20171208
Fork of LoRaWAN-lib by
Embed:
(wiki syntax)
Show/hide line numbers
LoRaMac.h
Go to the documentation of this file.
00001 /*! 00002 * \file LoRaMac.h 00003 * 00004 * \brief LoRa MAC layer implementation 00005 * 00006 * \copyright Revised BSD License, see section \ref LICENSE. 00007 * 00008 * \code 00009 * ______ _ 00010 * / _____) _ | | 00011 * ( (____ _____ ____ _| |_ _____ ____| |__ 00012 * \____ \| ___ | (_ _) ___ |/ ___) _ \ 00013 * _____) ) ____| | | || |_| ____( (___| | | | 00014 * (______/|_____)_|_|_| \__)_____)\____)_| |_| 00015 * (C)2013 Semtech 00016 * 00017 * ___ _____ _ ___ _ _____ ___ ___ ___ ___ 00018 * / __|_ _/_\ / __| |/ / __/ _ \| _ \/ __| __| 00019 * \__ \ | |/ _ \ (__| ' <| _| (_) | / (__| _| 00020 * |___/ |_/_/ \_\___|_|\_\_| \___/|_|_\\___|___| 00021 * embedded.connectivity.solutions=============== 00022 * 00023 * \endcode 00024 * 00025 * \author Miguel Luis ( Semtech ) 00026 * 00027 * \author Gregory Cristian ( Semtech ) 00028 * 00029 * \author Daniel Jäckle ( STACKFORCE ) 00030 * 00031 * \defgroup LORAMAC LoRa MAC layer implementation 00032 * This module specifies the API implementation of the LoRaMAC layer. 00033 * This is a placeholder for a detailed description of the LoRaMac 00034 * layer and the supported features. 00035 * \{ 00036 * 00037 * \example classA/LoRaMote/main.c 00038 * LoRaWAN class A application example for the LoRaMote. 00039 * 00040 * \example classB/LoRaMote/main.c 00041 * LoRaWAN class B application example for the LoRaMote. 00042 * 00043 * \example classC/LoRaMote/main.c 00044 * LoRaWAN class C application example for the LoRaMote. 00045 */ 00046 #ifndef __LORAMAC_H__ 00047 #define __LORAMAC_H__ 00048 00049 // Includes board dependent definitions such as channels frequencies 00050 #include "LoRaMac-definitions.h" 00051 00052 /*! 00053 * Beacon interval in ms 00054 */ 00055 #define BEACON_INTERVAL 128000 00056 00057 /*! 00058 * Class A&B receive delay 1 in ms 00059 */ 00060 #define RECEIVE_DELAY1 1000 00061 00062 /*! 00063 * Class A&B receive delay 2 in ms 00064 */ 00065 #define RECEIVE_DELAY2 2000 00066 00067 /*! 00068 * Join accept receive delay 1 in ms 00069 */ 00070 #define JOIN_ACCEPT_DELAY1 5000 00071 00072 /*! 00073 * Join accept receive delay 2 in ms 00074 */ 00075 #define JOIN_ACCEPT_DELAY2 6000 00076 00077 /*! 00078 * Class A&B maximum receive window delay in ms 00079 */ 00080 #define MAX_RX_WINDOW 3000 00081 00082 /*! 00083 * Maximum allowed gap for the FCNT field 00084 */ 00085 #define MAX_FCNT_GAP 16384 00086 00087 /*! 00088 * ADR acknowledgement counter limit 00089 */ 00090 #define ADR_ACK_LIMIT 64 00091 00092 /*! 00093 * Number of ADR acknowledgement requests before returning to default datarate 00094 */ 00095 #define ADR_ACK_DELAY 32 00096 00097 /*! 00098 * Number of seconds after the start of the second reception window without 00099 * receiving an acknowledge. 00100 * AckTimeout = \ref ACK_TIMEOUT + Random( -\ref ACK_TIMEOUT_RND, \ref ACK_TIMEOUT_RND ) 00101 */ 00102 #define ACK_TIMEOUT 2000 00103 00104 /*! 00105 * Random number of seconds after the start of the second reception window without 00106 * receiving an acknowledge 00107 * AckTimeout = \ref ACK_TIMEOUT + Random( -\ref ACK_TIMEOUT_RND, \ref ACK_TIMEOUT_RND ) 00108 */ 00109 #define ACK_TIMEOUT_RND 1000 00110 00111 /*! 00112 * Check the Mac layer state every MAC_STATE_CHECK_TIMEOUT in ms 00113 */ 00114 #define MAC_STATE_CHECK_TIMEOUT 1000 00115 00116 /*! 00117 * Maximum number of times the MAC layer tries to get an acknowledge. 00118 */ 00119 #define MAX_ACK_RETRIES 8 00120 00121 /*! 00122 * RSSI free threshold [dBm] 00123 */ 00124 #define RSSI_FREE_TH ( int8_t )( -90 ) 00125 00126 /*! 00127 * Frame direction definition for up-link communications 00128 */ 00129 #define UP_LINK 0 00130 00131 /*! 00132 * Frame direction definition for down-link communications 00133 */ 00134 #define DOWN_LINK 1 00135 00136 /*! 00137 * Sets the length of the LoRaMAC footer field. 00138 * Mainly indicates the MIC field length 00139 */ 00140 #define LORAMAC_MFR_LEN 4 00141 00142 /*! 00143 * LoRaWAN devices classes definition 00144 */ 00145 typedef enum eDeviceClass 00146 { 00147 /*! 00148 * LoRaWAN device class A 00149 * 00150 * LoRaWAN Specification V1.0.1, chapter 3ff 00151 */ 00152 CLASS_A , 00153 /*! 00154 * LoRaWAN device class B 00155 * 00156 * LoRaWAN Specification V1.0.1, chapter 8ff 00157 */ 00158 CLASS_B , 00159 /*! 00160 * LoRaWAN device class C 00161 * 00162 * LoRaWAN Specification V1.0.1, chapter 17ff 00163 */ 00164 CLASS_C , 00165 }DeviceClass_t ; 00166 00167 /*! 00168 * LoRaMAC channels parameters definition 00169 */ 00170 typedef union uDrRange 00171 { 00172 /*! 00173 * Byte-access to the bits 00174 */ 00175 int8_t Value ; 00176 /*! 00177 * Structure to store the minimum and the maximum datarate 00178 */ 00179 struct sFields 00180 { 00181 /*! 00182 * Minimum data rate 00183 * 00184 * EU868 - [DR_0, DR_1, DR_2, DR_3, DR_4, DR_5, DR_6, DR_7] 00185 * 00186 * US915 - [DR_0, DR_1, DR_2, DR_3, DR_4] 00187 */ 00188 int8_t Min : 4; 00189 /*! 00190 * Maximum data rate 00191 * 00192 * EU868 - [DR_0, DR_1, DR_2, DR_3, DR_4, DR_5, DR_6, DR_7] 00193 * 00194 * US915 - [DR_0, DR_1, DR_2, DR_3, DR_4] 00195 */ 00196 int8_t Max : 4; 00197 }Fields; 00198 }DrRange_t ; 00199 00200 /*! 00201 * LoRaMAC band parameters definition 00202 */ 00203 typedef struct sBand 00204 { 00205 /*! 00206 * Duty cycle 00207 */ 00208 uint16_t DCycle ; 00209 /*! 00210 * Maximum Tx power 00211 */ 00212 int8_t TxMaxPower ; 00213 /*! 00214 * Time stamp of the last Tx frame 00215 */ 00216 TimerTime_t LastTxDoneTime ; 00217 /*! 00218 * Holds the time where the device is off 00219 */ 00220 TimerTime_t TimeOff ; 00221 }Band_t ; 00222 00223 /*! 00224 * LoRaMAC channel definition 00225 */ 00226 typedef struct sChannelParams 00227 { 00228 /*! 00229 * Frequency in Hz 00230 */ 00231 uint32_t Frequency ; 00232 /*! 00233 * Data rate definition 00234 */ 00235 DrRange_t DrRange ; 00236 /*! 00237 * Band index 00238 */ 00239 uint8_t Band ; 00240 }ChannelParams_t ; 00241 00242 /*! 00243 * LoRaMAC receive window 2 channel parameters 00244 */ 00245 typedef struct sRx2ChannelParams 00246 { 00247 /*! 00248 * Frequency in Hz 00249 */ 00250 uint32_t Frequency ; 00251 /*! 