
Modification into 17th July New display demo code
Dependencies: SDFileSystem ds3231 eeprom_Nikita mbed testUniGraphic_150217
Fork of Ext_Demo_17July2017_newdis by
ecgg.cpp
00001 /** ECG ADS1291 Test program. 00002 ADS1291 is a single channel ECG chip 00003 with a 24 bit Sigma-Delta ADC 00004 */ 00005 00006 #include "mbed.h" 00007 #include <string.h> 00008 #include <stdio.h> 00009 #include "ds3231.h" 00010 #include "SDFileSystem.h" 00011 #include "ecg_dec.h" 00012 #include "rtc.h" 00013 #include "sdcard.h" 00014 #include "ec_bp.h" 00015 00016 #include "struct.h" // Added on 31/5/17_Nidhin 00017 //#include "test_type.h" //Added on 31/5/17_Nidhin 00018 FILE *fpeecg1; // FILE pointer to ECG file 00019 Serial pc(USBTX,USBRX); 00020 00021 00022 uint16_t ecg(int32_t pid) 00023 //float ecg(int pid) 00024 { 00025 00026 uint8_t lead_reg=0; // added on 14/06 to check lead -off 00027 //----------------------- Structure for Bluetooth Added Nidhin 1/6/2017-------------------// 00028 00029 //BLUETOOTH STRUCTURE 00030 00031 BLEMsg_info *ptr_BLEMsg_info_ecg, BLEMsg_info_ecg; // A copy of master strcuture [ "BLEMsg_info" ] by name "BLEMsg_info_ecg" is created 00032 ptr_BLEMsg_info_ecg = &BLEMsg_info_ecg; // *ptr_BLEMsg_info_bp is the pointer to local copy; 00033 00034 // Declaration of Date Structure 00035 DateTime_info *ptr_DateTime_info_ecg, DateTime_info_ecg; // A copy of Master Structure "DateTime_info" created, 00036 ptr_DateTime_info_ecg = &DateTime_info_ecg; // Structure pointer points to that copy. 00037 00038 00039 // RTC operations 00040 time_t epoch_time_ecg; //A copy of time_t by name epoch_time_bp is created 00041 epoch_time_ecg = rtc_read(); // time is got from get epoch function. 00042 00043 struct tm * ptr_time_info_ecg, time_info_ecg; // Sturucture copy of tm is created 00044 ptr_time_info_ecg = localtime(&epoch_time_ecg); // Structure accepts the time in local format from "time_t" type. 00045 00046 //BELOW LINE IS TO CHECK Date and TIME 00047 //pc.printf("Time is %d: %d: %d\n", (*ptr_time_info_ecg).tm_hour, (*ptr_time_info_ecg).tm_min, (*ptr_time_info_ecg).tm_sec); 00048 //pc.printf("Date is %d:%d:%d\n", (*ptr_time_info_ecg).tm_mday, (*ptr_time_info_ecg).tm_mon+1, (*ptr_time_info_ecg).tm_year-100); 00049 00050 //Copying from one structure to the other using variables 00051 DateTime_info_ecg.hour = (uint8_t)(*ptr_time_info_ecg).tm_hour; 00052 DateTime_info_ecg.mins = (uint8_t)(*ptr_time_info_ecg).tm_min; 00053 DateTime_info_ecg.sec = (uint8_t)(*ptr_time_info_ecg).tm_sec; 00054 00055 DateTime_info_ecg.date = (uint8_t) (*ptr_time_info_ecg).tm_mday; 00056 DateTime_info_ecg.month =(uint8_t)(*ptr_time_info_ecg).tm_mon+1; 00057 DateTime_info_ecg.year = (uint8_t)(*ptr_time_info_ecg).tm_year-100; 00058 00059 // Copying Time to Main structure 00060 BLEMsg_info_ecg.date_time.hour = DateTime_info_ecg.hour; 00061 BLEMsg_info_ecg.date_time.mins = DateTime_info_ecg.mins; 00062 BLEMsg_info_ecg.date_time.sec = DateTime_info_ecg.sec; 00063 00064 BLEMsg_info_ecg.date_time.date = DateTime_info_ecg.date ; 00065 BLEMsg_info_ecg.date_time.month = DateTime_info_ecg.month ; 00066 BLEMsg_info_ecg.date_time.year = DateTime_info_ecg.year ; 00067 00068 