
teste de publish
Dependencies: DS1820 HighSpeedAnalogIn devices mbed
modem.cpp
00001 #include "modem.h" 00002 00003 modemStatus modemCom::status; 00004 char* modemCom::bufIn; //Ponteiro para buffer de entrada do modem; 00005 uint32_t modemCom::timeOutModem; 00006 bool modemCom::atendendoWebServer; 00007 bool modemCom::exibeEntradaPacote; 00008 int16_t numeroDeBytesPorEnvio; 00009 uint16_t modemCom::timeOutEnvioDados; 00010 uint8_t connID; 00011 uint16_t IPDNumBytes; 00012 bool enviaSendData; 00013 char *bufInPtr; 00014 bool mudaRede = false; 00015 char webServerBuff[256]; 00016 00017 void modemCom::atendeSRING(uint8_t conId){ 00018 //pc.printf("Atendendo a conexao entrante.\n"); 00019 00020 if(modemCom::status.emComunicacao){ 00021 modemCom::cipSend(conId,"ocupado",7); 00022 pc.printf("Fechando socket chave 1.\r\n"); 00023 modemCom::closeConnection(&modemCom::status.connIDServerCommand); 00024 modemCom::closeConnection(&modemCom::status.connIDWebServer); 00025 modemCom::closeConnection(&modemCom::status.connIDSendData); 00026 } 00027 00028 modemCom::status.socketEntranteAtivo=true; 00029 modemCom::status.timeOut = 30; 00030 modemCom::timeOutModem = 10; 00031 00032 //pc.printf("Entrei em modemCom::atendeSRING.\r\n"); 00033 00034 while(modemCom::status.timeOut){ 00035 if(modemCom::status.timeOut){modemCom::status.timeOut--;} 00036 /*//Aqui devo perguntar e pedir os dados em buffer 00037 */ 00038 osDelay(100); 00039 } 00040 00041 //Para parar de colocar dentro do arquivo 00042 modemCom::status.recebendoArquivoDoServer = false; 00043 00044 modemCom::status.serverConnected=0; 00045 modemCom::timeOutModem = 10; 00046 00047 //Fechando o socket 00048 pc.printf("Fechando socket chave 2.\r\n"); 00049 modemCom::closeConnection(&modemCom::status.connIDServerCommand); 00050 modemCom::closeConnection(&modemCom::status.connIDWebServer); 00051 modemCom::closeConnection(&modemCom::status.connIDSendData); 00052 modemCom::status.socketEntranteAtivo=false; 00053 modemCom::status.emComunicacao = false; 00054 modemCom::timeOutModem = 10; 00055 } 00056 00057 bool modemCom::sendBufferCommandMode(uint8_t sId, char *buffer, uint16_t bufferLength){ 00058 char aux[25]; 00059 uint16_t numeroByteSendoEnviado; 00060 00061 sprintf(aux,"AT+CIPSEND=%u,%lu\r\n",sId,bufferLength); 00062 if(modemCom::sendToModem(aux,1,&modemCom::status.PROMPT_ENVIO_COMMAND_MODE,NULL,500,2,1)) { 00063 for(numeroByteSendoEnviado = 0; numeroByteSendoEnviado<bufferLength; numeroByteSendoEnviado++) { 00064 modem.printf("%c",buffer[numeroByteSendoEnviado]); 00065 if(debug){ 00066 pc.printf("%c",buffer[numeroByteSendoEnviado]); 00067 } 00068 00069 } 00070 numeroByteSendoEnviado = modemCom::sendToModem(NULL,1,&modemCom::status.SEND_OK,NULL,500,1,1); 00071 }else{ 00072 numeroByteSendoEnviado = false; //Reaproveitando numeroByteSendoEnviado para resultado de retorno 00073 } 00074 return numeroByteSendoEnviado; //Reaproveitando numeroByteSendoEnviado para resultado de retorno 00075 } 00076 00077 void modemCom::leMAC(void) 00078 { 00079 char *ptr; 00080 if(modemCom::sendToModem("AT+CIPSTAMAC?\r\n",1,&modemCom::status.modemResponse,NULL,500,2,1)){ 00081 //resposta: +CIPSTAMAC:"18:fe:34:f4:5f:c4" 00082 ptr = strtok(bufModem.getRowBuffer(),"\""); 00083 ptr = strtok(NULL,"\""); 00084 if(ptr) { 00085 strcpy(modemCom::status.MAC,ptr); 00086 //pc.printf("Lido MAC <%s>.\n",modemCom::status.MAC); 00087 } 00088 } 00089 } 00090 00091 00092 00093 bool modemCom::writeStatusToSD(void){ 00094 char aux[50],*ptr; 00095 int16_t RSSI; 00096 RSSI = modemCom::getRSSI(); 00097 sdCardBuf.del(); 00098 sdCardBuf.fill("log{status:ip=255.255.255.255;csq=",34); 00099 00100 sprintf(aux,"%d",RSSI); 00101 sdCardBuf.fill(aux,strlen(aux)); 00102 00103 00104 00105 sprintf(aux,";fv=%s",firmVersion); 00106 sdCardBuf.fill(aux,strlen(aux)); 00107 00108 sprintf(aux,";rc=%s",resetCauses); 00109 sdCardBuf.fill(aux,strlen(aux)); 00110 00111 sprintf(aux,";imsi=%s}log",modemCom::status.MAC); 00112 sdCardBuf.fill(aux,strlen(aux)); 00113 00114 //Escrevendo uma única vez o WDT 00115 ptr = strstr(resetCauses,",WDT"); 00116 if(ptr!=NULL){ 00117 ptr[0]=0; 00118 }; 00119 00120 //Escrevendo uma única vez o WDT 00121 ptr = strstr(resetCauses,",NOW"); 00122 if(ptr!=NULL){ 00123 ptr[0]=0; 00124 }; 00125 00126 return sdCard::insereDadosBank(sdCardBuf.get(),sdCardBuf.getLength()); 00127 } 00128 00129 bool modemCom::montaStatus(void) 00130 { 00131 if(sdCard::abreArquivo(&sdCard::status,"w")) { 00132 fprintf(sdCard::status.fp,"log{status:ip:255.255.255.255;csq:30;imsi:%s}log",modemCom::status.MAC); 00133 sdCard::fechaArquivo(&sdCard::status); 00134 sdCard::exibeArquivo(&sdCard::status); 00135 } else { 00136 return 0; 00137 } 00138 return true; 00139 } 00140 00141 00142 00143 uint8_t modemCom::enviaDados() 00144 { 00145 uint8_t result=false; 00146 pc.printf("modemCom::enviaDados[1].\r\n"); 00147 if(!sdCard::preparaEnvio()){ 00148 pc.printf("modemCom::enviaDados[2].\r\n"); 00149 //pc.printf("Nao foi possivel preparar o envio.\n"); 00150 return false; 00151 } 00152 sdCard::verificaCurrentBank(); 00153 pc.printf("modemCom::enviaDados[3], currentBank = %lu currentBankSending = %lu.