Simple code to demonstrate how to manually use RPC functionality to easily access the microcontroller hardware via WebInterface. Note: Demo program to be used on the GeekSessionLab Talk (November 2011). http://devrendezvous.com/?lang=en
Dependencies: EthernetNetIf mbed HTTPServer
main.cpp
00001 /* //--------------------------------------------------------------------------------------------- 00002 http://192.168.1.100/rpc/DigitalOut/new%20LED1%20myled1 00003 00004 http://192.168.1.100/rpc/DigitalOut/new LED1 myled1 00005 http://192.168.1.100/rpc/DigitalOut/new LED2 myled2 00006 http://192.168.1.100/rpc/DigitalOut/new LED3 myled3 00007 http://192.168.1.100/rpc/DigitalOut/new LED4 myled4 00008 00009 http://192.168.1.100/rpc/ 00010 00011 http://192.168.1.100/rpc/myled1/read 00012 http://192.168.1.100/rpc/myled1/write 1 00013 00014 http://192.168.1.100/rpc/myled2/write 2 00015 http://192.168.1.100/rpc/myled3/write 3 00016 http://192.168.1.100/rpc/myled4/write 4 00017 00018 //--------------------------------------------------------------------------------------------- 00019 // resources 00020 //--------------------------------------------------------------------------------------------- 00021 http://mbed.org/handbook/C-Data-Types 00022 http://mbed.org/cookbook/RPC-Interface-Library 00023 http://mbed.org/cookbook/HTTP-Server 00024 http://mbed.org/cookbook/Ethernet 00025 http://mbed.org/handbook/Ticker 00026 //--------------------------------------------------------------------------------------------- */ 00027 #include "mbed.h" 00028 #include "EthernetNetIf.h" 00029 #include "HTTPServer.h" 00030 #include "RPCVariable.h" 00031 #include "RPCFunction.h" 00032 //--------------------------------------------------------------------------------------------- 00033 DigitalOut myled1(LED1); 00034 DigitalOut myled2(LED2); 00035 DigitalOut myled3(LED3); 00036 DigitalOut myled4(LED4); 00037 Serial pc(USBTX, USBRX); // tx, rx 00038 //--------------------------------------------------------------------------------------------- 00039 #define internaldebug // send debug messages to USB Serial port (9600,1,N) 00040 //#define dhcpenable // auto-setup IP Address from DHCP router 00041 //--------------------------------------------------------------------------------------------- 00042 // Timer Interrupt - NetPool 00043 //--------------------------------------------------------------------------------------------- 00044 Ticker netpool; 00045 //--------------------------------------------------------------------------------------------- 00046 // Ethernet Object Setup 00047 //--------------------------------------------------------------------------------------------- 00048 #ifdef dhcpenable 00049 EthernetNetIf eth; 00050 #else 00051 EthernetNetIf eth( 00052 IpAddr(192,168,1,100), //IP Address 00053 IpAddr(255,255,255,0), //Network Mask 00054 IpAddr(192,168,1,254), //Gateway 00055 IpAddr(192,168,1,254) //DNS 00056 ); 00057 #endif 00058 //--------------------------------------------------------------------------------------------- 00059 // HTTP Server 00060 //--------------------------------------------------------------------------------------------- 00061 HTTPServer httpserver; 00062 LocalFileSystem fs("webfs"); 00063 //--------------------------------------------------------------------------------------------- 00064 00065 //--------------------------------------------------------------------------------------------- 00066 // ISR -> Pool Ethernet - will be triggered by netpool ticker 00067 //--------------------------------------------------------------------------------------------- 00068 void netpoolupdate() 00069 { 00070 Net::poll(); 00071 } 00072 //--------------------------------------------------------------------------------------------- 00073 00074 //--------------------------------------------------------------------------------------------- 00075 // MAIN 00076 //--------------------------------------------------------------------------------------------- 00077 int main() 00078 { 00079 // Set Serial Port Transfer Rate 00080 pc.baud(115200); 00081 00082 //-------------------------------------------------------- 00083 // Setting RPC 00084 //-------------------------------------------------------- 00085 Base::add_rpc_class<AnalogIn>(); 00086 Base::add_rpc_class<AnalogOut>(); 00087 Base::add_rpc_class<DigitalIn>(); 00088 Base::add_rpc_class<DigitalOut>(); 00089 Base::add_rpc_class<DigitalInOut>(); 00090 Base::add_rpc_class<PwmOut>(); 00091 Base::add_rpc_class<Timer>(); 00092 Base::add_rpc_class<SPI>(); 00093 Base::add_rpc_class<BusOut>(); 00094 Base::add_rpc_class<BusIn>(); 00095 Base::add_rpc_class<BusInOut>(); 00096 Base::add_rpc_class<Serial>(); 00097 //-------------------------------------------------------- 00098 00099 //-------------------------------------------------------- 00100 // Setting Ethernet 00101 //-------------------------------------------------------- 00102 #ifdef internaldebug 00103 printf("\r\nSetting up Ethernet interface!\r\n"); 00104 #endif 00105 // Create return object for error check 00106 EthernetErr ethErr = eth.setup(); 00107 if(ethErr) 00108 { 00109 #ifdef internaldebug 00110 printf("\r\nError %d in Ethernet setup.\r\n", ethErr); 00111 #endif 00112 return -1; 00113 } 00114 #ifdef internaldebug 00115 printf("\r\nEthernet setup completed with success!\r\n"); 00116 #endif 00117 //-------------------------------------------------------- 00118 00119 //-------------------------------------------------------- 00120 // adding Handlers 00121 //-------------------------------------------------------- 00122 FSHandler::mount("/webfs", "/"); //Mount /webfs path on web root path 00123 00124 httpserver.addHandler<RPCHandler>("/rpc"); 00125 httpserver.addHandler<FSHandler>("/"); //Default handler 00126 //-------------------------------------------------------- 00127 00128 //-------------------------------------------------------- 00129 // bind http server to port 80 (Listen) 00130 //-------------------------------------------------------- 00131 httpserver.bind(80); 00132 #ifdef internaldebug 00133 printf("Listening on port 80!\r\n"); 00134 #endif 00135 //-------------------------------------------------------- 00136 00137 //-------------------------------------------------------- 00138 // ISR -> attach timer interrupt to update Net::Pool(); 00139 //-------------------------------------------------------- 00140 netpool.attach(&netpoolupdate, 0.1); 00141 //-------------------------------------------------------- 00142 00143 //-------------------------------------------------------- 00144 // main loop 00145 //-------------------------------------------------------- 00146 // main loop 00147 while(1) 00148 wait(0.5); 00149 } 00150 //---------------------------------------------------------------------------------------------
Generated on Mon Jul 18 2022 16:25:22 by
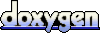