
Twitter client, with TextLCD, Ethernet Connection, and USB Host for keyboard.
Dependencies: EthernetNetIf TextLCD mbed
AutoEvents.cpp
00001 00002 /* 00003 Copyright (c) 2010 Peter Barrett 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #include "mbed.h" 00025 #include "USBHost.h" 00026 #include "Utils.h" 00027 00028 #define AUTOEVT(_class,_subclass,_protocol) (((_class) << 16) | ((_subclass) << 8) | _protocol) 00029 #define AUTO_KEYBOARD AUTOEVT(CLASS_HID,1,1) 00030 #define AUTO_MOUSE AUTOEVT(CLASS_HID,1,2) 00031 00032 u8 auto_mouse[4]; // buttons,dx,dy,scroll 00033 u8 auto_keyboard[8]; // modifiers,reserved,keycode1..keycode6 00034 u8 auto_joystick[4]; // x,y,buttons,throttle 00035 00036 void HandleKeyboardCallback(u8 *data, int len); // defined in main.cpp 00037 00038 void AutoEventCallback(int device, int endpoint, int status, u8* data, int len, void* userData) 00039 { 00040 int evt = (int)userData; 00041 switch (evt) 00042 { 00043 case AUTO_KEYBOARD: 00044 printf("AUTO_KEYBOARD "); 00045 HandleKeyboardCallback(data, len); 00046 break; 00047 case AUTO_MOUSE: 00048 printf("AUTO_MOUSE "); 00049 break; 00050 default: 00051 printf("HUH "); 00052 } 00053 printfBytes("data",data,len); 00054 USBInterruptTransfer(device,endpoint,data,len,AutoEventCallback,userData); 00055 } 00056 00057 // Establish transfers for interrupt events 00058 void AddAutoEvent(int device, InterfaceDescriptor* id, EndpointDescriptor* ed) 00059 { 00060 if ((ed->bmAttributes & 3) != ENDPOINT_INTERRUPT || !(ed->bEndpointAddress & 0x80)) 00061 return; 00062 00063 // Make automatic interrupt enpoints for known devices 00064 u32 evt = AUTOEVT(id->bInterfaceClass,id->bInterfaceSubClass,id->bInterfaceProtocol); 00065 u8* dst = 0; 00066 int len; 00067 switch (evt) 00068 { 00069 case AUTO_MOUSE: 00070 dst = auto_mouse; 00071 len = sizeof(auto_mouse); 00072 break; 00073 case AUTO_KEYBOARD: 00074 dst = auto_keyboard; 00075 len = sizeof(auto_keyboard); 00076 break; 00077 default: 00078 printf("Interrupt endpoint %02X %08X\n",ed->bEndpointAddress,evt); 00079 break; 00080 } 00081 if (dst) 00082 { 00083 printf("Auto Event for %02X %08X\n",ed->bEndpointAddress,evt); 00084 USBInterruptTransfer(device,ed->bEndpointAddress,dst,len,AutoEventCallback,(void*)evt); 00085 } 00086 } 00087 00088 void PrintString(int device, int i) 00089 { 00090 u8 buffer[256]; 00091 int le = GetDescriptor(device,DESCRIPTOR_TYPE_STRING,i,buffer,255); 00092 if (le < 0) 00093 return; 00094 char* dst = (char*)buffer; 00095 for (int j = 2; j < le; j += 2) 00096 *dst++ = buffer[j]; 00097 *dst = 0; 00098 printf("%d:%s\n",i,(const char*)buffer); 00099 } 00100 00101 // Walk descriptors and create endpoints for a given device 00102 int StartAutoEvent(int device, int configuration, int interfaceNumber) 00103 { 00104 u8 buffer[255]; 00105 int err = GetDescriptor(device,DESCRIPTOR_TYPE_CONFIGURATION,0,buffer,255); 00106 if (err < 0) 00107 return err; 00108 00109 int len = buffer[2] | (buffer[3] << 8); 00110 u8* d = buffer; 00111 u8* end = d + len; 00112 while (d < end) 00113 { 00114 if (d[1] == DESCRIPTOR_TYPE_INTERFACE) 00115 { 00116 InterfaceDescriptor* id = (InterfaceDescriptor*)d; 00117 if (id->bInterfaceNumber == interfaceNumber) 00118 { 00119 d += d[0]; 00120 while (d < end && d[1] != DESCRIPTOR_TYPE_INTERFACE) 00121 { 00122 if (d[1] == DESCRIPTOR_TYPE_ENDPOINT) 00123 AddAutoEvent(device,id,(EndpointDescriptor*)d); 00124 d += d[0]; 00125 } 00126 } 00127 } 00128 d += d[0]; 00129 } 00130 return 0; 00131 } 00132 00133 void OnLoadDevice(int device, DeviceDescriptor* deviceDesc, InterfaceDescriptor* interfaceDesc) 00134 { 00135 printf("LoadDevice %d %02X:%02X:%02X\n",device,interfaceDesc->bInterfaceClass,interfaceDesc->bInterfaceSubClass,interfaceDesc->bInterfaceProtocol); 00136 char s[128]; 00137 for (int i = 1; i < 3; i++) 00138 { 00139 if (GetString(device,i,s,sizeof(s)) < 0) 00140 break; 00141 printf("%d: %s\n",i,s); 00142 } 00143 00144 switch (interfaceDesc->bInterfaceClass) 00145 { 00146 case CLASS_MASS_STORAGE: 00147 break; 00148 default: 00149 StartAutoEvent(device,1,0); 00150 break; 00151 } 00152 }
Generated on Wed Jul 13 2022 00:18:19 by
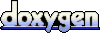