\r\n",sdCard::currentBank,sdCard::currentBankSending); 00154 sdCard::getFileTam(&sdCard::bank0); 00155 sdCard::getFileTam(&sdCard::bank1); 00156 if((sdCard::bank0.bytes > 230400) || (sdCard::bank1.bytes > 230400)){ 00157 pc.printf("Arquivo muito grande. Deletado."); 00158 sdCard::excluiArquivo(&sdCard::currentBankFile); 00159 sdCard::excluiArquivo(&sdCard::bank0); 00160 sdCard::excluiArquivo(&sdCard::bank1); 00161 return false; 00162 } 00163 switch(sdCard::currentBankSending){ 00164 case 0: 00165 if(sdCard::currentBank==0){ 00166 sdCard::modificaCurrentBank(1,0); 00167 } 00168 pc.printf("modemCom::enviaDados[4].\r\n"); 00169 00170 if(!sdCard::getFileTam(&sdCard::bank0)){ 00171 sdCard::modificaCurrentBank(0,1); 00172 }else{ 00173 pc.printf("modemCom::enviaDados[11].\r\n"); 00174 if(modemCom::postFileCommandMode(modemCom::status.serverIP,modemCom::status.host,"/drome/Parser/",&sdCard::bank0)){ 00175 //if(modemCom::postFileCommandMode(modemCom::status.serverIP,modemCom::status.host,"/sistema/Parser/",&sdCard::bank0)){ 00176 pc.printf("Enviado o bank0.\r\n"); 00177 result = true; 00178 } 00179 } 00180 00181 break; 00182 case 1: 00183 if(sdCard::currentBank==1){ 00184 sdCard::modificaCurrentBank(0,1); 00185 } 00186 pc.printf("modemCom::enviaDados[5].\r\n"); 00187 if(!sdCard::getFileTam(&sdCard::bank1)){ 00188 sdCard::modificaCurrentBank(1,0); 00189 }else{ 00190 pc.printf("modemCom::enviaDados[12].\r\n"); 00191 if(modemCom::postFileCommandMode(modemCom::status.serverIP,modemCom::status.host,"/drome/Parser/",&sdCard::bank1)){ 00192 //if(modemCom::postFileCommandMode(modemCom::status.serverIP,modemCom::status.host,"/sistema/Parser/",&sdCard::bank1)){ 00193 pc.printf("Enviado o bank1.\r\n"); 00194 result = true; 00195 } 00196 } 00197 break; 00198 case 2: 00199 pc.printf("modemCom::enviaDados[10].\r\n"); 00200 result = false; 00201 break; 00202 } 00203 return result; 00204 } 00205 00206 00207 00208 uint8_t modemCom::postFileCommandMode(char *serverIP, char *host, char *uri, arquivoSD *arquivo) 00209 { 00210 #define maxRetentativasEnvioParteArquivo 20 00211 uint32_t numeroDePartes; 00212 uint16_t restoDoEnvio; 00213 int32_t parteSendoEnviada; 00214 uint16_t numeroByteSendoEnviado; 00215 uint16_t maxTentativas=0; 00216 //unsigned int result; 00217 char header[512]; 00218 char aux[40]; 00219 uint16_t headerTam; 00220 uint32_t dataTam=0; 00221 char c; 00222 bool passaParaProximaParte = false; 00223 char nomeArquivo[50]; 00224 uint32_t parteEnviadaAntes = 0; 00225 sdCard::deleteSentFiles = false; 00226 00227 if(strstr(arquivo->nome,"bank0.txt")||strstr(arquivo->nome,"bank1.txt")){ 00228 strcpy(nomeArquivo,"/sd/RAD/envio.txt"); 00229 }else{ 00230 strcpy(nomeArquivo,arquivo->nome); 00231 } 00232 00233 //Para garantir que não seja duvidoso proveniente de outra conexão. 00234 modemCom::status.ServerAck=0; 00235 00236 modemCom::status.dnsError=0; 00237 00238 pc.printf("Iniciando envio de arquivo.\r\n"); 00239 if(arquivo->bytes==0){ 00240 if((!sdCard::getFileTam(arquivo))||(arquivo->bytes == 0)){ 00241 if(arquivo->bytes == 0){ 00242 sdCard::excluiArquivo(arquivo); 00243 } 00244 return false; 00245 } 00246 } 00247 00248 pc.printf("Abrindo o socket em <%s>.\n",serverIP); 00249 modemCom::status.ALREADY_CONNECTED=0; 00250 sprintf(header,"AT+CIPSTART=1,\"TCP\",\"%s\",80\r\n",serverIP); 00251 if(modemCom::sendToModem(header,1,&modemCom::status.OK,NULL,20000,3,1000)){ 00252 00253 pc.printf("Aberto o socket em command mode.\n"); 00254 pc.printf("Enviando arquivo com nome %s.\n",nomeArquivo); 00255 modemCom::status.connIDSendData=1; 00256 modemCom::status.serverConnected=1; 00257 modemCom::timeOutModem = 50; 00258 modemCom::status.emComunicacao = true; 00259 } else { 00260 pc.printf("Nao foi possivel Abrir o socket. Abortando tentativa.\n"); 00261 if(modemCom::status.ALREADY_CONNECTED){ 00262 //Socket aberto, fecho-o antes de partir. 00263 modemCom::closeConnection(&modemCom::status.connIDSendData); 00264 } 00265 modemCom::timeOutModem = 20; 00266 modemCom::status.emComunicacao = false; 00267 return 0; 00268 } 00269 00270 numeroDePartes = arquivo->bytes / numeroDeBytesPorEnvio; 00271 restoDoEnvio = arquivo->bytes % numeroDeBytesPorEnvio; 00272 00273 00274 if(xeretaModem){ 00275 pc.printf("Iniciando o envio do arquivo de %lu bytes\nnumeroDePartes = %lu\nrestoDoEnvio = %lu\n",arquivo->bytes,numeroDePartes,restoDoEnvio); 00276 } 00277 00278 if(!sdCard::abreArquivo(arquivo,"r")){ 00279 pc.printf("Nao foi posssivel abrir o arquivo de dentro da funcao postFileCommandMode().\n"); 00280 modemCom::status.emComunicacao = false; 00281 return 0; 00282 } 00283 00284 00285 //Monta Header aqui! 00286 //application/octet-stream 00287 sprintf(header,"--xxBOUNDARYxx\r\nContent-Type: text/plain\r\nContent-Disposition: form-data; name=\"IMEI\"\r\n\r\n%s\r\n--xxBOUNDARYxx\r\nContent-Type: application/octet-stream\r\nContent-Disposition: form-data; name=\"file\"; filename=\"%s\"\r\n\r\n",modemCom::status.MAC,nomeArquivo); 00288 00289 dataTam = strlen(header); 00290 dataTam+=arquivo->bytes; 00291 dataTam+= strlen("\r\n--xxBOUNDARYxx--"); 00292 //"/drome/parser/index.php" uri drome 00293 sprintf(header,"POST %s HTTP/1.1\r\nHost: %s\r\nContent-Type: multipart/form-data; boundary=xxBOUNDARYxx\r\nContent-Length: %lu\r\n\r\n",uri,host,dataTam); 00294 headerTam = strlen(header); //Pego o tamanho parcial para fazer a concatenaçao a fim de predizer o tamanho do post total 00295 00296 sprintf(&header[headerTam],"--xxBOUNDARYxx\r\nContent-Type: text/plain\r\nContent-Disposition: form-data; name=\"IMEI\"\r\n\r\n%s\r\n--xxBOUNDARYxx\r\nContent-Type: application/octet-stream\r\nContent-Disposition: form-data; name=\"file\"; filename=\"%s\"\r\n\r\n",modemCom::status.MAC,nomeArquivo); 00297 headerTam = strlen(header); 00298 00299 sprintf(aux,"AT+CIPSEND=1,%u\r\n",headerTam); 00300 if(modemCom::sendToModem(aux,1,&modemCom::status.PROMPT_ENVIO_COMMAND_MODE,NULL,1000,1,1)) { 00301 if(!modemCom::sendToModem(header,1,&modemCom::status.SEND_OK,NULL,1000,1,1)) { 00302 sdCard::fechaArquivo(arquivo); 00303 modemCom::status.emComunicacao = false; 00304 pc.printf("Cabecalho http nao enviado.