THEME AND FONT
Embed:
(wiki syntax)
Show/hide line numbers
lv_theme_rb.c
Go to the documentation of this file.
00001 /** 00002 * @file lv_theme_rb.c 00003 * 00004 */ 00005 00006 /********************* 00007 * INCLUDES 00008 *********************/ 00009 #include "lv_theme.h" 00010 00011 00012 /********************* 00013 * DEFINES 00014 *********************/ 00015 00016 /********************** 00017 * TYPEDEFS 00018 **********************/ 00019 00020 /********************** 00021 * STATIC PROTOTYPES 00022 **********************/ 00023 00024 /********************** 00025 * STATIC VARIABLES 00026 **********************/ 00027 static lv_theme_t theme; 00028 static lv_style_t def; 00029 static lv_style_t bg; 00030 static lv_style_t panel; 00031 static lv_style_t scr; 00032 00033 /*Static style definitions*/ 00034 static lv_style_t sb; 00035 static lv_style_t plain_bordered; 00036 static lv_style_t label_prim; 00037 static lv_style_t label_sec; 00038 static lv_style_t label_hint; 00039 00040 /*Saved input parameters*/ 00041 static uint16_t _hue; 00042 static lv_font_t * _font; 00043 00044 00045 static lv_style_t btn_rel, btn_pr, btn_trel, btn_tpr, btn_ina; 00046 00047 /********************** 00048 * MACROS 00049 **********************/ 00050 00051 /********************** 00052 * STATIC FUNCTIONS 00053 **********************/ 00054 00055 static void basic_init(void) 00056 { 00057 /* lv_style_copy(&def, &lv_style_pretty); //Initialize the default style 00058 00059 lv_style_copy(&scr, &def); 00060 scr.body.padding.bottom = 0; 00061 scr.body.padding.top = 0; 00062 scr.body.padding.left = 0; 00063 scr.body.padding.right = 0; 00064 00065 lv_style_copy(&sb, &lv_style_pretty_color); 00066 sb.body.grad_color = sb.body.main_color; 00067 sb.body.padding.right = sb.body.padding.right / 2; //Make closer to the edges 00068 sb.body.padding.bottom = sb.body.padding.bottom / 2; 00069 00070 lv_style_copy(&plain_bordered, &lv_style_plain); 00071 plain_bordered.body.border.width = 2; 00072 plain_bordered.body.border.color = lv_color_hex3(0xbbb); 00073 00074 theme.style.bg = &lv_style_plain; 00075 theme.style.scr = &scr; 00076 theme.style.panel = &lv_style_pretty;*/ 00077 00078 //GABOR: I copied the lv_theme_default.c and i tried to change someting. Here i copy the basic init of night theme, but nothing change on my screen. What's wrong? 00079 00080 lv_style_copy(&def, &lv_style_pretty); /*Initialize the default style*/ 00081 def.text.font = _font; 00082 00083 lv_style_copy(&bg, &lv_style_plain); 00084 bg.body.main_color = lv_color_hsv_to_rgb(_hue, 11, 30); 00085 bg.body.grad_color = lv_color_hsv_to_rgb(_hue, 11, 30); 00086 bg.text.color = lv_color_hsv_to_rgb(_hue, 5, 95); 00087 bg.text.font = _font; 00088 bg.image.color = lv_color_hsv_to_rgb(_hue, 5, 95); 00089 00090 lv_style_copy(&scr, &bg); 00091 scr.body.padding.bottom = 0; 00092 scr.body.padding.top = 0; 00093 scr.body.padding.left = 0; 00094 scr.body.padding.right = 0; 00095 00096 lv_style_copy(&sb, &def); 00097 sb.body.main_color = lv_color_hsv_to_rgb(_hue, 30, 60); 00098 sb.body.grad_color = lv_color_hsv_to_rgb(_hue, 30, 60); 00099 sb.body.border.width = 0; 00100 sb.body.padding.inner = LV_DPI / 20; 00101 