
control the laser with the MBED
Embed:
(wiki syntax)
Show/hide line numbers
cmd_set_get.cpp
00001 #include "mbed.h" 00002 #include "CommandProcessor.h" 00003 00004 #include "defaults.h" 00005 #include "prefs.h" 00006 #include "globals.h" 00007 00008 void cmd_ls(Serial *pc) { 00009 DPRINT(PULSE_WIDTH); 00010 DPRINT(PULSE_FREQ); 00011 } 00012 00013 #define ls_specialf(a) if (strcmp(buf, #a) == 0) {pc->printf("%s: %f\n", #a, a); return;} 00014 #define ls_speciald(a) if (strcmp(buf, #a) == 0) {pc->printf("%s: %d\n", #a, a); return;} 00015 void cmd_ls2(Serial *pc, char *buf) { 00016 float *fptr = checkf(buf); 00017 if (fptr != NULL) pc->printf("%s: %f\n", buf, *fptr); 00018 int *nptr = NULL; 00019 if (fptr == NULL) nptr = checkn(buf); 00020 if (nptr != NULL) pc->printf("%s: %d\n", buf, *nptr); 00021 if (nptr == NULL && fptr == NULL) pc->printf("%s\n", "No Such Parameter"); 00022 } 00023 00024 void cmd_defaults(Serial *pc) { 00025 DEFAULT(PULSE_WIDTH); 00026 DEFAULT(PULSE_FREQ); 00027 pc->printf("Defaults Loaded\n"); 00028 } 00029 00030 void cmd_set(Serial *pc, char *buf, char *val) { 00031 float *fptr = checkf(buf); 00032 if (fptr != NULL) *fptr = (float) (atof(val)); 00033 int *nptr = NULL; 00034 if (fptr == NULL) nptr = checkn(buf); 00035 if (nptr != NULL) *nptr = (int) (atoi(val)); 00036 if (nptr != NULL || fptr != NULL) cmd_ls2(pc, buf); 00037 if (nptr == NULL && fptr == NULL) pc->printf("%s\n", "No Such Parameter"); 00038 00039 if (strcmp(buf, "PULSE_FREQ") == 0) { 00040 out.period_us(1000 / _PULSE_FREQ); 00041 } 00042 }
Generated on Sat Aug 27 2022 17:05:16 by
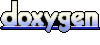