
Simple demo with GPIO MQTT protocol test on STM32 broker tests.mosquitto.org WIFI interface ESP8266 Issue of topic0 by pressing the button If reception of ', switching of the led If received from 'q' end of program
MQTTNetwork.h
00001 #ifndef _MQTTNETWORK_H_ 00002 #define _MQTTNETWORK_H_ 00003 00004 #include "NetworkInterface.h" 00005 00006 class MQTTNetwork { 00007 public: 00008 MQTTNetwork(NetworkInterface* aNetwork) : network(aNetwork) { 00009 socket = new TCPSocket(); 00010 } 00011 00012 ~MQTTNetwork() { 00013 delete socket; 00014 } 00015 00016 int read(unsigned char* buffer, int len, int timeout) { 00017 return socket->recv(buffer, len); 00018 } 00019 00020 int write(unsigned char* buffer, int len, int timeout) { 00021 return socket->send(buffer, len); 00022 } 00023 00024 int connect(const char* hostname, int port) { 00025 socket->open(network); 00026 return socket->connect(hostname, port); 00027 } 00028 00029 int disconnect() { 00030 return socket->close(); 00031 } 00032 00033 private: 00034 NetworkInterface* network; 00035 TCPSocket* socket; 00036 }; 00037 00038 #endif // _MQTTNETWORK_H_
Generated on Fri Jul 22 2022 15:58:27 by
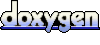