
vr1.1
Dependencies: FreescaleIAP mbed-rtos mbed
Fork of CDMS_RTOS_v1_1 by
PL.cpp
00001 #include "mbed.h" 00002 #include "PL.h" 00003 #include "all_funcs.h" 00004 #include "Flags.h" 00005 #include "pin_config.h" 00006 00007 SPISlave pl_spi(PIN16, PIN17, PIN15, PIN14); // mosi, miso, sclk, ssel --> using SPI1 00008 00009 Serial sr(USBTX,USBRX); 00010 00011 void FCTN_PL_RCV_SC_DATA() 00012 { 00013 sr.printf("in FCTN_PL_RCV_SC_DATA\r\n"); 00014 uint8_t scienceRawPacket[payloadBins]; 00015 for(int i=0; i<payloadBins; i++) 00016 { 00017 while(!pl_spi.receive()); // blocking statement --> waiting for data from Payload 00018 uint8_t v = pl_spi.read(); // Read byte from master 00019 scienceRawPacket[i] = v; 00020 } 00021 // serial feedback 00022 sr.printf("Packet recieved\r\r\n"); 00023 for(int i=0; i<payloadBins; i++) 00024 { 00025 sr.printf("%0x\t",scienceRawPacket[i]); 00026 scienceRawPacket[i] = 0; 00027 } 00028 sr.printf("\r\r\n"); 00029 } 00030 00031 void FCTN_PL_SCIENCE() 00032 { 00033 all_flags|=PL_SCIENCE_STATUS; 00034 FCTN_PL_RCV_SC_DATA(); 00035 if(all_flags&IS_FIRST_TIME_SC_DATA == 0) //What happens to TIME_ELAPSED_LAST_SRP on reset? Should it be stored in Flash? 00036 { 00037 TIME_ELAPSED_LAST_SRP=SRP_INTER_VAL_COUNTER; 00038 SRP_INTER_VAL_COUNTER.reset(); 00039 } 00040 else 00041 { 00042 SRP_INTER_VAL_COUNTER.start(); 00043 all_flags&=(~IS_FIRST_TIME_SC_DATA); 00044 } 00045 if(all_flags&IS_PL_FRAME_SIZE_CORRECT == 1) //How to identify FRAME_SIZE or size of data that is received via SPI? 00046 { 00047 //FCTN_COM_COMP_SC_DATA(scienceRawPacket); 00048 //FCTN_WR_SD(const uint8_t *, uint64_t) //Store SD 00049 //FCTN_CDMS_WR_FLASH(); // 00050 all_flags|=PL_FRAME_SIZE_CORRECT; 00051 } 00052 if(all_flags&IS_PL_FRAME_SIZE_CORRECT == 0) 00053 { 00054 //FCTN_WR_SD(const uint8_t *, uint64_t) 00055 //FCTN_CDMS_WR_FLASH(); 00056 all_flags&=~(PL_FRAME_SIZE_CORRECT); 00057 } 00058 if(TIME_ELAPSED_LAST_SRP>4) 00059 { 00060 //FCTN_CDMS_WR_FLASH(); 00061 all_flags|= TIME_ELAPSED_LAST_SRP_INTERVAL_HIGH; 00062 } 00063 if(TIME_ELAPSED_LAST_SRP<2) 00064 { 00065 //FCTN_CDMS_WR_FLASH(); 00066 all_flags|= TIME_ELAPSED_LAST_SRP_INTERVAL_LOW; 00067 } 00068 all_flags&=~(PL_SCIENCE_STATUS); 00069 } 00070 00071 void FCTN_PL_MAIN() 00072 { 00073 all_flags|=PL_MAIN_STATUS; 00074 all_flags&=~(PL_LOW_POWER); 00075 all_flags = all_flags|((all_flags&PL_STATE)<<2); //pl_prev_state = pl_state; 00076 if(all_flags&IS_PL_SCHEDULE == 1) //is pl_schedule == 1 00077 { 00078 all_flags = (all_flags&(~PL_STATE))|pl_schedule_TC; 00079 } 00080 else 00081 { 00082 if((all_flags&PL_PREV_STATE) == PL_SCIENCE) 00083 { 00084 all_flags = (all_flags&(~PL_STATUS))|PL_HIBERNATE; 00085 } 00086 else 00087 { 00088 all_flags = (all_flags&(~PL_STATE))|((all_flags&PL_PREV_STATE)>>2); //pl_state = pl_prev_state; 00089 } 00090 } 00091 switch(all_flags&PL_STATE) 00092 { 00093 case PL_OFF: 00094 { 00095 if(all_flags&PL_PREV_STATE!=PL_OFF) 00096 { 00097 sr.printf("Command SPEED DL to go to Standby State (I2C) \r\n"); 00098 } 00099 sr.printf("Power off SPEED\r\n"); 00100 all_flags = (all_flags&(~PL_STATUS))|PL_OFF; 00101 sr.printf("Disable SRP_INTERVAL_COUNTER\r\n"); 00102 all_flags = all_flags&(~PL_MAIN_STATUS); 00103 } 00104 case PL_STANDBY: 00105 { 00106 if(power_level<0) 00107 { 00108 all_flags = all_flags|PL_LOW_POWER; 00109 if(all_flags&PL_PREV_STATE!