
AT&T example LPC1768
Dependencies: EthernetInterface M2XStreamClient jsonlite mbed-rtos mbed
Fork of m2x-demo-all by
main.cpp
00001 #define MBED_PLATFORM 00002 00003 #include <jsonlite.h> 00004 #include "M2XStreamClient.h" 00005 00006 #include "mbed.h" 00007 #include "EthernetInterface.h" 00008 00009 char deviceId[] = "8f4eeb39f1cb1dc95699bfa266e57662"; // Device you want to push to 00010 char streamName[] = "temp_data"; // Stream you want to push to 00011 char m2xKey[] = "4c3ba5e22fc074d4358888afbe96efa7"; // Your M2X API Key or Master API Key 00012 00013 // For Static IP 00014 static const char* mbedIp = "192.168.0.85"; //IP 00015 static const char* mbedMask = "255.255.255.0"; // Mask 00016 static const char* mbedGateway = "192.168.0.254"; //Gateway 00017 00018 Client client; 00019 M2XStreamClient m2xClient(&client, m2xKey); 00020 00021 EthernetInterface eth; 00022 00023 00024 int main() { 00025 float data = 32.5; 00026 00027 eth.init(mbedIp,mbedMask,mbedGateway); 00028 eth.connect(); 00029 printf("IP Address: %s\n", eth.getIPAddress()); 00030 00031 while (true) { 00032 double val = data; 00033 printf("Current temperature is: %lf", val); 00034 00035 int response = m2xClient.updateStreamValue(deviceId, streamName, val); 00036 printf("Response code: %d\n", response); 00037 00038 if (response == -1) while (true) ; 00039 00040 delay(5000); 00041 } 00042 }
Generated on Fri Jul 15 2022 21:50:22 by
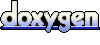