00252 * Data rate 00253 * 00254 * EU868 - [DR_0, DR_1, DR_2, DR_3, DR_4, DR_5, DR_6, DR_7] 00255 * 00256 * US915 - [DR_8, DR_9, DR_10, DR_11, DR_12, DR_13] 00257 */ 00258 uint8_t Datarate ; 00259 }Rx2ChannelParams_t ; 00260 00261 /*! 00262 * Global MAC layer parameters 00263 */ 00264 typedef struct sLoRaMacParams 00265 { 00266 /*! 00267 * Channels TX power 00268 */ 00269 int8_t ChannelsTxPower ; 00270 /*! 00271 * Channels data rate 00272 */ 00273 int8_t ChannelsDatarate ; 00274 /*! 00275 * System overall timing error in milliseconds. 00276 * [-SystemMaxRxError : +SystemMaxRxError] 00277 * Default: +/-10 ms 00278 */ 00279 uint32_t SystemMaxRxError ; 00280 /*! 00281 * Minimum required number of symbols to detect an Rx frame 00282 * Default: 6 symbols 00283 */ 00284 uint8_t MinRxSymbols ; 00285 /*! 00286 * LoRaMac maximum time a reception window stays open 00287 */ 00288 uint32_t MaxRxWindow ; 00289 /*! 00290 * Receive delay 1 00291 */ 00292 uint32_t ReceiveDelay1 ; 00293 /*! 00294 * Receive delay 2 00295 */ 00296 uint32_t ReceiveDelay2 ; 00297 /*! 00298 * Join accept delay 1 00299 */ 00300 uint32_t JoinAcceptDelay1 ; 00301 /*! 00302 * Join accept delay 1 00303 */ 00304 uint32_t JoinAcceptDelay2 ; 00305 /*! 00306 * Number of uplink messages repetitions [1:15] (unconfirmed messages only) 00307 */ 00308 uint8_t ChannelsNbRep ; 00309 /*! 00310 * Datarate offset between uplink and downlink on first window 00311 */ 00312 uint8_t Rx1DrOffset ; 00313 /*! 00314 * LoRaMAC 2nd reception window settings 00315 */ 00316 Rx2ChannelParams_t Rx2Channel ; 00317 /*! 00318 * Mask indicating which channels are enabled 00319 */ 00320 uint16_t ChannelsMask [6]; 00321 }LoRaMacParams_t ; 00322 00323 /*! 00324 * LoRaMAC multicast channel parameter 00325 */ 00326 typedef struct sMulticastParams 00327 { 00328 /*! 00329 * Address 00330 */ 00331 uint32_t Address ; 00332 /*! 00333 * Network session key 00334 */ 00335 uint8_t NwkSKey [16]; 00336 /*! 00337 * Application session key 00338 */ 00339 uint8_t AppSKey [16]; 00340 /*! 00341 * Downlink counter 00342 */ 00343 uint32_t DownLinkCounter ; 00344 /*! 00345 * Reference pointer to the next multicast channel parameters in the list 00346 */ 00347 struct sMulticastParams *Next ; 00348 }MulticastParams_t ; 00349 00350 /*! 00351 * LoRaMAC frame types 00352 * 00353 * LoRaWAN Specification V1.0.1, chapter 4.2.1, table 1 00354 */ 00355 typedef enum eLoRaMacFrameType 00356 { 00357 /*! 00358 * LoRaMAC join request frame 00359 */ 00360 FRAME_TYPE_JOIN_REQ = 0x00, 00361 /*! 00362 * LoRaMAC join accept frame 00363 */ 00364 FRAME_TYPE_JOIN_ACCEPT = 0x01, 00365 /*! 00366 * LoRaMAC unconfirmed up-link frame 00367 */ 00368 FRAME_TYPE_DATA_UNCONFIRMED_UP = 0x02, 00369 /*! 00370 * LoRaMAC unconfirmed down-link frame 00371 */ 00372 FRAME_TYPE_DATA_UNCONFIRMED_DOWN = 0x03, 00373 /*! 00374 * LoRaMAC confirmed up-link frame 00375 */ 00376 FRAME_TYPE_DATA_CONFIRMED_UP = 0x04, 00377 /*! 00378 * LoRaMAC confirmed down-link frame 00379 */ 00380 FRAME_TYPE_DATA_CONFIRMED_DOWN = 0x05, 00381 /*! 00382 * LoRaMAC RFU frame 00383 */ 00384 FRAME_TYPE_RFU = 0x06, 00385 /*! 00386 * LoRaMAC proprietary frame 00387 */ 00388 FRAME_TYPE_PROPRIETARY = 0x07, 00389 }LoRaMacFrameType_t ; 00390 00391 /*! 00392 * LoRaMAC mote MAC commands 00393 * 00394 * LoRaWAN Specification V1.0.1, chapter 5, table 4 00395 */ 00396 typedef enum eLoRaMacMoteCmd 00397 { 00398 /*! 00399 * LinkCheckReq 00400 */ 00401 MOTE_MAC_LINK_CHECK_REQ = 0x02, 00402 /*! 00403 * LinkADRAns 00404 */ 00405 MOTE_MAC_LINK_ADR_ANS = 0x03, 00406 /*! 00407 * DutyCycleAns 00408 */ 00409 MOTE_MAC_DUTY_CYCLE_ANS = 0x04, 00410 /*! 00411 * RXParamSetupAns 00412 */ 00413 MOTE_MAC_RX_PARAM_SETUP_ANS = 0x05, 00414 /*! 00415 * DevStatusAns 00416 */ 00417 MOTE_MAC_DEV_STATUS_ANS = 0x06, 00418 /*! 00419 * NewChannelAns 00420 */ 00421 MOTE_MAC_NEW_CHANNEL_ANS = 0x07, 00422 /*! 00423 * RXTimingSetupAns 00424 */ 00425 MOTE_MAC_RX_TIMING_SETUP_ANS = 0x08, 00426 }LoRaMacMoteCmd_t ; 00427 00428 /*! 00429 * LoRaMAC server MAC commands 00430 * 00431 * LoRaWAN Specification V1.0.1 chapter 5, table 4 00432 */ 00433 typedef enum eLoRaMacSrvCmd 00434 { 00435 /*! 00436 * LinkCheckAns 00437 */ 00438 SRV_MAC_LINK_CHECK_ANS = 0x02, 00439 /*! 00440 * LinkADRReq 00441 */ 00442 SRV_MAC_LINK_ADR_REQ = 0x03, 00443 /*! 00444 * DutyCycleReq 00445 */ 00446 SRV_MAC_DUTY_CYCLE_REQ = 0x04, 00447 /*! 00448 * RXParamSetupReq 00449 */ 00450 SRV_MAC_RX_PARAM_SETUP_REQ = 0x05, 00451 /*! 00452 * DevStatusReq 00453 */ 00454 SRV_MAC_DEV_STATUS_REQ = 0x06, 00455 /*! 00456 * NewChannelReq 00457 */ 00458 SRV_MAC_NEW_CHANNEL_REQ = 0x07, 00459 /*! 00460 * RXTimingSetupReq 00461 */ 00462 SRV_MAC_RX_TIMING_SETUP_REQ = 0x08, 00463 }LoRaMacSrvCmd_t ; 00464 00465 /*! 00466 * LoRaMAC Battery level indicator 00467 */ 00468 typedef enum eLoRaMacBatteryLevel 00469 { 00470 /*! 00471 * External power source 00472 */ 00473 BAT_LEVEL_EXT_SRC = 0x00, 00474 /*! 00475 * Battery level empty 00476 */ 00477 BAT_LEVEL_EMPTY = 0x01, 00478 /*! 00479 * Battery level full 00480 */ 00481 BAT_LEVEL_FULL = 0xFE, 00482 /*! 00483 * Battery level - no measurement available 00484 */ 00485 BAT_LEVEL_NO_MEASURE = 0xFF, 00486 }LoRaMacBatteryLevel_t ; 00487 00488 /*! 00489 * LoRaMAC header field definition (MHDR field) 00490 * 00491 * LoRaWAN Specification V1.0.1, chapter 4.2 00492 */ 00493 typedef union uLoRaMacHeader 00494 { 00495 /*! 00496 * Byte-access to the bits 00497 */ 00498 uint8_t Value ; 00499 /*! 00500 * Structure containing single access to header bits 00501 */ 00502 struct sHdrBits 00503 { 00504 /*! 00505 * Major version 00506 */ 00507 uint8_t Major : 2; 00508 /*! 00509 * RFU 00510 */ 00511 uint8_t RFU : 3; 00512 /*! 00513 * Message type 00514 */ 00515 uint8_t MType : 3; 00516 }Bits; 00517 }LoRaMacHeader_t ; 00518 00519 /*! 00520 * LoRaMAC frame control field definition (FCtrl) 00521 * 00522 * LoRaWAN Specification V1.0.1, chapter 4.3.1 00523 */ 00524 typedef union uLoRaMacFrameCtrl 00525 { 00526 /*! 00527 * Byte-access to the bits 00528 */ 00529 uint8_t Value ; 00530 /*! 00531 * Structure containing single access to bits 00532 */ 00533 struct sCtrlBits 00534 { 00535 /*! 00536 * Frame options length 00537 */ 00538 uint8_t FOptsLen : 4; 00539 /*! 