\n"); 00305 return 0; 00306 } else { 00307 pc.printf("Cabecalho http enviado.\n"); 00308 } 00309 } else { 00310 pc.printf("Erro enviando arquivo.\n"); 00311 sdCard::fechaArquivo(arquivo); 00312 modemCom::status.emComunicacao = false; 00313 return 0; 00314 } 00315 00316 //Enviando as partes inteiras 00317 maxTentativas = maxRetentativasEnvioParteArquivo; 00318 for(parteSendoEnviada=0; parteSendoEnviada<numeroDePartes; parteSendoEnviada++) { 00319 //Aqui realizo o envio de cada parte. 00320 passaParaProximaParte = false; 00321 if((parteSendoEnviada%10)==0){ 00322 //Kick no watchdog 00323 if(parteEnviadaAntes!=parteSendoEnviada){ 00324 diversos::wdt.kick(90.0); 00325 parteEnviadaAntes=parteSendoEnviada; 00326 if(debug){pc.printf("Watchdog KICK!.");} 00327 } 00328 } 00329 while((maxTentativas)&&(!passaParaProximaParte)) { 00330 sprintf(aux,"AT+CIPSEND=1,%u\r\n",numeroDeBytesPorEnvio); 00331 if(modemCom::sendToModem(aux,1,&modemCom::status.PROMPT_ENVIO_COMMAND_MODE,NULL,1000,1,1)) { 00332 if(maxTentativas!=maxRetentativasEnvioParteArquivo) { 00333 //Estou realizando reenvio desta parte logo preciso fazer fseek para a quantidade de bytes do envio anterior 00334 fseek(arquivo->fp,-numeroDeBytesPorEnvio,SEEK_CUR); 00335 } 00336 if(debug){pc.printf("Numero de bytes por envio = %lu.\r\n",numeroDeBytesPorEnvio);} 00337 for(numeroByteSendoEnviado=0; numeroByteSendoEnviado<(numeroDeBytesPorEnvio); numeroByteSendoEnviado++) { 00338 c = fgetc(arquivo->fp); 00339 modem.printf("%c",c); 00340 if(debug){ 00341 pc.printf("%c",c); 00342 } 00343 } 00344 00345 if(modemCom::sendToModem(NULL,1,&modemCom::status.SEND_OK,NULL,5000,1,1)) { 00346 diversos::progressBar(parteSendoEnviada,numeroDePartes); 00347 //Preciso verificar se o buffer esvaziou. Se deu "flush" 00348 //if(modemCom::aguardaFlush(0)==1) { 00349 //passa pra proxima parte 00350 passaParaProximaParte = true; 00351 maxTentativas = maxRetentativasEnvioParteArquivo; 00352 } else { 00353 maxTentativas--; 00354 if(maxTentativas == 1) { 00355 if(debug){pc.printf("MaxTentativas dentro de envio das partes == 1, vou aguardar o flush.\n");} 00356 } 00357 if(maxTentativas == 0) { 00358 sdCard::fechaArquivo(arquivo); 00359 modemCom::status.emComunicacao = false; 00360 return 0; 00361 } 00362 } 00363 } else { 00364 pc.printf("Erro enviando o arquivo nao recebeu a tentativa de envio de parte inteira.\n"); 00365 sdCard::fechaArquivo(arquivo); 00366 modemCom::status.emComunicacao = false; 00367 return 0; 00368 } 00369 } 00370 if(!passaParaProximaParte) { 00371 pc.printf("Erro enviando o arquivo nao recebeu a tentativa de envio de parte inteira.\n"); 00372 sdCard::fechaArquivo(arquivo); 00373 modemCom::status.emComunicacao = false; 00374 return 0; 00375 } 00376 } 00377 00378 //Enviando o resto 00379 passaParaProximaParte = false; 00380 maxTentativas = maxRetentativasEnvioParteArquivo; 00381 while((maxTentativas)&&(!passaParaProximaParte)) { 00382 sprintf(aux,"AT+CIPSEND=1,%u\r\n",restoDoEnvio+strlen("\r\n--xxBOUNDARYxx--\r\n\r\n")); //Para incluir "\n--xxBOUNDARYxx--\n\n" no fim da msg http 00383 if(modemCom::sendToModem(aux,1,&modemCom::status.PROMPT_ENVIO_COMMAND_MODE,NULL,1000,1,1)) { 00384 for(numeroByteSendoEnviado=0; numeroByteSendoEnviado<restoDoEnvio; numeroByteSendoEnviado++) { 00385 //Envio os bytes aqui! Menos o ultimo para ter o check; 00386 c = fgetc(arquivo->fp); 00387 modem.printf("%c",c); 00388 if(debug){ 00389 pc.printf("%c",c); 00390 } 00391 } 00392 if(modemCom::sendToModem("\r\n--xxBOUNDARYxx--\r\n\r\n",1,&modemCom::status.SEND_OK,NULL,5000,1,1)) { 00393 if(numeroDePartes) { 00394 diversos::progressBar(parteSendoEnviada,numeroDePartes); 00395 } 00396 passaParaProximaParte = true; 00397 if(debug){ 00398 pc.printf("\nEnviado o resto do envio com fim de cabecalho!\n",parteSendoEnviada); 00399 } 00400 modemCom::timeOutModem = 250; 00401 //} 00402 /*else { 00403 pc.printf("Erro enviando arquivo.\n"); 00404 fclose(fp); 00405 *aberto = false; 00406 return 0; 00407 }*/ 00408 } else { 00409 sdCard::fechaArquivo(arquivo); 00410 modemCom::status.emComunicacao = false; 00411 return 0; 00412 } 00413 } else { 00414 maxTentativas--; 00415 modemCom::sendToModem("AT\r\n",1,&modemCom::status.OK,NULL,1000,1,1); 00416 } 00417 } 00418 00419 if(!passaParaProximaParte) { 00420 pc.printf("Erro enviando o arquivo nao recebeu a tentativa de envio de parte inteira.