
Bootload from SD card to sector 0, and jump to sector 24 where new firmware resides
Fork of Panel-Controller-Bootloader by
main.cpp
00001 /* Includes */ 00002 #include <stddef.h> 00003 #include "stm32f10x.h" 00004 #include "stm32f10x_usart.h" 00005 #include "main.h" 00006 00007 extern "C" 00008 { 00009 #include "flash.h" 00010 #include "usart.h" 00011 } 00012 00013 /* Private define */ 00014 /* Private macro */ 00015 /* Private variables */ 00016 typedef void (*pFunction)(void); 00017 uint32_t JumpAddress; 00018 pFunction Jump_To_Application; 00019 FLASH_RESULT flashResult; 00020 00021 /* Private function prototypes */ 00022 /* Private functions */ 00023 void RCC_Configuration(); 00024 void NVIC_Configuration(void); 00025 void Timer2_Init(void); 00026 void Timer2_DeInit(void); 00027 void Timer3_Init(void); 00028 void Timer3_DeInit(void); 00029 /** 00030 **=========================================================================== 00031 ** 00032 ** Abstract: main program 00033 ** 00034 **=========================================================================== 00035 */ 00036 int main(void) 00037 { 00038 USART_Initialise(); 00039 USART_SendString("Bootloader EMMA\r\n"); 00040 RCC_Configuration(); 00041 NVIC_Configuration(); 00042 Timer2_Init(); 00043 Timer3_Init(); 00044 USART_SendString("Starting\r\n"); 00045 00046 //If new firmware present on microSD card -> Flash to MCU 00047 flashResult = FlashFirmware(); 00048 USART_SendString("OK\r\n"); 00049 00050 /* Check if the result of the Flash Firmware function */ 00051 if(flashResult == FLASH_OK 00052 || flashResult == FLASH_NO_FILE 00053 || flashResult == FLASH_NO_SD_CARD) 00054 { 00055 USART_SendString("FLASH_OK or NO_FILE or NO_SD\r\n"); 00056 /* Test if user code is programmed starting from address "ApplicationAddress" */ 00057 NVIC_SetVectorTable(NVIC_VectTab_FLASH, 0x6000); 00058 if (((*(__IO uint32_t*)ApplicationAddress) & 0x2FFE0000 ) == 0x20000000) 00059 { 00060 // Disable Timers 00061 Timer2_DeInit(); 00062 Timer3_DeInit(); 00063 00064 /* Jump to user application */ 00065 JumpAddress = *(__IO uint32_t*) (ApplicationAddress + 4); 00066 __disable_irq (); 00067 Jump_To_Application = (pFunction) JumpAddress; 00068 00069 /* Initialise user application's Stack Pointer */ 00070 __set_MSP(*(__IO uint32_t*) ApplicationAddress); 00071 Jump_To_Application(); 00072 } 00073 } 00074 00075 while(1) 00076 { 00077 // Led1Flash(1, 5000); 00078 } 00079 } 00080 00081 void RCC_Configuration() 00082 { 00083 /* GPIOA */ 00084 RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE); 00085 00086 /* GPIOB */ 00087 RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE); 00088 00089 /* GPIOC */ 00090 RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOC, ENABLE); 00091 00092 /* GPIOD */ 00093 RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOD, ENABLE); 00094 00095 /* GPIOE */ 00096 RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOE, ENABLE); 00097 00098 /* AFIO */ 00099 RCC_APB2PeriphClockCmd(RCC_APB2Periph_AFIO, ENABLE); 00100 00101 /* TIM2 */ 00102 RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM2, ENABLE); 00103 00104 /* TIM3 */ 00105 RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM3, ENABLE); 00106 } 00107 00108 void NVIC_Configuration(void) 00109 { 00110 #ifdef VECT_TAB_RAM 00111 /* Set the Vector Table base location at 0x20000000 */ 00112 NVIC_SetVectorTable(NVIC_VectTab_RAM, 0x0); 00113 #else /* VECT_TAB_FLASH */ 00114 /* Set the Vector Table base location at 0x08000000 */ 00115 NVIC_SetVectorTable(NVIC_VectTab_FLASH, 