Allows mbed to send data to an HTML5 web browser through a 4 pole mini jack.
Embed:
(wiki syntax)
Show/hide line numbers
MicIO.h
00001 /* Mbed MicIO library 00002 * Copyright (c) 2014, Colin Bookman, cobookman [at] gmail [dot] com 00003 * 00004 * This program is free software: you can redistribute it and/or modify 00005 * it under the terms of the GNU General Public License as published by 00006 * the Free Software Foundation, either version 3 of the License, or 00007 * (at your option) any later version. 00008 * 00009 * This program is distributed in the hope that it will be useful, 00010 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00011 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00012 * GNU General Public License for more details. 00013 * 00014 * You should have received a copy of the GNU General Public License 00015 * along with this program. If not, see <http://www.gnu.org/licenses/>. 00016 */ 00017 00018 #ifndef MBED_MICIO_H 00019 #define MBED_MICIO_H 00020 00021 #include "mbed.h" 00022 00023 /** 00024 * @code 00025 * #include "MicIO.h" 00026 * 00027 * MicIO micIO(p18,p19); //AnalogOut (Output - Mic), AnalogIn (Input Headphone Left/Right) 00028 * 00029 * int main() { 00030 * micIO.send("HI WORLD", 8); 00031 * micIO.send([0xFF, 0xFA, 0xFB, 0x00], 4); 00032 * } 00033 * @endcode 00034 */ 00035 class MicIO { 00036 public: 00037 00038 /** Create a MicIO instance */ 00039 MicIO(PinName output, PinName input); 00040 float clockPeriod; 00041 00042 /* Send byte array through micIO */ 00043 void send(const char * arr, int length); 00044 00045 /* Send just 4 bits */ 00046 void send4Bits(unsigned char byte); 00047 00048 /* 00049 Read the current value of the input clock. 00050 1 = high 00051 0 = low 00052 -1 = unchanged 00053 */ 00054 int clock(); 00055 00056 /* Extracts the lower 4 bits of a byte */ 00057 unsigned char lower4Bits(unsigned char byte); 00058 /* Extracts the upper 4 bits of a byte */ 00059 unsigned char upper4Bits(unsigned char byte); 00060 00061 protected: 00062 AnalogOut _micOut; 00063 AnalogIn _clockIn; 00064 float _sinTable[361]; //361 in case of overflow...not that it SHOULD happen 00065 /* 00066 sendSin outputs a sin wave for 1 period. 00067 ------------------------- 00068 |Speed | | 00069 |Factor | Frequency | 00070 |------------------------ 00071 | 4.75 | 5.73Khz | 00072 | 4.50 | 5.45Khz | 00073 | 4.25 | 5.13Khz | 00074 | 4.00 | 4.84Khz | 00075 | 3.75 | 4.54Khz | 00076 | 3.50 | 4.23Khz | 00077 | 3.25 | 3.92Khz | 00078 | 3.00 | 3.65-3.62Khz| 00079 | 2.75 | 3.33Khz | 00080 | 2.50 | 3Khz | 00081 | 2.25 | 2.73Khz | 00082 | 2.00 | 2.41Khz | 00083 | 1.75 | 2.12Khz | 00084 | 1.50 | 1.818Khz | 00085 | 1.25 | 1.5Khz | 00086 | 1.00 | 1.2Khz | 00087 | 0.75 | 0.909KHz | 00088 | 0.5 | 0.606Khz | 00089 ------------------------- 00090 */ 00091 void _sendSin(float sinSeed); //sinSeed of 1 = 5khz 00092 00093 /* Get the number of cycles the sin wave should run for */ 00094 int _numCycles(float sinSeed); 00095 00096 /* Generate a sin Table */ 00097 void _genSinTable(); 00098 00099 /* Go from 4 bits to a sin seed */ 00100 float _getSinSeed(unsigned char bits4); 00101 00102 }; 00103 00104 #endif
Generated on Wed Jul 13 2022 03:37:26 by
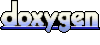