
Twitter client, with TextLCD, Ethernet Connection, and USB Host for keyboard.
Dependencies: EthernetNetIf TextLCD mbed
main.cpp
00001 /* 00002 Copyright (c) 2011 blmarket.net 00003 */ 00004 /* 00005 Copyright (c) 2010 Peter Barrett 00006 00007 Permission is hereby granted, free of charge, to any person obtaining a copy 00008 of this software and associated documentation files (the "Software"), to deal 00009 in the Software without restriction, including without limitation the rights 00010 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00011 copies of the Software, and to permit persons to whom the Software is 00012 furnished to do so, subject to the following conditions: 00013 00014 The above copyright notice and this permission notice shall be included in 00015 all copies or substantial portions of the Software. 00016 00017 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00018 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00019 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00020 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00021 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00022 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00023 THE SOFTWARE. 00024 */ 00025 00026 #include "mbed.h" 00027 #include "USBHost.h" 00028 #include "Utils.h" 00029 #include "FATFileSystem.h" 00030 #include "EthernetNetIf.h" 00031 #include "HTTPClient.h" 00032 #include "TextLCD.h" 00033 00034 Serial pc(USBTX, USBRX); 00035 TextLCD lcd(p21, p23, p22, p27, p28, p29, p30); // rs, rw, e, d0-d3 00036 EthernetNetIf eth( 00037 IpAddr(110,76,72,244), //IP Address 00038 IpAddr(255,255,255,0), //Network Mask 00039 IpAddr(110,76,72,1), //Gateway 00040 IpAddr(143,248,1,177) //DNS 00041 ); 00042 HTTPClient *twitterclient = NULL; // will be set by initialization func. 00043 00044 u8 prevkey[8] = {0}; 00045 char keyMap[256] = {0,0,0,0,'a','b','c','d','e','f','g','h','i','j','k','l','m','n','o','p','q','r','s','t','u','v','w','x','y','z',0,0,0,0,0,0,0,0,0,0,0/*return*/,0,0,0,' '}; 00046 00047 int msglen = 0; 00048 char msgtxt[256]; 00049 bool needcls = false; 00050 00051 void sendTweet(void) 00052 { 00053 if(msglen == 0) return; 00054 if(twitterclient == NULL) return; 00055 HTTPMap msg; 00056 msg["status"] = msgtxt; 00057 00058 twitterclient->basicAuth("blmarketmbed", "1324"); //We use basic authentication, replace with you account's parameters 00059 00060 //No need to retieve data sent back by the server 00061 HTTPResult r = twitterclient->post("http://api.supertweet.net/1/statuses/update.xml", msg, NULL); 00062 if( r == HTTP_OK ) 00063 { 00064 printf("Tweet sent with success!\n"); 00065 lcd.cls(); 00066 lcd.printf("Tweet success!\nType Tweet msg\n"); 00067 } 00068 else 00069 { 00070 printf("Problem during tweeting, return code %d\n", r); 00071 lcd.cls(); 00072 lcd.printf("Tweet failure\n"); 00073 } 00074 needcls = true; 00075 00076 msglen = 0; 00077 msgtxt[msglen]=0; 00078 } 00079 00080 void onPressedKey(u8 value) 00081 { 00082 if(value == 40) 00083 { 00084 sendTweet(); 00085 return; 00086 } 00087 00088 if(keyMap[value] != 0) 00089 { 00090 if(msglen >= 32) return; 00091 if(needcls) 00092 { 00093 lcd.cls(); 00094 needcls = false; 00095 } 00096 lcd.printf("%c",keyMap[value]); 00097 msgtxt[msglen++] = keyMap[value]; 00098 msgtxt[msglen] = 0; 00099 } 00100 else 00101 { 00102 printf("Unknown keycode %d\n",value); 00103 } 00104 } 00105 00106 void HandleKeyboardCallback(u8 *data, int len) 00107 { 00108 if(len != 8) 00109 { 00110 printf("Wrong KeyboardCallback : len should be 8\n"); 00111 return; 00112 } 00113 00114 for(int i=2;i<len;i++) if(data[i] != 0) 00115 { 00116 bool omit = false; 00117 for(int j=2;j<len;j++) 00118 { 00119 if(prevkey[j] == data[i]) 00120 { 00121 omit = true; 00122 break; 00123 } 00124 } 00125 if(omit) continue; 00126 onPressedKey(data[i]); 00127 } 00128 memcpy(prevkey, data, sizeof(u8)*len); 00129 } 00130 00131 int main() 00132 { 00133 printf("Init\n"); 00134 lcd.printf("TwitterClient\nLoading...\n"); 00135 00136 EthernetErr ethErr = eth.setup(); 00137 if(ethErr) 00138 { 00139 printf("Error %d in setup.\n", ethErr); 00140 return -1; 00141 } 00142 printf("\r\nSetup OK\r\n"); 00143 00144 pc.baud(9600); 00145 USBInit(); 00146 00147 twitterclient = new HTTPClient(); 00148 00149 lcd.cls(); 00150 lcd.printf("Type Tweet msg\n"); 00151 needcls = true; 00152 00153 while(1) 00154 { 00155 USBLoop(); 00156 } 00157 00158 /* 00159 printf("\r\nSetting up...\r\n"); 00160 00161 HTTPClient twitter; 00162 00163 HTTPMap msg; 00164 msg["status"] = "Now I need to attach my USB keyboard to mbed"; //A good example of Key/Value pair use with Web APIs 00165 00166 twitter.basicAuth("blmarketmbed", "1324"); //We use basic authentication, replace with you account's parameters 00167 00168 //No need to retieve data sent back by the server 00169 HTTPResult r = twitter.post("http://api.supertweet.net/1/statuses/update.xml", msg, NULL); 00170 if( r == HTTP_OK ) 00171 { 00172 printf("Tweet sent with success!\n"); 00173 lcd.printf("Tweet success!\n"); 00174 } 00175 else 00176 { 00177 printf("Problem during tweeting, return code %d\n", r); 00178 lcd.printf("Tweet failure\n"); 00179 } 00180 */ 00181 00182 return 0; 00183 } 00184
Generated on Wed Jul 13 2022 00:18:19 by
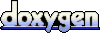