0x0); 00116 #endif 00117 00118 NVIC_InitTypeDef NVIC_InitStructure; 00119 00120 // Enable the TIM3 Interrupt 00121 NVIC_InitStructure.NVIC_IRQChannel = TIM3_IRQn; 00122 NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0; 00123 NVIC_InitStructure.NVIC_IRQChannelSubPriority = 1; 00124 NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE; 00125 NVIC_Init(&NVIC_InitStructure); 00126 } 00127 00128 void Timer2_Init(void) 00129 { 00130 // Time base configuration Timer2 clock 00131 TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure; 00132 TIM_TimeBaseStructure.TIM_Period = 65535; 00133 TIM_TimeBaseStructure.TIM_Prescaler = 7199; 00134 TIM_TimeBaseStructure.TIM_ClockDivision = TIM_CKD_DIV2; 00135 TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up; 00136 TIM_TimeBaseInit(TIM2, &TIM_TimeBaseStructure); 00137 00138 TIM_ARRPreloadConfig(TIM2, ENABLE); 00139 TIM_Cmd(TIM2, ENABLE); 00140 } 00141 00142 void Timer3_Init(void) 00143 { 00144 // Time base configuration Timer3 clock 00145 TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure; 00146 TIM_TimeBaseStructure.TIM_Period = 10; 00147 TIM_TimeBaseStructure.TIM_Prescaler = 7199; 00148 TIM_TimeBaseStructure.TIM_ClockDivision = TIM_CKD_DIV2; 00149 TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up; 00150 TIM_TimeBaseInit(TIM3, &TIM_TimeBaseStructure); 00151 00152 TIM_ARRPreloadConfig(TIM3, ENABLE); 00153 TIM_ClearITPendingBit(TIM3, TIM_IT_Update); 00154 TIM_ITConfig(TIM3, TIM_IT_Update, ENABLE); 00155 TIM_Cmd(TIM3, ENABLE); 00156 } 00157 00158 void Timer2_DeInit() 00159 { 00160 TIM_Cmd(TIM2, DISABLE); 00161 TIM_DeInit(TIM2); 00162 RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM2, DISABLE); 00163 } 00164 00165 void Timer3_DeInit(void) 00166 { 00167 TIM_Cmd(TIM3, DISABLE); 00168 TIM_ITConfig(TIM3, TIM_IT_Update, DISABLE); 00169 TIM_ARRPreloadConfig(TIM3, DISABLE); 00170 NVIC_DisableIRQ(TIM3_IRQn); 00171 TIM_DeInit(TIM3); 00172 RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM3, DISABLE); 00173 } 00174 00175 void SD_LowLevel_DeInit(void) 00176 { 00177 GPIO_InitTypeDef GPIO_InitStructure; 00178 00179 SPI_Cmd(SD_SPI, DISABLE); /*!< SD_SPI disable */ 00180 SPI_I2S_DeInit(SD_SPI); /*!< DeInitializes the SD_SPI */ 00181 00182 /*!< SD_SPI Periph clock disable */ 00183 RCC_APB2PeriphClockCmd(SD_SPI_CLK, DISABLE); 00184 00185 /*!< Configure SD_SPI pins: SCK */ 00186 GPIO_InitStructure.GPIO_Pin = SD_SPI_SCK_PIN; 00187 GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING; 00188 GPIO_Init(SD_SPI_SCK_GPIO_PORT, &GPIO_InitStructure); 00189 00190 /*!< Configure SD_SPI pins: MISO */ 00191 GPIO_InitStructure.GPIO_Pin = SD_SPI_MISO_PIN; 00192 GPIO_Init(SD_SPI_MISO_GPIO_PORT, &GPIO_InitStructure); 00193 00194 /*!< Configure SD_SPI pins: MOSI */ 00195 GPIO_InitStructure.GPIO_Pin = SD_SPI_MOSI_PIN; 00196 GPIO_Init(SD_SPI_MOSI_GPIO_PORT, &GPIO_InitStructure); 00197 00198 /*!< Configure SD_SPI_CS_PIN pin: SD Card CS pin */ 00199 GPIO_InitStructure.GPIO_Pin = SD_CS_PIN; 00200 GPIO_Init(SD_CS_GPIO_PORT, &GPIO_InitStructure); 00201 00202 /*!< Configure SD_SPI_DETECT_PIN pin: SD Card detect pin */ 00203 GPIO_InitStructure.GPIO_Pin = SD_DETECT_PIN; 00204 GPIO_Init(SD_DETECT_GPIO_PORT, &GPIO_InitStructure); 00205 } 00206 00207 00208 void SD_LowLevel_Init(void) 00209 { 00210 GPIO_InitTypeDef GPIO_InitStructure; 00211 SPI_InitTypeDef SPI_InitStructure; 00212 00213 /*!