00069 //Checking if the structure has these values 00070 //pc.printf("Time 2 is %d:%d:%d\n", DateTime_info_ecg.hour, DateTime_info_ecg.mins, DateTime_info_ecg.sec); 00071 //pc.printf("\t Date is %d:%d:%d\n",DateTime_info_ecg.date, DateTime_info_ecg.month, DateTime_info_ecg.year); 00072 00073 00074 //Loading values to of Test type 00075 test_type_info test_type_info_ecg; // copy of " test_type_info" created 00076 test_type_info_ecg = ECG_Test; // Loaded value 00 to the test type 00077 00078 BLEMsg_info_ecg.test_type = test_type_info_ecg; 00079 //Check if 00 is getting printed 00080 //pc.printf("Test Type is : %d\n", test_type_info_ecg); 00081 00082 00083 // Loading values of Length , PID, DID, sampling frequency, number of samples, calculated data. 00084 BLEMsg_info_ecg.device_id = 01; // Device ID fixed 00085 BLEMsg_info_ecg.patient_id = (uint32_t)pid; // Patient ID 00086 BLEMsg_info_ecg.sampling_freq = 500; // sampling frrquency 00087 BLEMsg_info_ecg.length = 8022; //Total length of data in bytes 22 B+ 4000 data 00088 00089 /* 00090 //Loading number of samples 00091 NumSamples_info NumSamples_info_bp; //Copy of structure NumSamples_info 00092 NumSamples_info_bp.num_ppg_sample = 1664; // PPG & ECG Sample number loaded in structure copy 00093 NumSamples_info_bp.num_ecg_sample = 1024; 00094 */ 00095 00096 BLEMsg_info_ecg.num_samples.num_sample_ppg_dummy = 0 ;// PPG number of samples copied to master struct 00097 BLEMsg_info_ecg.num_samples.num_sample_ecg_OTtyp = 2000 ; // ECG number of samples copied to master struct 00098 00099 //----------------------------------------END Structure for Bluetooth - Added Nidhin 1/6/2017------- 00100 00101 uint32_t concatenate_value2 = 0; // ORG. "int concatenate_value2 = 0;" Nidhin 1/6/17 00102 uint32_t *ecg_ptr; // Added 1/6/2017 Nidhin 00103 ecg_ptr = &concatenate_value2; // Pointer to pass for ECG write into SD card Nidhin 1/6/2017 00104 00105 uint16_t count = 0; 00106 uint16_t fs = 500; 00107 //uint32_t ecg_buf[N_ECG]; 00108 Timer t; 00109 00110 //------------------ Declaration for Peak value detection ------------------------------------ 00111 uint32_t ecg_samp1[1] ; uint32_t ecg_samp2[1]; uint32_t ecg_samp3[1]; // Buff 12 &3 Stores sample 1, 2 & 10th sample 00112 uint32_t fppos; // Variable to hold pointer position 00113 uint32_t hi_val; 00114 uint32_t pk_val[20]; 00115 uint16_t pk_pos[20]={0}; 00116 // unsigned int a; //uint32_t pk=0; 00117 int32_t hi_dif = 0; //diff between high value and it's consecutive value 00118 uint16_t j=0; // int count1 = N_ECG/fs, a_dif=0, fs1 = fs ,h=0; 00119 int32_t m =0; // Variable to move the file pointer in fseek fun 00120 int32_t samp_10 = 28; // Variable to move to 10th sampple from current 00121 char buffer3[32]; 00122 00123 // ------------------------- Declaration for Heart Rate calculation -------------------------- 00124 uint8_t n=0; 00125 float pos_dif, HR[10], HR1,t_pos_dif; 00126 uint8_t t_sec = 60; 00127 float HR_sum = 0,HR_avg; 00128 // ------------------------------------------------------------------------------------------- 00129 // unsigned char chk = 1; 00130 pc.baud(baud_rate); 00131 freqset(); // setting the frequency 00132 setupfunc(); 