Add the RTOS processing. for the Network radio streaming receiver.
Fork of VS1053b by
VS1053.cpp
00001 /* mbed VLSI VS1053b library 00002 * Copyright (c) 2010 Christian Schmiljun 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 00023 /* This code based on: 00024 * mbeduino_MP3_Shield_MP3Player 00025 * http://mbed.org/users/xshige/programs/mbeduino_MP3_Shield_MP3Player/lgcx63 00026 * 2010-10-16 00027 */ 00028 00029 #include "VS1053.h" 00030 #include "mbed.h" 00031 00032 // patch binarys 00033 #include "Patches/VS1053b_patch_1_5.c" 00034 #include "Patches/VS1053b_patch_1_5_flac.c" 00035 #include "Patches/VS1053b_patch_1_4_flac.c" 00036 #include "Patches/VS1053b_specana_0_9.c" 00037 #include "Patches/VS1053b_pcm_recorder_0_9.c" 00038 00039 const char VS1053::_sampleRateTable[4][4] = { 00040 11, 12, 8, 0, 00041 11, 12, 8, 0, 00042 22, 24, 16, 0, 00043 44, 48, 32, 0 00044 }; 00045 00046 /* ================================================================== 00047 * Constructor 00048 * =================================================================*/ 00049 VS1053::VS1053( 00050 PinName mosi, PinName miso, PinName sck, PinName cs, PinName rst, 00051 PinName dreq, PinName dcs, char* buffer, int buffer_size) 00052 : 00053 _spi(mosi, miso, sck), 00054 _CS(cs), 00055 _RST(rst), 00056 _DCS(dcs), 00057 _DREQ(dreq), 00058 #ifdef DEBUG 00059 _led(D8), 00060 #endif 00061 #ifdef VS1053_RTOS 00062 _thread(VS1053::dataRequestHandler_start, this, osPriorityNormal, 1024) 00063 #else 00064 _DREQ_INTERUPT_IN(dreq) 00065 #endif 00066 { 00067 cs_high(); 00068 dcs_high(); 00069 00070 _volume = DEFAULT_VOLUME; 00071 _balance = DEFAULT_BALANCE_DIFERENCE_LEFT_RIGHT; 00072 _sb_amplitude = DEFAULT_BASS_AMPLITUDE; 00073 _sb_freqlimit = DEFAULT_BASS_FREQUENCY; 00074 _st_amplitude = DEFAULT_TREBLE_AMPLITUDE; 00075 _st_freqlimit = DEFAULT_TREBLE_FREQUENCY; 00076 _buffer = buffer; 00077 BUFFER_SIZE = buffer_size; 00078 #ifndef VS1053_RTOS 00079 _DREQ_INTERUPT_IN.mode(PullDown); 00080 #endif 00081 INTERRUPT_HANDLER_DISABLE; 00082 bufferReset(); 00083 00084 #ifdef VS1053_RTOS 00085 _thread.signal_set(START_THREAD); 00086 #endif 00087 } 00088 00089 00090 /*=================================================================== 00091 * Functions 00092 *==================================================================*/ 00093 00094 void VS1053::cs_low(void) { 00095 _CS = 0; 00096 } 00097 void VS1053::cs_high(void) { 00098 _CS = 1; 00099 } 00100 void VS1053::dcs_low(void) { 00101 _DCS = 0; 00102 00103 } 00104 void VS1053::dcs_high(void) { 00105 _DCS = 1; 00106 } 00107 void VS1053::sci_en(void) { //SCI enable 00108 cs_high(); 00109 dcs_high(); 00110 cs_low(); 00111 } 00112 void VS1053::sci_dis(void) { //SCI disable 00113 cs_high(); 00114 } 00115 void VS1053::sdi_en(void) { //SDI enable 00116 dcs_high(); 00117 cs_high(); 00118 dcs_low(); 00119 } 00120 void VS1053::sdi_dis(void) { //SDI disable 00121 dcs_high(); 00122 } 00123 void VS1053::reset(void) { //hardware reset 00124 INTERRUPT_HANDLER_DISABLE; 00125 