00540 * Frame pending bit 00541 */ 00542 uint8_t FPending : 1; 00543 /*! 00544 * Message acknowledge bit 00545 */ 00546 uint8_t Ack : 1; 00547 /*! 00548 * ADR acknowledgment request bit 00549 */ 00550 uint8_t AdrAckReq : 1; 00551 /*! 00552 * ADR control in frame header 00553 */ 00554 uint8_t Adr : 1; 00555 }Bits; 00556 }LoRaMacFrameCtrl_t ; 00557 00558 /*! 00559 * Enumeration containing the status of the operation of a MAC service 00560 */ 00561 typedef enum eLoRaMacEventInfoStatus 00562 { 00563 /*! 00564 * Service performed successfully 00565 */ 00566 LORAMAC_EVENT_INFO_STATUS_OK = 0, 00567 /*! 00568 * An error occurred during the execution of the service 00569 */ 00570 LORAMAC_EVENT_INFO_STATUS_ERROR , 00571 /*! 00572 * A Tx timeout occurred 00573 */ 00574 LORAMAC_EVENT_INFO_STATUS_TX_TIMEOUT , 00575 /*! 00576 * An Rx timeout occurred on receive window 2 00577 */ 00578 LORAMAC_EVENT_INFO_STATUS_RX2_TIMEOUT , 00579 /*! 00580 * An Rx error occurred on receive window 1 00581 */ 00582 LORAMAC_EVENT_INFO_STATUS_RX1_ERROR , 00583 /*! 00584 * An Rx error occurred on receive window 2 00585 */ 00586 LORAMAC_EVENT_INFO_STATUS_RX2_ERROR , 00587 /*! 00588 * An error occurred in the join procedure 00589 */ 00590 LORAMAC_EVENT_INFO_STATUS_JOIN_FAIL , 00591 /*! 00592 * A frame with an invalid downlink counter was received. The 00593 * downlink counter of the frame was equal to the local copy 00594 * of the downlink counter of the node. 00595 */ 00596 LORAMAC_EVENT_INFO_STATUS_DOWNLINK_REPEATED , 00597 /*! 00598 * The MAC could not retransmit a frame since the MAC decreased the datarate. The 00599 * payload size is not applicable for the datarate. 00600 */ 00601 LORAMAC_EVENT_INFO_STATUS_TX_DR_PAYLOAD_SIZE_ERROR , 00602 /*! 00603 * The node has lost MAX_FCNT_GAP or more frames. 00604 */ 00605 LORAMAC_EVENT_INFO_STATUS_DOWNLINK_TOO_MANY_FRAMES_LOSS , 00606 /*! 00607 * An address error occurred 00608 */ 00609 LORAMAC_EVENT_INFO_STATUS_ADDRESS_FAIL , 00610 /*! 00611 * message integrity check failure 00612 */ 00613 LORAMAC_EVENT_INFO_STATUS_MIC_FAIL , 00614 }LoRaMacEventInfoStatus_t ; 00615 00616 /*! 00617 * LoRaMac tx/rx operation state 00618 */ 00619 typedef union eLoRaMacFlags_t 00620 { 00621 /*! 00622 * Byte-access to the bits 00623 */ 00624 uint8_t Value ; 00625 /*! 00626 * Structure containing single access to bits 00627 */ 00628 struct sMacFlagBits 00629 { 00630 /*! 00631 * MCPS-Req pending 00632 */ 00633 uint8_t McpsReq : 1; 00634 /*! 00635 * MCPS-Ind pending 00636 */ 00637 uint8_t McpsInd : 1; 00638 /*! 00639 * MCPS-Ind pending. Skip indication to the application layer 00640 */ 00641 uint8_t McpsIndSkip : 1; 00642 /*! 00643 * MLME-Req pending 00644 */ 00645 uint8_t MlmeReq : 1; 00646 /*! 00647 * MAC cycle done 00648 */ 00649 uint8_t MacDone : 1; 00650 }Bits; 00651 }LoRaMacFlags_t ; 00652 00653 /*! 00654 * 00655 * \brief LoRaMAC data services 00656 * 00657 * \details The following table list the primitives which are supported by the 00658 * specific MAC data service: 00659 * 00660 * Name | Request | Indication | Response | Confirm 00661 * --------------------- | :-----: | :--------: | :------: | :-----: 00662 * \ref MCPS_UNCONFIRMED | YES | YES | NO | YES 00663 * \ref MCPS_CONFIRMED | YES | YES | NO | YES 00664 * \ref MCPS_MULTICAST | NO | YES | NO | NO 00665 * \ref MCPS_PROPRIETARY | YES | YES | NO | YES 00666 * 00667 * The following table provides links to the function implementations of the 00668 * related MCPS primitives: 00669 * 00670 * Primitive | Function 00671 * ---------------- | :---------------------: 00672 * MCPS-Request | \ref LoRaMacMlmeRequest 00673 * MCPS-Confirm | MacMcpsConfirm in \ref LoRaMacPrimitives_t 00674 * MCPS-Indication | MacMcpsIndication in \ref LoRaMacPrimitives_t 00675 */ 00676 typedef enum eMcps 00677 { 00678 /*! 00679 * Unconfirmed LoRaMAC frame 00680 */ 00681 MCPS_UNCONFIRMED , 00682 /*! 00683 * Confirmed LoRaMAC frame 00684 */ 00685 MCPS_CONFIRMED , 00686 /*! 00687 * Multicast LoRaMAC frame 00688 */ 00689 MCPS_MULTICAST , 00690 /*! 00691 * Proprietary frame 00692 */ 00693 MCPS_PROPRIETARY , 00694 }Mcps_t; 00695 00696 /*! 00697 * LoRaMAC MCPS-Request for an unconfirmed frame 00698 */ 00699 typedef struct sMcpsReqUnconfirmed 00700 { 00701 /*! 00702 * Frame port field. Must be set if the payload is not empty. Use the 00703 * application specific frame port values: [1...223] 00704 * 00705 * LoRaWAN Specification V1.0.1, chapter 4.3.2 00706 */ 00707 uint8_t fPort ; 00708 /*! 00709 * Pointer to the buffer of the frame payload 00710 */ 00711 void *fBuffer ; 00712 /*! 00713 * Size of the frame payload 00714 */ 00715 uint16_t fBufferSize ; 00716 /*! 00717 * Uplink datarate, if ADR is off 00718 */ 00719 int8_t Datarate ; 00720 }McpsReqUnconfirmed_t ; 00721 00722 /*! 00723 * LoRaMAC MCPS-Request for a confirmed frame 00724 */ 00725 typedef struct sMcpsReqConfirmed 00726 { 00727 /*! 00728 * Frame port field. Must be set if the payload is not empty. Use the 00729 * application specific frame port values: [1...223] 00730 * 00731 * LoRaWAN Specification V1.0.1, chapter 4.3.2 00732 */ 00733 uint8_t fPort ; 00734 /*! 00735 * Pointer to the buffer of the frame payload 00736 */ 00737 void *fBuffer ; 00738 /*! 00739 * Size of the frame payload 00740 */ 00741 uint16_t fBufferSize ; 00742 /*! 00743 * Uplink datarate, if ADR is off 00744 */ 00745 int8_t Datarate ; 00746 /*! 00747 * Number of trials to transmit the frame, if the LoRaMAC layer did not 00748 * receive an acknowledgment. The MAC performs a datarate adaptation, 00749 * according to the LoRaWAN Specification V1.0.1, chapter 19.4, according 00750 * to the following table: 00751 * 00752 * Transmission nb | Data Rate 00753 * ----------------|----------- 00754 * 1 (first) | DR 00755 * 2 | DR 00756 * 3 | max(DR-1,0) 00757 * 4 | max(DR-1,0) 00758 * 5 | max(DR-2,0) 00759 * 6 | max(DR-2,0) 00760 * 7 | max(DR-3,0) 00761 * 8 | max(DR-3,0) 00762 * 00763 * Note, that if NbTrials is set to 1 or 2, the MAC will not decrease 00764 * the datarate, in case the LoRaMAC layer did not receive an acknowledgment 00765 */ 00766 uint8_t NbTrials ; 00767 }McpsReqConfirmed_t ; 00768 00769 /*! 00770 * LoRaMAC MCPS-Request for a proprietary frame 00771 */ 00772 typedef struct sMcpsReqProprietary 00773 { 00774 /*! 