
Polytech school project. RICM4 students, see http://air.imag.fr/index.php/Projets-2016-2017-Station_de_pompage_connect%C3%A9e for more information
Fork of SX1276PingPong by
trame.cpp
00001 #include "mbed.h" 00002 #include "trame.h" 00003 #include "debug.h" 00004 00005 TrameData::TrameData(char idE, char idS, int nbNiveau, char etatP, char niveau, char batterie){ 00006 idEmetteur = idE; 00007 idStation = idS; 00008 if(nbNiveau >= 63){ 00009 nombreNiveau = 0x1F; 00010 }else{ 00011 nombreNiveau = (char) nbNiveau; 00012 } 00013 mettreAJourEtatPompe(etatP); 00014 mettreAJourNiveauCuve(niveau); 00015 mettreAJourNiveauBatterie(batterie); 00016 trame[4]=0; 00017 00018 } 00019 char getiemebit(char c, int i) 00020 { 00021 return ((c>>(7-i)) & 1); 00022 } 00023 00024 00025 TrameData::TrameData(char* Buff){ 00026 idStation = Buff[0]; 00027 idEmetteur = Buff[1]; 00028 etatPompe = Buff[2]>>7; 00029 nombreNiveau = (Buff[2] & 0x7C) >> 2; 00030 niveauCuve = ((Buff[2] & 0x3)<<3) | ((Buff[3] & 0xE0)>>5); 00031 niveauBatterie = (Buff[3] & 0xF); 00032 trame[4]=0; 00033 00034 /* 00035 00036 00037 00038 //Etat Pompe 00039 for(int i=0; i < 8; i++){ 00040 if(getiemebit(etatPompe, i)){ 00041 debug("1"); 00042 } 00043 else{ 00044 debug("0"); 00045 } 00046 } 00047 debug("\r\n"); 00048 //nombreNiveau 00049 for(int i=0; i < 8; i++){ 00050 if(getiemebit(nombreNiveau, i)){ 00051 debug("1"); 00052 } 00053 else{ 00054 debug("0"); 00055 } 00056 } 00057 debug("\r\n niveauCuve \r\n"); 00058 //niveauCuve 00059 for(int i=0; i < 8; i++){ 00060 if(getiemebit(niveauCuve, i)){ 00061 debug("1"); 00062 } 00063 else{ 00064 debug("0"); 00065 } 00066 } 00067 00068 debug("\r\n"); 00069 //niveauBatterie 00070 for(int i=0; i < 8; i++){ 00071 if(getiemebit(niveauBatterie, i)){ 00072 debug("1"); 00073 } 00074 else{ 00075 debug("0"); 00076 } 00077 }*/ 00078 } 00079 00080 char TrameData::getIdEmetteur(){ 00081 return idEmetteur; 00082 } 00083 char TrameData::getIdStation(){ 00084 return idStation; 00085 } 00086 00087 char* TrameData::creerTrame(){ 00088 trame[0] = idStation; 00089 trame[1] = idEmetteur; 00090 trame[2] = (etatPompe<<7)|(nombreNiveau<<2)|(niveauCuve>>3); 00091 trame[3] = (niveauCuve<<5)|(niveauBatterie); 00092 trame[4] = 0; 00093 00094 /*char courant; 00095 for(int j=0; j<4;j++){ 00096 courant = trame[j]; 00097 for(int i=0; i < 8; i++){ 00098 if(getiemebit(courant, i)){ 00099 debug("1"); 00100 } 00101 else{ 00102 debug("0"); 00103 } 00104 } 00105 debug(" "); 00106 } 00107 debug("\r\n"); 00108 00109 00110 //Etat Pompe 00111 for(int i=0; i < 8; i++){ 00112 if(getiemebit(etatPompe, i)){ 00113 debug("1"); 00114 } 00115 else{ 00116 debug("0"); 00117 } 00118 } 00119 debug("\r\n"); 00120 //nombreNiveau 00121 for(int i=0; i < 8; i++){ 00122 if(getiemebit(nombreNiveau, i)){ 00123 debug("1"); 00124 } 00125 else{ 00126 debug("0"); 00127 } 00128 } 00129 debug("\r\n"); 00130 //niveauCuve 00131 for(int i=0; i < 8; i++){ 00132 if(getiemebit(niveauCuve, i)){ 00133 debug("1"); 00134 } 00135 else{ 00136 debug("0"); 00137 } 00138 } 00139 00140 debug("\r\n"); 00141 //niveauBatterie 00142 for(int i=0; i < 8; i++){ 00143 if(getiemebit(niveauBatterie, i)){ 00144 debug("1"); 00145 } 00146 else{ 00147 debug("0"); 00148 } 00149 } 00150 00151 //debug("%c ; %c ; %c\r\n",trame[0],trame[1],trame[2]); 00152 char courant; 00153 debug("%d\r\n", sizeof(char)); 00154 for(int j=0; j<3;j++){ 00155 courant = trame[j]; 00156 for(int i=0; i < 8; i++){ 00157 if(getiemebit(courant, i)){ 00158 debug("1"); 00159 } 00160 else{ 00161 debug("0"); 00162 } 00163 } 00164 debug(" "); 00165 } 00166 debug("\r\n"); 00167 */ 00168 00169 return trame; 00170 } 00171 void TrameData::mettreAJourEtatPompe(char etatP){ 00172 etatPompe = etatP; 00173 } 00174 void TrameData::mettreAJourNiveauCuve(char niveau){ 00175 niveauCuve = niveau; 00176 } 00177 void TrameData::mettreAJourNiveauBatterie(char niveau){ 00178 niveauBatterie = niveau; 00179 }
Generated on Thu Jul 14 2022 10:24:05 by
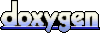