Christian Dupaty 03/2021 Library and demo for BMM150 see datasheet here : https://www.bosch-sensortec.com/products/motion-sensors/magnetometers-bmm150/ Adaptation of BOSCH driver https://github.com/BoschSensortec/BMM150-Sensor-API for ARM MBED and tested on NUCLEO-L073RZ and GEOMAGNETIC CLICK https://www.mikroe.com/geomagnetic-click
Dependents: BMM150_HelloWorld2
bmm150_defs.h
00001 /* 00002 Christian Dupaty 03/2021 00003 Library and demo for BMM150 see datasheet here : https://www.bosch-sensortec.com/products/motion-sensors/magnetometers-bmm150/ 00004 Adaptation of BOSCH driver https://github.com/BoschSensortec/BMM150-Sensor-API 00005 for ARM MBED and tested on NUCLEO-L073RZ and GEOMAGNETIC CLICK 00006 https://www.mikroe.com/geomagnetic-click 00007 */ 00008 00009 #ifndef _BMM150_DEFS_H_ 00010 #define _BMM150_DEFS_H_ 00011 00012 /**\name Macro definitions */ 00013 /* 00014 typedef unsigned int unsigned int; 00015 typedef int int; 00016 typedef unsigned char unsigned char; 00017 typedef char char; 00018 */ 00019 /**\name API success code */ 00020 #define BMM150_OK (0) 00021 00022 /**\name API error codes */ 00023 #define BMM150_E_ID_NOT_CONFORM (-1) 00024 #define BMM150_E_INVALID_CONFIG (-2) 00025 // #define BMM150_E_ID_WRONG (-3) 00026 00027 /**\name API warning codes */ 00028 #define BMM150_W_NORMAL_SELF_TEST_YZ_FAIL INT8_C(1) 00029 #define BMM150_W_NORMAL_SELF_TEST_XZ_FAIL INT8_C(2) 00030 #define BMM150_W_NORMAL_SELF_TEST_Z_FAIL INT8_C(3) 00031 #define BMM150_W_NORMAL_SELF_TEST_XY_FAIL INT8_C(4) 00032 #define BMM150_W_NORMAL_SELF_TEST_Y_FAIL INT8_C(5) 00033 #define BMM150_W_NORMAL_SELF_TEST_X_FAIL INT8_C(6) 00034 #define BMM150_W_NORMAL_SELF_TEST_XYZ_FAIL INT8_C(7) 00035 #define BMM150_W_ADV_SELF_TEST_FAIL INT8_C(8) 00036 00037 #define BMM150_I2C_Address (0x13 << 1) 00038 #define i2cWadr (BMM150_I2C_Address<<1) 00039 #define i2cRadr (BMM150_I2C_Address<<1)+1 00040 #define ACK 1 00041 #define NACK 0 00042 00043 /**\name CHIP ID & SOFT RESET VALUES */ 00044 #define BMM150_CHIP_ID 0x32 00045 #define BMM150_SET_SOFT_RESET 0x82 00046 00047 /**\name POWER MODE DEFINTIONS */ 00048 #define BMM150_NORMAL_MODE 0x00 00049 #define BMM150_FORCED_MODE 0x01 00050 #define BMM150_SLEEP_MODE 0x03 00051 #define BMM150_SUSPEND_MODE 0x04 00052 00053 /**\name PRESET MODE DEFINITIONS */ 00054 #define BMM150_PRESETMODE_LOWPOWER 0x01 00055 #define BMM150_PRESETMODE_REGULAR 0x02 00056 #define BMM150_PRESETMODE_HIGHACCURACY 0x03 00057 #define BMM150_PRESETMODE_ENHANCED 0x04 00058 00059 /**\name Power mode settings */ 00060 #define BMM150_POWER_CNTRL_DISABLE 0x00 00061 #define BMM150_POWER_CNTRL_ENABLE 0x01 00062 00063 /**\name Sensor delay time settings */ 00064 #define