sb.body.padding.left = 0; 00102 sb.body.padding.right = 0; 00103 sb.body.padding.top = 0; 00104 sb.body.padding.bottom = 0; 00105 sb.body.radius = LV_DPI / 30; 00106 sb.body.opa = LV_OPA_COVER; 00107 00108 lv_style_copy(&panel, &bg); 00109 panel.body.main_color = lv_color_hsv_to_rgb(_hue, 11, 18); 00110 panel.body.grad_color = lv_color_hsv_to_rgb(_hue, 11, 18); 00111 panel.body.radius = LV_DPI / 20; 00112 panel.body.border.color = lv_color_hsv_to_rgb(_hue, 10, 25); 00113 panel.body.border.width = 1; 00114 panel.body.border.opa = LV_OPA_COVER; 00115 panel.body.padding.left = LV_DPI / 10; 00116 panel.body.padding.right = LV_DPI / 10; 00117 panel.body.padding.top = LV_DPI / 10; 00118 panel.body.padding.bottom = LV_DPI / 10; 00119 panel.line.color = lv_color_hsv_to_rgb(_hue, 20, 40); 00120 panel.line.width = 1; 00121 00122 theme.style.scr = &scr; 00123 theme.style.bg = &bg; 00124 theme.style.panel = &def; 00125 } 00126 00127 static void btn_init(void) 00128 { 00129 #if LV_USE_BTN != 0 00130 static lv_style_t btn_rel, btn_pr, btn_tgl_rel, btn_tgl_pr, btn_ina; 00131 00132 lv_style_copy(&btn_rel, &def); 00133 btn_rel.body.main_color = lv_color_hsv_to_rgb(_hue, 10, 40); 00134 btn_rel.body.grad_color = lv_color_hsv_to_rgb(_hue, 10, 20); 00135 btn_rel.body.border.color = lv_color_hex3(0x111); 00136 btn_rel.body.border.width = 1; 00137 btn_rel.body.border.opa = LV_OPA_70; 00138 btn_rel.body.padding.left = LV_DPI / 4; 00139 btn_rel.body.padding.right = LV_DPI / 4; 00140 btn_rel.body.padding.top = LV_DPI / 8; 00141 btn_rel.body.padding.bottom = LV_DPI / 8; 00142 btn_rel.body.shadow.type = LV_SHADOW_BOTTOM; 00143 btn_rel.body.shadow.color = lv_color_hex3(0x111); 00144 btn_rel.body.shadow.width = LV_DPI / 30; 00145 btn_rel.text.color = lv_color_hex3(0xeee); 00146 btn_rel.image.color = lv_color_hex3(0xeee); 00147 00148 lv_style_copy(&btn_pr, &btn_rel); 00149 btn_pr.body.main_color = lv_color_hsv_to_rgb(_hue, 10, 30); 00150 btn_pr.body.grad_color = lv_color_hsv_to_rgb(_hue, 10, 10); 00151 00152 lv_style_copy(&btn_tgl_rel, &btn_rel); 00153 btn_tgl_rel.body.main_color = lv_color_hsv_to_rgb(_hue, 10, 20); 00154 btn_tgl_rel.body.grad_color = lv_color_hsv_to_rgb(_hue, 10, 40); 00155 btn_tgl_rel.body.shadow.width = LV_DPI / 40; 00156 btn_tgl_rel.text.color = lv_color_hex3(0xddd); 00157 btn_tgl_rel.image.color = lv_color_hex3(0xddd); 00158 00159 lv_style_copy(&btn_tgl_pr, &btn_rel); 00160 btn_tgl_pr.body.main_color = lv_color_hsv_to_rgb(_hue, 10, 10); 00161 btn_tgl_pr.body.grad_color = lv_color_hsv_to_rgb(_hue, 10, 30); 00162 btn_tgl_pr.body.shadow.width = LV_DPI / 30; 00163 btn_tgl_pr.text.color = lv_color_hex3(0xddd); 00164 btn_tgl_pr.image.color = lv_color_hex3(0xddd); 00165 00166 lv_style_copy(&btn_ina, &btn_rel); 00167 btn_ina.body.main_color = lv_color_hsv_to_rgb(_hue, 10, 20); 00168 btn_ina.body.grad_color = lv_color_hsv_to_rgb(_hue, 10, 20); 00169 btn_ina.body.shadow.width = 0; 00170 btn_ina.text.color = lv_color_hex3(0xaaa); 00171 btn_ina.image.color = lv_color_hex3(0xaaa); 00172 00173 theme.style.btn.rel = &btn_rel; 00174 theme.style.btn.pr = &btn_pr; 00175 theme.style.btn.tgl_rel = &btn_tgl_rel; 00176 theme.style.btn.tgl_pr = &btn_tgl_pr; 00177 