
Sender Mbed program for "Magician Mobile Robot Arm"
Embed:
(wiki syntax)
Show/hide line numbers
p4Send.cpp
00001 #include "mbed.h" 00002 #include "nRF24L01P.h" 00003 00004 Serial pc(USBTX, USBRX); // tx, rx 00005 00006 nRF24L01P NRFT(p5, p6, p7, p8, p9, p10); // mosi, miso, sck, csn, ce, irq 00007 00008 AnalogIn Vert(p19); 00009 AnalogIn Horz(p20); 00010 AnalogIn Lslide(p16); 00011 AnalogIn Rslide(p15); 00012 00013 PwmOut led1(LED1); 00014 PwmOut led2(LED2); 00015 PwmOut led3(LED3); 00016 PwmOut led4(LED4); 00017 00018 int main() { 00019 00020 // The nRF24L01+ supports transfers from 1 to 32 bytes, but Sparkfun's 00021 // "Nordic Serial Interface Board" (http://www.sparkfun.com/products/9019) 00022 // only handles 4 byte transfers in the ATMega code. 00023 #define TRANSFER_SIZE 17 00024 00025 char txData[TRANSFER_SIZE]; 00026 //int txDataCnt = 0; 00027 00028 NRFT.powerUp(); 00029 00030 // Display the (default) setup of the nRF24L01+ chip 00031 pc.printf( "nRF24L01+ Frequency : %d MHz\r\n", NRFT.getRfFrequency() ); 00032 pc.printf( "nRF24L01+ Output power : %d dBm\r\n", NRFT.getRfOutputPower() ); 00033 pc.printf( "nRF24L01+ Data Rate : %d kbps\r\n", NRFT.getAirDataRate() ); 00034 pc.printf( "nRF24L01+ TX Address : 0x%010llX\r\n", NRFT.getTxAddress() ); 00035 pc.printf( "nRF24L01+ RX Address : 0x%010llX\r\n", NRFT.getRxAddress() ); 00036 00037 pc.printf( "Type keys to test transfers:\r\n (transfers are grouped into %d characters)\r\n", TRANSFER_SIZE ); 00038 00039 NRFT.setTransferSize( TRANSFER_SIZE ); 00040 00041 NRFT.setTransmitMode(); 00042 NRFT.enable(); 00043 00044 while (1) { 00045 00046 /*// If we've received anything over the host serial link... 00047 if ( pc.readable() ) { 00048 00049 // ...add it to the transmit buffer 00050 txData[txDataCnt++] = pc.getc(); 00051 00052 // If the transmit buffer is full 00053 if ( txDataCnt >= sizeof( txData ) ) { 00054 00055 // Send the transmitbuffer via the nRF24L01+ 00056 NRFT.write( NRF24L01P_PIPE_P0, txData, txDataCnt ); 00057 00058 txDataCnt = 0; 00059 } 00060 00061 // Toggle LED1 (to help debug Host -> nRF24L01+ communication) 00062 myled1 = !myled1; 00063 }*/ 00064 int LSL,RSL,VER,HOR; 00065 00066 while(1) 00067 { 00068 //wait(0.1); 00069 00070 LSL = float(Lslide)*1000; 00071 txData[0] = LSL & 255; 00072 txData[1] = (LSL >> 8) & 255; 00073 txData[2] = (LSL >> 16) & 255; 00074 txData[3] = (LSL >> 24) & 255; 00075 led1 = Lslide; 00076 00077 VER = float(Vert)*1000; 00078 txData[4] = VER & 255; 00079 txData[5] = (VER >> 8) & 255; 00080 txData[6] = (VER >> 16) & 255; 00081 txData[7] = (VER >> 24) & 255; 00082 led2 = Vert; 00083 00084 HOR = float(Horz)*1000; 00085 txData[8] = HOR & 255; 00086 txData[9] = (HOR >> 8) & 255; 00087 txData[10] = (HOR >> 16) & 255; 00088 txData[11] = (HOR >> 24) & 255; 00089 led3 = Horz; 00090 00091 RSL = float(Rslide)*1000; 00092 txData[12] = RSL & 255; 00093 txData[13] = (RSL >> 8) & 255; 00094 txData[14] = (RSL >> 16) & 255; 00095 txData[15] = (RSL >> 24) & 255; 00096 led4 = Rslide; 00097 txData[16] = 'a'; 00098 00099 NRFT.write( NRF24L01P_PIPE_P0, txData, TRANSFER_SIZE ); 00100 //wait(0.1); 00101 } 00102 } 00103 }
Generated on Tue Jul 12 2022 20:50:58 by
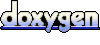