
testing n-Bed SPI flash
Fork of SPIFLASH_AT45DB by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 // N.C. n-bed test AT45DB161E 00002 // PAGE_SIZE 512, PAGE_NUM 8192 00003 00004 00005 00006 /* 00007 * SPI FLASH AT45DB081D (Atmel) 00008 * 8Mbit 00009 */ 00010 #include "mbed.h" 00011 #include "at45db161d.h" 00012 00013 #undef PAGE_SIZE 00014 //#define PAGE_SIZE 264 // AT45DB081D (1MB) 00015 #define PAGE_SIZE 512 // AT45DB081D (1MB) N.C. 00016 //#define PAGE_SIZE 528 // AT45DB321D (4MB) 00017 #define PAGE_NUM 4095 // AT45DB081D (1MB) 00018 //#define PAGE_NUM 8192 // AT45DB321D (4MB) 00019 00020 #define WRITE_BUFFER 1 00021 #define READ_BUFFER 2 00022 00023 DigitalOut myled(LED1); 00024 Serial pc(USBTX, USBRX); 00025 00026 SPI spi(p11, p12, p13); // mosi, miso, sclk 00027 ATD45DB161D memory(spi, p14); 00028 00029 void flash_write (int addr, char *buf, int len) { 00030 int i; 00031 memory.BufferWrite(WRITE_BUFFER, addr % PAGE_SIZE); 00032 for (i = 0; i < len; i ++) { 00033 spi.write(buf[i]); 00034 } 00035 memory.BufferToPage(WRITE_BUFFER, addr / PAGE_SIZE, 1); 00036 } 00037 00038 void flash_read (int addr, char *buf, int len) { 00039 int i; 00040 memory.PageToBuffer(addr / PAGE_SIZE, READ_BUFFER); 00041 memory.BufferRead(READ_BUFFER, addr % PAGE_SIZE, 1); 00042 for (i = 0; i < len; i ++) { 00043 buf[i] = spi.write(0xff); 00044 } 00045 } 00046 00047 int main() { 00048 int i; 00049 char buf[PAGE_SIZE]; 00050 Timer t; 00051 ATD45DB161D::ID id; 00052 00053 //pc.baud(115200); 00054 spi.frequency(16000000); 00055 wait_ms(500); 00056 00057 memory.ReadManufacturerAndDeviceID(&id); 00058 printf("RAM Manufacturer ID : %02x\r\n", id.manufacturer); 00059 printf("RAM Device ID : %02x %02x\r\n", id.device[0], id.device[1]); 00060 wait_ms(10); 00061 00062 printf("\r\nHELLO test\r\n"); 00063 00064 printf("RAM write\r\n"); 00065 strcpy(buf, "Hello!"); 00066 flash_write(0, buf, 6); 00067 00068 for (i = 0; i < PAGE_SIZE; i ++) { 00069 buf[i] = i; 00070 } 00071 flash_write(6, buf, PAGE_SIZE - 6); 00072 00073 wait(1); 00074 memset(buf, 0, PAGE_SIZE); 00075 00076 printf("RAM read\r\n"); 00077 flash_read(0, buf, PAGE_SIZE); 00078 for (i = 0; i < PAGE_SIZE; i ++) { 00079 printf(" %02x", buf[i]); 00080 if ((i & 0x0f) == 0x0f) 00081 printf("\r\n"); 00082 } 00083 00084 wait(1); 00085 00086 printf("\r\nWrite/Read time\r\n"); 00087 00088 printf("RAM write\r\n"); 00089 t.reset(); 00090 t.start(); 00091 for (i = 0; i < 0x20000; i += PAGE_SIZE) { 00092 buf[0] = (i >> 8) & 0xff; 00093 flash_write(i, buf, PAGE_SIZE); 00094 if ((i & 0x0fff) == 0) printf("."); 00095 } 00096 t.stop(); 00097 printf("\r\ntime %f, %f KBytes/sec\r\n", t.read(), (float)0x20000 / 1024 / t.read()); 00098 00099 wait(1); 00100 00101 printf("RAM read\r\n"); 00102 t.reset(); 00103 t.start(); 00104 for (i = 0; i < 0x20000; i += PAGE_SIZE) { 00105 flash_read(i, buf, PAGE_SIZE); 00106 if (buf[0] != ((i >> 8) & 0xff)) { 00107 printf("error %d\r\n", i); 00108 break; 00109 } 00110 if ((i & 0x0fff) == 0) printf("."); 00111 } 00112 t.stop(); 00113 printf("\r\ntime %f, %f KBytes/sec\r\n", t.read(), 0x20000 / 1024 / t.read()); 00114 00115 }
Generated on Tue Jul 19 2022 19:22:58 by
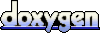