theme.style.btn.ina = &btn_ina; 00178 00179 /* 00180 lv_style_copy(&btn_rel, &def); 00181 btn_rel.glass = 0; 00182 btn_rel.body.opa = LV_OPA_TRANSP; 00183 btn_rel.body.radius = LV_RADIUS_CIRCLE; 00184 btn_rel.body.border.width = 2; 00185 btn_rel.body.border.color = lv_color_hsv_to_rgb(_hue, 70, 90); 00186 btn_rel.body.border.opa = LV_OPA_80; 00187 btn_rel.body.padding.left = LV_DPI / 4; 00188 btn_rel.body.padding.right = LV_DPI / 4; 00189 btn_rel.body.padding.top = LV_DPI / 6; 00190 btn_rel.body.padding.bottom = LV_DPI / 6; 00191 btn_rel.body.padding.inner = LV_DPI / 10; 00192 btn_rel.text.color = lv_color_hsv_to_rgb(0, 0, 0); 00193 btn_rel.text.font = _font; 00194 btn_rel.image.color = lv_color_hsv_to_rgb(_hue, 8, 96); 00195 00196 // theme.style.btn.rel = &lv_style_btn_rel; 00197 theme.style.btn.rel = &lv_style_btn_rel; 00198 theme.style.btn.pr = &lv_style_btn_pr; 00199 theme.style.btn.tgl_rel = &lv_style_btn_tgl_rel; 00200 theme.style.btn.tgl_pr = &lv_style_btn_tgl_pr; 00201 theme.style.btn.ina = &lv_style_btn_ina; 00202 */ 00203 #endif 00204 } 00205 00206 static void label_init(void) 00207 { 00208 #if LV_USE_LABEL != 0 00209 00210 lv_style_copy(&label_prim, &lv_style_plain); 00211 lv_style_copy(&label_sec, &lv_style_plain); 00212 lv_style_copy(&label_hint, &lv_style_plain); 00213 00214 label_prim.text.color = lv_color_hex3(0x111); 00215 label_sec.text.color = lv_color_hex3(0x888); 00216 label_hint.text.color = lv_color_hex3(0xaaa); 00217 00218 theme.style.label.prim = &label_prim; 00219 theme.style.label.sec = &label_sec; 00220 theme.style.label.hint = &label_hint; 00221 #endif 00222 } 00223 00224 static void img_init(void) 00225 { 00226 #if LV_USE_IMG != 0 00227 00228 theme.style.img.light = &def; 00229 theme.style.img.dark = &def; 00230 #endif 00231 } 00232 00233 static void line_init(void) 00234 { 00235 #if LV_USE_LINE != 0 00236 00237 theme.style.line.decor = &def; 00238 #endif 00239 } 00240 00241 static void led_init(void) 00242 { 00243 #if LV_USE_LED != 0 00244 static lv_style_t led; 00245 00246 lv_style_copy(&led, &lv_style_pretty_color); 00247 led.body.shadow.width = LV_DPI / 10; 00248 led.body.radius = LV_RADIUS_CIRCLE; 00249 led.body.border.width = LV_DPI / 30; 00250 led.body.border.opa = LV_OPA_30; 00251 led.body.shadow.color = led.body.main_color; 00252 00253 theme.style.led = &led; 00254 #endif 00255 } 00256 00257 static void bar_init(void) 00258 { 00259 #if LV_USE_BAR 00260 00261 theme.style.bar.bg = &lv_style_pretty; 00262 theme.style.bar.indic = &lv_style_pretty_color; 00263 #endif 00264 } 00265 00266 static void slider_init(void) 00267 { 00268 #if LV_USE_SLIDER != 0 00269 static lv_style_t slider_bg; 00270 lv_style_copy(&slider_bg, &lv_style_pretty); 00271 slider_bg.body.padding.left = LV_DPI / 20; 00272 slider_bg.body.padding.right = LV_DPI / 20; 00273 slider_bg.body.padding.top = LV_DPI / 20; 00274 slider_bg.body.padding.bottom = LV_DPI / 20; 00275 00276 theme.style.slider.bg = &slider_bg; 00277 theme.style.slider.indic = &lv_style_pretty_color; 00278 theme.style.slider.knob = &lv_style_pretty; 00279 #endif 00280 } 00281 00282 static void sw_init(void) 00283 { 00284 #if LV_USE_SW != 0 00285 static lv_style_t sw_bg; 00286 