Library allowing up to 16 strings of 60 WS2811 or WS2812 LEDs to be driven from a single FRDM-KL25Z board. Uses hardware DMA to do a full 800 KHz rate without much CPU burden.
LedStrip.cpp
00001 #include <stdint.h> 00002 #include <cstring> 00003 00004 #include "LedStrip.h" 00005 00006 LedStrip::LedStrip(int n) 00007 { 00008 // Allocate 3 bytes per pixel: 00009 numLEDs = n; 00010 pixels = new uint8_t[ numPixelBytes() ]; 00011 if (pixels) { 00012 std::memset(pixels, 0x00, numPixelBytes()); // Init to RGB 'off' state 00013 } 00014 } 00015 00016 LedStrip::~LedStrip() 00017 { 00018 delete[] pixels; 00019 } 00020 00021 uint32_t LedStrip::total_luminance(void) 00022 { 00023 uint32_t running_total; 00024 running_total = 0; 00025 for (int i=0; i< numPixelBytes(); i++) 00026 running_total += pixels[i]; 00027 return running_total; 00028 } 00029 00030 // Convert R,G,B to combined 32-bit color 00031 uint32_t LedStrip::Color(uint8_t r, uint8_t g, uint8_t b) 00032 { 00033 // Take the lowest 7 bits of each value and append them end to end 00034 // We have the top bit set high (its a 'parity-like' bit in the protocol 00035 // and must be set!) 00036 return ((uint32_t)g << 16) | ((uint32_t)r << 8) | (uint32_t)b; 00037 } 00038 00039 // store the rgb component in our array 00040 void LedStrip::setPixelColor(uint16_t n, uint8_t r, uint8_t g, uint8_t b) 00041 { 00042 if (n >= numLEDs) return; // '>=' because arrays are 0-indexed 00043 00044 pixels[n*3 ] = g; 00045 pixels[n*3+1] = r; 00046 pixels[n*3+2] = b; 00047 } 00048 00049 void LedStrip::setPixelR(uint16_t n, uint8_t r) 00050 { 00051 if (n >= numLEDs) return; // '>=' because arrays are 0-indexed 00052 00053 pixels[n*3+1] = r; 00054 } 00055 00056 void LedStrip::setPixelG(uint16_t n, uint8_t g) 00057 { 00058 if (n >= numLEDs) return; // '>=' because arrays are 0-indexed 00059 00060 pixels[n*3] = g; 00061 } 00062 00063 void LedStrip::setPixelB(uint16_t n, uint8_t b) 00064 { 00065 if (n >= numLEDs) return; // '>=' because arrays are 0-indexed 00066 00067 pixels[n*3+2] = b; 00068 } 00069 00070 void LedStrip::setPackedPixels(uint8_t * buffer, uint32_t n) 00071 { 00072 if (n >= numLEDs) return; 00073 std::memcpy(pixels, buffer, (std::size_t) (n*3)); 00074 } 00075 00076 void LedStrip::setPixelColor(uint16_t n, uint32_t c) 00077 { 00078 if (n >= numLEDs) return; // '>=' because arrays are 0-indexed 00079 00080 pixels[n*3 ] = (c >> 16); 00081 pixels[n*3+1] = (c >> 8); 00082 pixels[n*3+2] = c; 00083 }
Generated on Wed Jul 13 2022 13:18:58 by
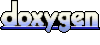