A library for the MQ2 sensor. Based on https://github.com/labay11 and http://sandboxelectronics.com/?p=165
Dependents: mq2_example mq2_midtermproject ECE595_Group9_FinalProject mq2_example ... more
MQ2.h
00001 #ifndef MQ2_h 00002 #define MQ2_h 00003 00004 #include "mbed.h" 00005 00006 #define RL_VALUE 1 //define the load resistance on the board, in kilo ohms 00007 #define RO_DEFAULT 10 //Ro is initialized to 10 kilo ohms 00008 #define RO_CLEAN_AIR_FACTOR 9.83f //RO_CLEAR_AIR_FACTOR=(Sensor resistance in clean air)/RO, which is derived from the chart in datasheet 00009 #define CALIBARAION_SAMPLE_TIMES 5 //define how many samples you are going to take in the calibration phase 00010 #define CALIBRATION_SAMPLE_INTERVAL 50 //define the time interal(in milisecond) between each samples 00011 #define READ_SAMPLE_INTERVAL 50 //define how many samples you are going to take in normal operation 00012 #define READ_SAMPLE_TIMES 5 //define the time interal(in milisecond) between each samples 00013 00014 //The curves 00015 static float LPGCurve[] = {2.3f,0.21f,-0.47f}; 00016 static float COCurve[] = {2.3f,0.72f,-0.34f}; 00017 static float SmokeCurve[] = {2.3f,0.53f,-0.44f}; 00018 00019 typedef struct { 00020 float lpg; 00021 float co; 00022 float smoke; 00023 } MQ2_data_t; 00024 00025 typedef enum { 00026 GAS_LPG = 0, 00027 GAS_CO = 1, 00028 GAS_SMOKE = 2 00029 } GasType; 00030 00031 00032 class MQ2 { 00033 public: 00034 MQ2(PinName pin) : _pin(pin){ 00035 Ro = RO_DEFAULT; 00036 }; 00037 void read(MQ2_data_t *ptr); 00038 float readLPG(); 00039 float readCO(); 00040 float readSmoke(); 00041 void begin(); 00042 private: 00043 AnalogIn _pin; 00044 float MQRead(); 00045 float MQGetGasPercentage(float rs_ro_ratio, GasType gas_id); 00046 int MQGetPercentage(float rs_ro_ratio, float *pcurve); 00047 float MQCalibration(); 00048 float MQResistanceCalculation(int raw_adc); 00049 float Ro; 00050 }; 00051 00052 #endif
Generated on Tue Jul 12 2022 17:52:55 by
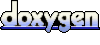