00775 * Pointer to the buffer of the frame payload 00776 */ 00777 void *fBuffer ; 00778 /*! 00779 * Size of the frame payload 00780 */ 00781 uint16_t fBufferSize ; 00782 /*! 00783 * Uplink datarate, if ADR is off 00784 */ 00785 int8_t Datarate ; 00786 }McpsReqProprietary_t ; 00787 00788 /*! 00789 * LoRaMAC MCPS-Request structure 00790 */ 00791 typedef struct sMcpsReq 00792 { 00793 /*! 00794 * MCPS-Request type 00795 */ 00796 Mcps_t Type ; 00797 00798 /*! 00799 * MCPS-Request parameters 00800 */ 00801 union uMcpsParam 00802 { 00803 /*! 00804 * MCPS-Request parameters for an unconfirmed frame 00805 */ 00806 McpsReqUnconfirmed_t Unconfirmed ; 00807 /*! 00808 * MCPS-Request parameters for a confirmed frame 00809 */ 00810 McpsReqConfirmed_t Confirmed ; 00811 /*! 00812 * MCPS-Request parameters for a proprietary frame 00813 */ 00814 McpsReqProprietary_t Proprietary ; 00815 }Req; 00816 }McpsReq_t ; 00817 00818 /*! 00819 * LoRaMAC MCPS-Confirm 00820 */ 00821 typedef struct sMcpsConfirm 00822 { 00823 /*! 00824 * Holds the previously performed MCPS-Request 00825 */ 00826 Mcps_t McpsRequest ; 00827 /*! 00828 * Status of the operation 00829 */ 00830 LoRaMacEventInfoStatus_t Status ; 00831 /*! 00832 * Uplink datarate 00833 */ 00834 uint8_t Datarate ; 00835 /*! 00836 * Transmission power 00837 */ 00838 int8_t TxPower ; 00839 /*! 00840 * Set if an acknowledgement was received 00841 */ 00842 bool AckReceived ; 00843 /*! 00844 * Provides the number of retransmissions 00845 */ 00846 uint8_t NbRetries ; 00847 /*! 00848 * The transmission time on air of the frame 00849 */ 00850 TimerTime_t TxTimeOnAir ; 00851 /*! 00852 * The uplink counter value related to the frame 00853 */ 00854 uint32_t UpLinkCounter ; 00855 /*! 00856 * The uplink frequency related to the frame 00857 */ 00858 uint32_t UpLinkFrequency ; 00859 }McpsConfirm_t ; 00860 00861 /*! 00862 * LoRaMAC MCPS-Indication primitive 00863 */ 00864 typedef struct sMcpsIndication 00865 { 00866 /*! 00867 * MCPS-Indication type 00868 */ 00869 Mcps_t McpsIndication ; 00870 /*! 00871 * Status of the operation 00872 */ 00873 LoRaMacEventInfoStatus_t Status ; 00874 /*! 00875 * Multicast 00876 */ 00877 uint8_t Multicast ; 00878 /*! 00879 * Application port 00880 */ 00881 uint8_t Port ; 00882 /*! 00883 * Downlink datarate 00884 */ 00885 uint8_t RxDatarate ; 00886 /*! 00887 * Frame pending status 00888 */ 00889 uint8_t FramePending ; 00890 /*! 00891 * Pointer to the received data stream 00892 */ 00893 uint8_t *Buffer ; 00894 /*! 00895 * Size of the received data stream 00896 */ 00897 uint8_t BufferSize ; 00898 /*! 00899 * Indicates, if data is available 00900 */ 00901 bool RxData ; 00902 /*! 00903 * Rssi of the received packet 00904 */ 00905 int16_t Rssi ; 00906 /*! 00907 * Snr of the received packet 00908 */ 00909 uint8_t Snr ; 00910 /*! 00911 * Receive window 00912 * 00913 * [0: Rx window 1, 1: Rx window 2] 00914 */ 00915 uint8_t RxSlot ; 00916 /*! 00917 * Set if an acknowledgement was received 00918 */ 00919 bool AckReceived ; 00920 /*! 00921 * The downlink counter value for the received frame 00922 */ 00923 uint32_t DownLinkCounter ; 00924 }McpsIndication_t ; 00925 00926 /*! 00927 * \brief LoRaMAC management services 00928 * 00929 * \details The following table list the primitives which are supported by the 00930 * specific MAC management service: 00931 * 00932 * Name | Request | Indication | Response | Confirm 00933 * --------------------- | :-----: | :--------: | :------: | :-----: 00934 * \ref MLME_JOIN | YES | NO | NO | YES 00935 * \ref MLME_LINK_CHECK | YES | NO | NO | YES 00936 * \ref MLME_TXCW | YES | NO | NO | YES 00937 * 00938 * The following table provides links to the function implementations of the 00939 * related MLME primitives. 00940 * 00941 * Primitive | Function 00942 * ---------------- | :---------------------: 00943 * MLME-Request | \ref LoRaMacMlmeRequest 00944 * MLME-Confirm | MacMlmeConfirm in \ref LoRaMacPrimitives_t 00945 */ 00946 typedef enum eMlme 00947 { 00948 /*! 00949 * Initiates the Over-the-Air activation 00950 * 00951 * LoRaWAN Specification V1.0.1, chapter 6.2 00952 */ 00953 MLME_JOIN , 00954 /*! 00955 * LinkCheckReq - Connectivity validation 00956 * 00957 * LoRaWAN Specification V1.0.1, chapter 5, table 4 00958 */ 00959 MLME_LINK_CHECK , 00960 /*! 00961 * Sets Tx continuous wave mode 00962 * 00963 * LoRaWAN end-device certification 00964 */ 00965 MLME_TXCW , 00966 /*! 00967 * Sets Tx continuous wave mode (new LoRa-Alliance CC definition) 00968 * 00969 * LoRaWAN end-device certification 00970 */ 00971 MLME_TXCW_1 , 00972 }Mlme_t; 00973 00974 /*! 00975 * LoRaMAC MLME-Request for the join service 00976 */ 00977 typedef struct sMlmeReqJoin 00978 { 00979 /*! 00980 * Globally unique end-device identifier 00981 * 00982 * LoRaWAN Specification V1.0.1, chapter 6.2.1 00983 */ 00984 uint8_t *DevEui ; 00985 /*! 00986 * Application identifier 00987 * 00988 * LoRaWAN Specification V1.0.1, chapter 6.1.2 00989 */ 00990 uint8_t *AppEui ; 00991 /*! 00992 * AES-128 application key 00993 * 00994 * LoRaWAN Specification V1.0.1, chapter 6.2.2 00995 */ 00996 uint8_t *AppKey ; 00997 /*! 00998 * Number of trials for the join request. 00999 */ 01000 uint8_t NbTrials ; 01001 }MlmeReqJoin_t ; 01002 01003 /*! 01004 * LoRaMAC MLME-Request for Tx continuous wave mode 01005 */ 01006 typedef struct sMlmeReqTxCw 01007 { 01008 /*! 01009 * Time in seconds while the radio is kept in continuous wave mode 01010 */ 01011 uint16_t Timeout ; 01012 /*! 01013 * RF frequency to set (Only used with new way) 01014 */ 01015 uint32_t Frequency ; 01016 /*! 01017 * RF output power to set (Only used with new way) 01018 */ 01019 uint8_t Power ; 01020 }MlmeReqTxCw_t ; 01021 01022 /*! 01023 * LoRaMAC MLME-Request structure 01024 */ 01025 typedef struct sMlmeReq 01026 { 01027 /*! 01028 * MLME-Request type 01029 */ 01030 Mlme_t Type ; 01031 01032 /*! 01033 * MLME-Request parameters 01034 */ 01035 union uMlmeParam 01036 { 01037 /*! 01038 * MLME-Request parameters for a join request 01039 */ 01040 MlmeReqJoin_t Join ; 01041 /*! 01042 * MLME-Request parameters for Tx continuous mode request 01043 */ 01044 MlmeReqTxCw_t TxCw ; 01045 }Req; 01046 }MlmeReq_t ; 01047 01048 /*! 01049 * LoRaMAC MLME-Confirm primitive 01050 */ 01051 typedef struct sMlmeConfirm 01052 { 01053 /*! 