lv_style_copy(&sw_bg, &lv_style_pretty); 00287 sw_bg.body.padding.left = 3; 00288 sw_bg.body.padding.right = 3; 00289 sw_bg.body.padding.top = 3; 00290 sw_bg.body.padding.bottom = 3; 00291 00292 theme.style.sw.bg = &sw_bg; 00293 theme.style.sw.indic = &lv_style_pretty_color; 00294 theme.style.sw.knob_off = &lv_style_pretty; 00295 theme.style.sw.knob_on = &lv_style_pretty; 00296 #endif 00297 } 00298 00299 static void lmeter_init(void) 00300 { 00301 #if LV_USE_LMETER != 0 00302 static lv_style_t lmeter; 00303 lv_style_copy(&lmeter, &lv_style_pretty_color); 00304 lmeter.line.color = lv_color_hex3(0xddd); 00305 lmeter.line.width = 2; 00306 lmeter.body.main_color = lv_color_mix(lmeter.body.main_color, LV_COLOR_WHITE, LV_OPA_50); 00307 lmeter.body.grad_color = lv_color_mix(lmeter.body.grad_color, LV_COLOR_BLACK, LV_OPA_50); 00308 00309 theme.style.lmeter = &lmeter; 00310 #endif 00311 } 00312 00313 static void gauge_init(void) 00314 { 00315 #if LV_USE_GAUGE != 0 00316 static lv_style_t gauge; 00317 lv_style_copy(&gauge, theme.style.lmeter); 00318 gauge.line.color = theme.style.lmeter->body.grad_color; 00319 gauge.line.width = 2; 00320 gauge.body.main_color = lv_color_hex3(0x888); 00321 gauge.body.grad_color = theme.style.lmeter->body.main_color; 00322 gauge.text.color = lv_color_hex3(0x888); 00323 00324 theme.style.gauge = &gauge; 00325 #endif 00326 } 00327 00328 static void chart_init(void) 00329 { 00330 #if LV_USE_CHART 00331 00332 theme.style.chart = &lv_style_pretty; 00333 #endif 00334 } 00335 00336 static void cb_init(void) 00337 { 00338 #if LV_USE_CB != 0 00339 00340 theme.style.cb.bg = &lv_style_transp; 00341 theme.style.cb.box.rel = &lv_style_pretty; 00342 theme.style.cb.box.pr = &lv_style_btn_pr; 00343 theme.style.cb.box.tgl_rel = &lv_style_btn_tgl_rel; 00344 theme.style.cb.box.tgl_pr = &lv_style_btn_tgl_pr; 00345 theme.style.cb.box.ina = &lv_style_btn_ina; 00346 #endif 00347 } 00348 00349 static void btnm_init(void) 00350 { 00351 #if LV_USE_BTNM 00352 00353 theme.style.btnm.bg = &lv_style_pretty; 00354 theme.style.btnm.btn.rel = &lv_style_btn_rel; 00355 theme.style.btnm.btn.pr = &lv_style_btn_pr; 00356 theme.style.btnm.btn.tgl_rel = &lv_style_btn_tgl_rel; 00357 theme.style.btnm.btn.tgl_pr = &lv_style_btn_tgl_pr; 00358 theme.style.btnm.btn.ina = &lv_style_btn_ina; 00359 #endif 00360 } 00361 00362 static void kb_init(void) 00363 { 00364 #if LV_USE_KB 00365 00366 theme.style.kb.bg = &lv_style_pretty; 00367 theme.style.kb.btn.rel = &lv_style_btn_rel; 00368 theme.style.kb.btn.pr = &lv_style_btn_pr; 00369 theme.style.kb.btn.tgl_rel = &lv_style_btn_tgl_rel; 00370 theme.style.kb.btn.tgl_pr = &lv_style_btn_tgl_pr; 00371 theme.style.kb.btn.ina = &lv_style_btn_ina; 00372 #endif 00373 } 00374 00375 static void mbox_init(void) 00376 { 00377 #if LV_USE_MBOX 00378 00379 theme.style.mbox.bg = &lv_style_pretty; 00380 theme.style.mbox.btn.bg = &lv_style_transp; 00381 theme.style.mbox.btn.rel = &lv_style_btn_rel; 00382 theme.style.mbox.btn.pr = &lv_style_btn_tgl_pr; 00383 #endif 00384 } 00385 00386 static void page_init(void) 00387 { 00388 #if LV_USE_PAGE 00389 00390 theme.style.page.bg = &lv_style_pretty; 00391 theme.style.page.scrl = &lv_style_transp_tight; 