Library for the DS1721, 2-Wire Digital Thermometer and Thermostat from Dallas Semiconductor (Maxim Integrated)
DS1721.h
00001 /* 00002 * @file DS1721.h 00003 * @author Cameron Haegle 00004 * 00005 * @section LICENSE 00006 * 00007 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00008 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00009 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00010 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00011 * furnished to do so, subject to the following conditions: 00012 * 00013 * The above copyright notice and this permission notice shall be included in all copies or 00014 * substantial portions of the Software. 00015 * 00016 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00017 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00018 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00019 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * @section DESCRIPTION 00023 * 00024 * Library for the DS1721, 2-Wire Digital Thermometer and Thermostat, from Maxim Semiconductor 00025 * (www.maiximintegrated.com). 00026 * 00027 * @section LIMITATIONS 00028 * 00029 * This library was not written with for use with the mbed-RTOS, as the I2C read/write calls are 00030 * not thread safe. 00031 * 00032 * ChangeLog 00033 * 07/30/2013 00034 * - 00035 */ 00036 00037 #ifndef DS1721_H 00038 #define DS1721_H 00039 00040 #include "mbed.h" 00041 00042 // default DS1721 I2C address 00043 #define DS1721_ADDR 0x48 00044 00045 // DS1721 commands 00046 #define CMD_START_CONVT 0x51 00047 #define CMD_STOP_CONVT 0x22 00048 #define CMD_READ_TEMP 0xAA 00049 #define CMD_ACCESS_CFG 0xAC 00050 #define CMD_ACCESS_TH 0xA1 00051 #define CMD_ACCESS_TL 0xA2 00052 00053 // thermometer config register values 00054 #define CONV_FOREVER 0x00 00055 #define CONV_ONE_SHOT 0x01 00056 #define POLARITY_ACTIVE_LOW 0x00 00057 #define POLARITY_ACTIVE_HIGH 0x02 00058 #define RES_9_BIT 0x00 // 0000 00059 #define RES_10_BIT 0x04 // 0100 00060 #define RES_11_BIT 0x08 // 1000 00061 #define RES_12_BIT 0x0C // 1100 00062 00063 // DS1721 operating modes (HEAT = active low, Cool = active high) 00064 #define MODE_HEAT POLARITY_ACTIVE_LOW 00065 #define MODE_COOL POLARITY_ACTIVE_HIGH 00066 00067 00068 /** 00069 * !Library for the DS1721 temperature sensor. 00070 * The DS1721 is an I2C digital temperature sensor, with a range of -55C to +125C and a 0.125C resolution. 00071 * 00072 * @code 00073 * #include "mbed.h" 00074 * #include "DS1721.h" 00075 * 00076 * #define DS1721_I2C_ADDRESS (0x48<<1) 00077 * 00078 * int main(void) { 00079 * I2C m_i2c(PTC9, PTC8); 00080 * DS1721 thermo(m_i2c, DS1721_I2C_ADDRESS); 00081 * 00082 * // initialize the temperature sensor 00083 * thermo.startConversion(); 00084 * thermo.setLowSp(25); 00085 * thermo.setHighSp(27); 00086 * thermo.setPolarity(POLARITY_ACTIVE_HIGH); 00087 * 00088 * while (true) { 00089 * printf("Temp: %d\r\n", thermo.getTemp()); 00090 * } 00091 * } 00092 * @endcode 00093 **/ 00094 class DS1721 00095 { 00096 public: 00097 /** 00098 * DS1721 constructor 00099 * 00100 * @param &i2c pointer to i2c object 00101 * @param addr addr of the I2C peripheral default = (DS1721_ADDR<<1) 00102 **/ 00103 DS1721(I2C &i2c, int addr = (DS1721_ADDR<<1)); 00104 00105 /** 00106 * DS1721 constructor 00107 * 00108 * @param &i2c pointer to I2C object 00109 * @param resolution readout resolution of the theremometer (9, 10, 11, 12 bit) 00110 * @param polarity the state on which the thermostat output is enabled 00111 * @param mode the temperature conversion mode (single shot or continuous) 00112 * @param addr addr of the I2C peripheral default = (DS1721_ADDR<<1) 00113 **/ 00114 DS1721(I2C &i2c,int resolution, int polarity, int mode, int addr = (DS1721_ADDR<<1)); 00115 00116 /*! 