BMM150_SOFT_RESET_wait_ms (1) 00065 #define BMM150_SOFT_RESET_DELAY (1) 00066 #define BMM150_NORMAL_SELF_TEST_DELAY (2) 00067 #define BMM150_START_UP_TIME (3) 00068 #define BMM150_ADV_SELF_TEST_DELAY (4) 00069 00070 /**\name ENABLE/DISABLE DEFINITIONS */ 00071 #define BMM150_XY_CHANNEL_ENABLE 0x00 00072 #define BMM150_XY_CHANNEL_DISABLE 0x03 00073 00074 /**\name Register Address */ 00075 #define BMM150_CHIP_ID_ADDR 0x40 00076 #define BMM150_DATA_X_LSB 0x42 00077 #define BMM150_DATA_X_MSB 0x43 00078 #define BMM150_DATA_Y_LSB 0x44 00079 #define BMM150_DATA_Y_MSB 0x45 00080 #define BMM150_DATA_Z_LSB 0x46 00081 #define BMM150_DATA_Z_MSB 0x47 00082 #define BMM150_DATA_READY_STATUS 0x48 00083 #define BMM150_INTERRUPT_STATUS 0x4A 00084 #define BMM150_POWER_CONTROL_ADDR 0x4B 00085 #define BMM150_OP_MODE_ADDR 0x4C 00086 #define BMM150_INT_CONFIG_ADDR 0x4D 00087 #define BMM150_AXES_ENABLE_ADDR 0x4E 00088 #define BMM150_LOW_THRESHOLD_ADDR 0x4F 00089 #define BMM150_HIGH_THRESHOLD_ADDR 0x50 00090 #define BMM150_REP_XY_ADDR 0x51 00091 #define BMM150_REP_Z_ADDR 0x52 00092 00093 /**\name DATA RATE DEFINITIONS */ 00094 #define BMM150_DATA_RATE_10HZ (0x00) 00095 #define BMM150_DATA_RATE_02HZ (0x01) 00096 #define BMM150_DATA_RATE_06HZ (0x02) 00097 #define BMM150_DATA_RATE_08HZ (0x03) 00098 #define BMM150_DATA_RATE_15HZ (0x04) 00099 #define BMM150_DATA_RATE_20HZ (0x05) 00100 #define BMM150_DATA_RATE_25HZ (0x06) 00101 #define BMM150_DATA_RATE_30HZ (0x07) 00102 00103 /**\name TRIM REGISTERS */ 00104 /* Trim Extended Registers */ 00105 #define BMM150_DIG_X1 uint8_t(0x5D) 00106 #define BMM150_DIG_Y1 uint8_t(0x5E) 00107 #define BMM150_DIG_Z4_LSB uint8_t(0x62) 00108 #define BMM150_DIG_Z4_MSB uint8_t(0x63) 00109 #define BMM150_DIG_X2 uint8_t(0x64) 00110 #define BMM150_DIG_Y2 uint8_t(0x65) 00111 #define BMM150_DIG_Z2_LSB uint8_t(0x68) 00112 #define BMM150_DIG_Z2_MSB uint8_t(0x69) 00113 #define BMM150_DIG_Z1_LSB uint8_t(0x6A) 00114 #define BMM150_DIG_Z1_MSB uint8_t(0x6B) 00115 #define BMM150_DIG_XYZ1_LSB uint8_t(0x6C) 00116 #define BMM150_DIG_XYZ1_MSB uint8_t(0x6D) 00117 #define BMM150_DIG_Z3_LSB uint8_t(0x6E) 00118 #define BMM150_DIG_Z3_MSB uint8_t(0x6F) 00119 #define BMM150_DIG_XY2 uint8_t(0x70) 00120 #define BMM150_DIG_XY1 uint8_t(0x71) 00121 00122 /**\name PRESET MODES - REPETITIONS-XY RATES */ 00123 #define BMM150_LOWPOWER_REPXY (1) 00124 #define BMM150_REGULAR_REPXY (4) 00125 #define BMM150_ENHANCED_REPXY (7) 00126 #define BMM150_HIGHACCURACY_REPXY (23) 00127 00128 /**\name PRESET MODES - REPETITIONS-Z RATES */ 00129 #define BMM150_LOWPOWER_REPZ (2) 00130 #define BMM150_REGULAR_REPZ (14) 00131 #define BMM150_ENHANCED_REPZ (26) 00132 #define BMM150_HIGHACCURACY_REPZ (82) 00133 