wait_ms(10); 00126 _RST = 0; 00127 wait_ms(5); 00128 _RST = 1; 00129 wait_ms(10); 00130 } 00131 void VS1053::power_down(void) { //hardware and software reset 00132 cs_low(); 00133 reset(); 00134 // sci_write(0x00, SM_PDOWN); 00135 sci_write(0x00, 0x10); // tempo 00136 wait(0.01); 00137 reset(); 00138 } 00139 void VS1053::spi_initialise(void) { 00140 _RST = 1; //no reset 00141 _spi.format(8,0); //spi 8bit interface, steady state low 00142 // _spi.frequency(1000000); //rising edge data record, freq. 1Mhz 00143 _spi.frequency(2000000); //rising edge data record, freq. 2Mhz 00144 00145 00146 cs_low(); 00147 for (int i=0; i<4; i++) { 00148 _spi.write(0xFF); //clock the chip a bit 00149 } 00150 cs_high(); 00151 dcs_high(); 00152 wait_us(5); 00153 } 00154 void VS1053::sdi_initialise(void) { 00155 _spi.frequency(8000000); //set to 8 MHz to make fast transfer 00156 cs_high(); 00157 dcs_high(); 00158 } 00159 void VS1053::sci_write(unsigned char address, unsigned short int data) { 00160 // TODO disable all interrupts 00161 __disable_irq(); 00162 sci_en(); //enables SCI/disables SDI 00163 00164 while (!_DREQ); //wait unitl data request is high 00165 _spi.write(0x02); //SCI write 00166 _spi.write(address); //register address 00167 _spi.write((data >> 8) & 0xFF); //write out first half of data word 00168 _spi.write(data & 0xFF); //write out second half of data word 00169 00170 sci_dis(); //enables SDI/disables SCI 00171 wait_us(5); 00172 00173 // TODO enable all interrupts 00174 __enable_irq(); 00175 } 00176 void VS1053::sdi_write(unsigned char datum) { 00177 00178 sdi_en(); 00179 00180 while (!_DREQ); 00181 _spi.write(datum); 00182 00183 sdi_dis(); 00184 } 00185 unsigned short VS1053::sci_read(unsigned short int address) { 00186 // TODO disable all interrupts 00187 __disable_irq(); 00188 00189 cs_low(); //enables SCI/disables SDI 00190 00191 while (!_DREQ); //wait unitl data request is high 00192 _spi.write(0x03); //SCI write 00193 _spi.write(address); //register address 00194 unsigned short int received = _spi.write(0x00); //write out dummy byte 00195 received <<= 8; 00196 received |= _spi.write(0x00); //write out dummy byte 00197 00198 cs_high(); //enables SDI/disables SCI 00199 00200 // TODO enable all interrupts 00201 __enable_irq(); 00202 return received; //return received word 00203 } 00204 void VS1053::sine_test_activate(unsigned char wave) { 00205 cs_high(); //enables SDI/disables SCI 00206 00207 while (!_DREQ); //wait unitl data request is high 00208 _spi.write(0x53); //SDI write 00209 _spi.write(0xEF); //SDI write 00210 _spi.write(0x6E); //SDI write 00211 _spi.write(wave); //SDI write 00212 _spi.write(0x00); //filler byte 00213 _spi.write(0x00); //filler byte 00214 _spi.write(0x00); //filler byte 00215 _spi.write(0x00); //filler byte 00216 00217 cs_low(); //enables SCI/disables SDI 00218 } 00219 void VS1053::sine_test_deactivate(void) { 00220 cs_high(); 00221 00222 while (!