
teste de publish
Dependencies: DS1820 HighSpeedAnalogIn devices mbed
IRPack.cpp
00001 #include "IRPack.h" 00002 //bool dataIn[maxBytesMsg*8]; 00003 uint16_t bits[maxBitsMsg*4][2]; 00004 int indexIr=0,botao; 00005 int startBitAlto=0, startBitBaixo=0, bitBaixo[maxBitsMsg], bitAlto[maxBitsMsg], i; 00006 //unsigned char byte=0,msg[maxBytesMsg],msgBytes=0; 00007 00008 Timer IrTmr; 00009 Timer IrDetect; 00010 bool irInAntes=1; 00011 CircularBuffer bufIROut(maxBitsMsg*2*4,0); 00012 00013 //PwmOut IrOut(P1_18); 00014 DigitalIn IrIn(P1_24); //Não utilizado na placa 00015 00016 int round(float in){ 00017 int x = floor(in); 00018 float y = in-x; 00019 if(y>=0.5){ 00020 x++; 00021 } 00022 return x; 00023 } 00024 00025 uint16_t pulseDuration_us(bool high,DigitalIn pin,unsigned long int timeout){ 00026 unsigned long int durationBefore, duration; 00027 durationBefore = us_ticker_read(); 00028 do{ 00029 wait_us(1); 00030 timeout--; 00031 }while(timeout && (pin==high)); 00032 duration = us_ticker_read() - durationBefore; 00033 00034 //IrTmr.stop(); 00035 if(timeout){ 00036 return duration; 00037 } 00038 else{ 00039 return 65535; 00040 } 00041 } 00042 00043 00044 00045 void enviaComandoIR(uint8_t freq,uint8_t port){ 00046 float sd1Duty = SD1.read(); 00047 float sd2Duty = SD2.read(); 00048 float sd3Duty = SD3.read(); 00049 float sd4Duty = SD4.read(); 00050 float sd5Duty = SD5.read(); 00051 float sd6Duty = SD6.read(); 00052 00053 float frequencia = freq*1000.0; 00054 float periodo = 1000000.0/frequencia; 00055 PwmOut *SD; 00056 00057 SD1.write(0.0f); 00058 SD2.write(0.0f); 00059 SD3.write(0.0f); 00060 SD4.write(0.0f); 00061 SD5.write(0.0f); 00062 SD6.write(0.0f); 00063 00064 switch(port){ 00065 case 0: 00066 SD = &SD1; 00067 break; 00068 case 1: 00069 SD = &SD2; 00070 break; 00071 case 2: 00072 SD = &SD3; 00073 break; 00074 case 3: 00075 SD = &SD4; 00076 break; 00077 case 4: 00078 SD = &SD5; 00079 break; 00080 case 5: 00081 SD = &SD6; 00082 break; 00083 } 00084 00085 //pc.printf("Enviando o pacote IR na porta %lu com periodo %f e frequencia %f.\r\n",port,periodo,frequencia); 00086 00087 //SD->period(1.0/(freq*1000.0)); 00088 SD->period_us(periodo); 00089 00090 //pc.printf("Iniciando o envio IR.\r\n"); 00091 for(i=0;i<indexIr;i++){ 00092 SD->write(0.5); 00093 wait_us(bits[i][0]); 00094 00095 SD->write(0.0); 00096 wait_us(bits[i][1]); 00097 } 00098 //pc.printf("Finalizado o envio IR.\r\n"); 00099 00100 SD1.period_us(pwmPeriod); 00101 SD1.write(sd1Duty); 00102 SD2.write(sd2Duty); 00103 SD3.write(sd3Duty); 00104 SD4.write(sd4Duty); 00105 SD5.write(sd5Duty); 00106 SD6.write(sd6Duty); 00107 } 00108 00109 00110 /*static const long hextable[] = { 00111 [0 ... 