forking microbit-dal
Dependencies: BLE_API mbed-dev-bin nRF51822-bluetooth-mdw
Fork of microbit-dal by
MicroBitAnimationService.cpp
00001 /* 00002 The MIT License (MIT) 00003 00004 Copyright (c) 2016 British Broadcasting Corporation. 00005 This software is provided by Lancaster University by arrangement with the BBC. 00006 00007 Permission is hereby granted, free of charge, to any person obtaining a 00008 copy of this software and associated documentation files (the "Software"), 00009 to deal in the Software without restriction, including without limitation 00010 the rights to use, copy, modify, merge, publish, distribute, sublicense, 00011 and/or sell copies of the Software, and to permit persons to whom the 00012 Software is furnished to do so, subject to the following conditions: 00013 00014 The above copyright notice and this permission notice shall be included in 00015 all copies or substantial portions of the Software. 00016 00017 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00018 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00019 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO Animation SHALL 00020 THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00021 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING 00022 FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER 00023 DEALINGS IN THE SOFTWARE. 00024 */ 00025 00026 /** 00027 * Class definition for a MicroBit BLE Animation Service. 00028 * Provides a BLE gateway onto an Animation Model. 00029 */ 00030 00031 #include "MicroBitConfig.h" 00032 #include "MicroBitAnimationService.h" 00033 #include "ble/UUID.h" 00034 #include "Animator.h" 00035 00036 /** 00037 * Constructor. 00038 * Create a representation of the AnimationService 00039 * @param _ble The instance of a BLE device that we're running on. 00040 */ 00041 MicroBitAnimationService::MicroBitAnimationService(BLEDevice &_ble) : 00042 ble(_ble) 00043 { 00044 // TODO: Three GattCharacteristic objects. UUID, valuePTR, length, max length, properties. 00045 GattCharacteristic animationTypeCharacteristic( 00046 MicroBitAnimationServiceAnimationTypeCharacteristicUUID, 00047 (uint8_t *)animation_type_buffer, 00048 1, 00049 1, 00050 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE); 00051 00052 GattCharacteristic animationStatusCharacteristic( 00053 MicroBitAnimationServiceAnimationStatusCharacteristicUUID, 00054 (uint8_t *)animation_status_buffer, 00055 1, 00056 1, 00057 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY); 00058 00059 GattCharacteristic animationControlCharacteristic( 00060 MicroBitAnimationServiceAnimationControlCharacteristicUUID, 00061 (uint8_t *)animation_control_buffer, 00062 1, 00063 1, 00064 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE); 00065 00066 // TODO: initialise characteristic value buffers 00067 animation_type_buffer[0] = 0x00; 00068 animation_status_buffer[0] = 0x00; 00069 animation_control_buffer[0] = 0x00; 00070 00071 // Set default security requirements - optional 00072 // animationTypeCharacteristic.requireSecurity(SecurityManager::MICROBIT_BLE_SECURITY_LEVEL); 00073 // animationStatusCharacteristic.requireSecurity(SecurityManager::MICROBIT_BLE_SECURITY_LEVEL); 00074 // animationControlCharacteristic.requireSecurity(SecurityManager::MICROBIT_BLE_SECURITY_LEVEL); 00075 00076 // TODO: create GattService containing the three characteristics 00077 // create an array of our characteristics so we can pass them to the service when we create it 00078 GattCharacteristic *characteristics[] = {&animationTypeCharacteristic, &animationStatusCharacteristic, &animationControlCharacteristic}; 00079 00080 // create the animation service and specify its characteristics. 3rd arg is the number of characteristics. 00081 GattService service(MicroBitAnimationServiceUUID, characteristics, sizeof(characteristics) / sizeof(GattCharacteristic *)); 00082 00083 00084 // TODO: add the service to the Bluetooth attribute table 00085 ble.addService(service); 00086 00087 // TODO: take a note of the handles of our new characteristics now that they're in the attribute table 00088 animationTypeCharacteristicHandle = animationTypeCharacteristic.getValueHandle(); 00089 animationStatusCharacteristicHandle = animationStatusCharacteristic.getValueHandle(); 00090 animationControlCharacteristicHandle = animationControlCharacteristic.getValueHandle(); 00091 00092 // TODO: register a callback function for when a characteristic is written to 00093 ble.onDataWritten(this, &MicroBitAnimationService::onDataWritten); 00094 00095 // subscribe to animation status events so they can be notified to the connected client 00096 if (EventModel::defaultEventBus) 00097 EventModel::defaultEventBus->listen(ANIMATION_STATUS_EVENT, MICROBIT_EVT_ANY, this, &MicroBitAnimationService::animationStatusUpdate, MESSAGE_BUS_LISTENER_IMMEDIATE); 00098 } 00099 00100 00101 /** 00102 * Callback. Invoked when any of our attributes are written to 00103 */ 00104 void MicroBitAnimationService::onDataWritten(const GattWriteCallbackParams *params) 00105 { 00106 00107 // TODO: handle write to animation type characteristic 00108 if (params->handle == animationTypeCharacteristicHandle && params->len == 1) { 00109 uint8_t *value = (uint8_t *)params->data; 00110 uint16_t event_value = static_cast<uint16_t>(value[0]); 00111 MicroBitEvent evt(ANIMATION_TYPE_EVENT, event_value); 00112 return; 00113 } 00114 00115 // TODO: handle write to animation control characteristic 00116 if (params->handle == animationControlCharacteristicHandle && params->len == 1) { 00117 uint8_t *value = (uint8_t *)params->data; 00118 uint16_t event_value = static_cast<uint16_t>(value[0]); 00119 MicroBitEvent evt(ANIMATION_CONTROL_EVENT, event_value); 00120 return; 00121 } 00122 } 00123 00124 void MicroBitAnimationService::animationStatusUpdate(MicroBitEvent e) 00125 { 00126 // TODO: notify connected client of change to the animation status characteristic 00127 if (ble.getGapState().connected) 00128 { 00129 animation_status_buffer[0] = e.value; 00130 ble.gattServer().notify(animationStatusCharacteristicHandle,(uint8_t *)animation_status_buffer, 1); 00131 } 00132 } 00133 00134 const uint8_t MicroBitAnimationServiceUUID[] = { 00135 0xe9,0x5d,0x71,0x70,0x25,0x1d,0x47,0x0a,0xa0,0x62,0xfa,0x19,0x22,0xdf,0xa9,0xa8 00136 }; 00137 00138 const uint8_t MicroBitAnimationServiceAnimationTypeCharacteristicUUID[] = { 00139 0xe9,0x5d,0xC3,0x06,0x25,0x1d,0x47,0x0a,0xa0,0x62,0xfa,0x19,0x22,0xdf,0xa9,0xa8 00140 }; 00141 00142 const uint8_t MicroBitAnimationServiceAnimationStatusCharacteristicUUID[] = { 00143 0xe9,0x5d,0x45,0x92,0x25,0x1d,0x47,0x0a,0xa0,0x62,0xfa,0x19,0x22,0xdf,0xa9,0xa8 00144 }; 00145 00146 const uint8_t MicroBitAnimationServiceAnimationControlCharacteristicUUID[] = { 00147 0xe9,0x5d,0xb8,0x4c,0x25,0x1d,0x47,0x0a,0xa0,0x62,0xfa,0x19,0x22,0xdf,0xa9,0xa8 00148 }; 00149
Generated on Tue Jul 12 2022 19:14:05 by
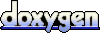