\n"); 00421 sdCard::fechaArquivo(arquivo); 00422 modemCom::status.emComunicacao = false; 00423 return 0; 00424 } 00425 00426 sdCard::fechaArquivo(arquivo); 00427 00428 //Logica de timeout esperando confirmação modemCom::status.ServerAck 20 segundos 00429 parteSendoEnviada = 300; //Reaproveitando variável 00430 while((parteSendoEnviada)&&(!modemCom::status.ServerAck)) { 00431 osDelay(100); 00432 parteSendoEnviada--; 00433 } 00434 00435 modemCom::status.serverConnected=0; 00436 //modemCom::sendToModem("AT+CIPCLOSE=1\r\n",1,&modemCom::status.OK,500,3); 00437 modemCom::closeConnection(&modemCom::status.connIDSendData); 00438 modemCom::status.emComunicacao = false; 00439 00440 return modemCom::status.ServerAck; 00441 } 00442 00443 uint8_t modemCom::sendToModem(char *string,uint8_t confirmado,char *codigoConfirmacao,char *stringConfirmacao,uint16_t timeOut,uint8_t maxTentativas,uint16_t delayEntreTentativas) 00444 { 00445 uint16_t timeOutProcesso; 00446 uint16_t indexString,strLen; //Para exibir retentativas! 00447 timeOut/=5; 00448 while(maxTentativas){ 00449 timeOutProcesso=timeOut; 00450 00451 00452 bufModem.del(); //Deletando o buffer antes de iniciar envio 00453 00454 if(string!=NULL) { 00455 modem.puts(string); 00456 if(xeretaModem){ 00457 pc.printf("Sai pro modem <%s>\n",string); //Enviando na serial PC para debug 00458 } 00459 } 00460 if(confirmado==1){ 00461 modemCom::status.ERROR=0; 00462 modemCom::status.busy=0; 00463 00464 if(codigoConfirmacao!=NULL){ 00465 *codigoConfirmacao=0; 00466 while((timeOutProcesso)&&(!(*codigoConfirmacao))&&(!modemCom::status.ERROR)&&(!modemCom::status.busy)){ 00467 osDelay(5); //timeOut em mS 00468 timeOutProcesso--; 00469 } 00470 }else{ 00471 while((timeOutProcesso)&&(!modemCom::status.ERROR)&&(!modemCom::status.busy)&&(!strstr(bufModem.getRowBuffer(),stringConfirmacao))) { 00472 osDelay(5); //timeOut em mS 00473 timeOutProcesso--; 00474 } 00475 } 00476 if(modemCom::status.ERROR||modemCom::status.busy) { 00477 osDelay(1000);//espera 1S 00478 } 00479 00480 if(modemCom::status.dnsError){ 00481 modemCom::status.dnsError=false; 00482 return 0; 00483 } 00484 00485 if(timeOutProcesso) { 00486 if(confirmado==1){ 00487 if(!(*codigoConfirmacao)){return 0;} 00488 }else{ 00489 if(!strstr(bufModem.getRowBuffer(),stringConfirmacao)){return 0;} 00490 } 00491 return maxTentativas; 00492 } 00493 00494 strLen = strlen(string); 00495 for(indexString=0; indexString<(strLen-1); indexString++) { 00496 pc.putc(string[indexString]); 00497 } 00498 00499 if(xeretaModem){ 00500 pc.printf("> maxTentativas = %lu.\n",maxTentativas); 00501 } 00502 00503 }else{return 1;} 00504 maxTentativas--; 00505 osDelay(delayEntreTentativas); 00506 } 00507 return 0; 00508 } 00509 00510 char modemCom::closeConnection(uint8_t *id){ 00511 char command[20]; 00512 if(debug){pc.printf("Lido %u como sendo o id em closeConnection.\r\n",*id);} 00513 if((*id)==255){ 00514 return 2; 00515 } 00516 sprintf(command,"AT+CIPCLOSE=%u\r\n",*id); 00517 if(modemCom::sendToModem(command,1,NULL,",CLOSED",1000,1,300)){ 00518 *id = 255; 00519 return 1; 00520 } 00521 return 0; 00522 } 00523 00524 char modemCom::cipSend(uint8_t idConnection,char *buffer,uint16_t len){ 00525 char aux[20]; 00526 uint16_t i; 00527 if(!len){ 00528 len = strlen(buffer); 00529 } 00530 if(debug){printf("conteudo de cipsend.<");} 00531 sprintf(aux,"AT+CIPSENDEX=%u,%lu\r\n",idConnection,len); 00532 if(sendToModem(aux,1,&modemCom::status.OK,NULL,2000,1,1)){ 00533 for(i=0;i<len;i++){ 00534 modem.putc(buffer[i]); 00535 if(debug){pc.putc(buffer[i]);} 00536 } 00537 }else{ 00538 return 0; 00539 } 00540 if(debug){printf(">\r\n");} 00541 if(sendToModem("",1,&modemCom::status.SEND_OK,NULL,2000,1,1)){ 00542 return 1; 00543 } 00544 return 2; 00545 } 00546 00547 void modemCom::webServer(uint8_t id){ 00548 //char aux[50]; 00549 bool encontrado=0; 00550 char buf[1024]; 00551 char *strOutput; 00552 //uint32_t aux_int; 00553 00554 if(strstr(webServerBuff,"favicon.ico")){ 00555 //sprintf(buf,"<!DOCTYPE html><html><body><h1>DROME-Seu processo na palma da sua mao!</h1><img src='http://criandoriquezaimagens.s3.amazonaws.com/wp-content/uploads/2014/12/casaPropria.png' width='200' height='210'><br><p><a href='/lecomandocontrole.htm'>Ler comando do controle.</a></p><br></body></html>"); 00556 sprintf(buf,"HTTP/1.1 200 OK\r\nContent-Type: text/html\r\n\r\n<!DOCTYPE html><html><body><link rel=\"icon\" href=\"http://%s/drome/assets/local/images/favicon/favicon.ico\" type=\"image/x-icon\"></body></html>",modemCom::status.host); 00557 if(cipSend(id,buf,0)==1){ 00558 //printf("Requisicao enviada!\n"); 00559 }else{ 00560 printf("Requisicao nao enviada 1!!\n"); 00561 } 00562 encontrado = 1; 00563 } 00564 00565 if(strstr(webServerBuff,"comandos.htm")&&(!encontrado)){ 00566 00567 //sprintf(buf,"<!DOCTYPE html><html><body><h1>DROME-Seu processo na palma da sua mao!</h1><img src='http://criandoriquezaimagens.s3.amazonaws.com/wp-content/uploads/2014/12/casaPropria.png' width='200' height='210'><br><p><a href='/lecomandocontrole.htm'>Ler comando do controle.</a></p><br></body></html>"); 00568 sprintf(buf,"HTTP/1.1 200 OK\r\nContent-Type: text/html\r\n\r\n<!DOCTYPE html><html><head><title>ConfigRede DROME</title></head><body><h1>DROME-Dispositivo Remoto de Operacao e Monitoramento de Equipamentos</h1><br><p><a href='/lecomandocontrole.htm'>Ler comando do controle.</a><a href='/configRede.htm'><br>Configurar rede.</a></p><br></body></html>"); 00569 if(cipSend(id,buf,0)==1){ 00570 printf("Requisicao enviada!\n"); 00571 }else{ 00572 //printf("Requisicao nao enviada 1!!