Generic Step Motor WebInterface - control a step motor using a Pololu A4983 driver from a webinterface (EXPERIMENTAL PROTOTYPE - just to be used as a proof-of-concept for a IoT talk, will not be updating this code so often)
Dependencies: EthernetNetIf RPCInterface mbed HTTPServer
TObjects.h
00001 //--------------------------------------------------------------------------------------------- 00002 #include "mbed.h" 00003 //--------------------------------------------------------------------------------------------- 00004 #define internaldebug 00005 //--------------------------------------------------------------------------------------------- 00006 class TObjects 00007 { 00008 private: 00009 00010 public: 00011 00012 TObjects(); 00013 }; 00014 //--------------------------------------------------------------------------------------------- 00015 00016 class TObjectStep 00017 { 00018 private: 00019 00020 public: 00021 static const uint8_t typecode = 0x01; 00022 uint32_t nsteps; 00023 uint32_t deltatime; 00024 uint8_t direction; 00025 00026 TObjectStep(uint32_t insteps, uint32_t ideltatime, uint8_t idirection) 00027 { 00028 nsteps = insteps; 00029 deltatime = ideltatime; 00030 direction = idirection; 00031 00032 #ifdef internaldebug 00033 printf("->->TObjectStep(%d,%d,%d).\r\n",nsteps,deltatime,direction); 00034 #endif 00035 }; 00036 }; 00037 //--------------------------------------------------------------------------------------------- 00038 00039 class TObjectWait 00040 { 00041 private: 00042 00043 public: 00044 static const uint8_t typecode = 0x04; 00045 uint32_t waittime; 00046 00047 TObjectWait(uint32_t iwaittime) 00048 { 00049 waittime = iwaittime; 00050 }; 00051 }; 00052 //---------------------------------------------------------------------------------------------
Generated on Wed Jul 13 2022 06:36:07 by
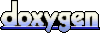