
Example of how it might be good to start with the DS1306
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 DigitalOut myled(LED1); 00004 00005 Serial pc (USBTX,USBRX); 00006 00007 SPI spi (p5,p6,p7); 00008 DigitalOut cs (p8); 00009 00010 int main() { 00011 00012 spi.format(8,2); 00013 00014 // set initial state of CS pin 00015 cs = 0; 00016 wait (0.1); 00017 00018 // start by clearing WP in Control register 00019 // the RAM can not be accessed until WP is clear 00020 00021 cs = 1; 00022 wait (0.1); 00023 spi.write (0x8f); // write to control register 00024 spi.write(0x0); // clear all the bits 00025 cs = 0; 00026 00027 wait (0.1); 00028 00029 // Now write to the RAM 00030 cs = 1; 00031 wait (0.1); 00032 spi.write (0xA0); // write to first RAM location 00033 spi.write(0xa5); // set a pattern 00034 cs = 0; 00035 00036 wait (0.1); 00037 00038 // Now read from RAM 00039 cs = 1; 00040 wait (0.1); 00041 spi.write (0x20); // read from first RAM location 00042 char data = spi.write(0x00); 00043 cs = 0; 00044 00045 00046 printf("Read from location 0x20 and received 0x%X\n",data); 00047 00048 00049 while (1) {} 00050 00051 }
Generated on Sat Jul 30 2022 23:14:27 by
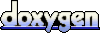