255] = -1, // bit aligned access into this table is considerably 00112 ['0'] = 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, // faster for most modern processors, 00113 ['A'] = 10, 11, 12, 13, 14, 15, // for the space conscious, reduce to 00114 ['a'] = 10, 11, 12, 13, 14, 15 // signed char. 00115 };*/ 00116 00117 uint8_t hextable(char c){ 00118 uint8_t ret=0; 00119 if(c>='a'){ 00120 ret = c-87; 00121 }else if(c>='A'){ 00122 ret = c-55; 00123 }else{ 00124 ret = c-48; 00125 } 00126 return ret; 00127 } 00128 00129 uint16_t hexdec(char *hex,uint16_t tam) { 00130 uint16_t ret = 0; 00131 //uint8_t auxHex=0; 00132 uint16_t i; 00133 for(i=0;i<tam;i++){ 00134 //ret += hextable[hex[i]] << (i*4); 00135 ret += hextable(hex[i]) << (i*4); 00136 //pc.printf("%c",hex[i]); 00137 } 00138 //pc.printf("\r\n"); 00139 return ret; 00140 } 00141 00142 00143 void deserializaPacoteIR(char *strIr){ 00144 uint16_t len; 00145 uint16_t i; 00146 00147 len = strlen(strIr); 00148 len = len/4; //Separando de quatro em quatro pra formar um uint16_t 00149 00150 for(i=0;i<len;i++){ 00151 bits[i/2][(i%2)]=hexdec(strIr,4); 00152 strIr = strIr+4; 00153 } 00154 indexIr = len/2; 00155 } 00156 00157 void serializaPacoteIR(uint8_t connectionID){ 00158 char aux[50]; 00159 uint32_t length; 00160 char *ptr; 00161 uint16_t i; 00162 union{ 00163 char c[2]; 00164 uint16_t v; 00165 }u; 00166 sdCardBuf.del(); 00167 for(i=0;i<indexIr;i++){ 00168 u.v = bits[i][0]; 00169 sprintf(aux,"%01X%01X%01X%01X" 00170 ,u.c[0]&0xF 00171 ,u.c[0]>>4 00172 ,u.c[1]&0xF 00173 ,u.c[1]>>4 00174 ); 00175 sdCardBuf.fill(aux,strlen(aux)); 00176 00177 u.v = bits[i][1]; 00178 sprintf(aux,"%01X%01X%01X%01X" 00179 ,u.c[0]&0xF 00180 ,u.c[0]>>4 00181 ,u.c[1]&0xF 00182 ,u.c[1]>>4 00183 ); 00184 sdCardBuf.fill(aux,strlen(aux)); 00185 } 00186 length = sdCardBuf.getLength(); 00187 ptr = sdCardBuf.get(); 00188 modemCom::cipSend(connectionID,ptr,length); 00189 //pc.printf("Conteudo de sdCardBuf <%s>.\r\n",ptr); 00190 } 00191 00192 bool detectaIRIn(){ 00193 bool detectado=0; 00194 if((irInAntes!=IrIn) && (!IrIn)){ 00195 indexIr = 0; 00196 do{ 00197 bits[indexIr][0] = pulseDuration_us(0,IrIn,65000); 00198 bits[indexIr][1] = pulseDuration_us(1,IrIn,65000); 00199 indexIr++; 00200 }while((bits[indexIr-1][0]!=65535)&&(bits[indexIr-1][1]!=65535)); 00201 detectado=1; 00202 } 00203 irInAntes = IrIn; 00204 pc.printf("Total de indexIr = %lu bits.\r\n",indexIr); 00205 return detectado; 00206 } 00207 00208 void pesquisaIRIn(){ 00209 if((irInAntes!=IrIn) && (!IrIn)){ 00210 indexIr = 0; 00211 do{ 00212 bitAlto[indexIr] = pulseDuration_us(0,IrIn,65000); 00213 bitBaixo[indexIr] = pulseDuration_us(1,IrIn,65000); 00214 //dataIn[indexIr] = (bitBaixo[indexIr] > 1000); 00215 indexIr++; 00216 }while((bitAlto[indexIr-1]!=65535)&&(bitBaixo[indexIr-1]!