added init function from NXP Rapid ioT SDK, read_proximity_sensors function now works well.
Dependents: rIoTwear-touch rIoTwear-snake
sx9500.h
00001 #include "mbed.h" 00002 00003 #define SX9500_REG_IRQSRC 0x00 00004 #define SX9500_REG_STAT 0x01 00005 #define SX9500_REG_IRQMSK 0x03 00006 #define SX9500_REG_PROXCTRL0 0x06 00007 #define SX9500_REG_PROXCTRL1 0x07 00008 #define SX9500_REG_PROXCTRL2 0x08 00009 #define SX9500_REG_PROXCTRL3 0x09 00010 #define SX9500_REG_PROXCTRL4 0x0A 00011 #define SX9500_REG_PROXCTRL5 0x0B 00012 #define SX9500_REG_PROXCTRL6 0x0C 00013 #define SX9500_REG_PROXCTRL7 0x0D 00014 #define SX9500_REG_PROXCTRL8 0x0E 00015 #define SX9500_REG_SENSORSEL 0x20 00016 #define SX9500_REG_USEMSB 0x21 00017 #define SX9500_REG_USELSB 0x22 00018 #define SX9500_REG_AVGMSB 0x23 00019 #define SX9500_REG_AVGLSB 0x24 00020 #define SX9500_REG_DIFFMSB 0x25 00021 #define SX9500_REG_DIFFLSB 0x26 00022 #define SX9500_REG_OFFSETMSB 0x27 00023 #define SX9500_REG_OFFSETLSB 0x28 00024 #define SX9500_REG_RESET 0x7F 00025 00026 #define SX9500_RESET_CMD 0xDE 00027 00028 /* 00029 * Copyright (c) 2018 NXP 00030 * 00031 * Redistribution and use in source and binary forms, with or without modification, 00032 * are permitted provided that the following conditions are met: 00033 * 00034 * o Redistributions of source code must retain the above copyright notice, this list 00035 * of conditions and the following disclaimer. 00036 * 00037 * o Redistributions in binary form must reproduce the above copyright notice, this 00038 * list of conditions and the following disclaimer in the documentation and/or 00039 * other materials provided with the distribution. 00040 * 00041 * o Neither the name of the copyright holder nor the names of its 00042 * contributors may be used to endorse or promote products derived from this 00043 * software without specific prior written permission. 00044 * 00045 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 00046 * ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00047 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00048 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR 00049 * ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00050 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00051 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON 00052 * ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00053 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00054 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00055 */ 00056 00057 /* Define registers that need to be initialized to values different than 00058 * default 00059 */ 00060 typedef struct smtc_reg_data { 00061 unsigned char reg; 00062 unsigned char val; 00063 }smtc_reg_data_t; 00064 static smtc_reg_data_t sx9500_i2c_reg_setup[] = { 00065 { 00066 .reg = SX9500_REG_IRQMSK, 00067 .val = 0x60, /* CLOSEIRQEN[6]=1 (close interrupt is on), */ 00068 /* FARIRQEN[5]=1 (far interrupt is on), */ 00069 /* COMPDONEIRQEN[4]=0 (compensation interrupt is off), */ 00070 /* CONVDONEIRQEN[3]=0 (conversion interrupt is off) */ 00071 }, 00072 { 00073 .reg = SX9500_REG_PROXCTRL1, 00074 .val = 0x03, /* SHIELDEN[7:6]=0 (no shield), */ 00075 /* RANGE[1:0]=3 (small, +/-2.5pF Full Scale) */ 00076 }, 00077 { 00078 .reg = SX9500_REG_PROXCTRL2, 00079 .val = 0x27, /* GAIN[6:5]=1 (digital gain x2), */ 00080 /* FREQ[4:3]=0 (83kHz sampling frequency), */ 00081 /* RESOLUTION[2:0]=0 (finest resolution) */ 00082 }, 00083 { 00084 .reg = SX9500_REG_PROXCTRL3, 00085 .val = 0x41, /* DOZEEN[6]=1 (enables doze mode), */ 00086 /* DOZEPERIOD[5:4]=0 (2*scan period), */ 00087 /* RAWFILT[1:0]=1 (Low) */ 00088 }, 00089 { 00090 .reg = SX9500_REG_PROXCTRL4, 00091 .val = 0x80, /* AVGTHRESH[7:0]=0x80 (threshold triggering compensation = +/-128*value (typ between 16384 and 24576) */ 00092 }, 00093 { 00094 .reg = SX9500_REG_PROXCTRL5, 00095 .val = 0x0F, /* AVGDEB[7:6]=0 (debounce=off), */ 00096 /* AVGNEGFILT[5:3]=1 (lowest negative filter), */ 00097 /* AVGPOSFILT[2:0]=7 (highest positive filter) */ 00098 }, 00099 { 00100 .reg = SX9500_REG_PROXCTRL6, 00101 .val = 0x06, /* PROXTHRESH[4:0]=6 (sensitivity=120) */ 00102 }, 00103 { 00104 .reg = SX9500_REG_PROXCTRL7, 00105 .val = 0x00, /* AVGCOMPDIS[7]=0 (compensation enabled), */ 00106 /* COMPMETHOD[6]=0 (separate CSx compensation), */ 00107 /* HYST[5:4]=0 (hysteresis=32), */ 00108 /* CLOSEDEB[3:2]=0 (close debouncer=off), */ 00109 /* FARDEB[1:0]=0 (far debouncer=off) */ 00110 }, 00111 { 00112 .reg = SX9500_REG_PROXCTRL8, 00113 .val = 0x08, /* STUCK[7:4]=0 (stuck timeout=off), */ 00114 /* COMPPRD[3:0]=8 (periodic compensation every 8*128 samples) */ 00115 }, 00116 { 00117 .reg = SX9500_REG_PROXCTRL0, 00118 .val = 0x0F, /* SCANPERIOD[6:4]=0 (scan every 30ms), */ 00119 /* SENSOREN[3:0]=15 (enable all sensors) */ 00120 }, 00121 }; 00122 00123 typedef enum 00124 { 00125 SX9500_SUCCESS, 00126 SX9500_I2C_ERROR, 00127 SX9500_INTERNAL_ERROR, 00128 SX9500_NOINIT_ERROR 00129 } SX9500_status; 00130 00131 typedef struct { 00132 bool downPressed; 00133 bool upPressed; 00134 bool leftPressed; 00135 bool rightPressed; 00136 } SX9500_TouchState_t; 00137 00138 /* 00139 * END Copyright (c) 2018 NXP 00140 * 00141 */ 00142 00143 typedef union { 00144 struct { // sx9500 register 0x09 00145 uint8_t txen_stat : 1; // 0 00146 uint8_t reserved : 2; // 1,2 00147 uint8_t conv_done : 1; // 3 00148 uint8_t comp_done : 1; // 4 00149 uint8_t far : 1; // 5 00150 uint8_t close : 1; // 6 00151 uint8_t reset : 1; // 7 00152 } bits; 00153 uint8_t octet; 00154 } RegIrqSrc_t; 00155 00156 typedef union { 00157 struct { // sx9500 register 0x09 00158 uint8_t compstat : 4; // 0,1,2,3 00159 uint8_t proxstat0 : 1; // 4 00160 uint8_t proxstat1 : 1; // 5 00161 uint8_t proxstat2 : 1; // 6 00162 uint8_t proxstat3 : 1; // 7 00163 } bits; 00164 uint8_t octet; 00165 } RegStat_t; 00166 00167 typedef union { 00168 struct { // sx9500 register 0x06 00169 uint8_t sensor_en : 4; // 0,1,2,3 00170 uint8_t scan_period : 3; // 4,5,6 00171 uint8_t reserved : 1; // 7 00172 } bits; 00173 uint8_t octet; 00174 } RegProxCtrl0_t; 00175 00176 typedef union { 00177 struct { // sx9500 register 0x09 00178 uint8_t raw_filt : 2; // 0,1 00179 uint8_t reserved : 2; // 2,3 00180 uint8_t doze_period : 2; // 4,5 00181 uint8_t doze_en : 1; // 6 00182 uint8_t res7 : 1; // 7 00183 } bits; 00184 uint8_t octet; 00185 } RegProxCtrl3_t; 00186 00187 class SX9500 { 00188 public: 00189 SX9500(I2C& r, PinName en_pin, PinName nirq_pin); 00190 ~SX9500(); 00191 //void try_read(void); 00192 uint8_t read_single(uint8_t addr); 00193 void read(uint8_t addr, uint8_t *dst_buf, int length); 00194 void write(uint8_t addr, uint8_t data); 00195 void reset(void); 00196 //uint16_t get_sensor(char CSn); 00197 void print_sensor(char CSn); 00198 SX9500_TouchState_t read_proximity_sensors(); 00199 void set_active(bool); 00200 bool get_active(void); 00201 void service(void); 00202 uint8_t init(void); 00203 00204 RegIrqSrc_t RegIrqSrc; 00205 RegProxCtrl0_t RegProxCtrl0; 00206 00207 private: 00208 I2C& m_i2c; 00209 DigitalOut m_txen; 00210 DigitalIn m_nirq; // polled irq pin, because i2c is shared 00211 }; 00212
Generated on Wed Jul 20 2022 16:14:59 by
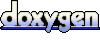