
with gcc-arm-none-eabi-7-2017-q4-major the default typedef of time_t is "long long int". older releases typedef time_t as "long int". mbed lib cannot handle this correctly This applies to exported projects as "GCC (ARM-Embedded)"
Fork of USBSerial_HelloWorld by
main.cpp
00001 #include "mbed.h" 00002 00003 #define SERIAL_TX PA_9 00004 #define SERIAL_RX PA_10 00005 Serial pc(SERIAL_TX, SERIAL_RX, 115200); 00006 00007 int main(void) 00008 { 00009 time_t t; 00010 struct tm *tx; 00011 00012 pc.printf("++++++++++++++++++++++\nsizeof(time_t) = %d\n++++++++++++++++++++++\n",sizeof(time_t)); 00013 00014 time(&t); 00015 pc.printf("read RTC: %u\n",(int)t); 00016 tx = localtime(&t); 00017 pc.printf("ctime: %s", ctime(&t)); 00018 pc.printf("T: %d:%d:%d D: %d.%d.%d X: %d\n", 00019 tx->tm_hour,tx->tm_min,tx->tm_sec, 00020 tx->tm_mday,tx->tm_mon+1,tx->tm_year+1900, 00021 tx->tm_isdst); 00022 00023 pc.printf("t = 0\n"); 00024 t = 0; 00025 tx = localtime(&t); 00026 pc.printf("ctime: %s", ctime(&t)); 00027 pc.printf("T: %d:%d:%d D: %d.%d.%d X: %d\n", 00028 tx->tm_hour,tx->tm_min,tx->tm_sec, 00029 tx->tm_mday,tx->tm_mon+1,tx->tm_year+1900, 00030 tx->tm_isdst); 00031 00032 pc.printf("loop start\n"); 00033 while (1) { 00034 tx = localtime(&t); 00035 pc.printf("ctime: %s", ctime(&t)); 00036 pc.printf("T: %d:%d:%d D: %d.%d.%d X: %d\n", 00037 tx->tm_hour,tx->tm_min,tx->tm_sec, 00038 tx->tm_mday,tx->tm_mon+1,tx->tm_year+1900, 00039 tx->tm_isdst); 00040 wait(1); 00041 t += 1; 00042 } 00043 }
Generated on Fri Jul 15 2022 02:06:47 by
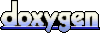