
Inductance Testing Code
Fork of CurrentModeSine by
Embed:
(wiki syntax)
Show/hide line numbers
math_ops.cpp
00001 00002 #include "math_ops.h" 00003 00004 00005 float fmaxf(float x, float y){ 00006 return (((x)>(y))?(x):(y)); 00007 } 00008 00009 float fminf(float x, float y){ 00010 return (((x)<(y))?(x):(y)); 00011 } 00012 00013 float fmaxf3(float x, float y, float z){ 00014 return (x > y ? (x > z ? x : z) : (y > z ? y : z)); 00015 } 00016 00017 float fminf3(float x, float y, float z){ 00018 return (x < y ? (x < z ? x : z) : (y < z ? y : z)); 00019 } 00020 00021 void limit_norm(float *x, float *y, float limit){ 00022 float norm = sqrt(*x * *x + *y * *y); 00023 if(norm > limit){ 00024 *x = *x * limit/norm; 00025 *y = *y * limit/norm; 00026 } 00027 } 00028 00029 void limit_abs(float *x, float limit){ 00030 limit = abs(limit); 00031 if(*x > limit){ 00032 *x = limit; 00033 } 00034 if(*x < -limit){ 00035 *x = -limit; 00036 } 00037 }
Generated on Wed Jul 13 2022 19:52:22 by
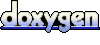