00117 * DS1721 destructor 00118 */ 00119 ~DS1721 (); 00120 00121 /** 00122 * Reads the temperature from the DS1721 and converts it to a useable value. 00123 * 00124 * @returns the current temperature, as a float 00125 **/ 00126 float getTemp(void); 00127 00128 /** 00129 * Sets the temperature polarity (POL) bit of the sensor. This bit determines 00130 * upon which set point the theremostat output is active. 00131 * 00132 * @param int the new polarity value 00133 * @returns the result of the function (0 - Failure, 1 - Success) 00134 **/ 00135 int setPolarity(int polarity); 00136 00137 /** 00138 * Gets the temperature polarity (POL) bit of the sensor. This bit determines 00139 * upon which set point the theremostat output (Tout) is active. 00140 * 00141 * @returns the value of the POL bit (0 - active low, 1 - active high) 00142 **/ 00143 int getPolarity(void); 00144 00145 /** 00146 * Initiates a temperature conversion. If the DS1721 is configured for single shot mode 00147 * this function must be called prior to reading the temperature (re: getTemp()) 00148 * 00149 * @returns the result of the function (0 - Failure, 1 - Success) 00150 **/ 00151 int startConversion(void); 00152 00153 /** 00154 * Stops a temperature conversion. 00155 * 00156 * @returns the result of the function (0 - Failure, 1 - Success) 00157 **/ 00158 int stopConversion(void); 00159 00160 /** 00161 * Sets the configuration register of the DS1721. 00162 * 00163 * @param int the new configuration value (resolution | polarity | mode) 00164 * @returns the result of the function (0 - Failure, 1 - Success) 00165 **/ 00166 int setConfig(int config); 00167 00168 /** 00169 * Retrieves the configuration register of the DS1721. 00170 * 00171 * @returns 00172 **/ 00173 int getConfig(int* config); 00174 00175 /** 00176 * Retrieves the configured low temperature set point value. 00177 * 00178 * @returns the current low temperature set point value (degrees C) 00179 **/ 00180 float getLowSp(void); 00181 00182 /** 00183 * Sets the low temperature set point value. 00184 * 00185 * @param int the new low set point temperature (degrees C) 00186 * @returns the result of the function (0 - Failure, 1 - Success) 00187 **/ 00188 int setLowSp(float newSp); 00189 00190 /** 00191 * Retrieves the configured high temperature set point value. 00192 * 00193 * @returns the current high temperature set point value (degrees C) 00194 **/ 00195 float getHighSp(void); 00196 00197 /** 00198 * Sets the high temperature set point value. 00199 * 00200 * @param int the new high temperature set point value (degrees C) 00201 * @returns the result of the function (0 - Failure, 1 - Success) 00202 **/ 00203 int setHighSp(float newSp); 00204 00205 /* 00206 * Helper function to convert degrees Celcius to Fahrenheit 00207 * 00208 * @param temperature in degrees Celcius 00209 * @return temperature in degrees Fahrenheit 00210 */ 00211 float temp_CtoF(float tempC); 00212 00213 /* 00214 * Helper function to convert degrees Fahrenheit to Celcius 00215 * 00216 * @param temperature in degrees Fahrenheit 00217 * @return temperature in degrees Celcius 00218 */ 00219 float temp_FtoC(float tempF); 00220 00221 00222 private: 00223 I2C &m_i2c; 00224 int m_addr; 00225 int _resolution; 00226 int _polarity; 00227 int _mode; 00228 int _tl; 00229 int _num_read; 00230 int _th; 00231 00232 int writeData(char* data, int length); 00233 int readData(char* data, int length); 00234 }; 00235 00236 #endif 00237
Generated on Mon Jul 18 2022 17:03:36 by
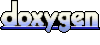