01054 * Holds the previously performed MLME-Request 01055 */ 01056 Mlme_t MlmeRequest ; 01057 /*! 01058 * Status of the operation 01059 */ 01060 LoRaMacEventInfoStatus_t Status ; 01061 /*! 01062 * The transmission time on air of the frame 01063 */ 01064 TimerTime_t TxTimeOnAir ; 01065 /*! 01066 * Demodulation margin. Contains the link margin [dB] of the last 01067 * successfully received LinkCheckReq 01068 */ 01069 uint8_t DemodMargin ; 01070 /*! 01071 * Number of gateways which received the last LinkCheckReq 01072 */ 01073 uint8_t NbGateways ; 01074 /*! 01075 * Provides the number of retransmissions 01076 */ 01077 uint8_t NbRetries ; 01078 }MlmeConfirm_t ; 01079 01080 /*! 01081 * LoRa Mac Information Base (MIB) 01082 * 01083 * The following table lists the MIB parameters and the related attributes: 01084 * 01085 * Attribute | Get | Set 01086 * --------------------------------- | :-: | :-: 01087 * \ref MIB_DEVICE_CLASS | YES | YES 01088 * \ref MIB_NETWORK_JOINED | YES | YES 01089 * \ref MIB_ADR | YES | YES 01090 * \ref MIB_NET_ID | YES | YES 01091 * \ref MIB_DEV_ADDR | YES | YES 01092 * \ref MIB_NWK_SKEY | YES | YES 01093 * \ref MIB_APP_SKEY | YES | YES 01094 * \ref MIB_PUBLIC_NETWORK | YES | YES 01095 * \ref MIB_REPEATER_SUPPORT | YES | YES 01096 * \ref MIB_CHANNELS | YES | NO 01097 * \ref MIB_RX2_CHANNEL | YES | YES 01098 * \ref MIB_CHANNELS_MASK | YES | YES 01099 * \ref MIB_CHANNELS_DEFAULT_MASK | YES | YES 01100 * \ref MIB_CHANNELS_NB_REP | YES | YES 01101 * \ref MIB_MAX_RX_WINDOW_DURATION | YES | YES 01102 * \ref MIB_RECEIVE_DELAY_1 | YES | YES 01103 * \ref MIB_RECEIVE_DELAY_2 | YES | YES 01104 * \ref MIB_JOIN_ACCEPT_DELAY_1 | YES | YES 01105 * \ref MIB_JOIN_ACCEPT_DELAY_2 | YES | YES 01106 * \ref MIB_CHANNELS_DATARATE | YES | YES 01107 * \ref MIB_CHANNELS_DEFAULT_DATARATE| YES | YES 01108 * \ref MIB_CHANNELS_TX_POWER | YES | YES 01109 * \ref MIB_CHANNELS_DEFAULT_TX_POWER| YES | YES 01110 * \ref MIB_UPLINK_COUNTER | YES | YES 01111 * \ref MIB_DOWNLINK_COUNTER | YES | YES 01112 * \ref MIB_MULTICAST_CHANNEL | YES | NO 01113 * \ref MIB_SYSTEM_MAX_RX_ERROR | YES | YES 01114 * \ref MIB_MIN_RX_SYMBOLS | YES | YES 01115 * 01116 * The following table provides links to the function implementations of the 01117 * related MIB primitives: 01118 * 01119 * Primitive | Function 01120 * ---------------- | :---------------------: 01121 * MIB-Set | \ref LoRaMacMibSetRequestConfirm 01122 * MIB-Get | \ref LoRaMacMibGetRequestConfirm 01123 */ 01124 typedef enum eMib 01125 { 01126 /*! 01127 * LoRaWAN device class 01128 * 01129 * LoRaWAN Specification V1.0.1 01130 */ 01131 MIB_DEVICE_CLASS , 01132 /*! 01133 * LoRaWAN Network joined attribute 01134 * 01135 * LoRaWAN Specification V1.0.1 01136 */ 01137 MIB_NETWORK_JOINED , 01138 /*! 01139 * Adaptive data rate 01140 * 01141 * LoRaWAN Specification V1.0.1, chapter 4.3.1.1 01142 * 01143 * [true: ADR enabled, false: ADR disabled] 01144 */ 01145 MIB_ADR , 01146 /*! 01147 * Network identifier 01148 * 01149 * LoRaWAN Specification V1.0.1, chapter 6.1.1 01150 */ 01151 MIB_NET_ID , 01152 /*! 01153 * End-device address 01154 * 01155 * LoRaWAN Specification V1.0.1, chapter 6.1.1 01156 */ 01157 MIB_DEV_ADDR , 01158 /*! 01159 * Network session key 01160 * 01161 * LoRaWAN Specification V1.0.1, chapter 6.1.3 01162 */ 01163 MIB_NWK_SKEY , 01164 /*! 01165 * Application session key 01166 * 01167 * LoRaWAN Specification V1.0.1, chapter 6.1.4 01168 */ 01169 MIB_APP_SKEY , 01170 /*! 01171 * Set the network type to public or private 01172 * 01173 * LoRaWAN Specification V1.0.1, chapter 7 01174 * 01175 * [true: public network, false: private network] 01176 */ 01177 MIB_PUBLIC_NETWORK , 01178 /*! 01179 * Support the operation with repeaters 01180 * 01181 * LoRaWAN Specification V1.0.1, chapter 7 01182 * 01183 * [true: repeater support enabled, false: repeater support disabled] 01184 */ 01185 MIB_REPEATER_SUPPORT , 01186 /*! 01187 * Communication channels. A get request will return a 01188 * pointer which references the first entry of the channel list. The 01189 * list is of size LORA_MAX_NB_CHANNELS 01190 * 01191 * LoRaWAN Specification V1.0.1, chapter 7 01192 */ 01193 MIB_CHANNELS , 01194 /*! 01195 * Set receive window 2 channel 01196 * 01197 * LoRaWAN Specification V1.0.1, chapter 3.3.2 01198 */ 01199 MIB_RX2_CHANNEL , 01200 /*! 01201 * Set receive window 2 channel 01202 * 01203 * LoRaWAN Specification V1.0.1, chapter 3.3.2 01204 */ 01205 MIB_RX2_DEFAULT_CHANNEL , 01206 /*! 01207 * LoRaWAN channels mask 01208 * 01209 * LoRaWAN Specification V1.0.1, chapter 7 01210 */ 01211 MIB_CHANNELS_MASK , 01212 /*! 01213 * LoRaWAN default channels mask 01214 * 01215 * LoRaWAN Specification V1.0.1, chapter 7 01216 */ 01217 MIB_CHANNELS_DEFAULT_MASK , 01218 /*! 01219 * Set the number of repetitions on a channel 01220 * 01221 * LoRaWAN Specification V1.0.1, chapter 5.2 01222 */ 01223 MIB_CHANNELS_NB_REP , 01224 /*! 01225 * Maximum receive window duration in [ms] 01226 * 01227 * LoRaWAN Specification V1.0.1, chapter 3.3.3 01228 */ 01229 MIB_MAX_RX_WINDOW_DURATION , 01230 /*! 01231 * Receive delay 1 in [ms] 01232 * 01233 * LoRaWAN Specification V1.0.1, chapter 7 01234 */ 01235 MIB_RECEIVE_DELAY_1 , 01236 /*! 01237 * Receive delay 2 in [ms] 01238 * 01239 * LoRaWAN Specification V1.0.1, chapter 7 01240 */ 01241 MIB_RECEIVE_DELAY_2 , 01242 /*! 01243 * Join accept delay 1 in [ms] 01244 * 01245 * LoRaWAN Specification V1.0.1, chapter 7 01246 */ 01247 MIB_JOIN_ACCEPT_DELAY_1 , 01248 /*! 01249 * Join accept delay 2 in [ms] 01250 * 01251 * LoRaWAN Specification V1.0.1, chapter 7 01252 */ 01253 MIB_JOIN_ACCEPT_DELAY_2 , 01254 /*! 01255 * Default Data rate of a channel 01256 * 01257 * LoRaWAN Specification V1.0.1, chapter 7 01258 * 01259 * EU868 - [DR_0, DR_1, DR_2, DR_3, DR_4, DR_5, DR_6, DR_7] 01260 * 01261 * US915 - [DR_0, DR_1, DR_2, DR_3, DR_4, DR_8, DR_9, DR_10, DR_11, DR_12, DR_13] 01262 */ 01263 MIB_CHANNELS_DEFAULT_DATARATE , 01264 /*! 01265 * Data rate of a channel 01266 * 01267 * LoRaWAN Specification V1.0.1, chapter 7 01268 * 01269 * EU868 - [DR_0, DR_1, DR_2, DR_3, DR_4, DR_5, DR_6, DR_7] 01270 * 01271 * US915 - [DR_0, DR_1, DR_2, DR_3, DR_4, DR_8, DR_9, DR_10, DR_11, DR_12, DR_13] 01272 */ 01273 MIB_CHANNELS_DATARATE , 01274 /*! 