SB
Fork of MMA8451Q by
Embed:
(wiki syntax)
Show/hide line numbers
MMA8451Q.h
00001 /* Copyright (c) 2010-2011 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #ifndef MMA8451Q_H 00020 #define MMA8451Q_H 00021 #define MMA8415Q_SAMPLE800 0x00 00022 #define MMA8415Q_SAMPLE400 0x08 00023 #define MMA8415Q_SAMPLE200 0x10 00024 #define MMA8415Q_SAMPLE100 0x18 00025 #define MMA8415Q_SAMPLE50 0x20 00026 #define MMA8415Q_SAMPLE12_5 0x28 00027 #define MMA8415Q_SAMPLE6_25 0x30 00028 #define MMA8415Q_SAMPLE1_56 0x38 00029 00030 #include "mbed.h" 00031 00032 /** 00033 * MMA8451Q accelerometer example 00034 * 00035 * @code 00036 * #include "mbed.h" 00037 * #include "MMA8451Q.h" 00038 * 00039 * #define MMA8451_I2C_ADDRESS (0x1d<<1) 00040 * 00041 * int main(void) { 00042 * 00043 * MMA8451Q acc(P_E25, P_E24, MMA8451_I2C_ADDRESS); 00044 * PwmOut rled(LED_RED); 00045 * PwmOut gled(LED_GREEN); 00046 * PwmOut bled(LED_BLUE); 00047 * 00048 * while (true) { 00049 * rled = 1.0 - abs(acc.getAccX()); 00050 * gled = 1.0 - abs(acc.getAccY()); 00051 * bled = 1.0 - abs(acc.getAccZ()); 00052 * wait(0.1); 00053 * } 00054 * } 00055 * @endcode 00056 */ 00057 class MMA8451Q 00058 { 00059 public: 00060 /** 00061 * MMA8451Q constructor 00062 * 00063 * @param sda SDA pin 00064 * @param sdl SCL pin 00065 * @param addr addr of the I2C peripheral 00066 */ 00067 MMA8451Q(PinName sda, PinName scl, int addr); 00068 00069 /** 00070 * MMA8451Q destructor 00071 */ 00072 ~MMA8451Q(); 00073 00074 /** 00075 * Get the value of the WHO_AM_I register 00076 * 00077 * @returns WHO_AM_I value 00078 */ 00079 uint8_t getWhoAmI(); 00080 00081 /** 00082 * Get X axis acceleration 00083 * 00084 * @returns X axis acceleration 00085 */ 00086 float getAccX(); 00087 00088 /** 00089 * Get Y axis acceleration 00090 * 00091 * @returns Y axis acceleration 00092 */ 00093 float getAccY(); 00094 00095 /** 00096 * Get Z axis acceleration 00097 * 00098 * @returns Z axis acceleration 00099 */ 00100 float getAccZ(); 00101 00102 /** 00103 * Get XYZ axis acceleration 00104 * 00105 * @param res array where acceleration data will be stored 00106 */ 00107 void getAccAllAxis(float * res); 00108 00109 /** JK 00110 * Setup Double Tap detection 00111 00112 00113 Example: 00114 00115 #include "mbed.h" 00116 #include "MMA8451Q.h" 00117 00118 #define MMA8451_I2C_ADDRESS (0x1d<<1) 00119 #define ON 0 00120 #define OFF !ON 00121 00122 //Setup the interrupts for the MMA8451Q 00123 InterruptIn accInt1(PTA14); 00124 InterruptIn accInt2(PTA15);//not used in this prog but this is the other int from the accelorometer 00125 00126 uint8_t togstat=0;//Led status 00127 DigitalOut bled(LED_BLUE); 00128 00129 00130 void tapTrue(void){//ISR 00131 if(togstat == 0){ 00132 togstat = 1; 00133 bled=ON; 00134 } else { 00135 togstat = 0; 00136 bled=OFF; 00137 } 00138 00139 } 00140 00141 00142 int main(void) { 00143 00144 MMA8451Q acc(PTE25, PTE24, MMA8451_I2C_ADDRESS);//accelorometer instance 00145 00146 acc.setDoubleTap();//Setup the MMA8451Q to look for a double Tap 00147 accInt1.rise(&tapTrue);//call tapTrue when an interrupt is generated on PTA14 00148 00149 while (true) { 00150 //Interrupt driven so nothing in main loop 00151 } 00152 } 00153 00154 00155 */ 00156 void setDoubleTap(void); 00157 void Overwrite_dr(float dateRate); 00158 void Setdr(float dateRate); 00159 private: 00160 I2C m_i2c; 00161 int m_addr; 00162 void readRegs(int addr, uint8_t * data, int len); 00163 void writeRegs(uint8_t * data, int len); 00164 int16_t getAccAxis(uint8_t addr); 00165 char dr; 00166 00167 }; 00168 00169 #endif
Generated on Sun Jul 17 2022 05:33:13 by
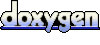