
control the laser with the MBED
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "CommandProcessor.h" 00003 #include "prefs.h" 00004 00005 float __float_reg[64]; 00006 int __int_reg[64]; 00007 00008 DigitalOut led(LED1); 00009 InterruptIn in(PA_10); 00010 PwmOut out(PA_8); 00011 00012 Serial pc(USBTX, USBRX); 00013 00014 char linebuf[128]; 00015 int index = 0; 00016 void rxCallback() { 00017 while (pc.readable()) { 00018 char c = pc.getc(); 00019 if (c != 127 && c != 8 && c != '\r' && c != '\t') { 00020 linebuf[index] = c; 00021 if (index < 127) index++; 00022 if (c < 128) pc.putc(c); 00023 } else if (c == 127 || c == 8) { 00024 if (index > 0) { 00025 index--; 00026 //BS (8) should delete previous char 00027 pc.putc(127); 00028 } 00029 } else if (c == '\r') { 00030 linebuf[index] = 0; 00031 if (index > 0) { 00032 pc.putc(c); 00033 processCmd(&pc, linebuf); 00034 pc.putc('>'); 00035 } else { 00036 pc.putc(c); 00037 pc.putc('>'); 00038 } 00039 index = 0; 00040 } 00041 } 00042 } 00043 00044 void turn_on() { 00045 out.pulsewidth_us(_PULSE_WIDTH); 00046 led = 1; 00047 } 00048 00049 void turn_off() { 00050 out.pulsewidth_us(0); 00051 led = 0; 00052 } 00053 00054 int main() { 00055 pc.baud(115200); 00056 pc.attach(rxCallback); 00057 pc.printf("PYROFLEX\n"); 00058 cmd_clear(&pc); 00059 cmd_defaults(&pc); 00060 pc.printf(">"); 00061 00062 out.period_us(10); 00063 out.pulsewidth_us(_PULSE_WIDTH); 00064 00065 in.rise(turn_on); 00066 in.fall(turn_off); 00067 00068 for (;;) {} 00069 }
Generated on Sat Aug 27 2022 17:05:16 by
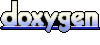