
testing n-Bed with a Logitech C270 camera
Fork of USBHostC270_example by
USBHostC270.cpp
00001 #include "USBHostC270.h" 00002 #include "dbg.h" 00003 00004 //#define C270_DEBUG 1 00005 #ifdef C270_DEBUG 00006 #define C270_DBG(x, ...) std::printf("[%s:%d]"x"\r\n", __PRETTY_FUNCTION__, __LINE__, ##__VA_ARGS__); 00007 #else 00008 #define C270_DBG(...) while(0); 00009 #endif 00010 00011 // ------------------ HcControl Register --------------------- 00012 #define OR_CONTROL_IE 0x00000008 00013 00014 USBHostC270::USBHostC270(int formatIndex, int frameIndex, uint32_t interval) 00015 { 00016 C270_DBG("formatIndex: %d, frameIndex: %d, interval: %d", formatIndex, frameIndex, interval); 00017 _formatIndex = formatIndex; 00018 _frameIndex = frameIndex; 00019 _interval = interval; 00020 clearOnResult(); 00021 host = USBHost::getHostInst(); 00022 m_isoEp = new IsochronousEp; 00023 init(); 00024 } 00025 00026 void USBHostC270::init() 00027 { 00028 C270_DBG(""); 00029 dev_connected = false; 00030 dev = NULL; 00031 c270_intf = -1; 00032 c270_device_found = false; 00033 c270_vid_pid_found = false; 00034 } 00035 00036 bool USBHostC270::connected() 00037 { 00038 return dev_connected; 00039 } 00040 00041 bool USBHostC270::connect() 00042 { 00043 if (dev_connected) { 00044 return true; 00045 } 00046 00047 for (uint8_t i = 0; i < MAX_DEVICE_CONNECTED; i++) { 00048 if ((dev = host->getDevice(i)) != NULL) { 00049 00050 C270_DBG("Trying to connect C270 device\r\n"); 00051 00052 if(host->enumerate(dev, this)) { 00053 break; 00054 } 00055 if (c270_device_found) { 00056 USB_INFO("New C270 device: VID:%04x PID:%04x [dev: %p - intf: %d]", dev->getVid(), dev->getPid(), dev, c270_intf); 00057 dev->setName("C270", c270_intf); 00058 host->registerDriver(dev, c270_intf, this, &USBHostC270::onDisconnect); 00059 int addr = dev->getAddress(); 00060 m_isoEp->init(addr, C270_EN, C270_MPS); 00061 uint8_t buf[26]; 00062 memset(buf, 0, sizeof(buf)); 00063 buf[2] = _formatIndex; 00064 buf[3] = _frameIndex; 00065 *reinterpret_cast<uint32_t*>(buf+4) = _interval; 00066 USB_TYPE res = Control(SET_CUR, VS_COMMIT_CONTROL, 1, buf, sizeof(buf)); 00067 if (res != USB_TYPE_OK) { 00068 C270_DBG("SET_CUR VS_COMMIT_CONTROL FAILED"); 00069 } 00070 res = setInterfaceAlternate(1, C270_IF_ALT); // alt=1 packet size = 192 00071 if (res != USB_TYPE_OK) { 00072 C270_DBG("SET_INTERFACE FAILED"); 00073 } 00074 for(int i = 0; i < 16; i++) { 00075 report_cc_count[i] = 0; 00076 report_ps_cc_count[i] = 0; 00077 } 00078 LPC_USB->HcControl |= OR_CONTROL_PLE; // PeriodicListEnable 00079 LPC_USB->HcControl |= OR_CONTROL_IE; // IsochronousEnable 00080 00081 dev_connected = true; 00082 return true; 00083 } 00084 } 00085 } 00086 init(); 00087 return false; 00088 } 00089 00090 void USBHostC270::onDisconnect() 00091 { 00092 C270_DBG("dev_connected: %d", dev_connected); 00093 if (dev_connected) { 00094 m_isoEp->disconnect(); 00095 init(); 00096 } 00097 } 00098 00099 /*virtual*/ void USBHostC270::setVidPid(uint16_t vid, uint16_t pid) 00100 { 00101 C270_DBG("vid:%04x,pid:%04x", vid, pid); 00102 if (vid == C270_VID && pid == C270_PID) { 00103 c270_vid_pid_found = true; 00104 } else { 00105 c270_vid_pid_found = false; 00106 } 00107 } 00108 00109 /*virtual*/ bool USBHostC270::parseInterface(uint8_t intf_nb, uint8_t intf_class, uint8_t intf_subclass, uint8_t intf_protocol) //Must return true if the interface should be parsed 00110 { 00111 C270_DBG("intf_nb=%d,intf_class=%02X,intf_subclass=%d,intf_protocol=%d", intf_nb, intf_class, intf_subclass, intf_protocol); 00112 if ((c270_intf == -1) && c270_vid_pid_found) { 00113 c270_intf = intf_nb; 00114 c270_vid_pid_found = false; 00115 c270_device_found = true; 00116 return true; 00117 } 00118 return false; 00119 } 00120 00121 /*virtual*/ bool USBHostC270::useEndpoint(uint8_t intf_nb, ENDPOINT_TYPE type, ENDPOINT_DIRECTION dir) //Must return true if the endpoint will be used 00122 { 00123 C270_DBG("intf_nb:%d,type:%d,dir:%d",intf_nb, type, dir); 00124 return false; 00125 } 00126 00127 #define SEQ_READ_IDOL 0 00128 #define SEQ_READ_EXEC 1 00129 #define SEQ_READ_DONE 2 00130 00131 int USBHostC270::readJPEG(uint8_t* buf, int size, int timeout_ms) { 00132 _buf = buf; 00133 _pos = 0; 00134 _size = size; 00135 _seq = SEQ_READ_IDOL; 00136 setOnResult(this, &USBHostC270::callback_motion_jpeg); 00137 Timer timeout_t; 00138 timeout_t.reset(); 00139 timeout_t.start(); 00140 while(timeout_t.read_ms() < timeout_ms && _seq != SEQ_READ_DONE) { 00141 poll(timeout_ms); 00142 } 00143 return _pos; 00144 } 00145 00146 /* virtual */ void USBHostC270::outputJPEG(uint8_t c, int status) { // from decodeMJPEG 00147 if (_seq == SEQ_READ_IDOL) { 00148 if (status == JPEG_START) { 00149 _pos = 0; 00150 _seq = SEQ_READ_EXEC; 00151 } 00152 } 00153 if (_seq == SEQ_READ_EXEC) { 00154 if (_pos < _size) { 00155 _buf[_pos++] = c; 00156 } 00157 if (status == JPEG_END) { 00158 _seq = SEQ_READ_DONE; 00159 } 00160 } 00161 } 00162 00163 void USBHostC270::callback_motion_jpeg(uint16_t frame, uint8_t* buf, int len) { 00164 inputPacket(buf, len); 00165 }
Generated on Mon Jul 18 2022 19:52:39 by
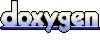