added init function from NXP Rapid ioT SDK, read_proximity_sensors function now works well.
Dependents: rIoTwear-touch rIoTwear-snake
sx9500.cpp
00001 #include "sx9500.h" 00002 00003 #define SX9500_I2C_ADDRESS 0x50 //0x28 00004 00005 00006 SX9500::SX9500(I2C& r, PinName en_pin, PinName nirq_pin) : m_i2c(r), m_txen(en_pin), m_nirq(nirq_pin) 00007 { 00008 m_nirq.mode(PullUp); // no pullup on board? 00009 RegProxCtrl0.octet = read_single(SX9500_REG_PROXCTRL0); 00010 } 00011 00012 SX9500::~SX9500() 00013 { 00014 } 00015 00016 void SX9500::reset() 00017 { 00018 write(SX9500_REG_RESET, SX9500_RESET_CMD); 00019 } 00020 00021 void SX9500::print_sensor(char CSn) 00022 { 00023 uint8_t buf[2]; 00024 00025 write(SX9500_REG_SENSORSEL, CSn); 00026 00027 read(SX9500_REG_USEMSB, buf, 2); 00028 printf("%d useful:0x%02x%02x\r\n", CSn, buf[0], buf[1]); 00029 00030 read(SX9500_REG_AVGMSB, buf, 2); 00031 //printf("%d avg:0x%02x%02x\r\n", CSn, buf[0], buf[1]); 00032 00033 read(SX9500_REG_DIFFMSB, buf, 2); 00034 // printf("%d diff:0x%02x%02x\r\n", CSn, buf[0], buf[1]); 00035 } 00036 00037 00038 /* 00039 * Copyright (c) 2018 NXP 00040 * 00041 * Redistribution and use in source and binary forms, with or without modification, 00042 * are permitted provided that the following conditions are met: 00043 * 00044 * o Redistributions of source code must retain the above copyright notice, this list 00045 * of conditions and the following disclaimer. 00046 * 00047 * o Redistributions in binary form must reproduce the above copyright notice, this 00048 * list of conditions and the following disclaimer in the documentation and/or 00049 * other materials provided with the distribution. 00050 * 00051 * o Neither the name of the copyright holder nor the names of its 00052 * contributors may be used to endorse or promote products derived from this 00053 * software without specific prior written permission. 00054 * 00055 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 00056 * ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00057 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00058 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR 00059 * ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00060 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00061 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON 00062 * ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00063 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00064 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00065 */ 00066 00067 uint8_t SX9500::init(void) 00068 { 00069 uint8_t lenRegTable = sizeof(sx9500_i2c_reg_setup) / sizeof(smtc_reg_data_t); 00070 uint8_t val; 00071 uint8_t i = 0; 00072 00073 // read IRQSRC to release NIRQ pin 00074 read(SX9500_REG_IRQSRC, &val,1); 00075 00076 while (i < lenRegTable) 00077 { 00078 /* Write all registers/values contained in i2c_reg */ 00079 write(sx9500_i2c_reg_setup[i].reg, sx9500_i2c_reg_setup[i].val); 00080 00081 /* Read back value from register and verify write */ 00082 val = read_single(sx9500_i2c_reg_setup[i].reg); 00083 00084 if (val != sx9500_i2c_reg_setup[i].val) 00085 { 00086 return SX9500_INTERNAL_ERROR; 00087 } 00088 00089 i++; 00090 } 00091 00092 return SX9500_SUCCESS; 00093 00094 } 00095 00096 /* 00097 * END Copyright (c) 2018 NXP 00098 * 00099 */ 00100 00101 SX9500_TouchState_t SX9500::read_proximity_sensors() 00102 { 00103 SX9500_TouchState_t touchState; 00104 RegStat_t prox; 00105 00106 read(SX9500_REG_STAT, &prox.octet, 2); 00107 00108 memset((void *)&touchState, 0, sizeof(SX9500_TouchState_t)); 00109 if(prox.octet > 0) 00110 { 00111 if(prox.bits.proxstat0) 00112 touchState.downPressed = true; 00113 00114 if(prox.bits.proxstat1) 00115 touchState.rightPressed = true; 00116 00117 if(prox.bits.proxstat2) 00118 touchState.upPressed = true; 00119 00120 if(prox.bits.proxstat3) 00121 touchState.leftPressed = true; 00122 } 00123 00124 return touchState; 00125 } 00126 00127 void SX9500::write(uint8_t addr, uint8_t data) 00128 { 00129 uint8_t cmd[2]; 00130 00131 cmd[0] = addr; 00132 cmd[1] = data; 00133 00134 if (m_i2c.write(SX9500_I2C_ADDRESS, (char *)cmd, 2)) 00135 printf("SX9500 write-fail\n"); 00136 } 00137 00138 void SX9500::read(uint8_t addr, uint8_t *dst_buf, int length) 00139 { 00140 char cmd[2]; 00141 00142 cmd[0] = addr; 00143 if (m_i2c.write(SX9500_I2C_ADDRESS, cmd, 1, true)) 00144 printf("SX9500 write-fail\n"); 00145 if (m_i2c.read(SX9500_I2C_ADDRESS, (char *)dst_buf, length)) 00146 printf("SX9500 read-fail\n"); 00147 } 00148 00149 uint8_t SX9500::read_single(uint8_t addr) 00150 { 00151 char cmd[2]; 00152 00153 cmd[0] = addr; 00154 if (m_i2c.write(SX9500_I2C_ADDRESS, cmd, 1, true)) 00155 printf("SX9500 write-fail\n"); 00156 if (m_i2c.read(SX9500_I2C_ADDRESS, cmd, 1)) 00157 printf("SX9500 read-fail\n"); 00158 00159 return cmd[0]; 00160 } 00161 00162 bool SX9500::get_active() 00163 { 00164 if (m_txen.read()) 00165 return true; 00166 else 00167 return false; 00168 } 00169 00170 void SX9500::set_active(bool en) 00171 { 00172 if (en) { 00173 m_txen = 1; 00174 } else { 00175 /* lowest power (non)operation */ 00176 m_txen = 0; 00177 write(SX9500_REG_PROXCTRL0, 0); // turn off all sensor pins 00178 } 00179 } 00180 00181 void SX9500::service() 00182 { 00183 if (m_nirq.read()) 00184 return; 00185 00186 RegIrqSrc.octet = read_single(SX9500_REG_IRQSRC); 00187 00188 /* 00189 printf("95irq:"); 00190 if (RegIrqSrc.bits.conv_done) { 00191 printf("conv_done:\r\n"); 00192 print_sensor(0); 00193 print_sensor(1); 00194 } 00195 if (RegIrqSrc.bits.comp_done) { 00196 printf("comp_done "); 00197 } 00198 if (RegIrqSrc.bits.far || RegIrqSrc.bits.close) { 00199 RegStat_t stat; 00200 printf("stat "); 00201 stat.octet = read_single(SX9500_REG_STAT); 00202 if (stat.bits.proxstat0) 00203 printf("cs0 "); 00204 if (stat.bits.proxstat1) 00205 printf("cs1 "); 00206 } 00207 00208 if (RegIrqSrc.bits.reset) { 00209 printf("reset "); 00210 } 00211 00212 printf("\r\n"); 00213 */ 00214 } 00215
Generated on Wed Jul 20 2022 16:14:59 by
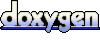