00134 /**\name Macros for bit masking */ 00135 #define BMM150_PWR_CNTRL_MSK (0x01) 00136 00137 #define BMM150_CONTROL_MEASURE_MSK (0x38) 00138 #define BMM150_CONTROL_MEASURE_POS (0x03) 00139 00140 #define BMM150_POWER_CONTROL_BIT_MSK (0x01) 00141 #define BMM150_POWER_CONTROL_BIT_POS (0x00) 00142 00143 #define BMM150_OP_MODE_MSK (0x06) 00144 #define BMM150_OP_MODE_POS (0x01) 00145 00146 #define BMM150_ODR_MSK (0x38) 00147 #define BMM150_ODR_POS (0x03) 00148 00149 #define BMM150_DATA_X_MSK (0xF8) 00150 #define BMM150_DATA_X_POS (0x03) 00151 00152 #define BMM150_DATA_Y_MSK (0xF8) 00153 #define BMM150_DATA_Y_POS (0x03) 00154 00155 #define BMM150_DATA_Z_MSK (0xFE) 00156 #define BMM150_DATA_Z_POS (0x01) 00157 00158 #define BMM150_DATA_RHALL_MSK (0xFC) 00159 #define BMM150_DATA_RHALL_POS (0x02) 00160 00161 #define BMM150_SELF_TEST_MSK (0x01) 00162 00163 #define BMM150_ADV_SELF_TEST_MSK (0xC0) 00164 #define BMM150_ADV_SELF_TEST_POS (0x06) 00165 00166 #define BMM150_DRDY_EN_MSK (0x80) 00167 #define BMM150_DRDY_EN_POS (0x07) 00168 00169 #define BMM150_INT_PIN_EN_MSK (0x40) 00170 #define BMM150_INT_PIN_EN_POS (0x06) 00171 00172 #define BMM150_DRDY_POLARITY_MSK (0x04) 00173 #define BMM150_DRDY_POLARITY_POS (0x02) 00174 00175 #define BMM150_INT_LATCH_MSK (0x02) 00176 #define BMM150_INT_LATCH_POS (0x01) 00177 00178 #define BMM150_INT_POLARITY_MSK (0x01) 00179 00180 #define BMM150_DATA_OVERRUN_INT_MSK (0x80) 00181 #define BMM150_DATA_OVERRUN_INT_POS (0x07) 00182 00183 #define BMM150_OVERFLOW_INT_MSK (0x40) 00184 #define BMM150_OVERFLOW_INT_POS (0x06) 00185 00186 #define BMM150_HIGH_THRESHOLD_INT_MSK (0x38) 00187 #define BMM150_HIGH_THRESHOLD_INT_POS (0x03) 00188 00189 #define BMM150_LOW_THRESHOLD_INT_MSK (0x07) 00190 00191 #define BMM150_DRDY_STATUS_MSK (0x01) 00192 00193 /**\name OVERFLOW DEFINITIONS */ 00194 #define BMM150_XYAXES_FLIP_OVERFLOW_ADCVAL (-4096) 00195 #define BMM150_ZAXIS_HALL_OVERFLOW_ADCVAL (-16384) 00196 #define BMM150_OVERFLOW_OUTPUT (-32768) 00197 #define BMM150_NEGATIVE_SATURATION_Z (-32767) 00198 #define BMM150_POSITIVE_SATURATION_Z (32767) 00199 #ifdef BMM150_USE_FLOATING_POINT 00200 #define BMM150_OVERFLOW_OUTPUT_FLOAT 0.0f 00201 #endif 00202 00203 /**\name Register read lengths */ 00204 #define BMM150_SELF_TEST_LEN (5) 00205 #define BMM150_SETTING_DATA_LEN (8) 00206 #define BMM150_XYZR_DATA_LEN (8) 00207 00208 /**\name Self test selection macros */ 00209 #define BMM150_NORMAL_SELF_TEST (0) 00210 #define BMM150_ADVANCED_SELF_TEST (1) 00211 00212 /**\name Self test settings */ 00213 #define BMM150_DISABLE_XY_AXIS (0x03) 00214 #define BMM150_SELF_TEST_REP_Z (0x04) 00215 00216 /**\name Advanced self-test current settings */ 00217 #define