_DREQ); 00223 _spi.write(0x45); //SDI write 00224 _spi.write(0x78); //SDI write 00225 _spi.write(0x69); //SDI write 00226 _spi.write(0x74); //SDI write 00227 _spi.write(0x00); //filler byte 00228 _spi.write(0x00); //filler byte 00229 _spi.write(0x00); //filler byte 00230 _spi.write(0x00); //filler byte 00231 } 00232 00233 unsigned short int VS1053::wram_read(unsigned short int address) { 00234 unsigned short int tmp1,tmp2; 00235 sci_write(SCI_WRAMADDR,address); 00236 tmp1=sci_read(SCI_WRAM); 00237 sci_write(SCI_WRAMADDR,address); 00238 tmp2=sci_read(SCI_WRAM); 00239 if (tmp1==tmp2) return tmp1; 00240 sci_write(SCI_WRAMADDR,address); 00241 tmp1=sci_read(SCI_WRAM); 00242 if (tmp1==tmp2) return tmp1; 00243 sci_write(SCI_WRAMADDR,address); 00244 tmp1=sci_read(SCI_WRAM); 00245 if (tmp1==tmp2) return tmp1; 00246 return tmp1; 00247 } 00248 00249 void VS1053::wram_write(unsigned short int address, unsigned short int data) { 00250 sci_write(SCI_WRAMADDR,address); 00251 sci_write(SCI_WRAM,data); 00252 return; 00253 } 00254 00255 void VS1053::setPlaySpeed(unsigned short speed) 00256 { 00257 wram_write(para_playSpeed, speed); 00258 DEBUGOUT("VS1053b: Change speed. New speed: %d\r\n", speed); 00259 } 00260 00261 void VS1053::terminateStream(void) { 00262 while(bufferCount() > 0) 00263 ; 00264 DEBUGOUT("VS1053b: Song terminating..\r\n"); 00265 // send at least 2052 bytes of endFillByte[7:0]. 00266 // read endFillByte (0 .. 15) from wram 00267 unsigned short endFillByte=wram_read(para_endFillByte); 00268 // clear endFillByte (8 .. 15) 00269 endFillByte = endFillByte ^0x00FF; 00270 for (int n = 0; n < 2052; n++) 00271 sdi_write(endFillByte); 00272 00273 // set SCI MODE bit SM CANCEL 00274 unsigned short sciModeByte = sci_read(SCI_MODE); 00275 sciModeByte |= SM_CANCEL; 00276 sci_write(SCI_MODE, sciModeByte); 00277 00278 // send up 2048 bytes of endFillByte[7:0]. 00279 for (int i = 0; i < 64; i++) 00280 { 00281 // send at least 32 bytes of endFillByte[7:0] 00282 for (int n = 0; n < 32; n++) 00283 sdi_write(endFillByte); 00284 // read SCI MODE; if SM CANCEL is still set, repeat 00285 sciModeByte = sci_read(SCI_MODE); 00286 if ((sciModeByte & SM_CANCEL) == 0x0000) 00287 { 00288 break; 00289 } 00290 } 00291 00292 if ((sciModeByte & SM_CANCEL) == 0x0000) 00293 { 00294 DEBUGOUT("VS1053b: Song sucessfully sent. Terminating OK\r\n"); 00295 DEBUGOUT("VS1053b: SCI MODE = %#x, SM_CANCEL = %#x\r\n", sciModeByte, sciModeByte & SM_CANCEL); 00296 sci_write(SCI_DECODE_TIME, 0x0000); 00297 } 00298 else 00299 { 00300 DEBUGOUT("VS1053b: SM CANCEL hasn't cleared after sending 2048 bytes, do software reset\r\n"); 00301 DEBUGOUT("VS1053b: SCI MODE = %#x, SM_CANCEL = %#x\r\n", sciModeByte, sciModeByte & SM_CANCEL); 00302 initialize(); 00303 } 00304 } 00305 00306 void VS1053::write_plugin(const unsigned short *plugin, unsigned int len) { 00307 unsigned int i; 00308 unsigned short addr, n, val; 00309 00310 for (i=0; i<len;) { 00311 addr = plugin[i++]; 00312 n = plugin[i++]; 00313 if (n & 0x8000U) { //RLE run, replicate n samples 00314 n &= 0x7FFF; 00315 val = plugin[i++]; 00316 while (n--) { 00317 sci_write(addr,val); 