=65535)); 00217 00218 pc.printf("Capturado um quadro com %lu bits. sbH %ld, sbL %ld <",indexIr,startBitAlto, startBitBaixo); 00219 for(i=0;i<(indexIr);i++){ 00220 pc.printf("[H%ld L%ld] ",bitAlto[i],bitBaixo[i]); 00221 } 00222 pc.printf(">.\n\n"); 00223 } 00224 irInAntes = IrIn; 00225 } 00226 00227 /* 00228 00229 float razaoBit(int iH, int iL){ 00230 float H=(float)iH,L=(float)iL; 00231 float razao = L/H; 00232 00233 if(razao>=1){ 00234 return (float) round(razao); 00235 }else if(razao>=0.5){ 00236 return 0.5; 00237 }else if(razao>=0.25){ 00238 return 0.25; 00239 }else if(razao>=0.125){ 00240 return 0.125; 00241 }else{ 00242 return -1; 00243 } 00244 00245 } 00246 00247 void descritorPacoteIR(int startBitAlto, int startBitBaixo, int *bitAlto, int *bitBaixo, int numBits){ 00248 unsigned int razao_maiorQue4=0; 00249 unsigned int razao_4=0; 00250 unsigned int razao_3=0; 00251 unsigned int razao_2=0; 00252 unsigned int razao_1=0; 00253 unsigned int razao_0_5=0; 00254 unsigned int razao_0_25=0; 00255 unsigned int razao_0_125=0; 00256 unsigned int razao_menorQue_0_125=0; 00257 00258 //Indice 0 ALto, 1 Baixo; 00259 unsigned long int bitRazao_maiorQue4[2]= {0,0}; 00260 unsigned long int bitRazao_4[2]= {0,0}; 00261 unsigned long int bitRazao_3[2]= {0,0}; 00262 unsigned long int bitRazao_2[2]= {0,0}; 00263 unsigned long int bitRazao_1[2]= {0,0}; 00264 unsigned long int bitRazao_0_5[2]= {0,0}; 00265 unsigned long int bitRazao_0_25[2]= {0,0}; 00266 unsigned long int bitRazao_0_125[2]= {0,0}; 00267 unsigned long int bitRazao_menorQue_0_125[2]= {0,0}; 00268 00269 unsigned long int indiceMaiorNEventos[2] = {0,0}; 00270 unsigned long int indiceSegundoMaiorNEventos[2] = {0,0}; 00271 00272 00273 00274 unsigned int bitAlto_H=0; 00275 unsigned int bitAlto_L=0; 00276 unsigned int bitBaixo_H=0; 00277 unsigned int bitBaixo_L=0; 00278 00279 unsigned int stopBitAlto = bitAlto[numBits-1]; 00280 00281 int i; 00282 00283 //printf("startBitAlto %d, startBitBaixo %d, stopBitAlto %d, stopBitBaixo %d numBits %d\n",startBitAlto,startBitBaixo,bitAlto[numBits-1],bitBaixo[numBits-1],numBits); 00284 00285 //Despresando o stopBit pois esse já sabemos como faz 00286 for(i=0;i<numBits-1;i++){ 00287 //printf("Razao entre [L %05d e H %05d]=%1.2f\n",bitBaixo[i],bitAlto[i],razaoBit(bitAlto[i],bitBaixo[i])); 00288 //Detecção de padroes 00289 if(razaoBit(bitAlto[i],bitBaixo[i])>=4){ 00290 razao_maiorQue4++; 00291 bitRazao_maiorQue4[0]+= bitAlto[i]; 00292 bitRazao_maiorQue4[1]+=bitBaixo[i]; 00293 00294 }else if(razaoBit(bitAlto[i],bitBaixo[i])==4){ 00295 razao_4++; 00296 bitRazao_4[0]+= bitAlto[i]; 00297 bitRazao_4[1]+=bitBaixo[i]; 00298 }else if(razaoBit(bitAlto[i],bitBaixo[i])==3){ 00299 razao_3++; 00300 bitRazao_3[0]+= bitAlto[i]; 00301 bitRazao_3[1]+=bitBaixo[i]; 00302 }else if(razaoBit(bitAlto[i],bitBaixo[i])==2){ 00303 razao_2++; 00304 bitRazao_2[0]+= bitAlto[i]; 