
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 #include "HTTPServer.h" 00004 #include "HTTPRPC.h" 00005 #include "HTTPFS.h" 00006 00007 #include "MobileLCD.h" 00008 #include "LIS302.h" 00009 #include "RFID.h" 00010 #include "RGBLED.h" 00011 #include "Servo.h" 00012 #include "usbhid.h" 00013 00014 LocalFileSystem local("local"); 00015 Serial pc(USBTX, USBRX); 00016 00017 InterruptIn RedButton(p30,"redbutton"); 00018 DigitalIn GreenButton(p29,"greenbutton"); 00019 DigitalIn BlueButton(p28,"bluebutton"); 00020 00021 DigitalOut led1(LED1,"led1"); 00022 DigitalOut led2(LED2,"led2"); 00023 DigitalOut led3(LED3,"led3"); 00024 DigitalOut led4(LED4,"led4"); 00025 00026 AnalogIn light(p16); 00027 AnalogIn pot(p20,"pot"); 00028 00029 RFID rfid (NC,p27); 00030 MobileLCD lcd(p11, p12, p13, p14, p15); 00031 LIS302 acc (p5,p6,p7,p8); 00032 RGBLED rgb (p24,p22,p23); 00033 Servo servo (p21); 00034 00035 usbhid hid; 00036 00037 // Function for Rising ede of RedButton 00038 void RedRise () { 00039 led1 = !led1; 00040 } 00041 00042 00043 00044 00045 int main() { 00046 00047 // Attach the interupt hander to RedButton 00048 RedButton.rise(&RedRise); 00049 00050 // Print to LCD and the serial port 00051 lcd.printf("Hello World!"); 00052 pc.printf("Hello World!"); 00053 00054 00055 while (1) { 00056 00057 00058 // ---------------------------------- 00059 // Accelerometer example 00060 // ---------------------------------- 00061 00062 /* 00063 float x = acc.x(); 00064 float y = acc.y(); 00065 float z = acc.z(); 00066 lcd.locate(0,0); 00067 lcd.printf("x=%0.3f ",x); 00068 lcd.locate(0,1); 00069 lcd.printf("y=%0.3f ",y); 00070 lcd.locate(0,2); 00071 lcd.printf("z=%0.3f ",z); 00072 wait (0.5); 00073 */ 00074 00075 00076 // ---------------------------------- 00077 // RFID Example 00078 // ---------------------------------- 00079 /* 00080 if (rfid.readable()) { 00081 int id=rfid.read(); 00082 lcd.cls(); 00083 lcd.printf("ID : %d",id); 00084 00085 } 00086 */ 00087 00088 00089 // ---------------------------------- 00090 // Accelerometer example 00091 // ---------------------------------- 00092 00093 /* 00094 float x = acc.x(); 00095 float y = acc.y(); 00096 float z = acc.z(); 00097 lcd.locate(0,0); 00098 lcd.printf("x=%0.3f ",x); 00099 lcd.locate(0,1); 00100 lcd.printf("y=%0.3f ",y); 00101 lcd.locate(0,2); 00102 lcd.printf("z=%0.3f ",z); 00103 wait (0.5); 00104 */ 00105 00106 00107 00108 // ---------------------------------- 00109 // Servo example 00110 // ---------------------------------- 00111 /* 00112 servo=pot; 00113 wait (0.01); 00114 */ 00115 00116 // ---------------------------------- 00117 // RGB LED example with accelerometer 00118 // ---------------------------------- 00119 /* 00120 rgb.red(abs(acc.x())); 00121 rgb.green(abs(acc.y())); 00122 rgb.blue(abs(acc.z())); 00123 */ 00124 00125 00126 // ---------------------------------- 00127 // Light sensor example 00128 // ---------------------------------- 00129 /* 00130 lcd.locate(0,0); 00131 lcd.printf("Light: %.2f ",light.read()); 00132 wait (0.2); 00133 */ 00134 00135 00136 // ---------------------------------- 00137 // USB HID 00138 // ---------------------------------- 00139 /* 00140 if (rfid.readable()) { 00141 int id; 00142 char msg[25]; 00143 id = rfid.read(); 00144 sprintf(msg,"Tag ID : %d\n",id); 00145 hid.keyboard(msg); 00146 } 00147 00148 */ 00149 00150 00151 00152 // ---------------------------------- 00153 // RPC over ethernet 00154 // ---------------------------------- 00155 00156 00157 // Create a HTTPServer on default Port 00158 HTTPServer *http = new HTTPServer(); 00159 // Register RPC in /rpc space 00160 http->addHandler(new HTTPRPC()); 00161 // HTTP File system 00162 http->addHandler(new HTTPFileSystemHandler("/", "/local/")); 00163 // Register the HTTPServer on the Network device (will hopfully disappear in the next Version) 00164 http->bind(); 00165 00166 NetServer *net = NetServer::get(); 00167 00168 lcd.locate(0,1); 00169 lcd.printf("%hhu.%hhu.%hhu.%hhu", (net->getIPAddr().addr)&0xFF, (net->getIPAddr().addr>>8)&0xFF, (net->getIPAddr().addr>>16)&0xFF, (net->getIPAddr().addr>>24)&0xFF); 00170 00171 while(1) { 00172 http->poll(); 00173 } 00174 00175 00176 00177 00178 00179 00180 00181 00182 00183 00184 00185 00186 00187 00188 00189 00190 00191 00192 00193 } 00194 00195 00196 } 00197 00198
Generated on Mon Jul 25 2022 12:41:11 by
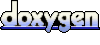