
Test program for RFM69 library. Listens and prints packets from "RFM69_Sender" nodes
Embed:
(wiki syntax)
Show/hide line numbers
Listener.cpp
00001 // Listener - print sender messages 00002 // From Tnode/Tsender @ anarduino.com 00003 // 2014 - anarduino.com 00004 // 00005 #include <RFM69.h> 00006 #include <SPI.h> 00007 00008 #define GATEWAY_ID 1 // this is ME, TGateway 00009 #define NETWORKID 101 //the same on all nodes that talk to each other 00010 00011 // Uncomment only one of the following three to match radio frequency 00012 //#define FREQUENCY RF69_433MHZ 00013 //#define FREQUENCY RF69_868MHZ 00014 #define FREQUENCY RF69_915MHZ 00015 00016 //#define IS_RFM69HW //NOTE: uncomment this ONLY for RFM69HW or RFM69HCW 00017 #define ENCRYPT_KEY "EncryptKey123456" // use same 16byte encryption key for all devices on net 00018 #define ACK_TIME 50 // max msec for ACK wait 00019 #define LED 9 // Anardino miniWireless has LEDs on D9 00020 #define SERIAL_BAUD 115200 00021 #define VERSION "1.0" 00022 00023 #define MSGBUFSIZE 64 // message buffersize, but for this demo we only use: 00024 // 1-byte NODEID + 4-bytes for time + 1-byte for temp in C + 2-bytes for vcc(mV) 00025 uint8_t msgBuf[MSGBUFSIZE]; 00026 InterruptIn mybutton(USER_BUTTON); 00027 00028 #ifdef TARGET_NUCLEO_F401RE 00029 Serial pc(USBTX, USBRX); 00030 DigitalOut myled(D9); //"Moteino Half Shield" has LED on D9 00031 //RFM69::RFM69(PinName PinName mosi, PinName miso, PinName sclk,slaveSelectPin, PinName int) 00032 RFM69 radio(D11,D12,D13,D10,D8); 00033 #elif defined(TARGET_NUCLEO_L152RE) 00034 Serial pc(USBTX, USBRX); 00035 DigitalOut myled(D9); //"Moteino Half Shield" has LED on D9 00036 //RFM69::RFM69(PinName PinName mosi, PinName miso, PinName sclk,slaveSelectPin, PinName int) 00037 RFM69 radio(D11,D12,D13,D10,D8); 00038 #elif defined(TARGET_LPC1114) 00039 Serial pc(USBTX, USBRX); 00040 DigitalOut myled(LED1); 00041 //RFM69::RFM69(PinName PinName mosi, PinName miso, PinName sclk,slaveSelectPin, PinName int) 00042 RFM69 radio(dp2,dp1,dp6,dp4,dp9); 00043 #elif defined(TARGET_LPC1768) 00044 Serial pc(USBTX, USBRX); 00045 DigitalOut myled(D9); //"Moteino Half Shield" has LED on D9 00046 //RFM69::RFM69(PinName PinName mosi, PinName miso, PinName sclk,slaveSelectPin, PinName int) 00047 RFM69 radio(D11,D12,D13,D10,D8); // Seeedstudio Arch Pro 00048 //RFM69 radio(p5, p6, p7, p10, p9,p8) // Mbed 1768 ? 00049 #endif 00050 bool promiscuousMode = false; // set 'true' to sniff all packets on the same network 00051 bool requestACK=false; 00052 Timer tmr; 00053 00054 main() { 00055 memset(msgBuf,0,sizeof(msgBuf)); 00056 uint8_t theNodeID; 00057 tmr.start(); 00058 00059 pc.baud(SERIAL_BAUD); 00060 pc.printf("\r\nListener %s startup at %d Mhz...\r\n",VERSION,(FREQUENCY==RF69_433MHZ ? 433 : FREQUENCY==RF69_868MHZ ? 868 : 915)); 00061 wait(1); 00062 radio.initialize(FREQUENCY, GATEWAY_ID, NETWORKID); 00063 radio.encrypt(0); 00064 radio.promiscuous(promiscuousMode); 00065 // radio.setPowerLevel(5); 00066 #ifdef IS_RFM69HW 00067 radio.setHighPower(); //uncomment #define ONLY if radio is of type: RFM69HW or RFM69HCW 00068 #endif 00069 00070 while(1) { 00071 if (radio.receiveDone()) { 00072 pc.printf("Received from TNODE: %d ",radio.SENDERID); 00073 pc.printf((char*)radio.DATA); 00074 if (radio.ACKRequested()){ 00075 theNodeID = radio.SENDERID; 00076 radio.sendACK(); 00077 pc.printf(" - ACK sent. Receive RSSI: %d\r\n",radio.RSSI); 00078 } else pc.printf("Receive RSSI: %d\r\n",radio.RSSI); 00079 } 00080 myled = !myled; 00081 } 00082 }
Generated on Thu Jul 14 2022 17:13:57 by
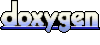