=PL_OFF) 00110 { 00111 sr.printf("Command SPEED DL to go to Standby State (I2C) \r\n"); 00112 } 00113 sr.printf("Power off SPEED\r\n"); 00114 all_flags = (all_flags&(~PL_STATUS))|PL_OFF; 00115 sr.printf("Disable SRP_INTERVAL_COUNTER\r\n"); 00116 all_flags = all_flags&(~PL_MAIN_STATUS); 00117 } 00118 else 00119 { 00120 if(all_flags&PL_PREV_STATE!=PL_OFF) 00121 { 00122 sr.printf("Command SPEED DL to go to Standby State (I2C) \r\n"); 00123 sr.printf("Power OFF SPEED PMTs\r\n"); 00124 if(all_flags&IS_I2C_ACK == 1) 00125 { 00126 all_flags = (all_flags&(~PL_STATUS))|PL_STANDBY; } 00127 else 00128 { 00129 all_flags = (all_flags&(~PL_STATUS))|PL_ERR_I2C; 00130 } 00131 sr.printf("Disable SRP_INTERVAL_COUNTER\r\n"); 00132 all_flags = all_flags&(~PL_MAIN_STATUS); 00133 } 00134 else 00135 { 00136 sr.printf("Power on SPPED DL\r\n"); 00137 sr.printf("Command SPEED DL to go to Standby State (I2C) \r\n"); 00138 if(all_flags&IS_I2C_ACK == 1) 00139 { 00140 all_flags = (all_flags&(~PL_STATUS))|PL_STANDBY; 00141 } 00142 else 00143 { 00144 all_flags = (all_flags&(~PL_STATUS))|PL_ERR_I2C; 00145 } 00146 sr.printf("Disable SRP_INTERVAL_COUNTER\r\n"); 00147 all_flags = all_flags&(~PL_MAIN_STATUS); 00148 } 00149 } 00150 } 00151 case PL_HIBERNATE: 00152 { 00153 if(power_level<1) 00154 { 00155 all_flags = all_flags|PL_LOW_POWER; 00156 if(power_level<0) 00157 { 00158 if(all_flags&PL_PREV_STATE!=PL_OFF) 00159 { 00160 sr.printf("Command SPEED DL to go to Standby State (I2C) \r\n"); 00161 } 00162 sr.printf("Power off SPEED\r\n"); 00163 all_flags = (all_flags&(~PL_STATUS))|PL_OFF; 00164 sr.printf("Disable SRP_INTERVAL_COUNTER\r\n"); 00165 all_flags = all_flags&(~PL_MAIN_STATUS); 00166 } 00167 else 00168 { 00169 if(all_flags&PL_PREV_STATE!=PL_OFF) 00170 { 00171 sr.printf("Command SPEED DL to go to Standby State (I2C) \r\n"); 00172 sr.printf("Power OFF SPEED PMTs\r\n"); 00173 if(all_flags&IS_I2C_ACK == 1) 00174 { 00175 all_flags = (all_flags&(~PL_STATUS))|PL_STANDBY; } 00176 else 00177 { 00178 all_flags = (all_flags&(~PL_STATUS))|PL_ERR_I2C; 00179 } 00180 sr.printf("Disable SRP_INTERVAL_COUNTER\r\n"); 00181 all_flags = all_flags&(~PL_MAIN_STATUS); 00182 } 00183 else 00184 { 00185 sr.printf("Power on SPPED DL\r\n"); 00186 sr.printf("Command SPEED DL to go to Standby State (I2C) \r\n"); 00187 if(all_flags&IS_I2C_ACK == 1) 00188 { 00189 all_flags = (all_flags&(~PL_STATUS))|PL_STANDBY; 00190 } 00191 else 00192 { 00193 all_flags = (all_flags&(~PL_STATUS))|PL_ERR_I2C; 00194 } 00195 sr.printf("Disable SRP_INTERVAL_COUNTER\r\n"); 00196 all_flags = all_flags&(~PL_MAIN_STATUS); 00197 } 00198 } 00199 } 00200 else if(power_level>1) 00201 { 00202 if(all_flags&PL_PREV_STATE==PL_OFF) 00203 { 00204 sr.printf("Power on SPEED DL\r\n"); 00205 } 00206 sr.printf("Power on Speed PMT with reduced Voltage\r\n"); 00207 sr.printf("Command SPEED DL to go to Standby State (I2C) \r\n"); 00208 if(all_flags&IS_I2C_ACK == 1) 00209 { 00210 all_flags = (all_flags&(~PL_STATUS))|PL_HIBERNATE; 00211 } 00212 else 00213 { 00214 all_flags = (all_flags&(~PL_STATUS))|PL_ERR_I2C; 00215 } 00216 sr.printf("Disable SRP_INTERVAL_COUNTER\r\n"); 00217 all_flags = all_flags&(~PL_MAIN_STATUS); 00218 } 00219 } 00220 case PL_SCIENCE: 00221 { 00222 if(power_level<2) 00223 { 00224 if(power_level<1) 00225 { 00226 all_flags = all_flags|PL_LOW_POWER; 00227 if(power_level<0) 00228 { 00229 if(all_flags&PL_PREV_STATE!