00133 //ecgtestsetupfunc(); // For test set up of 1Hz square wave signal 00134 lead_reg= ecgsetupfunc(); 00135 // chk = 1; 00136 00137 00138 //ORIGINAL sd_open_ECGfile(pid); // opening the ecg file COMMENTED Nidhin 1/6/2017 00139 if (lead_reg==0) // checking for proper lead contact// 14/06 00140 { 00141 sd_open_ECGfilee(pid); // REPLACED Nidhin 1/6/2017 Nidhin 00142 00143 pc.printf( "Raw data is = \n"); 00144 00145 for(int i=0; i<N_ECG; i++) 00146 { 00147 concatenate_value2= readvalue(); 00148 pc.printf( "%d\n", concatenate_value2); //ADDED Nidhin 21/6/2017 00149 00150 sd_ecgwrite(ecg_ptr); // REPLACED Nidhin 1/6/2017 00151 00152 } 00153 00154 //sd_close(); // closing the file COMMENTED Nidhin 1/6/2017 00155 sd_close_ecg(); // closing the ECG file REPLACED Nidhin 1/6/2017 Nidhin 00156 00157 00158 /* 00159 // ---------- reading back SD data for processing -------------------- 00160 sd_read_file(15); 00161 printf("Reading back SD data\n"); 00162 for(int i=10;i<N_ECG;i++) 00163 { 00164 ecg_buf= sd_read(); 00165 //printf("%d\n",ecg_buf[i]); 00166 } 00167 00168 sd_close(); */ 00169 00170 00171 //----------------------------- PEAK DETECTION AND HEART RATE CALCULATION --------------------------------------------------- 00172 00173 // -------------------------------------- PEAK DETECTION ------------------------------------------------------------- 00174 // ----------------------------------------- Main loop -------------------------------------------------- 00175 00176 sprintf(buffer3, "/sd/%d_ECG.csv", pid); // For opening a specific file 00177 fpeecg1 = fopen(buffer3, "r"); 00178 00179 for(uint16_t i=0;i<(N_ECG-10);i++){ 00180 count++; 00181 rewind(fpeecg1); // Go to start of file each time 00182 fseek(fpeecg1, m, SEEK_CUR); // Update the count value according to move pointer //// after every calc. the pointer moves to 0th position, as we have used fseek, hence to make it jump to the respective position by "m" bytes this command is used 00183 fread(ecg_samp1, sizeof(uint32_t), 1, fpeecg1); // Read sample 1 00184 fread(ecg_samp2, sizeof(uint32_t), 1, fpeecg1); // Read Sample 2 00185 fseek(fpeecg1, samp_10, SEEK_CUR); // Moving to tenth sample 00186 fread(ecg_samp3, sizeof(uint32_t), 1, fpeecg1); // Read 3rd sample 00187 //pc.printf("ecg_samp1 = %d , ecg_samp2 = %d, ecg_samp3 = %d\n",ecg_samp1[0],ecg_samp2[0],ecg_samp3[0] ); //Test Value held by buffer each round 00188 00189 00190 if(ecg_samp1[0]>ecg_samp2[0]) 00191 { 00192 hi_val = ecg_samp1[0]; //To find the high value 00193 //printf("high value= %d\n",hi_val); 00194 //a = i; 00195 //printf("a= %d\n",a); 00196 hi_dif = hi_val-ecg_samp3[0]; 00197 00198 // ---------------------------- If hi_val is greater than next ten input values, then compare the hi_val with the tenth input value. 