
Using touch screen features of LCD screen.
Dependencies: DmTouch_UniGraphic UniGraphic mbed
Fork of DisplayModule24_demo by
main.cpp
00001 #include "stdio.h" 00002 #include "mbed.h" 00003 #include "string" 00004 #include "ILI932x.h" 00005 #include "DmTouch.h" 00006 00007 00008 #include "Arial12x12.h" 00009 #include "Arial24x23.h" 00010 #include "pavement_48x34.h" 00011 00012 Serial pc(USBTX, USBRX); 00013 00014 /* Configure the DisplayModule ILI9325 2.4" display for 8-bit bus communication 00015 00016 mbed pin display pin 00017 -------- ----------- 00018 p15 CS (L15) 00019 p17 RST (L17) 00020 p16 RS (L4) 00021 p14 WR (L5) 00022 p20 RD (L6) 00023 00024 p30 DB8 (L7) 00025 p29 DB9 (L8) 00026 p28 DB10 (L9) 00027 p27 DB11 (L10) 00028 p26 DB12 (L11) 00029 p25 DB13 (L12) 00030 p24 DB14 (L13) 00031 p23 DB15 (L14) 00032 00033 p1 GND (L1) 00034 p40 Vin (L2) 00035 p40 LED Backlight (L19) 00036 */ 00037 PinName dataBus[]= {p30, p29, p28, p27, p26, p25, p24, p23}; 00038 ILI932x myLCD(BUS_8, dataBus, p15, p17, p16, p14, p20, "myLCD", 240, 320); // Bus 8 bit, bus pin array, CS, RST, DC, WR, RD, name, xpixels, ypixels 00039 00040 /* Additional connections to add touch response 00041 00042 mbed pin display pin 00043 -------- ----------- 00044 p5 T_MOSI (R11) 00045 p6 T_MISO (R13) 00046 p7 T_CLK (R9) 00047 p8 T_CS (R10) 00048 p9 T_IRQ (R14) 00049 */ 00050 DmTouch touch(DmTouch::DM_TFT24_104, p5, p6, p7, p8, p9); 00051 00052 char orient=3; 00053 int x,y; 00054 uint16_t tx, ty; 00055 Timer t; 00056 00057 int main() 00058 { 00059 bool down, lastDown; 00060 touch.init(); 00061 t.start(); 00062 myLCD.set_orientation(orient); 00063 myLCD.set_font((unsigned char*) Arial24x23); 00064 myLCD.background(Blue); // set background to Blue 00065 myLCD.foreground(White); // set chars to White 00066 myLCD.cls(); // clear the screen 00067 myLCD.locate(10,30); 00068 myLCD.printf("UniGraphics Demo\r\n"); 00069 wait(2); 00070 00071 while(1) 00072 { 00073 myLCD.cls(); // clear the screen 00074 myLCD.set_font((unsigned char*) Arial24x23); 00075 myLCD.locate(0,30); 00076 myLCD.printf("Orientation mode: %x\r\n", orient%4); 00077 wait(2); 00078 myLCD.printf("Font is\r\n"); 00079 myLCD.printf("Arial24x23\r\n"); 00080 wait(2); 00081 00082 myLCD.background(Black); 00083 myLCD.foreground(White); 00084 myLCD.cls(); // clear the screen 00085 myLCD.set_font((unsigned char*) Arial12x12); 00086 myLCD.locate(0,10); 00087 myLCD.printf("Font changed to Arial12x12\r\n"); 00088 myLCD.printf("Background and foreground color also changed.\n\r\n\r"); 00089 wait(2); 00090 myLCD.printf("Notice that if some text is too long to fit the width of the screen that it is automatically wrapped to the next line.\r\n\r\n"); 00091 wait(2); 00092 myLCD.set_font((unsigned char*) Terminal6x8); 00093 myLCD.printf("Font changed to Terminal6x8\r\n"); 00094 myLCD.printf("This is pretty small!