00392 theme.style.page.sb = &sb; 00393 #endif 00394 } 00395 00396 static void ta_init(void) 00397 { 00398 #if LV_USE_TA 00399 00400 theme.style.ta.area = &lv_style_pretty; 00401 theme.style.ta.oneline = &lv_style_pretty; 00402 theme.style.ta.cursor = NULL; 00403 theme.style.ta.sb = &sb; 00404 #endif 00405 } 00406 00407 static void list_init(void) 00408 { 00409 #if LV_USE_LIST != 0 00410 00411 theme.style.list.bg = &lv_style_pretty; 00412 theme.style.list.scrl = &lv_style_transp_fit; 00413 theme.style.list.sb = &sb; 00414 theme.style.list.btn.rel = &lv_style_btn_rel; 00415 theme.style.list.btn.pr = &lv_style_btn_pr; 00416 theme.style.list.btn.tgl_rel = &lv_style_btn_tgl_rel; 00417 theme.style.list.btn.tgl_pr = &lv_style_btn_tgl_pr; 00418 theme.style.list.btn.ina = &lv_style_btn_ina; 00419 #endif 00420 } 00421 00422 static void ddlist_init(void) 00423 { 00424 #if LV_USE_DDLIST != 0 00425 00426 theme.style.ddlist.bg = &lv_style_pretty; 00427 theme.style.ddlist.sel = &lv_style_plain_color; 00428 theme.style.ddlist.sb = &sb; 00429 #endif 00430 } 00431 00432 static void roller_init(void) 00433 { 00434 #if LV_USE_ROLLER != 0 00435 00436 theme.style.roller.bg = &lv_style_pretty; 00437 theme.style.roller.sel = &lv_style_plain_color; 00438 #endif 00439 } 00440 00441 static void tabview_init(void) 00442 { 00443 #if LV_USE_TABVIEW != 0 00444 00445 theme.style.tabview.bg = &plain_bordered; 00446 theme.style.tabview.indic = &lv_style_plain_color; 00447 theme.style.tabview.btn.bg = &lv_style_transp; 00448 theme.style.tabview.btn.rel = &lv_style_btn_rel; 00449 theme.style.tabview.btn.pr = &lv_style_btn_pr; 00450 theme.style.tabview.btn.tgl_rel = &lv_style_btn_tgl_rel; 00451 theme.style.tabview.btn.tgl_pr = &lv_style_btn_tgl_pr; 00452 #endif 00453 } 00454 00455 static void table_init(void) 00456 { 00457 #if LV_USE_TABLE != 0 00458 theme.style.table.bg = &lv_style_transp_tight; 00459 theme.style.table.cell = &lv_style_plain; 00460 #endif 00461 } 00462 00463 static void win_init(void) 00464 { 00465 #if LV_USE_WIN != 0 00466 00467 theme.style.win.bg = &plain_bordered; 00468 theme.style.win.sb = &sb; 00469 theme.style.win.header = &lv_style_plain_color; 00470 theme.style.win.content = &lv_style_transp; 00471 theme.style.win.btn.rel = &lv_style_btn_rel; 00472 theme.style.win.btn.pr = &lv_style_btn_pr; 00473 #endif 00474 } 00475 00476 #if LV_USE_GROUP 00477 00478 static void style_mod(lv_group_t * group, lv_style_t * style) 00479 { 00480 (void)group; /*Unused*/ 00481 #if LV_COLOR_DEPTH != 1 00482 /*Make the style to be a little bit orange*/ 00483 style->body.border.opa = LV_OPA_COVER; 00484 style->body.border.color = LV_COLOR_ORANGE; 00485 00486 /*If not empty or has border then emphasis the border*/ 00487 if(style->body.opa != LV_OPA_TRANSP || style->body.border.width != 0) style->body.border.width = LV_DPI / 20; 00488 00489 style->body.main_color = lv_color_mix(style->body.main_color, LV_COLOR_ORANGE, LV_OPA_70); 00490 style->body.grad_color = lv_color_mix(style->body.grad_color, LV_COLOR_ORANGE, LV_OPA_70); 00491 style->body.shadow.color = lv_color_mix(style->body.shadow.color, LV_COLOR_ORANGE, LV_OPA_60); 00492 00493 style->text.color = lv_color_mix(style->text.color, LV_COLOR_ORANGE, LV_OPA_70); 00494 #else 00495 style->body.border.opa = LV_OPA_COVER; 00496 style->body.border.color = LV_COLOR_BLACK; 00497 style->body.border.width = 2; 00498 #endif 00499 } 00500 00501 static void style_mod_edit(lv_group_t * group, lv_style_t * style) 00502 { 00503 (void)group; /*Unused*/ 00504 #if LV_COLOR_DEPTH != 1 00505 /*Make the style to be a little bit orange*/ 00506 style->body.border.opa = LV_OPA_COVER; 00507 style->body.border.color = LV_COLOR_GREEN; 00508 00509 /*If not empty or has border then emphasis the border*/ 00510 if(style->body.opa != LV_OPA_TRANSP || style->body.border.width != 0) style->body.border.width = LV_DPI / 20; 00511 00512 style->body.main_color = lv_color_mix(style->body.main_color, LV_COLOR_GREEN, LV_OPA_70); 00513 style->body.grad_color = lv_color_mix(style->body.grad_color, LV_COLOR_GREEN, LV_OPA_70); 00514 style->body.shadow.color = lv_color_mix(style->body.shadow.color, LV_COLOR_GREEN, LV_OPA_60); 00515 00516 style->text.color = lv_color_mix(style->text.color, LV_COLOR_GREEN, LV_OPA_70); 00517 #else 00518 style->body.border.opa = LV_OPA_COVER; 00519 style->body.border.color = LV_COLOR_BLACK; 00520 style->body.border.width = 3; 00521 #endif 00522 } 00523 00524 #endif /*LV_USE_GROUP*/ 00525 00526 /********************** 00527 * GLOBAL FUNCTIONS 00528 **********************/ 00529 00530 /** 00531 * Initialize the default theme 00532 * @param hue [0..360] hue value from HSV color space to define the theme's base color 00533 * @param font pointer to a font (NULL to use the default) 00534 * @return pointer to the initialized theme 00535 */ 00536 lv_theme_t * lv_theme_rb_init(uint16_t hue, lv_font_t * font) 00537 { 00538 if(font == NULL) font = LV_FONT_DEFAULT; 00539 // font = &lv_font_roboto_22; //GABOR : Doesn't work:? 00540 // font = &RB_lv_font_roboto_16; //GABOR : Doesn't work:? 00541 00542 00543 _hue = hue; 00544 _font = font; 00545 00546 /*For backward compatibility initialize all theme elements with a default style */ 00547 uint16_t i; 00548 lv_style_t ** style_p = (lv_style_t **)&theme.style; 00549 for(i = 0; i < LV_THEME_STYLE_COUNT; i++) { 00550 *style_p = &def; 00551 style_p++; 00552 } 00553 00554 basic_init(); 00555 btn_init(); 00556 label_init(); 00557 img_init(); 00558 line_init(); 00559 led_init(); 00560 bar_init(); 00561 slider_init(); 00562 sw_init(); 00563 lmeter_init(); 00564 gauge_init(); 00565 chart_init(); 00566 cb_init(); 00567 btnm_init(); 00568 kb_init(); 00569 mbox_init(); 00570 page_init(); 00571 ta_init(); 00572 list_init(); 00573 ddlist_init(); 00574 roller_init(); 00575 tabview_init(); 00576 table_init(); 00577 win_init(); 00578 00579 #if LV_USE_GROUP 00580 theme.group.style_mod_xcb = style_mod; 00581 theme.group.style_mod_edit_xcb = style_mod_edit; 00582 #endif 00583 00584 return &theme; 00585 } 00586 00587 /** 00588 * Get a pointer to the theme 00589 * @return pointer to the theme 00590 */ 00591 lv_theme_t * lv_theme_get_rb(void) 00592 { 00593 return &theme; 00594 } 00595 00596 /********************** 00597 * STATIC FUNCTIONS 00598 **********************/ 00599
Generated on Sun Jul 17 2022 20:20:43 by
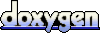