
2-wheel Inverted Pendulum control program
Dependencies: AsyncSerial Lib_DFPlayerMini Lib_MPU9250_SPI mbed
filter_func.h
00001 #ifndef __FILTER_FUNCTIONS_H__ 00002 #define __FILTER_FUNCTIONS_H__ 00003 00004 #include <math.h> 00005 #include "mbed.h" 00006 00007 typedef enum _BIQUADTYPE{ 00008 FILTER_LPF = 1, 00009 FILTER_HPF = 2, 00010 FILTER_BPF = 3, 00011 FILTER_NF = 4 00012 } BIQUADTYPE; 00013 00014 typedef struct _STCOMPFILTER{ 00015 float _int; 00016 float _adj; 00017 float _adj_temp; 00018 float _param; 00019 } STCOMPFILTER; 00020 00021 typedef struct _STBIQUADFILTER{ 00022 float in[2]; // input 00023 float out[2]; // output 00024 float a[3]; // coefficient A 00025 float b[3]; // coefficient B 00026 } STBIQUADFILTER; 00027 00028 // complimentary filter 00029 void init_comp_filter( STCOMPFILTER* filter, float param ); 00030 void proc_comp_filter( STCOMPFILTER* filter, float dt, float angle_raw, float angle_vec_raw, float* angle, float* angle_vec ); 00031 00032 // BiQuad filter 00033 void init_biquad_filter( STBIQUADFILTER* filter, float freq_sample, float freq, float param, BIQUADTYPE filter_pattern ); 00034 float proc_biquad_filter( STBIQUADFILTER* filter, float input ); 00035 00036 #endif
Generated on Sat Jul 16 2022 06:27:20 by
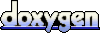