\n"); 00573 } 00574 encontrado = 1; 00575 } 00576 00577 if(strstr(webServerBuff,"configRede.htm")&&(!encontrado)){ 00578 //Pegando o IP 00579 modemCom::leIP(); 00580 sprintf(buf,"HTTP/1.1 200 OK\r\nContent-Type: text/html\r\n\r\n<!DOCTYPE html><html><head><title>ConfigRede DROME</title></head><body><form action='cwjap.htm' method='GET'><fieldset><legend>Parametros de Configuracao da rede:</legend>SSID WiFi:<br><input type='text' name='ssid' value='%s'><br>Senha WiFi:<br><input type='text' name='password' value='%s'><br>Porta:<br><input type='text' name='porta' value='%s'><br>ServerIP:<br><input type='text' name='serverIP' value='%s'><br>Host:<br><input type='text' name='host' value='%s'><br>Periodo<br><input type='text' name='periodo' value='%lu'><br>IP<br><input type='text' name='STAIP' value='%s'><br>Mascara de Sub Rede<br><input type='text' name='subnetmask' value='%s'><br>Gateway/dhcp<br><input type='text' name='gateway' value='%s'><br>------ModBus------<br>BaudRate<br><input type='text' name='baudrate' value='%lu'><br>TimeOut<br><input type='text' name='timeout' value='%lu'><br><input type='submit' value='Configurar'></fieldset></form></body>",modemCom::status.ssid,modemCom::status.password,modemCom::status.port,modemCom::status.serverIP,modemCom::status.host,modemCom::status.periodo,modemCom::status.STAIP,modemCom::status.subnetmask,modemCom::status.gateway,modBusMaster1::MODBUS_SERIAL_BAUD,modBusMaster1::MODBUS_TIMEOUT); 00581 cipSend(id,buf,0); 00582 encontrado = 1; 00583 } 00584 00585 if(strstr(webServerBuff,"lecomandocontrole.htm")&&(!encontrado)){ 00586 uint16_t timeout = 5000; 00587 00588 IrDetect.reset(); 00589 IrDetect.start(); 00590 00591 diversos::wdt.kick(90.0); 00592 ledUsoGeral = true; 00593 while((IrDetect.read_ms()<timeout) && (!detectaIRIn())); 00594 if(IrDetect.read_ms()<timeout){ 00595 serializaPacoteIR(modemCom::status.connIDWebServer); 00596 }else{ 00597 sprintf(buf,"HTTP/1.1 200 OK\r\nContent-Type: text\r\n\r\nerro"); 00598 if(cipSend(id,buf,0)==1){ 00599 //printf("Requisicao enviada!\n"); 00600 } 00601 } 00602 IrDetect.stop(); 00603 ledUsoGeral = false; 00604 encontrado = 1; 00605 } 00606 00607 if(strstr(webServerBuff,"wdtreset.htm")&&(!encontrado)){ 00608 sprintf(buf,"HTTP/1.1 200 OK\n\n ACK"); 00609 cipSend(id,buf,0); 00610 if(debug){pc.printf("Fechando socket chave 4.\r\n");} 00611 modemCom::closeConnection(&modemCom::status.connIDWebServer); 00612 diversos::wdt.kick(0.1); 00613 while(true); 00614 } 00615 00616 if(strstr(webServerBuff,"reset.htm")&&(!encontrado)){ 00617 sprintf(buf,"HTTP/1.1 200 OK\n\n ACK"); 00618 cipSend(id,buf,0); 00619 if(debug){pc.printf("Fechando socket chave 5.\r\n");} 00620 modemCom::closeConnection(&modemCom::status.connIDWebServer); 00621 NVIC_SystemReset(); 00622 } 00623 00624 if(strstr(webServerBuff,"cwjap.htm")&&(!encontrado)){ 00625 pc.printf("Lido de cwjap <%s>.\r\n\r\n",webServerBuff); 00626 00627 strtok(webServerBuff,"="); 00628 strcpy(modemCom::status.ssid,strtok(NULL,"&")); //pegando ssid 00629 00630 strtok(NULL,"="); 00631 strcpy(modemCom::status.password,strtok(NULL,"&")); //pegando password 00632 00633 strtok(NULL,"="); 00634 strcpy(modemCom::status.port,strtok(NULL,"&")); //pegando port 00635 00636 strtok(NULL,"="); 00637 strcpy(modemCom::status.serverIP,strtok(NULL,"&")); //pegando serverIP 00638 00639 strtok(NULL,"="); 00640 strcpy(modemCom::status.host,strtok(NULL,"&")); //pegando host 00641 00642 strtok(NULL,"="); 00643 strcpy(buf,strtok(NULL,"&")); //pegando periodo 00644 modemCom::status.periodo = atoi(buf); 00645 modemCom::status.periodoConfiguracao = modemCom::status.periodo; 00646 00647 //*************************IP ou DHCP**************************** 00648 00649 strtok(NULL,"="); 00650 strcpy(modemCom::status.STAIP,strtok(NULL,"&")); //pegando IP/DHCP 00651 00652 strtok(NULL,"="); 00653 strcpy(modemCom::status.subnetmask,strtok(NULL,"&")); //pegando subnetmask 00654 00655 strtok(NULL,"="); 00656 strcpy(modemCom::status.gateway,strtok(NULL,"&")); //pegando gateway 00657 00658 //************************* ModBus **************************** 00659 00660 strtok(NULL,"="); 00661 strcpy(buf,strtok(NULL,"&")); //pegando BaudRate 00662 modBusMaster1::MODBUS_SERIAL_BAUD = atoi(buf); 00663 00664 00665 strtok(NULL,"="); 00666 strcpy(buf,strtok(NULL," ")); //pegando timeout 00667 modBusMaster1::MODBUS_TIMEOUT = atoi(buf); 00668 00669 00670 //Decodificando URL 00671 strOutput = (char *) malloc(strlen(modemCom::status.ssid)+1); 00672 diversos::urldecode2(strOutput, modemCom::status.ssid); 00673 strcpy(modemCom::status.ssid,strOutput); 00674 free(strOutput); 00675 00676 strOutput = (char *) malloc(strlen(modemCom::status.password)+1); 00677 diversos::urldecode2(strOutput, modemCom::status.password); 00678 strcpy(modemCom::status.password,strOutput); 00679 free(strOutput); 00680 00681 00682 if((modemCom::status.ssid[0]!=0) && (modemCom::status.password[0]!=0)){ 00683 sprintf(buf,"HTTP/1.1 200 OK\n\nACK"); 00684 mudaRede = true; 00685 }else{ 00686 sprintf(buf,"HTTP/1.1 200 OK\n\nNACK"); 00687 } 00688 cipSend(id,buf,0); 00689 encontrado = 1; 00690 } 00691 00692 if(!encontrado){ 00693 sprintf(buf,"Pagina Nao Encontrada."); 00694 if(cipSend(id,buf,0)==1){ 00695 //printf("Requisicao enviada!\n"); 00696 }else{ 00697 printf("Requisicao nao enviada 3!!\n"); 00698 } 00699 } 00700 if(debug){pc.printf("Fechando socket chave 3.