00318 } 00319 } else { //copy run, copy n sample 00320 while (n--) { 00321 val = plugin[i++]; 00322 sci_write(addr,val); 00323 } 00324 } 00325 } 00326 00327 return; 00328 } 00329 00330 00331 bool VS1053::initialize(void) { 00332 _RST = 1; 00333 cs_high(); //chip disabled 00334 spi_initialise(); //initialise MBED 00335 00336 sci_write(SCI_MODE, (SM_SDINEW+SM_RESET)); // set mode reg. 00337 wait_ms(10); 00338 00339 #ifdef DEBUG 00340 unsigned int info = wram_read(para_chipID_0); 00341 DEBUGOUT("VS1053b: ChipID_0:%04X\r\n", info); 00342 info = wram_read(para_chipID_1); 00343 DEBUGOUT("VS1053b: ChipID_1:%04X\r\n", info); 00344 info = wram_read(para_version); 00345 DEBUGOUT("VS1053b: Structure version:%04X\r\n", info); 00346 #endif 00347 00348 //get chip version, set clock multiplier and load patch 00349 int i = (sci_read(SCI_STATUS) & 0xF0) >> 4; 00350 if (i == 4) { 00351 00352 DEBUGOUT("VS1053b: Installed Chip is: VS1053\r\n"); 00353 00354 sci_write(SCI_CLOCKF, (SC_MULT_XTALIx50)); 00355 wait_ms(10); 00356 #ifdef VS_PATCH 00357 // loading patch 00358 write_plugin(vs1053b_patch, sizeof(vs1053b_patch)/2); 00359 00360 DEBUGOUT("VS1053b: Patch is loaded.\r\n"); 00361 DEBUGOUT("VS1053b: Patch size:%d bytes\r\n",sizeof(vs1053b_patch)); 00362 00363 #endif // VS_PATCH 00364 } 00365 else 00366 { 00367 DEBUGOUT("VS1053b: Not Supported Chip\r\n"); 00368 return false; 00369 } 00370 00371 // change spi to higher speed 00372 sdi_initialise(); 00373 changeVolume(); 00374 changeBass(); 00375 _isIdle = true; 00376 _isPlay = false; 00377 return true; 00378 } 00379 00380 void VS1053::setVolume(float vol) 00381 { 00382 if (vol > -0.5) 00383 _volume = -0.5; 00384 else 00385 _volume = vol; 00386 00387 changeVolume(); 00388 } 00389 00390 float VS1053::getVolume(void) 00391 { 00392 return _volume; 00393 } 00394 00395 void VS1053::setBalance(float balance) 00396 { 00397 _balance = balance; 00398 00399 changeVolume(); 00400 } 00401 00402 float VS1053::getBalance(void) 00403 { 00404 return _balance; 00405 } 00406 00407 void VS1053::changeVolume(void) 00408 { 00409 // volume calculation 00410 unsigned short volCalced = (((char)(_volume / -0.5f)) << 8) + (char)((_volume - _balance) / -0.5f); 00411 00412 sci_write(SCI_VOL, volCalced); 00413 00414 DEBUGOUT("VS1053b: Change volume to %#x (%f, Balance = %f)\r\n", volCalced, _volume, _balance); 00415 } 00416 00417 int VS1053::getTrebleFrequency(void) 00418 { 00419 return _st_freqlimit * 1000; 00420 } 00421 00422 00423 void VS1053::setTrebleFrequency(int frequency) 00424 { 00425 frequency /= 1000; 00426 00427 if(frequency < 1) 00428 { 00429 frequency = 1; 00430 } 00431 else if(frequency > 15) 00432 { 00433 frequency = 15; 00434 } 00435 _st_freqlimit = frequency; 00436 changeBass(); 00437 } 00438 00439 int VS1053::getTrebleAmplitude(void) 00440 { 00441 return _st_amplitude; 00442 } 00443 00444 void VS1053::setTrebleAmplitude(int amplitude) 00445 { 00446 if(amplitude < -8) 00447 { 00448 amplitude = -8; 00449 } 00450 else if(amplitude > 7) 00451 { 00452 