
Interface layer for the mbed boards ready for the JAVA library
Dependencies: C12832 LM75B MMA7660 mbed FXOS8700Q
Fork of frdm_serial by
main.h
00001 #ifndef __main_h_ 00002 #define __main_h_ 00003 00004 #ifndef M_PI 00005 #define M_PI 3.14159265358979323f 00006 #endif 00007 00008 //COMMAND 00009 typedef enum command_character_t { 00010 //read commands 00011 READ_DIGITAL = 0, READ_POT, READ_TEMP, READ_BOARD_ACCEL, READ_SHIELD_ACCEL, READ_BOARD_MAGNO, 00012 //set commands 00013 SET_SHIELD_LED, SET_BOARD_LED, SET_PIEZO, 00014 //higher level get commands 00015 GET_ORIENTATION, GET_SIDE, GET_HEADING, 00016 //lcd commands 00017 SET_LCD_POSITION, PRINT_TEXT, SET_PIXEL, DRAW_CIRCLE, FILL_CIRCLE, DRAW_LINE, DRAW_RECT, FILL_RECT, CLEAR_LCD, 00018 //other commands 00019 RECONNECT, COMMAND_LENGTH 00020 } command_character; 00021 00022 void read_digital(); 00023 void read_pot(); 00024 void read_temp(); 00025 void read_board_accel(); 00026 void read_shield_accel(); 00027 void read_board_magno(); 00028 void set_shield_led(); 00029 void set_board_led(); 00030 void set_piezo(); 00031 void get_orientation(); 00032 void get_side(); 00033 void get_heading(); 00034 void set_lcd_position(); 00035 void print_text(); 00036 void set_lcd_pixel(); 00037 void draw_circle(); 00038 void fill_circle(); 00039 void draw_line(); 00040 void draw_rect(); 00041 void fill_rect(); 00042 void clear_lcd(); 00043 void reconnect(); 00044 00045 // Array of pointers to command handlers 00046 void (*commandFunctions[COMMAND_LENGTH]) (void) = { 00047 read_digital, read_pot, read_temp, read_board_accel, read_shield_accel, read_board_magno, 00048 00049 set_shield_led, set_board_led, set_piezo, 00050 00051 get_orientation, get_side, get_heading, 00052 00053 set_lcd_position, print_text, set_lcd_pixel, draw_circle, fill_circle, draw_line, draw_rect, fill_rect, clear_lcd, 00054 00055 reconnect 00056 }; 00057 00058 #endif // __main_h_
Generated on Sun Jul 17 2022 01:45:37 by
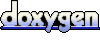