
A program to insert Valid User Code checksums
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 LocalFileSystem fs ("fs"); 00004 DigitalOut led1(LED1); 00005 DigitalOut led4(LED4); 00006 00007 int main() { 00008 00009 int ctr=32; // 32 because the fixed size of the vector table 00010 00011 printf("File patcher\n"); 00012 00013 FILE *f1 = fopen("/fs/file.unp","rb"); // read binary 00014 FILE *f2 = fopen("/fs/file.pat","wb"); // write binary 00015 00016 if (f1 == NULL) { 00017 printf("Cant open file for reading\n"); 00018 while (1) { 00019 led1 = !led1; 00020 wait(0.2); 00021 } 00022 } 00023 00024 else { 00025 00026 unsigned int vector [8]; // storage for the vector table 00027 unsigned int sum = 0; // 00028 00029 // read first 7 words (28 bytes) into an array 00030 for (int i = 0; i < 8; i++) { 00031 vector[i] = 0; // zero the vector location 00032 for (int j = 0; j < 4; j++) { 00033 char c = fgetc(f1); // read char from file 00034 vector[i] |= (c << (8*j)); // shift to byte location 00035 } 00036 sum += vector[i]; // keep a running total 00037 } 00038 00039 00040 // calculate the 2's compliment, for vector[7] 00041 vector[7] = (~sum) + 1; 00042 00043 for (int i = 0; i < 8; i++) { 00044 printf("Vector[%d] = 0x%08x\n",i,vector[i]); 00045 } 00046 00047 printf("Sum vector = 0x%08x\n",sum); 00048 00049 00050 // write out patched vector table 00051 // read first 7 words (28 bytes) into an array 00052 for (int i = 0; i < 8; i++) { 00053 for (int j = 0; j < 4; j++) { 00054 char c = ( vector[i] >> (8*j)) & 0xFF; 00055 fputc(c,f2); // read char from file 00056 } 00057 } 00058 00059 // read in and write remaining bytes of source binary 00060 while (!feof(f1)) { 00061 char c = fgetc (f1); // read char from file 00062 fputc(c,f2); // write byte to file 00063 ctr++; 00064 } 00065 00066 } 00067 00068 fclose(f1); 00069 fclose(f2); 00070 00071 printf("Done! %d bytes processed\n",ctr); 00072 while (1) { 00073 led4 = !led4; 00074 wait(0.2); 00075 } 00076 00077 00078 }
Generated on Fri Jul 15 2022 03:25:01 by
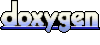