01275 * Transmission power of a channel 01276 * 01277 * LoRaWAN Specification V1.0.1, chapter 7 01278 * 01279 * EU868 - [TX_POWER_20_DBM, TX_POWER_14_DBM, TX_POWER_11_DBM, 01280 * TX_POWER_08_DBM, TX_POWER_05_DBM, TX_POWER_02_DBM] 01281 * 01282 * US915 - [TX_POWER_30_DBM, TX_POWER_28_DBM, TX_POWER_26_DBM, 01283 * TX_POWER_24_DBM, TX_POWER_22_DBM, TX_POWER_20_DBM, 01284 * TX_POWER_18_DBM, TX_POWER_14_DBM, TX_POWER_12_DBM, 01285 * TX_POWER_10_DBM] 01286 */ 01287 MIB_CHANNELS_TX_POWER , 01288 /*! 01289 * Transmission power of a channel 01290 * 01291 * LoRaWAN Specification V1.0.1, chapter 7 01292 * 01293 * EU868 - [TX_POWER_20_DBM, TX_POWER_14_DBM, TX_POWER_11_DBM, 01294 * TX_POWER_08_DBM, TX_POWER_05_DBM, TX_POWER_02_DBM] 01295 * 01296 * US915 - [TX_POWER_30_DBM, TX_POWER_28_DBM, TX_POWER_26_DBM, 01297 * TX_POWER_24_DBM, TX_POWER_22_DBM, TX_POWER_20_DBM, 01298 * TX_POWER_18_DBM, TX_POWER_14_DBM, TX_POWER_12_DBM, 01299 * TX_POWER_10_DBM] 01300 */ 01301 MIB_CHANNELS_DEFAULT_TX_POWER , 01302 /*! 01303 * LoRaWAN Up-link counter 01304 * 01305 * LoRaWAN Specification V1.0.1, chapter 4.3.1.5 01306 */ 01307 MIB_UPLINK_COUNTER , 01308 /*! 01309 * LoRaWAN Down-link counter 01310 * 01311 * LoRaWAN Specification V1.0.1, chapter 4.3.1.5 01312 */ 01313 MIB_DOWNLINK_COUNTER , 01314 /*! 01315 * Multicast channels. A get request will return a pointer to the first 01316 * entry of the multicast channel linked list. If the pointer is equal to 01317 * NULL, the list is empty. 01318 */ 01319 MIB_MULTICAST_CHANNEL , 01320 /*! 01321 * System overall timing error in milliseconds. 01322 * [-SystemMaxRxError : +SystemMaxRxError] 01323 * Default: +/-10 ms 01324 */ 01325 MIB_SYSTEM_MAX_RX_ERROR , 01326 /*! 01327 * Minimum required number of symbols to detect an Rx frame 01328 * Default: 6 symbols 01329 */ 01330 MIB_MIN_RX_SYMBOLS , 01331 }Mib_t ; 01332 01333 /*! 01334 * LoRaMAC MIB parameters 01335 */ 01336 typedef union uMibParam 01337 { 01338 /*! 01339 * LoRaWAN device class 01340 * 01341 * Related MIB type: \ref MIB_DEVICE_CLASS 01342 */ 01343 DeviceClass_t Class ; 01344 /*! 01345 * LoRaWAN network joined attribute 01346 * 01347 * Related MIB type: \ref MIB_NETWORK_JOINED 01348 */ 01349 bool IsNetworkJoined ; 01350 /*! 01351 * Activation state of ADR 01352 * 01353 * Related MIB type: \ref MIB_ADR 01354 */ 01355 bool AdrEnable ; 01356 /*! 01357 * Network identifier 01358 * 01359 * Related MIB type: \ref MIB_NET_ID 01360 */ 01361 uint32_t NetID ; 01362 /*! 01363 * End-device address 01364 * 01365 * Related MIB type: \ref MIB_DEV_ADDR 01366 */ 01367 uint32_t DevAddr ; 01368 /*! 01369 * Network session key 01370 * 01371 * Related MIB type: \ref MIB_NWK_SKEY 01372 */ 01373 uint8_t *NwkSKey ; 01374 /*! 01375 * Application session key 01376 * 01377 * Related MIB type: \ref MIB_APP_SKEY 01378 */ 01379 uint8_t *AppSKey ; 01380 /*! 01381 * Enable or disable a public network 01382 * 01383 * Related MIB type: \ref MIB_PUBLIC_NETWORK 01384 */ 01385 bool EnablePublicNetwork ; 01386 /*! 01387 * Enable or disable repeater support 01388 * 01389 * Related MIB type: \ref MIB_REPEATER_SUPPORT 01390 */ 01391 bool EnableRepeaterSupport ; 01392 /*! 01393 * LoRaWAN Channel 01394 * 01395 * Related MIB type: \ref MIB_CHANNELS 01396 */ 01397 ChannelParams_t * ChannelList ; 01398 /*! 01399 * Channel for the receive window 2 01400 * 01401 * Related MIB type: \ref MIB_RX2_CHANNEL 01402 */ 01403 Rx2ChannelParams_t Rx2Channel ; 01404 /*! 01405 * Channel for the receive window 2 01406 * 01407 * Related MIB type: \ref MIB_RX2_DEFAULT_CHANNEL 01408 */ 01409 Rx2ChannelParams_t Rx2DefaultChannel ; 01410 /*! 01411 * Channel mask 01412 * 01413 * Related MIB type: \ref MIB_CHANNELS_MASK 01414 */ 01415 uint16_t* ChannelsMask ; 01416 /*! 01417 * Default channel mask 01418 * 01419 * Related MIB type: \ref MIB_CHANNELS_DEFAULT_MASK 01420 */ 01421 uint16_t* ChannelsDefaultMask ; 01422 /*! 01423 * Number of frame repetitions 01424 * 01425 * Related MIB type: \ref MIB_CHANNELS_NB_REP 01426 */ 01427 uint8_t ChannelNbRep ; 01428 /*! 01429 * Maximum receive window duration 01430 * 01431 * Related MIB type: \ref MIB_MAX_RX_WINDOW_DURATION 01432 */ 01433 uint32_t MaxRxWindow ; 01434 /*! 01435 * Receive delay 1 01436 * 01437 * Related MIB type: \ref MIB_RECEIVE_DELAY_1 01438 */ 01439 uint32_t ReceiveDelay1 ; 01440 /*! 01441 * Receive delay 2 01442 * 01443 * Related MIB type: \ref MIB_RECEIVE_DELAY_2 01444 */ 01445 uint32_t ReceiveDelay2 ; 01446 /*! 01447 * Join accept delay 1 01448 * 01449 * Related MIB type: \ref MIB_JOIN_ACCEPT_DELAY_1 01450 */ 01451 uint32_t JoinAcceptDelay1 ; 01452 /*! 01453 * Join accept delay 2 01454 * 01455 * Related MIB type: \ref MIB_JOIN_ACCEPT_DELAY_2 01456 */ 01457 uint32_t JoinAcceptDelay2 ; 01458 /*! 01459 * Channels data rate 01460 * 01461 * Related MIB type: \ref MIB_CHANNELS_DEFAULT_DATARATE 01462 */ 01463 int8_t ChannelsDefaultDatarate ; 01464 /*! 01465 * Channels data rate 01466 * 01467 * Related MIB type: \ref MIB_CHANNELS_DATARATE 01468 */ 01469 int8_t ChannelsDatarate ; 01470 /*! 01471 * Channels TX power 01472 * 01473 * Related MIB type: \ref MIB_CHANNELS_DEFAULT_TX_POWER 01474 */ 01475 int8_t ChannelsDefaultTxPower ; 01476 /*! 01477 * Channels TX power 01478 * 01479 * Related MIB type: \ref MIB_CHANNELS_TX_POWER 01480 */ 01481 int8_t ChannelsTxPower ; 01482 /*! 01483 * LoRaWAN Up-link counter 01484 * 01485 * Related MIB type: \ref MIB_UPLINK_COUNTER 01486 */ 01487 uint32_t UpLinkCounter ; 01488 /*! 01489 * LoRaWAN Down-link counter 01490 * 01491 * Related MIB type: \ref MIB_DOWNLINK_COUNTER 01492 */ 01493 uint32_t DownLinkCounter ; 01494 /*! 01495 * Multicast channel 01496 * 01497 * Related MIB type: \ref MIB_MULTICAST_CHANNEL 01498 */ 01499 MulticastParams_t * MulticastList ; 01500 /*! 01501 * System overall timing error in milliseconds. 01502 * 01503 * Related MIB type: \ref MIB_SYSTEM_MAX_RX_ERROR 01504 */ 01505 uint32_t SystemMaxRxError ; 01506 /*! 01507 * Minimum required number of symbols to detect an Rx frame 01508 * 01509 * Related MIB type: \ref MIB_MIN_RX_SYMBOLS 01510 */ 01511 uint8_t MinRxSymbols ; 01512 }MibParam_t ; 01513 01514 /*! 01515 * LoRaMAC MIB-RequestConfirm structure 01516 */ 01517 typedef struct eMibRequestConfirm 01518 { 01519 /*! 