00199 // If the diff is greater than 10000, then it is a valid peak (pls chk the below condition)------------------------------------- 00200 // if((hi_dif > 10000) && ((a+10) < N_ECG)) 00201 if(hi_dif > 10000) 00202 { 00203 // if(a_dif <= 0) ------------------------ add this condition if needed ---------------------- 00204 // { 00205 pk_val[j] = hi_val; //if condition satisfied, put the "pk" value into "pk_val" buffer 00206 pc.printf("peak value= %d\n",pk_val[j]); 00207 pk_pos[j]=i; // also save the peak's position 00208 pc.printf("peak position is = %d\n",pk_pos[j]); 00209 i = i+120; // once confirmed that this is the necessary peak, skip the next 120 input values 00210 n = j; // where n is the number of peaks detected 00211 j = j+1; 00212 m = m + 480; //similar reason to considering 28, but to skip 120 samples. this cond. is satisfied only when we hit a peak - suhasini_26thjune17 00213 00214 // printf("j after peak detection is= %d\n",j); 00215 // } 00216 } 00217 00218 else 00219 { 00220 m = m+4; // this is when we do not hit a peak and have to continue searching thru, hence move to the next sample and not skip 120 samples- - suhasini_26thjune17 00221 } 00222 // store the peak value position in "pk_pos" 00223 } 00224 else 00225 { 00226 m = m+4; 00227 } 00228 //pc.printf("i=%d",i); 00229 } 00230 n=n+1; 00231 pc.printf("n=%d\n",n); 00232 00233 // ----------------- HEART RATE LOGIC --------------------------- 00234 00235 for(uint16_t i = 0;i < n-1;i++) 00236 { 00237 pos_dif = pk_pos[i+1] - pk_pos[i]; // difference between two consequtive peaks 00238 pc.printf("peak position diff is = %f\n",pos_dif); 00239 //printf("peak position i value is = %d\n",i); 00240 t_pos_dif = pos_dif/fs; // sample difference between peak positions divided by sampling frequency gives the difference value in terms of actual time 00241 pc.printf("time in seconds is = %f\n",t_pos_dif); 00242 HR[i] = t_sec/t_pos_dif; //HR calculation 00243 pc.printf("Heart Rate is = %f\n",HR[i]); 00244 // n = i; 00245 HR1 = HR[0]; 00246 } 00247 00248 00249 // ---------------------- To average individual HRs for higher number of samples ----------------------- 00250 for(uint16_t i = 0;i < n-1;i++) 00251 { 00252 HR_sum = HR[i]+HR_sum; 00253 } 00254 HR_avg = HR_sum/(n-1); // To find average of all the individual HRs calculated 00255 printf("Heart Rate sum is = %f\n",HR_sum); 00256 //printf("Denominator = %d\n",n); 00257 printf("Heart Rate avg is = %f\n",HR_avg); 00258 00259 fclose(fpeecg1); 00260 pc.printf("temporary file closed\n"); 00261 00262 if(HR_avg>100 || HR_avg<40) 00263 { 00264 00265 return 1; // out of range condition returning 1 //nikita//10/7 00266 00267 } 00268 else 00269 { 00270 00271 BLEMsg_info_ecg.cal_data.cal_sbp_dummy = 0; 00272 BLEMsg_info_ecg.cal_data.cal_dbp_OTtyp = HR_avg; //To be modified after HR code is added. // changed nikita 00273 00274 structure_file(ptr_BLEMsg_info_ecg, pid); //copy the ECG structure to Main file //COMMENTED Nidhin 10/6/2017 00275 ecgfile_mainfile(pid); // copy raw data to the main file and ECG file is cleared. //COMMENTED Nidhin 10/6/2017 00276 00277 pc.printf("Closed the main file\n"); 00278 00279 00280 00281 //return HR1; 00282 return HR_avg; 00283 } 00284 } 00285 else 00286 { 00287 pc.printf("improper lead connection"); 00288 return 0; // returning 0 for improper lead connection 00289 } 00290 pc.printf("closing temporary file\n"); 00291 // fclose(fpeecg1); 00292 // pc.printf("temporary file closed\n"); 00293 } // End of main function
Generated on Wed Jul 13 2022 16:15:21 by
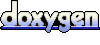