amplitude = 7; 00453 } 00454 _st_amplitude = amplitude; 00455 changeBass(); 00456 } 00457 00458 int VS1053::getBassFrequency(void) 00459 { 00460 return _sb_freqlimit * 10; 00461 } 00462 00463 void VS1053::setBassFrequency(int frequency) 00464 { 00465 frequency /= 10; 00466 00467 if(frequency < 2) 00468 { 00469 frequency = 2; 00470 } 00471 else if(frequency > 15) 00472 { 00473 frequency = 15; 00474 } 00475 _sb_freqlimit = frequency; 00476 changeBass(); 00477 } 00478 00479 int VS1053::getBassAmplitude(void) 00480 { 00481 return _sb_amplitude; 00482 } 00483 00484 void VS1053::setBassAmplitude(int amplitude) 00485 { 00486 if(amplitude < -15) 00487 { 00488 amplitude = -15; 00489 } 00490 else if(amplitude > 0) 00491 { 00492 amplitude = 0; 00493 } 00494 _sb_amplitude = amplitude; 00495 changeBass(); 00496 } 00497 00498 void VS1053::changeBass(void) 00499 { 00500 unsigned short bassCalced = ((_st_amplitude & 0x0f) << 12) 00501 | ((_st_freqlimit & 0x0f) << 8) 00502 | ((_sb_amplitude & 0x0f) << 4) 00503 | ((_sb_freqlimit & 0x0f) << 0); 00504 00505 sci_write(SCI_BASS, bassCalced); 00506 00507 DEBUGOUT("VS1053b: Change bass settings to:\r\n") 00508 DEBUGOUT("VS1053b: --Treble: Amplitude=%i, Frequency=%i\r\n", getTrebleAmplitude(), getTrebleFrequency()); 00509 DEBUGOUT("VS1053b: --Bass: Amplitude=%i, Frequency=%i\r\n", getBassAmplitude(), getBassFrequency()); 00510 } 00511 00512 /*=================================================================== 00513 * Buffer handling 00514 *==================================================================*/ 00515 00516 unsigned int VS1053::bufferLength(void) 00517 { 00518 return BUFFER_SIZE; 00519 } 00520 00521 unsigned char VS1053::bufferGetByte(void) 00522 { 00523 unsigned char retVal = 0x00; 00524 if (bufferCount() > 0x00) 00525 { 00526 retVal = *_bufferReadPointer++; 00527 if (_bufferReadPointer >= _buffer + BUFFER_SIZE) 00528 { 00529 _bufferReadPointer = _buffer; 00530 } 00531 } 00532 return retVal; 00533 } 00534 00535 bool VS1053::bufferSetByte(char c) 00536 { 00537 if (bufferFree() > 0x00) 00538 { 00539 *_bufferWritePointer++ = c; 00540 if (_bufferWritePointer >= _buffer + BUFFER_SIZE) 00541 { 00542 _bufferWritePointer = _buffer; 00543 } 00544 return true; 00545 } 00546 return false; 00547 } 00548 00549 bool VS1053::bufferPutStream(const char *s, unsigned int length) 00550 { 00551 if (bufferFree() >= length) 00552 { 00553 while (length--) 00554 { 00555 *_bufferWritePointer++ = *s++; 00556 if (_bufferWritePointer >= _buffer + BUFFER_SIZE) 00557 { 00558 _bufferWritePointer = _buffer; 00559 } 00560 } 00561 return true; 00562 } 00563 return false; 00564 } 00565 00566 unsigned int VS1053::bufferFree(void) 00567 { 00568 if(_bufferReadPointer > _bufferWritePointer) 00569 { 00570 return _bufferReadPointer - _bufferWritePointer - 1; 00571 } 00572 else if(_bufferReadPointer < _bufferWritePointer) 00573 { 00574 return BUFFER_SIZE - (_bufferWritePointer - _bufferReadPointer) - 1; 00575 } 00576 return BUFFER_SIZE - 1; 00577 } 00578 00579 unsigned int