GPS to Pulga
Embed:
(wiki syntax)
Show/hide line numbers
BLEProcess.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef GATT_SERVER_EXAMPLE_BLE_PROCESS_H_ 00018 #define GATT_SERVER_EXAMPLE_BLE_PROCESS_H_ 00019 00020 #include <stdint.h> 00021 #include <stdio.h> 00022 00023 #include "events/EventQueue.h" 00024 #include "platform/Callback.h" 00025 #include "platform/NonCopyable.h" 00026 00027 #include "ble/BLE.h" 00028 #include "ble/Gap.h" 00029 #include "ble/GapAdvertisingParams.h" 00030 #include "ble/GapAdvertisingData.h" 00031 #include "ble/FunctionPointerWithContext.h" 00032 00033 //DigitalOut led1(P1_13); 00034 /** 00035 * Handle initialization adn shutdown of the BLE Instance. 00036 * 00037 * Setup advertising payload and manage advertising state. 00038 * Delegate to GattClientProcess once the connection is established. 00039 */ 00040 class BLEProcess : private mbed::NonCopyable<BLEProcess> { 00041 public: 00042 /** 00043 * Construct a BLEProcess from an event queue and a ble interface. 00044 * 00045 * Call start() to initiate ble processing. 00046 */ 00047 BLEProcess(events::EventQueue &event_queue, BLE &ble_interface) : 00048 _event_queue(event_queue), 00049 _ble_interface(ble_interface), 00050 _post_init_cb() { 00051 } 00052 00053 ~BLEProcess() 00054 { 00055 stop(); 00056 } 00057 00058 /** 00059 * Subscription to the ble interface initialization event. 00060 * 00061 * @param[in] cb The callback object that will be called when the ble 00062 * interface is initialized. 00063 */ 00064 void on_init(mbed::Callback<void(BLE&, events::EventQueue&)> cb) 00065 { 00066 _post_init_cb = cb; 00067 } 00068 00069 /** 00070 * Initialize the ble interface, configure it and start advertising. 00071 */ 00072 bool start() 00073 { 00074 printf("Ble process started.\r\n"); 00075 00076 00077 if (_ble_interface.hasInitialized()) { 00078 printf("Error: the ble instance has already been initialized.\r\n"); 00079 return false; 00080 } 00081 00082 _ble_interface.onEventsToProcess( 00083 makeFunctionPointer(this, &BLEProcess::schedule_ble_events) 00084 ); 00085 00086 ble_error_t error = _ble_interface.init( 00087 this, &BLEProcess::when_init_complete 00088 ); 00089 00090 if (error) { 00091 printf("Error: %u returned by BLE::init.\r\n", error); 00092 return false; 00093 } 00094 00095 return true; 00096 } 00097 00098 /** 00099 * Close existing connections and stop the process. 00100 */ 00101 void stop() 00102 { 00103 if (_ble_interface.hasInitialized()) { 00104 _ble_interface.shutdown(); 00105 printf("Ble process stopped."); 00106 } 00107 } 00108 00109 private: 00110 00111 /** 00112 * Schedule processing of events from the BLE middleware in the event queue. 00113 */ 00114 void schedule_ble_events(BLE::OnEventsToProcessCallbackContext *event) 00115 { 00116 _event_queue.call(mbed::callback(&event->ble, &BLE::processEvents)); 00117 } 00118 00119 /** 00120 * Sets up adverting payload and start advertising. 00121 * 00122 * This function is invoked when the ble interface is initialized. 00123 */ 00124 void when_init_complete(BLE::InitializationCompleteCallbackContext *event) 00125 { 00126 if (event->error) { 00127 printf("Error %u during the initialization\r\n", event->error); 00128 return; 00129 } 00130 printf("Ble instance initialized\r\n"); 00131 00132 Gap &gap = _ble_interface.gap(); 00133 gap.onConnection(this, &BLEProcess::when_connection); 00134 gap.onDisconnection(this, &BLEProcess::when_disconnection); 00135 00136 if (!set_advertising_parameters()) { 00137 return; 00138 } 00139 00140 if (!set_advertising_data()) { 00141 return; 00142 } 00143 00144 if (!start_advertising()) { 00145 return; 00146 } 00147 00148 if (_post_init_cb) { 00149 _post_init_cb(_ble_interface, _event_queue); 00150 } 00151 } 00152 00153 void when_connection(const Gap::ConnectionCallbackParams_t *connection_event) 00154 { 00155 printf("Connected.\r\n"); 00156 } 00157 00158 void when_disconnection(const Gap::DisconnectionCallbackParams_t *event) 00159 { 00160 printf("Disconnected.\r\n"); 00161 start_advertising(); 00162 } 00163 00164 bool start_advertising(void) 00165 { 00166 Gap &gap = _ble_interface.gap(); 00167 00168 /* Start advertising the set */ 00169 ble_error_t error = gap.startAdvertising(ble::LEGACY_ADVERTISING_HANDLE); 00170 00171 if (error) { 00172 printf("Error %u during gap.startAdvertising.\r\n", error); 00173 return false; 00174 } else { 00175 printf("Advertising started.\r\n"); 00176 return true; 00177 } 00178 } 00179 00180 bool set_advertising_parameters() 00181 { 00182 Gap &gap = _ble_interface.gap(); 00183 00184 ble_error_t error = gap.setAdvertisingParameters( 00185 ble::LEGACY_ADVERTISING_HANDLE, 00186 ble::AdvertisingParameters() 00187 ); 00188 00189 if (error) { 00190 printf("Gap::setAdvertisingParameters() failed with error %d", error); 00191 return false; 00192 } 00193 00194 return true; 00195 } 00196 00197 bool set_advertising_data() 00198 { 00199 Gap &gap = _ble_interface.gap(); 00200 00201 /* Use the simple builder to construct the payload; it fails at runtime 00202 * if there is not enough space left in the buffer */ 00203 ble_error_t error = gap.setAdvertisingPayload( 00204 ble::LEGACY_ADVERTISING_HANDLE, 00205 ble::AdvertisingDataSimpleBuilder<ble::LEGACY_ADVERTISING_MAX_SIZE>() 00206 .setFlags() 00207 .setName("Pulga_Arquetipo") 00208 .getAdvertisingData() 00209 ); 00210 00211 if (error) { 00212 printf("Gap::setAdvertisingPayload() failed with error %d", error); 00213 return false; 00214 } 00215 00216 return true; 00217 } 00218 00219 events::EventQueue &_event_queue; 00220 BLE &_ble_interface; 00221 mbed::Callback<void(BLE&, events::EventQueue&)> _post_init_cb; 00222 }; 00223 00224 #endif /* GATT_SERVER_EXAMPLE_BLE_PROCESS_H_ */
Generated on Thu Jul 28 2022 03:16:26 by
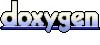