\r\n"); 00095 wait(2); 00096 00097 myLCD.cls(); 00098 myLCD.set_font((unsigned char*) Arial24x23); 00099 myLCD.locate(10,10); 00100 myLCD.printf("Draw lines\n\r"); 00101 myLCD.line(0,50,myLCD.width()-1,50,Yellow); 00102 myLCD.line(myLCD.width()-50,51,myLCD.width()-50,myLCD.height()-1,Green); 00103 wait(2); 00104 00105 myLCD.cls(); 00106 myLCD.locate(10,10); 00107 myLCD.printf("Draw rectangles"); 00108 myLCD.rect(10,50,50,80,Red); 00109 myLCD.rect(15,55,45,75,Red); 00110 myLCD.rect(20,60,40,70,Red); 00111 myLCD.fillrect(160,130,200,240,Blue); 00112 wait(2); 00113 00114 myLCD.cls(); 00115 myLCD.locate(10,10); 00116 myLCD.printf("Draw circles"); 00117 myLCD.circle(150,132,30,Yellow); 00118 myLCD.fillcircle(140,70,25,Cyan); 00119 wait(2); 00120 00121 myLCD.cls(); 00122 myLCD.set_font((unsigned char*) Arial12x12); 00123 myLCD.locate(10,10); 00124 myLCD.printf("Draw function with pixels"); 00125 double s; 00126 for (unsigned short i=0; i<myLCD.width(); i++) 00127 { 00128 s =10 * sin((long double) i / 10 ); 00129 myLCD.pixel(i,80 + (int)s ,White); 00130 } 00131 wait(3); 00132 00133 // Touch screen demo 00134 myLCD.background(Blue); // set background to Blue 00135 myLCD.foreground(White); // set chars to White 00136 myLCD.cls(); // clear the screen 00137 myLCD.locate(10,30); 00138 myLCD.set_font((unsigned char*) Arial24x23); 00139 myLCD.printf("DmTouch Demo\r\n"); 00140 myLCD.set_font((unsigned char*) Arial12x12); 00141 myLCD.locate(10,70); 00142 myLCD.printf("Coming soon...\r\nTouch screen and coordinates will display\r\n"); 00143 myLCD.printf("Moves to next portion of demo after 30 seconds\r\n"); 00144 wait(2); 00145 myLCD.background(Black); // set background to Black 00146 myLCD.foreground(White); // set chars to White 00147 myLCD.cls(); // clear the screen 00148 00149 touch.setOrientation(orient); 00150 down = false; 00151 lastDown = false; 00152 tx = (uint16_t)0; 00153 ty = (uint16_t)0; 00154 myLCD.locate(20,20); 00155 myLCD.printf("x:"); 00156 myLCD.locate(100, 20); 00157 myLCD.printf("y:"); 00158 00159 t.reset(); 00160 while (t.read()<30) { 00161 touch.readTouchData(tx, ty, down); 00162 if (down) { 00163 myLCD.locate(40, 20); 00164 myLCD.printf("%5i", tx); 00165 myLCD.locate(120, 20); 00166 myLCD.printf("%5i", ty); 00167 myLCD.fillcircle(tx, ty, 2, Red); 00168 } else if (lastDown) { 00169 // no longer pressed, clean text 00170 myLCD.locate(40, 20); 00171 myLCD.printf(" ", tx); 00172 myLCD.locate(120, 20); 00173 myLCD.printf(" ", ty); 00174 } 00175 wait(0.040); 00176 lastDown = down; 00177 } 00178 00179 00180 // scroll test, only for TFT 00181 myLCD.cls(); 00182 myLCD.set_font((unsigned char*) Arial24x23); 00183 myLCD.locate(2,10); 00184 myLCD.printf("Scrolling"); 00185 myLCD.rect(0,0,myLCD.width()-1,myLCD.height()-1,White); 00186 myLCD.rect(1,1,myLCD.width()-2,myLCD.height()-2,Blue); 00187 myLCD.setscrollarea(0,myLCD.sizeY()); 00188 wait(1); 00189 myLCD.scroll(1); //up 1 00190 wait(1); 00191 myLCD.scroll(0); //center 00192 wait(1); 00193 myLCD.scroll(myLCD.sizeY()-1); //down 1 00194 wait(1); 00195 myLCD.scroll(myLCD.sizeY()); // same as 0, center 00196 wait(1); 00197 myLCD.scroll(myLCD.sizeY()>>1); // half screen 00198 wait(1); 00199 myLCD.scrollreset(); // center 00200 wait(1); 00201 for(unsigned short i=1; i<=myLCD.sizeY(); i++) 00202 { 00203 myLCD.scroll(i); 00204 wait_ms(2); 00205 } 00206 wait(2); 00207 // color inversion 00208 for(unsigned short i=0; i<=8; i++) 00209 { 00210 myLCD.invert(i&1); 00211 wait_ms(200); 00212 } 00213 wait(2); 00214 // bmp 16bit test 00215 myLCD.cls(); 00216 for(int y=0; y<myLCD.height(); y+=34) 00217 { 00218 for(int x=0; x<myLCD.width(); x+=48) myLCD.Bitmap(x,y,48,34,(unsigned char *)pavement_48x34); 00219 } 00220 wait(2); 00221 myLCD.set_orientation((++orient)%4); 00222 } 00223 }
Generated on Mon Jul 18 2022 16:14:28 by
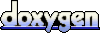