VS1053::bufferCount(void) 00580 { 00581 return BUFFER_SIZE - bufferFree() - 1; 00582 } 00583 00584 void VS1053::bufferReset(void) 00585 { 00586 _bufferReadPointer = _buffer; 00587 _bufferWritePointer = _buffer; 00588 } 00589 00590 #ifdef VS1053_RTOS 00591 void VS1053::dataRequestHandler_start(const void *args) 00592 { 00593 VS1053 *instance = (VS1053*)args; 00594 instance->dataRequestHandler(); 00595 } 00596 #endif 00597 00598 void VS1053::dataRequestHandler(void) 00599 { 00600 #ifdef VS1053_RTOS 00601 _thread.signal_wait(START_THREAD); 00602 00603 while(1) 00604 { 00605 if (!_DREQ || !_isPlay) { 00606 Thread::wait(10); 00607 continue; 00608 } 00609 #else 00610 if (_isIdle && _DREQ) 00611 { 00612 #endif 00613 _isIdle = false; 00614 00615 #ifdef DEBUG 00616 _led = 0; 00617 #endif 00618 00619 // write buffer to vs1053b 00620 unsigned length = bufferCount(); 00621 int i = 0; 00622 sdi_en(); 00623 00624 while (length > 0) 00625 { 00626 int l2 = (length > 32) ? 32 : length; 00627 //DEBUGOUT("L2: %i\r\n", l2); 00628 for( ; l2 != 0; l2--) 00629 { 00630 _spi.write(bufferGetByte()); 00631 } 00632 00633 length -= l2; 00634 00635 //wait_us(50); 00636 00637 if (!_DREQ || i > 4) 00638 break; 00639 i++; 00640 } 00641 00642 sdi_dis(); 00643 00644 #ifdef DEBUG 00645 _led = 1; 00646 #endif 00647 00648 _isIdle = true; 00649 00650 #ifdef VS1053_RTOS 00651 //Thread::wait(10); 00652 Thread::yield(); 00653 #endif 00654 } 00655 } 00656 00657 void VS1053::play(void) 00658 { 00659 INTERRUPT_HANDLER_ENABLE; 00660 DEBUGOUT("VS1053b: Play.\r\n"); 00661 } 00662 00663 void VS1053::pause(void) 00664 { 00665 INTERRUPT_HANDLER_DISABLE; 00666 DEBUGOUT("VS1053b: Pause.\r\n"); 00667 #ifdef DEBUG 00668 _led = 1; 00669 #endif 00670 } 00671 00672 void VS1053::stop(void) 00673 { 00674 INTERRUPT_HANDLER_DISABLE; 00675 #ifndef VS1053_RTOS 00676 __disable_irq(); 00677 #endif 00678 DEBUGOUT("VS1053b: Song stoping..\r\n"); 00679 while(!_isIdle) 00680 ; 00681 00682 // set SCI MODE bit SM CANCEL 00683 unsigned short sciModeByte = sci_read(SCI_MODE); 00684 sciModeByte |= SM_CANCEL; 00685 sci_write(SCI_MODE, sciModeByte); 00686 00687 // send up 2048 bytes of audio data. 00688 for (int i = 0; i < 64; i++) 00689 { 00690 // send at least 32 bytes of audio data 00691 int z = bufferCount(); 00692 if (z > 32) 00693 z = 32; 00694 for (int n = 0; n < z; n++) 00695 { 00696 _spi.write(bufferGetByte()); 00697 } 00698 // read SCI MODE; if SM CANCEL is still set, repeat 00699 sciModeByte = sci_read(SCI_MODE); 00700 if ((sciModeByte & SM_CANCEL) == 0x0000) 00701 { 00702 break; 00703 } 00704 } 00705 00706 if ((sciModeByte & SM_CANCEL) == 0x0000) 00707 { 00708 // send at least 2052 bytes of endFillByte[7:0]. 00709 // read endFillByte (0 .. 15) from wram 00710 unsigned short endFillByte=wram_read(para_endFillByte); 00711 // clear endFillByte (8 .. 15) 00712 endFillByte = endFillByte ^0x00FF; 00713 for (int n = 0; n < 2052; n++) 00714 sdi_write(endFillByte); 00715 DEBUGOUT("VS1053b: Song sucessfully stopped.