00139 00140 else if ( (led > 3) && (led < 8) ) { 00141 reg = PCA9532_REG_LS1;} 00142 00143 else if ( (led > 7) && (led < 12) ) { 00144 reg = PCA9532_REG_LS2;} 00145 00146 else if ( (led > 11) && (led < 16) ) { 00147 reg = PCA9532_REG_LS3;} 00148 00149 else { return; } 00150 00151 // read the current status of the register 00152 char regval = _read(reg); 00153 00154 // clear the two bit slice at the calculated offset 00155 regval &= ~(0x3 << (2 * offset)); 00156 00157 // now OR in the mode, shifted by 2 8 offset 00158 regval |= (mode << (2 * offset)); 00159 00160 // write this back 00161 00162 _write(reg,regval); 00163 00164 return; 00165 } 00166 00167 00168 void PCA9532::_write(int reg, int data) { 00169 char args[2]; 00170 args[0] = reg; 00171 args[1] = data; 00172 _i2c.write(_addr,args,2); 00173 } 00174 00175 00176 int PCA9532::_read(int reg) { 00177 char args[2]; 00178 args[0] = reg; 00179 _i2c.write(_addr,args,2); 00180 return(args[1]); 00181 } 00182 00183 00184 00185 00186
Generated on Fri Jul 15 2022 02:53:23 by
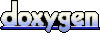