BMM150_DISABLE_SELF_TEST_CURRENT (0x00) 00218 #define BMM150_ENABLE_NEGATIVE_CURRENT (0x02) 00219 #define BMM150_ENABLE_POSITIVE_CURRENT (0x03) 00220 00221 /**\name Normal self-test status */ 00222 #define BMM150_SELF_TEST_STATUS_XYZ_FAIL (0x00) 00223 #define BMM150_SELF_TEST_STATUS_SUCCESS (0x07) 00224 00225 /**\name Macro to SET and GET BITS of a register*/ 00226 #define BMM150_SET_BITS(reg_data, bitname, data) \ 00227 ((reg_data & ~(bitname##_MSK)) | \ 00228 ((data << bitname##_POS) & bitname##_MSK)) 00229 00230 #define BMM150_GET_BITS(reg_data, bitname) ((reg_data & (bitname##_MSK)) >> \ 00231 (bitname##_POS)) 00232 00233 #define BMM150_SET_BITS_POS_0(reg_data, bitname, data) \ 00234 ((reg_data & ~(bitname##_MSK)) | \ 00235 (data & bitname##_MSK)) 00236 00237 #define BMM150_GET_BITS_POS_0(reg_data, bitname) (reg_data & (bitname##_MSK)) 00238 00239 00240 struct bmm150_mag_data { 00241 int x; 00242 int y; 00243 int z; 00244 }; 00245 00246 /* 00247 @brief bmm150 un-compensated (raw) magnetometer data 00248 */ 00249 struct bmm150_raw_mag_data { 00250 /*! Raw mag X data */ 00251 int raw_datax; 00252 /*! Raw mag Y data */ 00253 int raw_datay; 00254 /*! Raw mag Z data */ 00255 int raw_dataz; 00256 /*! Raw mag resistance value */ 00257 unsigned int raw_data_r; 00258 }; 00259 00260 /*! 00261 @brief bmm150 trim data structure 00262 */ 00263 struct bmm150_trim_registers { 00264 /*! trim x1 data */ 00265 char dig_x1 ; 00266 /*! trim y1 data */ 00267 char dig_y1 ; 00268 /*! trim x2 data */ 00269 char dig_x2 ; 00270 /*! trim y2 data */ 00271 char dig_y2 ; 00272 /*! trim z1 data */ 00273 unsigned int dig_z1 ; 00274 /*! trim z2 data */ 00275 int dig_z2 ; 00276 /*! trim z3 data */ 00277 int dig_z3 ; 00278 /*! trim z4 data */ 00279 int dig_z4 ; 00280 /*! trim xy1 data */ 00281 unsigned char dig_xy1 ; 00282 /*! trim xy2 data */ 00283 char dig_xy2 ; 00284 /*! trim xyz1 data */ 00285 unsigned int dig_xyz1 ; 00286 }; 00287 00288 /** 00289 @brief bmm150 sensor settings 00290 */ 00291 struct bmm150_settings { 00292 /*! Control measurement of XYZ axes */ 00293 unsigned char xyz_axes_control ; 00294 /*! Power control bit value */ 00295 unsigned char pwr_cntrl_bit ; 00296 /*! Power control bit value */ 00297 unsigned char pwr_mode ; 00298 /*! Data rate value (ODR) */ 00299 unsigned char data_rate ; 00300 /*! XY Repetitions */ 00301 unsigned char xy_rep ; 00302 /*! Z Repetitions */ 00303 unsigned char z_rep ; 00304 /*! Preset mode of sensor */ 00305 unsigned char preset_mode ; 00306 /*! Interrupt configuration settings */ 00307 // struct bmm150_int_ctrl_settings int_settings; 00308 }; 00309 00310 #endif
Generated on Sat Jul 16 2022 16:40:58 by
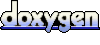