Library for the DS1721, 2-Wire Digital Thermometer and Thermostat from Dallas Semiconductor (Maxim Integrated)
DS1721.cpp
00001 /* 00002 * @file DS1721.cpp 00003 * @author Cameron Haegle 00004 * 00005 * @section LICENSE 00006 * 00007 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00008 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00009 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00010 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00011 * furnished to do so, subject to the following conditions: 00012 * 00013 * The above copyright notice and this permission notice shall be included in all copies or 00014 * substantial portions of the Software. 00015 * 00016 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00017 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00018 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00019 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * @section DESCRIPTION 00023 * 00024 * Library for the DS1721, 2-Wire Digital Thermometer and Thermostat, from Maxim Semiconductor 00025 * (www.maiximintegrated.com). 00026 * 00027 * @section LIMITATIONS 00028 * 00029 * This library was not written with for use with the mbed-RTOS, as the I2C read/write calls are 00030 * not thread safe. 00031 * 00032 * ChangeLog 00033 * 07/30/2013 00034 * - 00035 */ 00036 00037 00038 00039 #include "DS1721.h" 00040 00041 00042 DS1721::DS1721(I2C &i2c, int addr) : m_i2c(i2c) , m_addr(addr) 00043 { 00044 m_i2c.frequency(400000); 00045 _resolution = RES_9_BIT; 00046 _polarity = POLARITY_ACTIVE_HIGH; 00047 _mode = CONV_FOREVER; 00048 _num_read = 1; 00049 00050 //setConfig(_resolution | _polarity | _mode); 00051 } 00052 00053 DS1721::DS1721(I2C &i2c, int resolution, int polarity, int mode, int addr) 00054 : m_i2c(i2c) , _resolution(resolution) , _polarity(polarity) , _mode(mode) , m_addr(addr) 00055 { 00056 m_i2c.frequency(400000); 00057 _num_read = 1; 00058 //setConfig(_resolution | _polarity | _mode); 00059 } 00060 00061 DS1721::~DS1721 (void) 00062 { 00063 } 00064 00065 int DS1721::setConfig(int config) 00066 { 00067 char cmd[2]; 00068 cmd[0] = CMD_ACCESS_CFG; 00069 cmd[1] = (config & 0xFF); 00070 00071 if(writeData(cmd, 2) != 0) 00072 { 00073 return 1; 00074 } 00075 return 0; 00076 } 00077 00078 int DS1721::getConfig(int* config) 00079 { 00080 char cmd[2]; 00081 00082 cmd[0] = CMD_ACCESS_CFG; // 0xAC 00083 00084 if(writeData(cmd, 1) != 0) 00085 { 00086 return 1; 00087 } 00088 00089 if(readData(cmd, 1) != 0) 00090 { 00091 return 1; 00092 } 00093 *config = cmd[0]; 00094 return 0; 00095 } 00096 00097 00098 float DS1721::getTemp(void) 00099 { 00100 char cmd[3]; 00101 00102 cmd[0] = CMD_READ_TEMP; 00103 00104 // if this is the first read, since power up, perform the read twice 00105 // this eliminate an erroneous initial read. 00106 do 00107 { 00108 if(writeData(cmd, 1) != 0) 00109 { 00110 return 1; 00111 } 00112 00113 // read 1 byte, ignoring fractional portion of temperature 00114 if(readData(cmd, 2) != 0) 00115 { 00116 return 1; 00117 } 00118 _num_read--; 00119 }while(_num_read); 00120 00121 _num_read = 1; 00122 00123 return ((float)(cmd[1] + (cmd[0] << 8))/256); 00124 } 00125 00126 int DS1721::setPolarity(int polarity) 00127 { 00128 _polarity = polarity; 00129 return setConfig(_resolution | _polarity | _mode); 00130 } 00131 00132 int DS1721::getPolarity(void) 00133 { 00134 int config = 0; 00135 getConfig(&config); 00136 return ((config & (1<<1)) ? 1: 0); 00137 } 00138 00139 int DS1721::startConversion(void) 00140 { 00141 char cmd[1]; 00142 00143 cmd[0] = CMD_START_CONVT; // 0x51 00144 00145 if(writeData(cmd, 1) != 0) 00146 { 00147 return 0; 00148 } 00149 return 1; 00150 } 00151 00152 int DS1721::stopConversion(void) 00153 { 00154 char cmd[1]; 00155 00156 cmd[0] = CMD_STOP_CONVT; // 0x51 00157 00158 if(writeData(cmd, 1) != 0) 00159 { 00160 return 0; 00161 } 00162 return 1; 00163 } 00164 00165 float DS1721::getLowSp(void) 00166 { 00167 char cmd[4]; 00168 00169 cmd[0] = CMD_ACCESS_TL; 00170 00171 if(writeData(cmd, 1) != 0) 00172 { 00173 //return FALSE; 00174 } 00175 00176 // read back TL msb & lsb bytes 00177 if(readData(cmd, 2) != 0) 00178 { 00179 return 0; 00180 } 00181 00182 _tl = ((cmd[0] << 8) + cmd[1]); 00183 00184 return ((float)_tl/256); 00185 } 00186 00187 int DS1721::setLowSp(float newSp) 00188 { 00189 char cmd[3]; 00190 00191 _tl = (newSp*256); 00192 00193 cmd[0] = CMD_ACCESS_TL; 00194 cmd[1] = (char)(_tl >> 8); // temp MSB 00195 cmd[2] = (char)(_tl & 0x00FF); // temp LSB (+/-0.5) 00196 00197 if(writeData(cmd, 3) != 0) 00198 { 00199 return 0; 00200 } 00201 return 1; 00202 } 00203 00204 float DS1721::getHighSp(void) 00205 { 00206 char cmd[3]; 00207 00208 cmd[0] = CMD_ACCESS_TH; 00209 00210 if(writeData(cmd, 1) != 0) 00211 { 00212 //return 0; 00213 } 00214 // read back TL msb & lsb bytes 00215 if(readData(cmd, 2) != 0) 00216 { 00217 //return 0; 00218 } 00219 00220 _th = ((cmd[0] << 8) + cmd[1]); 00221 00222 return ((float)_th/256); 00223 } 00224 00225 int DS1721::setHighSp(float newSp) 00226 { 00227 char cmd[3]; 00228 00229 _th = (newSp * 256); 00230 00231 cmd[0] = CMD_ACCESS_TH; 00232 cmd[1] = (char)(_th >> 8); // setpoint MSB 00233 cmd[2] = (char)(_th & 0x00FF); // setpoint LSB (+/-0.5) 00234 00235 if(writeData(cmd, 3) != 0) 00236 { 00237 return 0; 00238 } 00239 return 1; 00240 } 00241 00242 float DS1721::temp_CtoF(float tempC) 00243 { 00244 return ((tempC * 1.8) + 32); 00245 } 00246 00247 float DS1721::temp_FtoC(float tempF) 00248 { 00249 return ((tempF - 32)* 0.55); 00250 } 00251 00252 int DS1721::writeData(char* data, int length) 00253 { 00254 return (m_i2c.write(m_addr, data, length)); 00255 } 00256 00257 int DS1721::readData(char* data, int length) 00258 { 00259 return (m_i2c.read(m_addr, data, length)); 00260 }
Generated on Mon Jul 18 2022 17:03:36 by
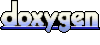