\r\n"); 00716 DEBUGOUT("VS1053b: SCI MODE = %#x, SM_CANCEL = %#x\r\n", sciModeByte, sciModeByte & SM_CANCEL); 00717 sci_write(SCI_DECODE_TIME, 0x0000); 00718 } 00719 else 00720 { 00721 DEBUGOUT("VS1053b: SM CANCEL hasn't cleared after sending 2048 bytes, do software reset\r\n"); 00722 DEBUGOUT("VS1053b: SCI MODE = %#x, SM_CANCEL = %#x\r\n", sciModeByte, sciModeByte & SM_CANCEL); 00723 initialize(); 00724 } 00725 00726 bufferReset(); 00727 #ifndef VS1053_RTOS 00728 __enable_irq(); 00729 #endif 00730 } 00731 00732 void VS1053::getAudioInfo(AudioInfo* aInfo) 00733 { 00734 // volume calculation 00735 unsigned short hdat0 = sci_read(SCI_HDAT0); 00736 unsigned short hdat1 = sci_read(SCI_HDAT1); 00737 00738 DEBUGOUT("VS1053b: Audio info\r\n"); 00739 00740 AudioInfo* retVal = aInfo; 00741 retVal->type = UNKNOWN; 00742 00743 if (hdat1 == 0x7665) 00744 { 00745 // audio is WAV 00746 retVal->type = WAV; 00747 } 00748 else if (hdat1 == 0x4154 || hdat1 == 0x4144 || hdat1 == 0x4D34 ) 00749 { 00750 // audio is AAC 00751 retVal->type = AAC; 00752 } 00753 else if (hdat1 == 0x574D ) 00754 { 00755 // audio is WMA 00756 retVal->type = WMA; 00757 } 00758 else if (hdat1 == 0x4D54 ) 00759 { 00760 // audio is MIDI 00761 retVal->type = MIDI; 00762 } 00763 else if (hdat1 == 0x4F76 ) 00764 { 00765 // audio is OGG VORBIS 00766 retVal->type = OGG_VORBIS; 00767 } 00768 else if (hdat1 >= 0xFFE0 && hdat1 <= 0xFFFF) 00769 { 00770 // audio is mp3 00771 retVal->type = MP3; 00772 00773 DEBUGOUT("VS1053b: Audio is mp3\r\n"); 00774 retVal->ext.mp3 .id = (MP3_ID)((hdat1 >> 3) & 0x0003); 00775 switch((hdat1 >> 1) & 0x0003) 00776 { 00777 case 3: 00778 retVal->ext.mp3 .layer = 1; 00779 break; 00780 case 2: 00781 retVal->ext.mp3 .layer = 2; 00782 break; 00783 case 1: 00784 retVal->ext.mp3 .layer = 3; 00785 break; 00786 default: 00787 retVal->ext.mp3 .layer = 0; 00788 break; 00789 } 00790 retVal->ext.mp3 .protrectBit = (hdat1 >> 0) & 0x0001; 00791 00792 char srate = (hdat0 >> 10) & 0x0003; 00793 retVal->ext.mp3 .kSampleRate = _sampleRateTable[retVal->ext.mp3 .id][srate]; 00794 00795 retVal->ext.mp3 .padBit = (hdat0 >> 9) & 0x0001; 00796 retVal->ext.mp3 .mode =(MP3_MODE)((hdat0 >> 6) & 0x0003); 00797 retVal->ext.mp3 .extension = (hdat0 >> 4) & 0x0003; 00798 retVal->ext.mp3 .copyright = (hdat0 >> 3) & 0x0001; 00799 retVal->ext.mp3 .original = (hdat0 >> 2) & 0x0001; 00800 retVal->ext.mp3 .emphasis = (hdat0 >> 0) & 0x0003; 00801 00802 DEBUGOUT("VS1053b: ID: %i, Layer: %i, Samplerate: %i, Mode: %i\r\n", retVal->ext.mp3 .id, retVal->ext.mp3 .layer, retVal->ext.mp3 .kSampleRate, retVal->ext.mp3 .mode); 00803 } 00804 00805 // read byteRate 00806 unsigned short byteRate = wram_read(para_byteRate); 00807 retVal->kBitRate = (byteRate * 8) / 1000; 00808 DEBUGOUT("VS1053b: BitRate: %i kBit/s\r\n", retVal->kBitRate ); 00809 00810 // decode time 00811 retVal->decodeTime = sci_read(SCI_DECODE_TIME); 00812 DEBUGOUT("VS1053b: Decodetime: %i s\r\n", retVal->decodeTime ); 00813 00814 }
Generated on Tue Jul 19 2022 01:19:15 by
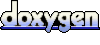