\r\n");} 00701 modemCom::closeConnection(&modemCom::status.connIDWebServer); 00702 if(mudaRede){ 00703 mudaRede = false; 00704 modemCom::sendToModem("AT+CIPSERVER=0\r\n",1,&modemCom::status.OK,NULL,2000,1,1); 00705 pc.printf("Mudando para rede:\r\nSSID:%s\r\nPASSWORD:%S\r\nPORTA:%s\r\nserverIP:%s\r\nHOST:%s\r\nPERIODO:%lu\r\nIP/DHCP:%s\r\nsubnetmask:%s\r\ngateway:%s\r\nBaudRate:%lu\r\nTimeOut:%lu\r\n\r\n",modemCom::status.ssid,modemCom::status.password,modemCom::status.port,modemCom::status.serverIP,modemCom::status.host,modemCom::status.periodo,modemCom::status.STAIP,modemCom::status.subnetmask,modemCom::status.gateway,modBusMaster1::MODBUS_SERIAL_BAUD,modBusMaster1::MODBUS_TIMEOUT); 00706 00707 if(strstr(modemCom::status.gateway,"dhcp")){ 00708 strcpy(modemCom::status.STAIP,"dhcp"); 00709 strcpy(modemCom::status.subnetmask,"dhcp"); 00710 } 00711 00712 if(sdCard::abreArquivo(&sdCard::config,"w")){ 00713 pc.printf("Guardando config.\r\n"); 00714 fprintf(sdCard::config.fp,"SSID:%s\r\nPASSWORD:%s\r\nPORT:%s\r\nserverIP:%s\r\nHOST:%s\r\nPERIODO:%lu\r\nSTAIP:%s\r\nsubnetmask:%s\r\ngateway:%s\r\nModBus_BaudRate:%lu\r\nModBus_TimeOut:%lu\r\n",modemCom::status.ssid,modemCom::status.password,modemCom::status.port,modemCom::status.serverIP,modemCom::status.host,modemCom::status.periodo,modemCom::status.STAIP,modemCom::status.subnetmask,modemCom::status.gateway,modBusMaster1::MODBUS_SERIAL_BAUD,modBusMaster1::MODBUS_TIMEOUT); 00715 sdCard::fechaArquivo(&sdCard::config); 00716 modemCom::inicializaModem(); 00717 modemCom::conectaWiFi(); 00718 } 00719 } 00720 } 00721 00722 bool modemCom::leIP(void){ 00723 char *ptr; 00724 sendToModem("AT+CIFSR\r\n",1,NULL,"+CIFSR:STAIP,",2000,1,1); 00725 pc.printf("Lido de leIP <%s>.\r\n",modemCom::bufIn); 00726 ptr=strstr(modemCom::bufIn,"+CIFSR:STAIP,"); 00727 if(ptr) { 00728 ptr = strtok(ptr,"\""); 00729 ptr = strtok(NULL,"\""); 00730 strcpy(modemCom::status.STAIP,ptr); 00731 } 00732 if(strstr(modemCom::status.STAIP,"0.0.0.0")){return false;} 00733 return true; 00734 } 00735 00736 int16_t modemCom::getRSSI(void){ 00737 char *ptr; 00738 int16_t RSSI = 255; 00739 00740 if(modemCom::sendToModem("AT+CWJAP?\r\n",1,&modemCom::status.OK,NULL,10000,1,1000)){ 00741 ptr = strstr(modemCom::bufIn,(const char*)modemCom::status.ssid); 00742 if(ptr){ 00743 ptr = strtok(ptr,","); //Descarta SSID 00744 ptr = strtok(NULL,","); //Descarta MAC 00745 ptr = strtok(NULL,","); //Descarta Canal 00746 ptr = strtok(NULL,","); //Captura RSSI 00747 RSSI = atoi(ptr); 00748 } 00749 } 00750 return RSSI; 00751 //return -50; 00752 } 00753 00754 bool modemCom::verificaConexao(void){ 00755 uint8_t i = 1; 00756 while(i){ 00757 if(modemCom::getRSSI()==255){ 00758 if(modemCom::conectaWiFi()){ 00759 return true; 00760 } 00761 i--; 00762 }else{ 00763 return true; 00764 } 00765 } 00766 return false; 00767 } 00768 00769 bool modemCom::conectaWiFi(void){ 00770 //Função para mudança de rede 00771 00772 char aux[128]; 00773 //modemCom::leParametrosConexaoSDCard(); 00774 //AT+CWJAP=\"\",\"\"\r\n 00775 sprintf(aux,"AT+CWJAP=\"%s\",\"%s\"\r\n",modemCom::status.ssid,modemCom::status.password); 00776 if(modemCom::sendToModem(aux,1,&modemCom::status.wifi_connected,NULL,7000,2,1000)) { 00777 if(debug){pc.printf("WiFi Conectado.\n");} 00778 return true; 00779 }else{ 00780 return false; 00781 } 00782 } 00783 00784 void modemCom::processaPacote(void const *args){ 00785 char *ptr,*scanPtr; 00786 uint16_t bufInLength = bufModem.getLength(); 00787 uint16_t timeOut; 00788 //bool getFound=false; 00789 modemCom::bufIn = bufModem.get(); 00790 00791 modemCom::status.modemResponse=true; 00792 00793 if(xeretaModem){pc.printf("Vem do modem <%s>.\r\n",modemCom::bufIn);} 00794 00795 //Reconhecimento dos status 00796 modemCom::status.PROMPT_ENVIO_COMMAND_MODE=0; 00797 ptr=strstr(modemCom::bufIn,"> "); 00798 if(ptr) { 00799 00800 modemCom::status.serverConnected=1; 00801 modemCom::status.commandMode=1; 00802 00803 modemCom::status.PROMPT_ENVIO_COMMAND_MODE=1; 00804 } 00805 00806 modemCom::status.busy=false; 00807 ptr=strstr(modemCom::bufIn,"busy p..."); 00808 if(ptr) { 00809 modemCom::status.OK=0; 00810 modemCom::status.ERROR=1; 00811 modemCom::status.busy=1; 00812 } 00813 00814 /*modemCom::status.busy=false; 00815 ptr=strstr(modemCom::bufIn,"busy s..."); 00816 if(ptr) { 00817 modemCom::status.OK=0; 00818 //modemCom::status.ERROR=1; 00819 modemCom::status.busy=1; 00820 }*/ 00821 00822 modemCom::status.SEND_OK=0; 00823 ptr=strstr(modemCom::bufIn,"SEND OK"); 00824 if(ptr) { 00825 00826 modemCom::status.SEND_OK=1; 00827 } 00828 00829 modemCom::status.ERROR=0; 00830 ptr=strstr(modemCom::bufIn,"ERROR"); 00831 if(ptr) { 00832 00833 modemCom::status.ERROR=1; 00834 modemCom::status.OK=0; 00835 } 00836 00837 ptr=strstr(modemCom::bufIn,"FAIL"); 00838 if(ptr) { 00839 00840 modemCom::status.ERROR=1; 00841 modemCom::status.OK=0; 00842 } 00843 00844 00845 00846 ptr=strstr(modemCom::bufIn,"DNS Fail"); 00847 if(ptr) { 00848 modemCom::status.dnsError=1; 00849 modemCom::status.ERROR=1; 00850 modemCom::status.OK=0; 00851 } 00852 00853 ptr=strstr(modemCom::bufIn,"WIFI CONNECTED"); 00854 if(ptr) { 00855 modemCom::status.wifi_connected=1; 00856 00857 } 00858 00859 ptr=strstr(modemCom::bufIn,"ALREADY CONNECTED"); 00860 if(ptr) { 00861 modemCom::status.ALREADY_CONNECTED=1; 00862 modemCom::status.ERROR=1; 00863 