
flash based config testing
Embed:
(wiki syntax)
Show/hide line numbers
cmd_set_get.cpp
00001 #include "mbed.h" 00002 #include "CommandProcessor.h" 00003 #include "PreferenceWriter.h" 00004 #include "globals.h" 00005 00006 #include "config.h" 00007 #include "config_driving.h" 00008 #include "config_inverter.h" 00009 #include "config_logging.h" 00010 #include "config_loop.h" 00011 #include "config_motor.h" 00012 #include "config_pins.h" 00013 #include "config_table.h" 00014 00015 void cmd_ls(Serial *pc) { 00016 DPRINT(TORQUE_MODE); 00017 FPRINT(MAX_TQPCT_PLUS); 00018 FPRINT(MAX_TQPCT_MINUS); 00019 FPRINT(TORQUE_MAX); 00020 FPRINT(W_MAX); 00021 FPRINT(BUS_VOLTAGE); 00022 FPRINT(F_SW); 00023 FPRINT(K_LOOP_D); 00024 FPRINT(KI_BASE_D); 00025 FPRINT(K_LOOP_Q); 00026 FPRINT(KI_BASE_Q); 00027 FPRINT(F_SLOW_LOOP); 00028 FPRINT(INTEGRAL_MAX); 00029 FPRINT(W_FILTER_STRENGTH); 00030 FPRINT(DQ_FILTER_STRENGTH); 00031 FPRINT(THROTTLE_FILTER_STRENGTH); 00032 FPRINT(KP_D); 00033 FPRINT(KI_D); 00034 FPRINT(KP_Q); 00035 FPRINT(KI_Q); 00036 FPRINT(SLOW_LOOP_COUNTER); 00037 FPRINT(POLE_PAIRS); 00038 FPRINT(POS_OFFSET); 00039 FPRINT(RESOLVER_LOBES); 00040 DPRINT(CPR); 00041 FPRINT(Ld); 00042 FPRINT(Lq); 00043 FPRINT(FLUX_LINKAGE); 00044 FPRINT(Rs); 00045 FPRINT(KT); 00046 FPRINT(W_SAFE); 00047 FPRINT(W_CRAZY); 00048 DPRINT(TH_LIMIT_LOW); 00049 DPRINT(TH_LIMIT_HIGH); 00050 DPRINT(TH_LIMIT_CRAZY); 00051 DPRINT(ROWS); 00052 DPRINT(COLUMNS); 00053 FPRINT(W_STEP); 00054 } 00055 00056 void cmd_ls2(Serial *pc, char *buf) { 00057 if (strcmp(buf, "mode") == 0) { 00058 pc->printf("%s\n", mode_to_str(BREMS_mode)); 00059 return; 00060 } 00061 if (strcmp(buf, "src") == 0) { 00062 pc->printf("%s\n", src_to_str(BREMS_src)); 00063 return; 00064 } 00065 if (strcmp(buf, "op") == 0) { 00066 pc->printf("%s\n", op_to_str(BREMS_op)); 00067 return; 00068 } 00069 00070 float *fptr = checkf(buf); 00071 if (fptr != NULL) pc->printf("%s: %f\n", buf, *fptr); 00072 int *nptr = NULL; 00073 if (fptr == NULL) nptr = checkn(buf); 00074 if (nptr != NULL) pc->printf("%s: %d\n", buf, *nptr); 00075 if (nptr == NULL && fptr == NULL) pc->printf("%s\n", "No Such Parameter"); 00076 } 00077 00078 void cmd_defaults(Serial *pc) { 00079 DEFAULT(TORQUE_MODE); 00080 DEFAULT(MAX_TQPCT_PLUS); 00081 DEFAULT(MAX_TQPCT_MINUS); 00082 DEFAULT(TORQUE_MAX); 00083 DEFAULT(W_MAX); 00084 DEFAULT(BUS_VOLTAGE); 00085 DEFAULT(F_SW); 00086 DEFAULT(K_LOOP_D); 00087 DEFAULT(KI_BASE_D); 00088 DEFAULT(K_LOOP_Q); 00089 DEFAULT(KI_BASE_Q); 00090 DEFAULT(F_SLOW_LOOP); 00091 DEFAULT(INTEGRAL_MAX); 00092 DEFAULT(W_FILTER_STRENGTH); 00093 DEFAULT(DQ_FILTER_STRENGTH); 00094 DEFAULT(THROTTLE_FILTER_STRENGTH); 00095 DEFAULT(KP_D); 00096 DEFAULT(KI_D); 00097 DEFAULT(KP_Q); 00098 DEFAULT(KI_Q); 00099 DEFAULT(SLOW_LOOP_COUNTER); 00100 DEFAULT(POLE_PAIRS); 00101 DEFAULT(POS_OFFSET); 00102 DEFAULT(RESOLVER_LOBES); 00103 DEFAULT(CPR); 00104 DEFAULT(Ld); 00105 DEFAULT(Lq); 00106 DEFAULT(FLUX_LINKAGE); 00107 DEFAULT(Rs); 00108 DEFAULT(KT); 00109 DEFAULT(W_SAFE); 00110 DEFAULT(W_CRAZY); 00111 DEFAULT(TH_LIMIT_LOW); 00112 DEFAULT(TH_LIMIT_HIGH); 00113 DEFAULT(TH_LIMIT_CRAZY); 00114 DEFAULT(ROWS); 00115 DEFAULT(COLUMNS); 00116 DEFAULT(W_STEP); 00117 pc->printf("Defaults Loaded\n"); 00118 } 00119 00120 void cmd_reload(Serial *pc, PreferenceWriter *pref) { 00121 pref->load(); 00122 pc->printf("Flash Values Loaded\n"); 00123 } 00124 00125 void cmd_set(Serial *pc, char *buf, char *val) { 00126 float *fptr = checkf(buf); 00127 if (fptr != NULL) *fptr = (float) (atof(val)); 00128 int *nptr = NULL; 00129 if (fptr == NULL) nptr = checkn(buf); 00130 if (nptr != NULL) *nptr = (int) (atoi(val)); 00131 cmd_ls2(pc, buf); 00132 if (nptr == NULL && fptr == NULL) pc->printf("%s\n", "No Such Parameter"); 00133 } 00134 00135 void cmd_flush(Serial *pc, PreferenceWriter *pref) { 00136 if (!pref->ready()) pref->open(); 00137 pref->flush(); 00138 }
Generated on Wed Jul 13 2022 04:37:22 by
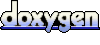