UIPEthernet library for Arduino IDE, Eclipse with arduino plugin and MBED/SMeshStudio (AVR,STM32F,ESP8266,Intel ARC32,Nordic nRF51,Teensy boards,Realtek Ameba(RTL8195A,RTL8710)), ENC28j60 network chip. Compatible with Wiznet W5100 Ethernet library API. Compiled and tested on Nucleo-F302R8. Master repository is: https://github.com/UIPEthernet/UIPEthernet/
Dns.h
00001 // Arduino DNS client for Enc28J60-based Ethernet shield 00002 // (c) Copyright 2009-2010 MCQN Ltd. 00003 // Released under Apache License, version 2.0 00004 00005 #ifndef DNSClient_h 00006 #define DNSClient_h 00007 00008 #include "utility/uipopt.h" 00009 #if UIP_UDP 00010 #include "UIPUdp.h" 00011 00012 class DNSClient 00013 { 00014 public: 00015 // ctor 00016 void begin(const IPAddress& aDNSServer); 00017 00018 /** Convert a numeric IP address string into a four-byte IP address. 00019 @param aIPAddrString IP address to convert 00020 @param aResult IPAddress structure to store the returned IP address 00021 @result 1 if aIPAddrString was successfully converted to an IP address, 00022 else error code 00023 */ 00024 int inet_aton(const char *aIPAddrString, IPAddress& aResult); 00025 00026 /** Resolve the given hostname to an IP address. 00027 @param aHostname Name to be resolved 00028 @param aResult IPAddress structure to store the returned IP address 00029 @result 1 if aIPAddrString was successfully converted to an IP address, 00030 else error code 00031 */ 00032 int getHostByName(const char* aHostname, IPAddress& aResult); 00033 00034 protected: 00035 uint16_t BuildRequest(const char* aName); 00036 int16_t ProcessResponse(uint16_t aTimeout, IPAddress& aAddress); 00037 00038 IPAddress iDNSServer; 00039 uint16_t iRequestId; 00040 UIPUDP iUdp; 00041 }; 00042 #endif 00043 00044 #endif
Generated on Tue Jul 12 2022 19:00:50 by
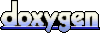