modemCom::status.OK=0; 00864 } 00865 00866 modemCom::status.OK=0; 00867 ptr=strstr(modemCom::bufIn,"OK\r"); 00868 if(ptr) { 00869 modemCom::status.OK=1; 00870 modemCom::status.ERROR=0; 00871 modemCom::status.busy=0; 00872 } 00873 00874 //modemCom::status.CLOSED=false; 00875 ptr=strstr(modemCom::bufIn,"CLOSED"); 00876 if(ptr) { 00877 modemCom::status.CLOSED=1; 00878 } 00879 00880 /*if(strstr(modemCom::bufIn,"GET /")){ 00881 getFound = true; 00882 }*/ 00883 00884 //Buscando connect 00885 /*if(!modemCom::atendendoWebServer){ 00886 ptr=strstr(modemCom::bufIn,"CONNECT\r\n"); 00887 if(ptr) { 00888 ptr = strstr(ptr,"+IPD"); 00889 ptr = strtok(ptr,","); 00890 ptr = strtok(NULL,","); 00891 connID = atoi(ptr); 00892 modemCom::status.connIDServerCommand = connID; 00893 modemCom::status.serverConnected=1; 00894 pc.printf("Recebido string CONNECT socket na conexao %u.\n",connID); 00895 if(!modemCom::status.emComunicacao){ 00896 if(!getFound){ 00897 pc.printf("Atendendo socket entrante na conexao %u.\n",connID); 00898 modemCom::status.SRINGsockEntrante = true; 00899 } 00900 } 00901 modemCom::status.emComunicacao=true; 00902 modemCom::bufIn = strtok(NULL,""); 00903 } 00904 }*/ 00905 00906 IPDNumBytes = 0; 00907 ptr = strstr(modemCom::bufIn,"+IPD"); 00908 if(ptr!=NULL){ 00909 //+IPD,0,480:GET 00910 strtok(ptr,","); //Separando +IPD 00911 00912 ptr = strtok(NULL,","); //Pegando o numero da conexão 00913 connID = atoi(ptr); 00914 00915 ptr = strtok(NULL,":"); //Pegando o numero de caracteres IPD 00916 IPDNumBytes = atoi(ptr); 00917 00918 modemCom::bufIn = strtok(NULL,""); 00919 00920 //printf("Recebido pacote via conexao %u com %lu bytes.\n",connID,IPDNumBytes); 00921 00922 } 00923 00924 if((connID==modemCom::status.connIDServerCommand)&& IPDNumBytes && modemCom::status.recebendoArquivoDoServer && (strstr(modemCom::bufIn,"CONNECT")==NULL) && (strstr(modemCom::bufIn,"*ServerCommand*")==NULL) ){ 00925 pc.printf("sendData recebidos %lu bytes\n",sdCard::nBytesArquivoRecebidos/2); 00926 sdCard::insereDadosArquivoHex(&sdCard::tempFile,modemCom::bufIn,IPDNumBytes); 00927 //sdCard::insereDadosArquivo(&sdCard::tempFile,modemCom::bufIn,IPDNumBytes); 00928 sdCard::nBytesArquivoRecebidos+=IPDNumBytes; 00929 bufModem.del(); 00930 sprintf(modemCom::bufIn,"AT+CIPSEND=%u,%lu\r\n",modemCom::status.connIDServerCommand,10); 00931 modem.puts(modemCom::bufIn); 00932 timeOut = 500; 00933 while(timeOut && (strstr(bufModem.getRowBuffer(),">")==NULL)){ 00934 osDelay(10); 00935 timeOut--; 00936 } 00937 modemCom::status.timeOut = 30; 00938 00939 modem.puts("sendData\r\n"); 00940 diversos::wdt.kick(); 00941 modemCom::status.recebendoArquivoDoServer = 250; 00942 return; 00943 } 00944 00945 //Reconhecendo GET HTTP e atendendo com um servidor web 00946 if(strstr(modemCom::bufIn,"HTTP/1.1")){ 00947 ptr=strstr(modemCom::bufIn,"GET /"); 00948 if(ptr) { 00949 ptr = strtok(ptr,"/"); 00950 ptr = strtok(NULL," "); 00951 bufInPtr = ptr; 00952 strcpy(webServerBuff,ptr); 00953 modemCom::atendendoWebServer = true; 00954 modemCom::status.connIDWebServer = connID; 00955 } 00956 } 00957 00958 ptr=strstr(modemCom::bufIn,"*ServerAck*"); 00959 if(ptr) { 00960 modemCom::status.ServerAck=1; 00961 } 00962 00963 ptr=strstr(modemCom::bufIn,"*ServerCommand*"); 00964 if(ptr) { 00965 scanPtr = strtok (ptr,"\\"); 00966 scanPtr = strtok (NULL,">"); 00967 strcpy(commands::buffer,scanPtr); 00968 if(debug){pc.printf("Comandos <%s>.\n",commands::buffer);} 00969 modemCom::status.connIDServerCommand = connID; 00970 executaComandoServer = true; 00971 } 00972 } 00973 00974 bool modemCom::leParametrosConexaoSDCard(){ 00975 char *ptr; 00976 char aux[128]; 00977 if(sdCard::abreArquivo(&sdCard::config,"r")){ 00978 //pc.printf("Abrindo arquivo de config para buscar dados de conexao.\r\n"); 00979 //"SSID:DROME\r\nPASSWORD:VITAE\r\nPORT:80" 00980 00981 //Pegando SSID 00982 fgets(modemCom::status.ssid,40,sdCard::config.fp); 00983 ptr = strstr(modemCom::status.ssid,"SSID"); 00984 if(ptr!=NULL){ 00985 ptr = strtok(modemCom::status.ssid,":"); 00986 ptr = strtok(NULL,"\r\n"); 00987 strcpy(modemCom::status.ssid,ptr); 00988 00989 //Pegando PASSWORD 00990 fgets(modemCom::status.password,50,sdCard::config.fp); 00991 ptr = strtok(modemCom::status.password,":"); 00992 ptr = strtok(NULL,"\r\n"); 00993 strcpy(modemCom::status.password,ptr); 00994 00995 //Pegando PORT 00996 fgets(modemCom::status.port,20,sdCard::config.fp); 00997 ptr = strtok(modemCom::status.port,":"); 00998 ptr = strtok(NULL,"\r\n"); 00999 strcpy(modemCom::status.port,ptr); 01000 01001 //Pegando serverIP 01002 fgets(aux,50,sdCard::config.fp); 01003 ptr = strtok(aux,":"); 01004 ptr = strtok(NULL,"\r\n"); 01005 strcpy(modemCom::status.serverIP,ptr); 01006 01007 //Pegando host 01008 fgets(aux,50,sdCard::config.fp); 01009 ptr = strtok(aux,":"); 01010 ptr = strtok(NULL,"\r\n"); 01011 strcpy(modemCom::status.host,ptr); 01012 01013 //Pegando periodo 01014 fgets(aux,20,sdCard::config.fp); 01015 ptr = strtok(aux,":"); 01016 ptr = strtok(NULL,"\r\n"); 01017 modemCom::status.periodo = atoi(ptr); 01018 modemCom::status.periodoConfiguracao = modemCom::status.periodo; 01019 01020 //*************IP ou DHCP***************** 01021 01022 //Pegando STAIP 01023 fgets(aux,50,sdCard::config.fp); 01024 ptr = strtok(aux,":"); 01025 ptr = strtok(NULL,"\r\n"); 01026 