
OLED display demonstration. 16-pin multi-mode 0.96in module (SPI, I2C, DIO parallel) configured in 4-wire SPI. STM32L152RE Nucleo platform.
main.cpp
00001 /* 00002 Demonstrate 0.96 in. OLED module (128x64/SPI). Revised for use with ST Nucleo L152RE 00003 00004 Note: On 16 pin OLED module from Taobao, MOSI connects to module D1 00005 and CLK (also called SCK) connects to module D0. Module RW and RD lines 00006 are connected to GND. 00007 */ 00008 00009 #include "mbed.h" 00010 00011 #include "ssd1306.h" 00012 #include "standard_font.h" 00013 #include "bold_font.h" 00014 00015 /* definitions for ST Nucleo L152RE */ 00016 #define CSEL PB_6 // CS D10 00017 #define RST PC_7 // D9 00018 #define DCMD PA_9 // DC D8 00019 #define CLK PA_5 // CLK D13 00020 #define DATA PA_7 // MOSI D11 00021 00022 // SSD1306 oled(p8 /* cs */, p9 /* reset */, p14 /* dc */, p13 /* clock */, p11 /* data */); // LPC1768 00023 // SSD1306 oled(PTA13 /* cs */, PTD5 /* reset */, PTD0 /* dc */, PTD1 /* clock */, PTD2 /* data */); // KL25Z 00024 // SSD1306 oled(D10 /* cs */, D9 /* reset */, D8 /* dc */, D13 /* clock */, D11 /* data */); // KL05Z or Arduino styles 00025 SSD1306 oled(CSEL, RST, DCMD, CLK, DATA); // STM32 Nucleo 00026 00027 #define NUMFLAKES 10 00028 #define XPOS 0 00029 #define YPOS 1 00030 #define DELTAY 2 00031 00032 #define LOGO16_GLCD_HEIGHT 16 00033 #define LOGO16_GLCD_WIDTH 16 00034 00035 static const unsigned char logo16_glcd_bmp[] = 00036 { 0x00, 0xc0, // B00000000, B11000000, 00037 0x01, 0xc0, // B00000001, B11000000, 00038 0x01, 0xc0, // B00000001, B11000000, 00039 0x03, 0xe0, // B00000011, B11100000, 00040 0xf3, 0xe0, // B11110011, B11100000, 00041 0xfe, 0xf8, // B11111110, B11111000, 00042 0x7e, 0xff, // B01111110, B11111111, 00043 0x33, 0x9f, // B00110011, B10011111, 00044 0x1f, 0xfc, // B00011111, B11111100, 00045 0x0d, 0x70, // B00001101, B01110000, 00046 0x1b, 0xa0, // B00011011, B10100000, 00047 0x3f, 0xe0, // B00111111, B11100000, 00048 0x3f, 0xf0, // B00111111, B11110000, 00049 0x7c, 0xf0, // B01111100, B11110000, 00050 0x70, 0x70, // B01110000, B01110000, 00051 0x00, 0x30 }; // B00000000, B00110000 }; 00052 00053 void testdrawbitmap(const unsigned char *bitmap, int w, int h) { 00054 uint8_t icons[NUMFLAKES][3]; 00055 srand((unsigned int)time(NULL)); // srandom(123); // whatever seed 00056 int i; 00057 00058 // initialize 00059 for (uint8_t f=0; f< NUMFLAKES; f++) { 00060 icons[f][XPOS] = rand() % SSD1306_LCDWIDTH; // display.width(); 00061 icons[f][YPOS] = 0; 00062 icons[f][DELTAY] = rand() % 5 + 1; 00063 #if 0 00064 Serial.print("x: "); 00065 Serial.print(icons[f][XPOS], DEC); 00066 Serial.print(" y: "); 00067 Serial.print(icons[f][YPOS], DEC); 00068 Serial.print(" dy: "); 00069 Serial.println(icons[f][DELTAY], DEC); 00070 #endif 00071 } 00072 00073 i = 0; 00074 while (1) { 00075 // draw each icon 00076 for (uint8_t f=0; f< NUMFLAKES; f++) { 00077 oled.drawBitmap(icons[f][XPOS], icons[f][YPOS], logo16_glcd_bmp, w, h, 1); // WHITE); 