00305 bitRazao_2[1]+=bitBaixo[i]; 00306 }else if(razaoBit(bitAlto[i],bitBaixo[i])==1){ 00307 razao_1++; 00308 bitRazao_1[0]+= bitAlto[i]; 00309 bitRazao_1[1]+=bitBaixo[i]; 00310 }else if(razaoBit(bitAlto[i],bitBaixo[i])==0.5){ 00311 razao_0_5++; 00312 bitRazao_0_5[0]+= bitAlto[i]; 00313 bitRazao_0_5[1]+=bitBaixo[i]; 00314 }else if(razaoBit(bitAlto[i],bitBaixo[i])==0.25){ 00315 razao_0_25++; 00316 bitRazao_0_25[0]+= bitAlto[i]; 00317 bitRazao_0_25[1]+=bitBaixo[i]; 00318 }else if(razaoBit(bitAlto[i],bitBaixo[i])==0.125){ 00319 razao_0_125++; 00320 bitRazao_0_125[0]+= bitAlto[i]; 00321 bitRazao_0_125[1]+=bitBaixo[i]; 00322 }else { 00323 razao_menorQue_0_125++; 00324 bitRazao_menorQue_0_125[0]+= bitAlto[i]; 00325 bitRazao_menorQue_0_125[1]+=bitBaixo[i]; 00326 } 00327 00328 } 00329 printf("Razoes <0.125 = %d\n 0.125 = %d\n 0.25 = %d\n 0.5 = %d\n 1 = %d\n 2 = %d\n 3 = %d\n 4 = %d\n maior que 4 = %d\n" 00330 ,razao_menorQue_0_125 00331 ,razao_0_125 00332 ,razao_0_25 00333 ,razao_0_5 00334 ,razao_1 00335 ,razao_2 00336 ,razao_3 00337 ,razao_4 00338 ,razao_maiorQue4 00339 ); 00340 00341 //Buscando os dois maiores em numero de eventos por Indice; 00342 //Buscando o primeiro 00343 for(i=0;i<9;i++){ 00344 //Buscando nos indices maior numero eventos 00345 switch(i){ 00346 case 0: 00347 if(razao_maiorQue4>indiceMaiorNEventos[0]){ 00348 indiceMaiorNEventos[0] = razao_maiorQue4; 00349 indiceMaiorNEventos[1] = i; 00350 } 00351 break; 00352 00353 case 1: 00354 if(razao_4>indiceMaiorNEventos[0]){ 00355 indiceMaiorNEventos[0] = razao_4; 00356 indiceMaiorNEventos[1] = i; 00357 } 00358 break; 00359 case 2: 00360 if(razao_3>indiceMaiorNEventos[0]){ 00361 indiceMaiorNEventos[0] = razao_3; 00362 indiceMaiorNEventos[1] = i; 00363 } 00364 break; 00365 case 3: 00366 if(razao_2>indiceMaiorNEventos[0]){ 00367 indiceMaiorNEventos[0] = razao_2; 00368 indiceMaiorNEventos[1] = i; 00369 } 00370 break; 00371 case 4: 00372 if(razao_1>indiceMaiorNEventos[0]){ 00373 indiceMaiorNEventos[0] = razao_1; 00374 indiceMaiorNEventos[1] = i; 00375 } 00376 break; 00377 case 5: 00378 if(razao_0_5>indiceMaiorNEventos[0]){ 00379 indiceMaiorNEventos[0] = razao_0_5; 00380 indiceMaiorNEventos[1] = i; 00381 } 00382 break; 00383 case 6: 00384 if(razao_0_25>indiceMaiorNEventos[0]){ 00385 indiceMaiorNEventos[0] = razao_0_25; 00386 indiceMaiorNEventos[1] = i; 00387 } 00388 break; 00389 case 7: 00390 if(razao_0_125>indiceMaiorNEventos[0]){ 00391 indiceMaiorNEventos[0] = razao_0_125; 00392 indiceMaiorNEventos[1] = i; 00393 } 00394 break; 00395 case 8: 00396 if(razao_menorQue_0_125>indiceMaiorNEventos[0]){ 00397 indiceMaiorNEventos[0] = razao_menorQue_0_125; 00398 indiceMaiorNEventos[1] = i; 00399 } 00400 break; 00401 00402 } 00403 } 00404 00405 //Buscando o segundo 00406 //Buscando o primeiro 00407 for(i=0;i<9;i++){ 00408 //Buscando nos indices maior numero eventos 00409 if(i!