
n-bed test program
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 // n-bed testing 24AA02E48 MAC address reading 00002 // based on MBED-LPC4088 EXAMPLE: 00003 // http://developer.mbed.org/users/embeddedartists/notebook/lpc4088-quickstart-board---how-to-expand---i2c/ 00004 00005 #include "mbed.h" 00006 00007 // 24AA02E48 datasheet: 00008 // http://ww1.microchip.com/downloads/en/DeviceDoc/20002124E.pdf 00009 00010 // the address of the EEPROM I2C device 00011 #define EEPROM_24AA02E48_ADDR (0xA0) 00012 00013 static I2C i2c(P0_27, P0_28); 00014 00015 int main(void) { 00016 00017 int i = 0; 00018 char offset = 0; 00019 bool verifiedOk = true; 00020 char buf[7]; 00021 00022 printf("Reading MAC address\n"); 00023 00024 do { 00025 00026 // the MAC address is stored at offset 0xFA in the EEPROM 00027 // start by telling the EEPROM that we want to access this offset 00028 offset = 0xFA; 00029 00030 // write returns non-0 on failure 00031 if (i2c.write(EEPROM_24AA02E48_ADDR, &offset, 1) != 0) { 00032 printf("Failed to write to I2C device (%x) at offset 0xFA\n", 00033 EEPROM_24AA02E48_ADDR); 00034 break; 00035 } 00036 00037 // read the MAC address (48 bits = 6 bytes). The read function 00038 // returns non-0 in case of failure 00039 if (i2c.read(EEPROM_24AA02E48_ADDR, buf, 6) != 0) { 00040 printf("Failed to read from I2C device (%x) at offset 0xFA\n", 00041 EEPROM_24AA02E48_ADDR); 00042 } 00043 00044 printf(" - the MAC address is %02X:%02X:%02X:%02X:%02X:%02X\n", 00045 buf[0],buf[1],buf[2],buf[3],buf[4],buf[5]); 00046 00047 } while (0); 00048 00049 printf("Writing data to EEPROM\n"); 00050 00051 do { 00052 00053 // initializing the buffer with values that will be 00054 // written to the EEPROM. Note that index 0 is used as 00055 // the offset and data are put in the remaining 6 bytes 00056 // see "write data" section below 00057 for (i = 0; i < 6; i++) { 00058 buf[i+1] = i+3; 00059 } 00060 00061 // We write to the beginning of the EEPROM (offset = 0) in this 00062 // example. 00063 // 00064 // Please note that there are limitations to how to write to the EEPROM 00065 // - At most one page (8 bytes for this EEPROM) can be written at a time 00066 // - A write request will wrap around if writing passed a page boundary 00067 // - Example: If writing 4 bytes starting at offset 6, positions 6,7 00068 // 0, and 1 will actually be written since page boundaries 00069 // are at even page sizes (0, 8, 16, ...) and a write 00070 // request will wrap around if passing a page boundary. 00071 // - the upper part (0x80-0xFF) are always write protected for this 00072 // particular EEPROM 00073 offset = 0; 00074 00075 00076 // write data 00077 // All data is written in one request (page write). The first byte must 00078 // therefore contain the offset to where the data should be written. 00079 // It is also possible to write one byte at a time. 00080 buf[0] = offset; 00081 if (i2c.write(EEPROM_24AA02E48_ADDR, buf, 7) != 0) { 00082 printf("Failed to write to I2C device (%x) 2\n", 00083 EEPROM_24AA02E48_ADDR); 00084 break; 00085 } 00086 00087 00088 } while (0); 00089 00090 printf("Reading back written data\n"); 00091 00092 do { 00093 // resetting the buffer 00094 memset(buf, 0, 6); 00095 00096 00097 // tell the EEPROM which offset to use 00098 if (i2c.write(EEPROM_24AA02E48_ADDR, &offset, 1) != 0) { 00099 printf("Failed to write to I2C device (%x) 3\n", 00100 EEPROM_24AA02E48_ADDR); 00101 break; 00102 } 00103 00104 // read data 00105 if (i2c.read(EEPROM_24AA02E48_ADDR, buf, 6) != 0) { 00106 printf("Failed to read from I2C device (%x) 3\n", 00107 EEPROM_24AA02E48_ADDR); 00108 } 00109 00110 // verifying read data 00111 for (i = 0; i < 6; i++) { 00112 if (buf[i] != (i+3)) { 00113 verifiedOk = false; 00114 printf(" - Read data not equal to written buf[%d]=%d != %d\n", 00115 i, buf[i], (i+3)); 00116 break; 00117 } 00118 } 00119 00120 if (verifiedOk) { 00121 printf(" - Read data is equal to written data\n"); 00122 } 00123 00124 } while (0); 00125 00126 return 0; 00127 }
Generated on Wed Jul 20 2022 00:26:58 by
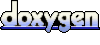