00078 } 00079 oled.update(); 00080 wait(0.2); // delay(200); 00081 00082 // then erase it + move it 00083 for (uint8_t f=0; f< NUMFLAKES; f++) { 00084 oled.drawBitmap(icons[f][XPOS], icons[f][YPOS], logo16_glcd_bmp, w, h, 0); // BLACK); 00085 // move it 00086 icons[f][YPOS] += icons[f][DELTAY]; 00087 // if its gone, reinit 00088 if (icons[f][YPOS] > SSD1306_LCDHEIGHT) { // display.height()) { 00089 icons[f][XPOS] = rand() % SSD1306_LCDWIDTH; // display.width(); 00090 icons[f][YPOS] = 0; 00091 icons[f][DELTAY] = rand() % 5 + 1; 00092 } 00093 } 00094 if (i++ > 100) break; 00095 } 00096 00097 } 00098 00099 void testdrawline() { 00100 for (int16_t i=0; i<SSD1306_LCDWIDTH; i+=4) { 00101 oled.line(0, 0, i, SSD1306_LCDHEIGHT-1); 00102 oled.update(); 00103 } 00104 for (int16_t i=0; i<SSD1306_LCDHEIGHT; i+=4) { 00105 oled.line(0, 0, SSD1306_LCDWIDTH-1, i); 00106 oled.update(); 00107 } 00108 wait(0.25); // delay(250); 00109 00110 oled.clear(); 00111 for (int16_t i=0; i<SSD1306_LCDWIDTH; i+=4) { 00112 oled.line(0, SSD1306_LCDHEIGHT-1, i, 0); 00113 oled.update(); 00114 } 00115 for (int16_t i=SSD1306_LCDHEIGHT-1; i>=0; i-=4) { 00116 oled.line(0, SSD1306_LCDHEIGHT-1, SSD1306_LCDWIDTH-1, i); 00117 oled.update(); 00118 } 00119 wait(0.25); // delay(250); 00120 00121 oled.clear(); 00122 for (int16_t i=SSD1306_LCDWIDTH-1; i>=0; i-=4) { 00123 oled.line(SSD1306_LCDWIDTH-1, SSD1306_LCDHEIGHT-1, i, 0); 00124 oled.update(); 00125 } 00126 for (int16_t i=SSD1306_LCDHEIGHT-1; i>=0; i-=4) { 00127 oled.line(SSD1306_LCDWIDTH-1, SSD1306_LCDHEIGHT-1, 0, i); 00128 oled.update(); 00129 } 00130 wait(0.25); // delay(250); 00131 00132 oled.clear(); 00133 for (int16_t i=0; i<SSD1306_LCDHEIGHT; i+=4) { 00134 oled.line(SSD1306_LCDWIDTH-1, 0, 0, i); 00135 oled.update(); 00136 } 00137 for (int16_t i=0; i<SSD1306_LCDWIDTH; i+=4) { 00138 oled.line(SSD1306_LCDWIDTH-1, 0, i, SSD1306_LCDHEIGHT-1); 00139 oled.update(); 00140 } 00141 wait(0.25); 00142 } 00143 00144 int main() 00145 { 00146 oled.initialise(); 00147 oled.clear(); 00148 oled.set_contrast(255); // max contrast 00149 00150 while(1) 00151 { 00152 00153 oled.drawBitmap(30, 16, logo16_glcd_bmp, 16, 16); 00154 oled.update(); 00155 wait(3); 00156 00157 testdrawline(); 00158 00159 oled.clear(); 00160 testdrawbitmap(logo16_glcd_bmp, LOGO16_GLCD_HEIGHT, LOGO16_GLCD_WIDTH); 00161 00162 oled.set_font(bold_font, 8); 00163 oled.printf("Heading\r\n"); 00164 00165 oled.set_font(standard_font, 6); 00166 oled.printf("Hello World!\r\n"); 00167 oled.printf("Some more text here...\r\n\r\n\r\n\r\n"); 00168 // oled.set_font(bold_font, 8); 00169 oled.line(127, 0, 0, 63); 00170 00171 oled.update(); 00172 wait(1); 00173 00174 int i = 10; 00175 while (i > 0) 00176 { 00177 wait(1); 00178 oled.printf("%d\r\n", i--); 00179 oled.update(); 00180 oled.scroll_up(); 00181 } 00182 00183 oled.clear(); 00184 00185 } // end outside loop for OLED demo 00186 } 00187 00188 // EOF
Generated on Sat Jul 16 2022 01:07:52 by
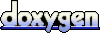