=indiceMaiorNEventos[1]){ 00410 switch(i){ 00411 case 0: 00412 if(razao_maiorQue4>indiceSegundoMaiorNEventos[0]){ 00413 indiceSegundoMaiorNEventos[0] = razao_maiorQue4; 00414 indiceSegundoMaiorNEventos[1] = i; 00415 } 00416 break; 00417 00418 case 1: 00419 if(razao_4>indiceSegundoMaiorNEventos[0]){ 00420 indiceSegundoMaiorNEventos[0] = razao_4; 00421 indiceSegundoMaiorNEventos[1] = i; 00422 } 00423 break; 00424 case 2: 00425 if(razao_3>indiceSegundoMaiorNEventos[0]){ 00426 indiceSegundoMaiorNEventos[0] = razao_3; 00427 indiceSegundoMaiorNEventos[1] = i; 00428 } 00429 break; 00430 case 3: 00431 if(razao_2>indiceSegundoMaiorNEventos[0]){ 00432 indiceSegundoMaiorNEventos[0] = razao_2; 00433 indiceSegundoMaiorNEventos[1] = i; 00434 } 00435 break; 00436 case 4: 00437 if(razao_1>indiceSegundoMaiorNEventos[0]){ 00438 indiceSegundoMaiorNEventos[0] = razao_1; 00439 indiceSegundoMaiorNEventos[1] = i; 00440 } 00441 break; 00442 case 5: 00443 if(razao_0_5>indiceSegundoMaiorNEventos[0]){ 00444 indiceSegundoMaiorNEventos[0] = razao_0_5; 00445 indiceSegundoMaiorNEventos[1] = i; 00446 } 00447 break; 00448 case 6: 00449 if(razao_0_25>indiceSegundoMaiorNEventos[0]){ 00450 indiceSegundoMaiorNEventos[0] = razao_0_25; 00451 indiceSegundoMaiorNEventos[1] = i; 00452 } 00453 break; 00454 case 7: 00455 if(razao_0_125>indiceSegundoMaiorNEventos[0]){ 00456 indiceSegundoMaiorNEventos[0] = razao_0_125; 00457 indiceSegundoMaiorNEventos[1] = i; 00458 } 00459 break; 00460 case 8: 00461 if(razao_menorQue_0_125>indiceSegundoMaiorNEventos[0]){ 00462 indiceSegundoMaiorNEventos[0] = razao_menorQue_0_125; 00463 indiceSegundoMaiorNEventos[1] = i; 00464 } 00465 break; 00466 00467 } 00468 } 00469 } 00470 00471 00472 //printf("Indice de maior aparecimento foi o de %d.\nIndice de segundo maior aparecimento foi o de %d.\n",indiceMaiorNEventos[1],indiceSegundoMaiorNEventos[1]); 00473 00474 //Calculando bitAlto 00475 switch(indiceMaiorNEventos[1]){ 00476 case 0: 00477 bitAlto_H = round(bitRazao_maiorQue4[0]/indiceMaiorNEventos[0]); 00478 bitAlto_L = round(bitRazao_maiorQue4[1]/indiceMaiorNEventos[0]); 00479 break; 00480 case 1: 00481 bitAlto_H = round(bitRazao_4[0]/indiceMaiorNEventos[0]); 00482 bitAlto_L = round(bitRazao_4[1]/indiceMaiorNEventos[0]); 00483 break; 00484 case 2: 00485 bitAlto_H = round(bitRazao_3[0]/indiceMaiorNEventos[0]); 00486 bitAlto_L = round(bitRazao_3[1]/indiceMaiorNEventos[0]); 00487 break; 00488 case 3: 00489 bitAlto_H = round(bitRazao_2[0]/indiceMaiorNEventos[0]); 00490 bitAlto_L = round(bitRazao_2[1]/indiceMaiorNEventos[0]); 00491 break; 00492 case 4: 00493 bitAlto_H = round(bitRazao_1[0]/indiceMaiorNEventos[0]); 00494 bitAlto_L = round(bitRazao_1[1]/indiceMaiorNEventos[0]); 