=PL_OFF) 00230 { 00231 sr.printf("Command SPEED DL to go to Standby State (I2C) \r\n"); 00232 } 00233 sr.printf("Power off SPEED\r\n"); 00234 all_flags = (all_flags&(~PL_STATUS))|PL_OFF; 00235 sr.printf("Disable SRP_INTERVAL_COUNTER\r\n"); 00236 all_flags = all_flags&(~PL_MAIN_STATUS); 00237 } 00238 else 00239 { 00240 if(all_flags&PL_PREV_STATE!=PL_OFF) 00241 { 00242 sr.printf("Command SPEED DL to go to Standby State (I2C) \r\n"); 00243 sr.printf("Power OFF SPEED PMTs\r\n"); 00244 if(all_flags&IS_I2C_ACK == 1) 00245 { 00246 all_flags = (all_flags&(~PL_STATUS))|PL_STANDBY; } 00247 else 00248 { 00249 all_flags = (all_flags&(~PL_STATUS))|PL_ERR_I2C; 00250 } 00251 sr.printf("Disable SRP_INTERVAL_COUNTER\r\n"); 00252 all_flags = all_flags&(~PL_MAIN_STATUS); 00253 } 00254 else 00255 { 00256 sr.printf("Power on SPPED DL\r\n"); 00257 sr.printf("Command SPEED DL to go to Standby State (I2C) \r\n"); 00258 if(all_flags&IS_I2C_ACK == 1) 00259 { 00260 all_flags = (all_flags&(~PL_STATUS))|PL_STANDBY; 00261 } 00262 else 00263 { 00264 all_flags = (all_flags&(~PL_STATUS))|PL_ERR_I2C; 00265 } 00266 sr.printf("Disable SRP_INTERVAL_COUNTER\r\n"); 00267 all_flags = all_flags&(~PL_MAIN_STATUS); 00268 } 00269 } 00270 } 00271 else if(power_level>1) 00272 { 00273 if(all_flags&PL_PREV_STATE==PL_OFF) 00274 { 00275 sr.printf("Power on SPEED DL\r\n"); 00276 } 00277 sr.printf("Power on Speed PMT with reduced Voltage\r\n"); 00278 sr.printf("Command SPEED DL to go to Standby State (I2C) \r\n"); 00279 if(all_flags&IS_I2C_ACK == 1) 00280 { 00281 all_flags = (all_flags&(~PL_STATUS))|PL_HIBERNATE; } 00282 else 00283 { 00284 all_flags = (all_flags&(~PL_STATUS))|PL_ERR_I2C; 00285 } 00286 sr.printf("Disable SRP_INTERVAL_COUNTER\r\n"); 00287 all_flags = all_flags&(~PL_MAIN_STATUS); 00288 } 00289 } 00290 else if(power_level>2) 00291 { 00292 if(all_flags&PL_PREV_STATE==PL_SCIENCE) 00293 { 00294 all_flags = (all_flags&(~PL_STATUS))|PL_SCIENCE; 00295 all_flags = all_flags&(~PL_MAIN_STATUS); 00296 } 00297 else if(all_flags&PL_PREV_STATE==PL_HIBERNATE) 00298 { 00299 sr.printf("Power on SPEED PMT with high voltage \r\n"); 00300 sr.printf("Command SPEED DL to go to Standby State (I2C) \r\n"); 00301 if(all_flags&IS_I2C_ACK == 1) 00302 { 00303 sr.printf("Enable SRP_INTERVAL_COUNTER\r\n"); 00304 all_flags = (all_flags&(~PL_STATUS))|PL_SCIENCE; 00305 all_flags = all_flags&(~PL_MAIN_STATUS); 00306 } 00307 else 00308 { 00309 all_flags = (all_flags&(~PL_STATUS))|PL_ERR_I2C; 00310 sr.printf("Disable SRP_INTERVAL_COUNTER\r\n"); 00311 all_flags = all_flags&(~PL_MAIN_STATUS); 00312 } 00313 } 00314 else 00315 00316 { 00317 if(all_flags&PL_PREV_STATE!=PL_OFF) 00318 { 00319 sr.printf("Power on SPEED DL\r\n"); 00320 } 00321 sr.printf("Power on Speed PMT with reduced Voltage\r\n"); 00322 sr.printf("Command SPEED DL to go to Standby State (I2C) \r\n"); 00323 if(all_flags&IS_I2C_ACK == 1) 00324 { 00325 all_flags = (all_flags&(~PL_STATUS))|PL_HIBERNATE; 00326 } 00327 else 00328 { 00329 all_flags = (all_flags&(~PL_STATUS))|PL_ERR_I2C; 00330 } 00331 sr.printf("Disable SRP_INTERVAL_COUNTER\r\n"); 00332 all_flags = all_flags&(~PL_MAIN_STATUS); 00333 } 00334 } 00335 } 00336 } 00337 }
Generated on Sat Jul 16 2022 21:48:56 by
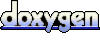