01520 * MIB-Request type 01521 */ 01522 Mib_t Type ; 01523 01524 /*! 01525 * MLME-RequestConfirm parameters 01526 */ 01527 MibParam_t Param ; 01528 }MibRequestConfirm_t ; 01529 01530 /*! 01531 * LoRaMAC tx information 01532 */ 01533 typedef struct sLoRaMacTxInfo 01534 { 01535 /*! 01536 * Defines the size of the applicative payload which can be processed 01537 */ 01538 uint8_t MaxPossiblePayload ; 01539 /*! 01540 * The current payload size, dependent on the current datarate 01541 */ 01542 uint8_t CurrentPayloadSize ; 01543 }LoRaMacTxInfo_t ; 01544 01545 /*! 01546 * LoRaMAC Status 01547 */ 01548 typedef enum eLoRaMacStatus 01549 { 01550 /*! 01551 * Service started successfully 01552 */ 01553 LORAMAC_STATUS_OK , 01554 /*! 01555 * Service not started - LoRaMAC is busy 01556 */ 01557 LORAMAC_STATUS_BUSY , 01558 /*! 01559 * Service unknown 01560 */ 01561 LORAMAC_STATUS_SERVICE_UNKNOWN , 01562 /*! 01563 * Service not started - invalid parameter 01564 */ 01565 LORAMAC_STATUS_PARAMETER_INVALID , 01566 /*! 01567 * Service not started - invalid frequency 01568 */ 01569 LORAMAC_STATUS_FREQUENCY_INVALID , 01570 /*! 01571 * Service not started - invalid datarate 01572 */ 01573 LORAMAC_STATUS_DATARATE_INVALID , 01574 /*! 01575 * Service not started - invalid frequency and datarate 01576 */ 01577 LORAMAC_STATUS_FREQ_AND_DR_INVALID , 01578 /*! 01579 * Service not started - the device is not in a LoRaWAN 01580 */ 01581 LORAMAC_STATUS_NO_NETWORK_JOINED , 01582 /*! 01583 * Service not started - payload lenght error 01584 */ 01585 LORAMAC_STATUS_LENGTH_ERROR , 01586 /*! 01587 * Service not started - payload lenght error 01588 */ 01589 LORAMAC_STATUS_MAC_CMD_LENGTH_ERROR , 01590 /*! 01591 * Service not started - the device is switched off 01592 */ 01593 LORAMAC_STATUS_DEVICE_OFF , 01594 }LoRaMacStatus_t ; 01595 01596 /*! 01597 * LoRaMAC events structure 01598 * Used to notify upper layers of MAC events 01599 */ 01600 typedef struct sLoRaMacPrimitives 01601 { 01602 /*! 01603 * \brief MCPS-Confirm primitive 01604 * 01605 * \param [OUT] MCPS-Confirm parameters 01606 */ 01607 void ( *MacMcpsConfirm )( McpsConfirm_t *McpsConfirm ); 01608 /*! 01609 * \brief MCPS-Indication primitive 01610 * 01611 * \param [OUT] MCPS-Indication parameters 01612 */ 01613 void ( *MacMcpsIndication )( McpsIndication_t *McpsIndication ); 01614 /*! 01615 * \brief MLME-Confirm primitive 01616 * 01617 * \param [OUT] MLME-Confirm parameters 01618 */ 01619 void ( *MacMlmeConfirm )( MlmeConfirm_t *MlmeConfirm ); 01620 }LoRaMacPrimitives_t ; 01621 01622 typedef struct sLoRaMacCallback 01623 { 01624 /*! 01625 * \brief Measures the battery level 01626 * 01627 * \retval Battery level [0: node is connected to an external 01628 * power source, 1..254: battery level, where 1 is the minimum 01629 * and 254 is the maximum value, 255: the node was not able 01630 * to measure the battery level] 01631 */ 01632 uint8_t ( *GetBatteryLevel )( void ); 01633 }LoRaMacCallback_t; 01634 01635 /*! 01636 * \brief LoRaMAC layer initialization 01637 * 01638 * \details In addition to the initialization of the LoRaMAC layer, this 01639 * function initializes the callback primitives of the MCPS and 01640 * MLME services. Every data field of \ref LoRaMacPrimitives_t must be 01641 * set to a valid callback function. 01642 * 01643 * \param [IN] events - Pointer to a structure defining the LoRaMAC 01644 * event functions. Refer to \ref LoRaMacPrimitives_t. 01645 * 01646 * \param [IN] events - Pointer to a structure defining the LoRaMAC 01647 * callback functions. Refer to \ref LoRaMacCallback_t. 01648 * 01649 * \retval LoRaMacStatus_t Status of the operation. Possible returns are: 01650 * returns are: 01651 * \ref LORAMAC_STATUS_OK, 01652 * \ref LORAMAC_STATUS_PARAMETER_INVALID. 01653 */ 01654 LoRaMacStatus_t LoRaMacInitialization( LoRaMacPrimitives_t *primitives, LoRaMacCallback_t *callbacks ); 01655 01656 /*! 01657 * \brief Queries the LoRaMAC if it is possible to send the next frame with 01658 * a given payload size. The LoRaMAC takes scheduled MAC commands into 01659 * account and reports, when the frame can be send or not. 01660 * 01661 * \param [IN] size - Size of applicative payload to be send next 01662 * 01663 * \param [OUT] txInfo - The structure \ref LoRaMacTxInfo_t contains 01664 * information about the actual maximum payload possible 01665 * ( according to the configured datarate or the next 01666 * datarate according to ADR ), and the maximum frame 01667 * size, taking the scheduled MAC commands into account. 01668 * 01669 * \retval LoRaMacStatus_t Status of the operation. When the parameters are 01670 * not valid, the function returns \ref LORAMAC_STATUS_PARAMETER_INVALID. 01671 * In case of a length error caused by the applicative payload size, the 01672 * function returns LORAMAC_STATUS_LENGTH_ERROR. In case of a length error 01673 * due to additional MAC commands in the queue, the function returns 01674 * LORAMAC_STATUS_MAC_CMD_LENGTH_ERROR. In case the query is valid, and 01675 * the LoRaMAC is able to send the frame, the function returns LORAMAC_STATUS_OK. * 01676 */ 01677 LoRaMacStatus_t LoRaMacQueryTxPossible( uint8_t size, LoRaMacTxInfo_t * txInfo ); 01678 01679 /*! 01680 * \brief LoRaMAC channel add service 01681 * 01682 * \details Adds a new channel to the channel list and activates the id in 01683 * the channel mask. For the US915 band, all channels are enabled 01684 * by default. It is not possible to activate less than 6 125 kHz 01685 * channels. 01686 * 01687 * \param [IN] id - Id of the channel. Possible values are: 01688 * 01689 * 0-15 for EU868 01690 * 0-72 for US915 01691 * 01692 * \param [IN] params - Channel parameters to set. 01693 * 01694 * \retval LoRaMacStatus_t Status of the operation. Possible returns are: 01695 * \ref LORAMAC_STATUS_OK, 01696 * \ref LORAMAC_STATUS_BUSY, 01697 * \ref LORAMAC_STATUS_PARAMETER_INVALID. 01698 */ 01699 LoRaMacStatus_t LoRaMacChannelAdd( uint8_t id, ChannelParams_t params ); 01700 01701 /*! 01702 * \brief LoRaMAC channel remove service 01703 * 01704 * \details Deactivates the id in the channel mask. 01705 * 01706 * \param [IN] id - Id of the channel. 