00495 break; 00496 case 5: 00497 bitAlto_H = round(bitRazao_0_5[0]/indiceMaiorNEventos[0]); 00498 bitAlto_L = round(bitRazao_0_5[1]/indiceMaiorNEventos[0]); 00499 break; 00500 case 6: 00501 bitAlto_H = round(bitRazao_0_25[0]/indiceMaiorNEventos[0]); 00502 bitAlto_L = round(bitRazao_0_25[1]/indiceMaiorNEventos[0]); 00503 break; 00504 case 7: 00505 bitAlto_H = round(bitRazao_0_125[0]/indiceMaiorNEventos[0]); 00506 bitAlto_L = round(bitRazao_0_125[1]/indiceMaiorNEventos[0]); 00507 break; 00508 case 8: 00509 bitAlto_H = round(bitRazao_menorQue_0_125[0]/indiceMaiorNEventos[0]); 00510 bitAlto_L = round(bitRazao_menorQue_0_125[1]/indiceMaiorNEventos[0]); 00511 break; 00512 } 00513 00514 //Calculando bitBaixo 00515 switch(indiceSegundoMaiorNEventos[1]){ 00516 case 0: 00517 bitBaixo_H = round(bitRazao_maiorQue4[0]/indiceSegundoMaiorNEventos[0]); 00518 bitBaixo_L = round(bitRazao_maiorQue4[1]/indiceSegundoMaiorNEventos[0]); 00519 break; 00520 case 1: 00521 bitBaixo_H = round(bitRazao_4[0]/indiceSegundoMaiorNEventos[0]); 00522 bitBaixo_L = round(bitRazao_4[1]/indiceSegundoMaiorNEventos[0]); 00523 break; 00524 case 2: 00525 bitBaixo_H = round(bitRazao_3[0]/indiceSegundoMaiorNEventos[0]); 00526 bitBaixo_L = round(bitRazao_3[1]/indiceSegundoMaiorNEventos[0]); 00527 break; 00528 case 3: 00529 bitBaixo_H = round(bitRazao_2[0]/indiceSegundoMaiorNEventos[0]); 00530 bitBaixo_L = round(bitRazao_2[1]/indiceSegundoMaiorNEventos[0]); 00531 break; 00532 case 4: 00533 bitBaixo_H = round(bitRazao_1[0]/indiceSegundoMaiorNEventos[0]); 00534 bitBaixo_L = round(bitRazao_1[1]/indiceSegundoMaiorNEventos[0]); 00535 break; 00536 case 5: 00537 bitBaixo_H = round(bitRazao_0_5[0]/indiceSegundoMaiorNEventos[0]); 00538 bitBaixo_L = round(bitRazao_0_5[1]/indiceSegundoMaiorNEventos[0]); 00539 break; 00540 case 6: 00541 bitBaixo_H = round(bitRazao_0_25[0]/indiceSegundoMaiorNEventos[0]); 00542 bitBaixo_L = round(bitRazao_0_25[1]/indiceSegundoMaiorNEventos[0]); 00543 break; 00544 case 7: 00545 bitBaixo_H = round(bitRazao_0_125[0]/indiceSegundoMaiorNEventos[0]); 00546 bitBaixo_L = round(bitRazao_0_125[1]/indiceSegundoMaiorNEventos[0]); 00547 break; 00548 case 8: 00549 bitBaixo_H = round(bitRazao_menorQue_0_125[0]/indiceSegundoMaiorNEventos[0]); 00550 bitBaixo_L = round(bitRazao_menorQue_0_125[1]/indiceSegundoMaiorNEventos[0]); 00551 break; 00552 } 00553 00554 printf("\n\nFinalmente.\n"); 00555 printf("startBitAlto = %ld, startBitBaixo = %ld\n", startBitAlto,startBitBaixo); 00556 printf("bitAlto_H = %ld bitAlto_l = %ld\n",bitAlto_H,bitAlto_L); 00557 printf("bitBaixo_H = %ld bitBaixo_l = %ld\n",bitBaixo_H,bitBaixo_L); 00558 printf("stopBitAlto = %ld\n",stopBitAlto); 00559 00560 return; 00561 }*/
Generated on Wed Jul 13 2022 12:46:24 by
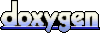