strcpy(modemCom::status.STAIP,ptr); 01027 01028 //Pegando subnetmask 01029 fgets(aux,50,sdCard::config.fp); 01030 ptr = strtok(aux,":"); 01031 ptr = strtok(NULL,"\r\n"); 01032 strcpy(modemCom::status.subnetmask,ptr); 01033 01034 //Pegando gateway 01035 fgets(aux,50,sdCard::config.fp); 01036 ptr = strtok(aux,":"); 01037 ptr = strtok(NULL,"\r\n"); 01038 strcpy(modemCom::status.gateway,ptr); 01039 01040 //------ModBus_BaudRate------ 01041 fgets(aux,50,sdCard::config.fp); 01042 ptr = strtok(aux,":"); 01043 ptr = strtok(NULL,"\r\n"); 01044 modBusMaster1::MODBUS_SERIAL_BAUD = atoi(ptr); 01045 modBusMaster1::setBaud(modBusMaster1::MODBUS_SERIAL_BAUD); 01046 01047 //------ModBus_TimeOut------ 01048 fgets(aux,50,sdCard::config.fp); 01049 ptr = strtok(aux,":"); 01050 ptr = strtok(NULL,"\r\n"); 01051 modBusMaster1::MODBUS_TIMEOUT = atoi(ptr); 01052 }else{ 01053 strcpy(modemCom::status.ssid,"VSE"); 01054 strcpy(modemCom::status.password,"vitaeBBYP"); 01055 strcpy(modemCom::status.port,"4012"); 01056 strcpy(modemCom::status.serverIP,"191.252.119.127"); 01057 strcpy(modemCom::status.host,"www.drome.com.br"); 01058 strcpy(modemCom::status.gateway,"dhcp"); 01059 modemCom::status.periodo = 900; 01060 modBusMaster1::MODBUS_SERIAL_BAUD = 19200; 01061 modBusMaster1::setBaud(modBusMaster1::MODBUS_SERIAL_BAUD); 01062 modBusMaster1::MODBUS_TIMEOUT = 100; 01063 modemCom::status.periodoConfiguracao = modemCom::status.periodo; 01064 } 01065 sdCard::fechaArquivo(&sdCard::config); 01066 if(debug){pc.printf("Lidos os parametros de configuracao de wifi como sendo:\r\nSSID:<%s>\r\nPASSWORD:<%s>\r\nPORT:<%s>\r\nserverIP:<%s>\r\nHOST:<%s>\r\nPERIODO:<%lu>\r\nSTAIP:<%s>\r\nsubnetmask:<%s>\r\ngateway:<%s>\r\nbaudRateModbus:<%lu>\r\ntimeOutModbus:<%lu>\r\n",modemCom::status.ssid,modemCom::status.password,modemCom::status.port,modemCom::status.serverIP,modemCom::status.host,modemCom::status.periodo,modemCom::status.STAIP,modemCom::status.subnetmask,modemCom::status.gateway,modBusMaster1::MODBUS_SERIAL_BAUD,modBusMaster1::MODBUS_TIMEOUT); 01067 pc.printf("Iniciando conexao wifi.\r\n");} 01068 return true; 01069 }else{ 01070 strcpy(modemCom::status.ssid,"VSE"); 01071 strcpy(modemCom::status.password,"vitaeBBYP"); 01072 strcpy(modemCom::status.port,"4012"); 01073 strcpy(modemCom::status.serverIP,"191.252.119.127"); 01074 strcpy(modemCom::status.host,"www.drome.com.br"); 01075 strcpy(modemCom::status.gateway,"dhcp"); 01076 modemCom::status.periodo = 900; 01077 modBusMaster1::MODBUS_SERIAL_BAUD = 19200; 01078 modBusMaster1::setBaud(modBusMaster1::MODBUS_SERIAL_BAUD); 01079 modBusMaster1::MODBUS_TIMEOUT = 100; 01080 modemCom::status.periodoConfiguracao = modemCom::status.periodo; 01081 return false; 01082 } 01083 } 01084 01085 uint8_t modemCom::inicializaModem(void) 01086 { 01087 char aux[255]; 01088 modemCom::timeOutModem = 10; 01089 modemCom::status.emComunicacao = true; 01090 01091 serialModem::configBaud(); 01092 01093 modemCom::leMAC(); 01094 01095 modemCom::leParametrosConexaoSDCard(); 01096 01097 //Colocando aqui o conteudo de conectaWiFi 01098 //Mudando mode para AP e Station 01099 if(modemCom::sendToModem("AT+CWMODE=3\r\n",1,&modemCom::status.OK,NULL,3000,2,250)) { 01100 pc.printf("Modo AP+STATION aceito.\n"); 01101 } 01102 01103 01104 if(modemCom::sendToModem("AT+CIPMUX=1\r\n",1,&modemCom::status.OK,NULL,500,2,100)) { 01105 pc.printf("Recebeu o comando AT+CIPMUX=1.\n"); 01106 } 01107 01108 01109 //AT+CIPSERVER=1,portaLidaDoSDCard 01110 sprintf(aux,"AT+CIPSERVER=1,%s\r\n",modemCom::status.port); 01111 if(modemCom::sendToModem(aux,1,&modemCom::status.OK,NULL,400,2,100)) { 01112 pc.printf("Recebeu o comando <%s>.\n",aux); 01113 } 01114 01115 if(modemCom::sendToModem("AT+CIPSTO=10\r\n",1,&modemCom::status.OK,NULL,400,2,100)) { 01116 pc.printf("Recebeu o comando AT+CIPSTO=10.\n"); 01117 } 01118 01119 sprintf(aux,"AT+CWSAP_CUR=\"DROME_WIFI_%s\",\"VseDROME\",5,3\r\n",modemCom::status.MAC); 01120 if(modemCom::sendToModem(aux,1,&modemCom::status.OK,NULL,1000,2,300)) { 01121 pc.printf("Recebeu o comando %s.\n",aux); 01122 } 01123 01124 01125 if(modemCom::sendToModem("AT+CIPAP_CUR=\"192.168.4.1\",\"192.168.4.1\",\"255.255.255.0\"\r\n",1,&modemCom::status.OK,NULL,500,2,100)) { 01126 pc.printf("Recebeu o comando AT+CIPAP_CUR=\"192.168.4.1\",\"192.168.4.1\",\"255.255.255.0\".\n"); 01127 } 01128 01129 //Aqui decido se uso DHCP ou não. 01130 if(strstr(modemCom::status.gateway,"dhcp")||(strlen(modemCom::status.gateway)==0)){ 01131 //usando dhcp 01132 if(modemCom::sendToModem("AT+CWDHCP_CUR=2,1\r\n",1,&modemCom::status.OK,NULL,500,2,100)) { 01133 pc.printf("Recebeu o comando AT+CWDHCP_CUR=2,1.\n"); 01134 } 01135 }else{ 01136 if(modemCom::sendToModem("AT+CWDHCP_CUR=2,0\r\n",1,&modemCom::status.OK,NULL,500,2,100)) { 01137 pc.printf("Recebeu o comando AT+CWDHCP_CUR=2,0.\n"); 01138 } 01139 //AT+CIPSTA_DEF="192.168.6.100","192.168.6.1","255.255.255.0" 01140 sprintf(aux,"AT+CIPSTA_CUR=\"%s\",\"%s\",\"%s\"\r\n",modemCom::status.STAIP,modemCom::status.gateway,modemCom::status.subnetmask); 01141 if(modemCom::sendToModem(aux,1,&modemCom::status.OK,NULL,500,2,100)) { 01142 pc.printf("Recebeu o comando <%s>.\n",aux); 01143 } 01144 01145 } 01146 modemCom::status.emComunicacao = false; 01147 return 1; 01148 } 01149 01150
Generated on Wed Jul 13 2022 12:46:24 by
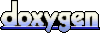