< SD_SPI_CS_GPIO, SD_SPI_MOSI_GPIO, SD_SPI_MISO_GPIO, SD_SPI_DETECT_GPIO 00214 and SD_SPI_SCK_GPIO Periph clock enable */ 00215 RCC_APB2PeriphClockCmd(SD_CS_GPIO_CLK | SD_SPI_MOSI_GPIO_CLK | SD_SPI_MISO_GPIO_CLK | 00216 SD_SPI_SCK_GPIO_CLK | SD_DETECT_GPIO_CLK, ENABLE); 00217 00218 /*!< SD_SPI Periph clock enable */ 00219 RCC_APB2PeriphClockCmd(SD_SPI_CLK, ENABLE); 00220 00221 /*!< Configure SD_SPI pins: SCK */ 00222 GPIO_InitStructure.GPIO_Pin = SD_SPI_SCK_PIN; 00223 GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz; 00224 GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP; 00225 GPIO_Init(SD_SPI_SCK_GPIO_PORT, &GPIO_InitStructure); 00226 00227 /*!< Configure SD_SPI pins: MOSI */ 00228 GPIO_InitStructure.GPIO_Pin = SD_SPI_MOSI_PIN; 00229 GPIO_Init(SD_SPI_MOSI_GPIO_PORT, &GPIO_InitStructure); 00230 00231 /*!< Configure SD_SPI pins: MISO */ 00232 GPIO_InitStructure.GPIO_Pin = SD_SPI_MISO_PIN; 00233 GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING; 00234 GPIO_Init(SD_SPI_MISO_GPIO_PORT, &GPIO_InitStructure); 00235 00236 /*!< Configure SD_SPI_CS_PIN pin: SD Card CS pin */ 00237 GPIO_InitStructure.GPIO_Pin = SD_CS_PIN; 00238 GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP; 00239 GPIO_Init(SD_CS_GPIO_PORT, &GPIO_InitStructure); 00240 00241 /*!< Configure SD_SPI_DETECT_PIN pin: SD Card detect pin */ 00242 GPIO_InitStructure.GPIO_Pin = SD_DETECT_PIN; 00243 GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU; 00244 GPIO_Init(SD_DETECT_GPIO_PORT, &GPIO_InitStructure); 00245 00246 /*!< SD_SPI Config */ 00247 SPI_InitStructure.SPI_Direction = SPI_Direction_2Lines_FullDuplex; 00248 SPI_InitStructure.SPI_Mode = SPI_Mode_Master; 00249 SPI_InitStructure.SPI_DataSize = SPI_DataSize_8b; 00250 SPI_InitStructure.SPI_CPOL = SPI_CPOL_High; 00251 SPI_InitStructure.SPI_CPHA = SPI_CPHA_2Edge; 00252 SPI_InitStructure.SPI_NSS = SPI_NSS_Soft; 00253 SPI_InitStructure.SPI_BaudRatePrescaler = SPI_BaudRatePrescaler_2; 00254 00255 SPI_InitStructure.SPI_FirstBit = SPI_FirstBit_MSB; 00256 SPI_InitStructure.SPI_CRCPolynomial = 7; 00257 SPI_Init(SD_SPI, &SPI_InitStructure); 00258 00259 SPI_Cmd(SD_SPI, ENABLE); /*!< SD_SPI enable */ 00260 } 00261 00262 #ifdef USE_FULL_ASSERT 00263 00264 /** 00265 * @brief Reports the name of the source file and the source line number 00266 * where the assert_param error has occurred. 00267 * @param file: pointer to the source file name 00268 * @param line: assert_param error line source number 00269 * @retval None 00270 */ 00271 void assert_failed(uint8_t* file, uint32_t line) 00272 { 00273 /* User can add his own implementation to report the file name and line number, 00274 ex: printf("Wrong parameters value: file %s on line %d\r\n", file, line) */ 00275 00276 /* Infinite loop */ 00277 while (1) 00278 { 00279 } 00280 } 00281 #endif 00282 00283 /* 00284 * Minimal __assert_func used by the assert() macro 00285 * */ 00286 void __assert_func(const char *file, int line, const char *func, const char *failedexpr) 00287 { 00288 while(1) 00289 {} 00290 } 00291 00292 /* 00293 * Minimal __assert() uses __assert__func() 00294 * */ 00295 void __assert(const char *file, int line, const char *failedexpr) 00296 { 00297 __assert_func (file, line, NULL, failedexpr); 00298 } 00299 00300 #ifdef USE_SEE 00301 #ifndef USE_DEFAULT_TIMEOUT_CALLBACK 00302 /** 00303 * @brief Basic management of the timeout situation. 00304 * @param None. 00305 * @retval sEE_FAIL. 00306 */ 00307 uint32_t sEE_TIMEOUT_UserCallback(void) 00308 { 00309 /* Return with error code */ 00310 return sEE_FAIL; 00311 } 00312 #endif 00313 #endif /* USE_SEE */
Generated on Fri Jul 15 2022 02:02:47 by
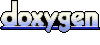