for Arduino TFT LCD Screen 160x128
Dependents: TFTLCDSCREEN Pong_ILI9163C
Fork of TFT_ILI9163C by
TFT_ILI9163C_F411RE_SOFT.cpp
00001 #include "TFT_ILI9163C.h" 00002 #if defined(__F411RE_SOFT__) 00003 00004 /** 00005 * TFT_ILI9163C library for ST Nucleo F411RE SOFT 00006 * 00007 * @author Copyright (c) 2014, .S.U.M.O.T.O.Y., coded by Max MC Costa 00008 * https://github.com/sumotoy/TFT_ILI9163C 00009 * 00010 * @author modified by masuda, Masuda Naika 00011 */ 00012 00013 //Serial pc(SERIAL_TX, SERIAL_RX); 00014 00015 //constructors 00016 TFT_ILI9163C::TFT_ILI9163C(PinName mosi, PinName miso, PinName sclk, PinName cs, PinName dc, PinName reset) 00017 : TFT_ILI9163C_BASE(mosi, miso, sclk, cs, dc, reset) { 00018 00019 _resetPinName = reset; 00020 init(cs, dc); 00021 } 00022 00023 TFT_ILI9163C::TFT_ILI9163C(PinName mosi, PinName miso, PinName sclk, PinName cs, PinName dc) 00024 : TFT_ILI9163C_BASE(mosi, miso, sclk, cs, dc) { 00025 00026 _resetPinName = NC; 00027 init(cs, dc); 00028 } 00029 00030 void TFT_ILI9163C::init(PinName cs, PinName dc){ 00031 00032 SPI_TypeDef *spi_ptr = (SPI_TypeDef*) _spi.spi; 00033 00034 uint32_t cs_port_index = (uint32_t) cs >> 4; 00035 uint32_t dc_port_index = (uint32_t) dc >> 4; 00036 00037 //set cs/dc port addresses and masks 00038 cs_port_reg = (GPIO_TypeDef *) (GPIOA_BASE + (cs_port_index << 10)); 00039 cs_reg_mask = 1 << ((uint32_t) cs & 0xf); 00040 dc_port_reg = (GPIO_TypeDef *) (GPIOA_BASE + (dc_port_index << 10)); 00041 dc_reg_mask = 1 << ((uint32_t) dc & 0xf); 00042 00043 // set bit band addresses 00044 // GPIO_TypeDef *cs_port_reg = (GPIO_TypeDef *) (GPIOA_BASE + (cs_port_index << 10)); 00045 // GPIO_TypeDef *dc_port_reg = (GPIO_TypeDef *) (GPIOA_BASE + (dc_port_index << 10)); 00046 // uint8_t cs_port_bit = (uint32_t) cs & 0xf; 00047 // uint8_t dc_port_bit = (uint32_t) dc & 0xf; 00048 // bb_cs_port = BITBAND_PERIPH(&cs_port_reg->ODR, cs_port_bit); 00049 // bb_dc_port = BITBAND_PERIPH(&dc_port_reg->ODR, dc_port_bit); 00050 00051 bb_spi_txe = BITBAND_PERIPH(&spi_ptr->SR, MASK_TO_BITNUM(SPI_SR_TXE)); 00052 bb_spi_bsy = BITBAND_PERIPH(&spi_ptr->SR, MASK_TO_BITNUM(SPI_SR_BSY)); 00053 bb_spi_spe = BITBAND_PERIPH(&spi_ptr->CR1, MASK_TO_BITNUM(SPI_CR1_SPE)); 00054 bb_spi_dff = BITBAND_PERIPH(&spi_ptr->CR1, MASK_TO_BITNUM(SPI_CR1_DFF)); 00055 } 00056 00057 inline void TFT_ILI9163C::selectSlave() { 00058 // _cs = 0; // Use DigitalOut 00059 // *bb_cs_port = 0; // Use bit band 00060 cs_port_reg->BSRRH = cs_reg_mask; // Use BSRR register 00061 } 00062 00063 inline void TFT_ILI9163C::deselectSlave() { 00064 // _cs = 1; 00065 // *bb_cs_port = 1; 00066 cs_port_reg->BSRRL = cs_reg_mask; 00067 } 00068 00069 inline void TFT_ILI9163C::setCommandMode() { 00070 // _dc = 0; 00071 // *bb_dc_port = 0; 00072 dc_port_reg->BSRRH = dc_reg_mask; 00073 } 00074 00075 inline void TFT_ILI9163C::setDataMode() { 00076 // _dc = 1; 00077 // *bb_dc_port = 1; 00078 dc_port_reg->BSRRL = dc_reg_mask; 00079 } 00080 00081 inline void TFT_ILI9163C::waitSpiFree() { 00082 00083 // SPI_TypeDef *spi_ptr = (SPI_TypeDef*) _spi.spi; 00084 // while ((spi_ptr->SR & SPI_SR_TXE) == 0); 00085 // while ((spi_ptr->SR & SPI_SR_BSY) != 0); 00086 00087 while (*bb_spi_txe == 0); 00088 while (*bb_spi_bsy != 0); 00089 } 00090 00091 inline void TFT_ILI9163C::waitBufferFree() { 00092 00093 // SPI_TypeDef *spi_ptr = (SPI_TypeDef*) _spi.spi; 00094 // while ((spi_ptr->SR & SPI_SR_TXE) == 0); 00095 00096 while (*bb_spi_txe == 0); 00097 } 00098 00099 inline void TFT_ILI9163C::set8bitMode() { 00100 00101 // SPI_TypeDef *spi_ptr = (SPI_TypeDef*) _spi.spi; 00102 // spi_ptr->CR1 &= ~(SPI_CR1_SPE | SPI_CR1_DFF); 00103 // spi_ptr->CR1 |= SPI_CR1_SPE; 00104 00105 *bb_spi_spe = 0; 00106 *bb_spi_dff = 0; 00107 *bb_spi_spe = 1; 00108 } 00109 00110 inline void TFT_ILI9163C::set16bitMode() { 00111 00112 // SPI_TypeDef *spi_ptr = (SPI_TypeDef*) _spi.spi; 00113 // spi_ptr->CR1 &= ~SPI_CR1_SPE; 00114 // spi_ptr->CR1 |= (SPI_CR1_SPE | SPI_CR1_DFF); 00115 00116 *bb_spi_spe = 0; 00117 *bb_spi_dff = 1; 00118 *bb_spi_spe = 1; 00119 } 00120 00121 void TFT_ILI9163C::writecommand(uint8_t c){ 00122 00123 set8bitMode(); 00124 setCommandMode(); 00125 selectSlave(); 00126 00127 SPI_TypeDef *spi_ptr = (SPI_TypeDef*) _spi.spi; 00128 spi_ptr->DR = c; 00129 00130 waitSpiFree(); 00131 deselectSlave(); 00132 } 00133 00134 void TFT_ILI9163C::writedata(uint8_t c){ 00135 00136 set8bitMode(); 00137 setDataMode(); 00138 selectSlave(); 00139 00140 SPI_TypeDef *spi_ptr = (SPI_TypeDef*) _spi.spi; 00141 spi_ptr->DR = c; 00142 00143 waitSpiFree(); 00144 deselectSlave(); 00145 } 00146 00147 void TFT_ILI9163C::writedata16(uint16_t d){ 00148 00149 set16bitMode(); 00150 setDataMode(); 00151 selectSlave(); 00152 00153 SPI_TypeDef *spi_ptr = (SPI_TypeDef*) _spi.spi; 00154 spi_ptr->DR = d; 00155 00156 waitSpiFree(); 00157 deselectSlave(); 00158 } 00159 00160 00161 void TFT_ILI9163C::writedata32(uint16_t d1, uint16_t d2){ 00162 00163 set16bitMode(); 00164 setDataMode(); 00165 selectSlave(); 00166 00167 SPI_TypeDef *spi_ptr = (SPI_TypeDef*) _spi.spi; 00168 spi_ptr->DR = d1; 00169 waitBufferFree(); 00170 spi_ptr->DR = d2; 00171 00172 waitSpiFree(); 00173 deselectSlave(); 00174 } 00175 00176 // use software loop, fast enough :-) 00177 void TFT_ILI9163C::writedata16burst(uint16_t d, int32_t len) { 00178 00179 len = len < 0 ? -len : len; 00180 00181 if (len > 0) { 00182 set16bitMode(); 00183 setDataMode(); 00184 selectSlave(); 00185 00186 SPI_TypeDef *spi_ptr = (SPI_TypeDef*) _spi.spi; 00187 while (len--) { 00188 waitBufferFree(); 00189 spi_ptr->DR = d; 00190 } 00191 00192 waitSpiFree(); 00193 deselectSlave(); 00194 } 00195 } 00196 #endif
Generated on Wed Jul 13 2022 11:29:01 by
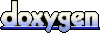