01707 * 01708 * \retval LoRaMacStatus_t Status of the operation. Possible returns are: 01709 * \ref LORAMAC_STATUS_OK, 01710 * \ref LORAMAC_STATUS_BUSY, 01711 * \ref LORAMAC_STATUS_PARAMETER_INVALID. 01712 */ 01713 LoRaMacStatus_t LoRaMacChannelRemove( uint8_t id ); 01714 01715 /*! 01716 * \brief LoRaMAC multicast channel link service 01717 * 01718 * \details Links a multicast channel into the linked list. 01719 * 01720 * \param [IN] channelParam - Multicast channel parameters to link. 01721 * 01722 * \retval LoRaMacStatus_t Status of the operation. Possible returns are: 01723 * \ref LORAMAC_STATUS_OK, 01724 * \ref LORAMAC_STATUS_BUSY, 01725 * \ref LORAMAC_STATUS_PARAMETER_INVALID. 01726 */ 01727 LoRaMacStatus_t LoRaMacMulticastChannelLink( MulticastParams_t *channelParam ); 01728 01729 /*! 01730 * \brief LoRaMAC multicast channel unlink service 01731 * 01732 * \details Unlinks a multicast channel from the linked list. 01733 * 01734 * \param [IN] channelParam - Multicast channel parameters to unlink. 01735 * 01736 * \retval LoRaMacStatus_t Status of the operation. Possible returns are: 01737 * \ref LORAMAC_STATUS_OK, 01738 * \ref LORAMAC_STATUS_BUSY, 01739 * \ref LORAMAC_STATUS_PARAMETER_INVALID. 01740 */ 01741 LoRaMacStatus_t LoRaMacMulticastChannelUnlink( MulticastParams_t *channelParam ); 01742 01743 /*! 01744 * \brief LoRaMAC MIB-Get 01745 * 01746 * \details The mac information base service to get attributes of the LoRaMac 01747 * layer. 01748 * 01749 * The following code-snippet shows how to use the API to get the 01750 * parameter AdrEnable, defined by the enumeration type 01751 * \ref MIB_ADR. 01752 * \code 01753 * MibRequestConfirm_t mibReq; 01754 * mibReq.Type = MIB_ADR; 01755 * 01756 * if( LoRaMacMibGetRequestConfirm( &mibReq ) == LORAMAC_STATUS_OK ) 01757 * { 01758 * // LoRaMAC updated the parameter mibParam.AdrEnable 01759 * } 01760 * \endcode 01761 * 01762 * \param [IN] mibRequest - MIB-GET-Request to perform. Refer to \ref MibRequestConfirm_t. 01763 * 01764 * \retval LoRaMacStatus_t Status of the operation. Possible returns are: 01765 * \ref LORAMAC_STATUS_OK, 01766 * \ref LORAMAC_STATUS_SERVICE_UNKNOWN, 01767 * \ref LORAMAC_STATUS_PARAMETER_INVALID. 01768 */ 01769 LoRaMacStatus_t LoRaMacMibGetRequestConfirm( MibRequestConfirm_t *mibGet ); 01770 01771 /*! 01772 * \brief LoRaMAC MIB-Set 01773 * 01774 * \details The mac information base service to set attributes of the LoRaMac 01775 * layer. 01776 * 01777 * The following code-snippet shows how to use the API to set the 01778 * parameter AdrEnable, defined by the enumeration type 01779 * \ref MIB_ADR. 01780 * 01781 * \code 01782 * MibRequestConfirm_t mibReq; 01783 * mibReq.Type = MIB_ADR; 01784 * mibReq.Param.AdrEnable = true; 01785 * 01786 * if( LoRaMacMibGetRequestConfirm( &mibReq ) == LORAMAC_STATUS_OK ) 01787 * { 01788 * // LoRaMAC updated the parameter 01789 * } 01790 * \endcode 01791 * 01792 * \param [IN] mibRequest - MIB-SET-Request to perform. Refer to \ref MibRequestConfirm_t. 01793 * 01794 * \retval LoRaMacStatus_t Status of the operation. Possible returns are: 01795 * \ref LORAMAC_STATUS_OK, 01796 * \ref LORAMAC_STATUS_BUSY, 01797 * \ref LORAMAC_STATUS_SERVICE_UNKNOWN, 01798 * \ref LORAMAC_STATUS_PARAMETER_INVALID. 01799 */ 01800 LoRaMacStatus_t LoRaMacMibSetRequestConfirm( MibRequestConfirm_t *mibSet ); 01801 01802 /*! 01803 * \brief LoRaMAC MLME-Request 01804 * 01805 * \details The Mac layer management entity handles management services. The 01806 * following code-snippet shows how to use the API to perform a 01807 * network join request. 01808 * 01809 * \code 01810 * static uint8_t DevEui[] = 01811 * { 01812 * 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 01813 * }; 01814 * static uint8_t AppEui[] = 01815 * { 01816 * 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 01817 * }; 01818 * static uint8_t AppKey[] = 01819 * { 01820 * 0x2B, 0x7E, 0x15, 0x16, 0x28, 0xAE, 0xD2, 0xA6, 01821 * 0xAB, 0xF7, 0x15, 0x88, 0x09, 0xCF, 0x4F, 0x3C 01822 * }; 01823 * 01824 * MlmeReq_t mlmeReq; 01825 * mlmeReq.Type = MLME_JOIN; 01826 * mlmeReq.Req.Join.DevEui = DevEui; 01827 * mlmeReq.Req.Join.AppEui = AppEui; 01828 * mlmeReq.Req.Join.AppKey = AppKey; 01829 * 01830 * if( LoRaMacMlmeRequest( &mlmeReq ) == LORAMAC_STATUS_OK ) 01831 * { 01832 * // Service started successfully. Waiting for the Mlme-Confirm event 01833 * } 01834 * \endcode 01835 * 01836 * \param [IN] mlmeRequest - MLME-Request to perform. Refer to \ref MlmeReq_t. 01837 * 01838 * \retval LoRaMacStatus_t Status of the operation. Possible returns are: 01839 * \ref LORAMAC_STATUS_OK, 01840 * \ref LORAMAC_STATUS_BUSY, 01841 * \ref LORAMAC_STATUS_SERVICE_UNKNOWN, 01842 * \ref LORAMAC_STATUS_PARAMETER_INVALID, 01843 * \ref LORAMAC_STATUS_NO_NETWORK_JOINED, 01844 * \ref LORAMAC_STATUS_LENGTH_ERROR, 01845 * \ref LORAMAC_STATUS_DEVICE_OFF. 01846 */ 01847 LoRaMacStatus_t LoRaMacMlmeRequest( MlmeReq_t *mlmeRequest ); 01848 01849 /*! 01850 * \brief LoRaMAC MCPS-Request 01851 * 01852 * \details The Mac Common Part Sublayer handles data services. The following 01853 * code-snippet shows how to use the API to send an unconfirmed 01854 * LoRaMAC frame. 01855 * 01856 * \code 01857 * uint8_t myBuffer[] = { 1, 2, 3 }; 01858 * 01859 * McpsReq_t mcpsReq; 01860 * mcpsReq.Type = MCPS_UNCONFIRMED; 01861 * mcpsReq.Req.Unconfirmed.fPort = 1; 01862 * mcpsReq.Req.Unconfirmed.fBuffer = myBuffer; 01863 * mcpsReq.Req.Unconfirmed.fBufferSize = sizeof( myBuffer ); 01864 * 01865 * if( LoRaMacMcpsRequest( &mcpsReq ) == LORAMAC_STATUS_OK ) 01866 * { 01867 * // Service started successfully. Waiting for the MCPS-Confirm event 01868 * } 01869 * \endcode 01870 * 01871 * \param [IN] mcpsRequest - MCPS-Request to perform. Refer to \ref McpsReq_t. 01872 * 01873 * \retval LoRaMacStatus_t Status of the operation. Possible returns are: 01874 * \ref LORAMAC_STATUS_OK, 01875 * \ref LORAMAC_STATUS_BUSY, 01876 * \ref LORAMAC_STATUS_SERVICE_UNKNOWN, 01877 * \ref LORAMAC_STATUS_PARAMETER_INVALID, 01878 * \ref LORAMAC_STATUS_NO_NETWORK_JOINED, 01879 * \ref LORAMAC_STATUS_LENGTH_ERROR, 01880 * \ref LORAMAC_STATUS_DEVICE_OFF. 01881 */ 01882 LoRaMacStatus_t LoRaMacMcpsRequest( McpsReq_t *mcpsRequest ); 01883 01884 /*! \} defgroup LORAMAC */ 01885 01886 #endif // __LORAMAC_H__
Generated on Sun Jul 24 2022 08:02:16 by
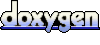