
Slurp
Embed:
(wiki syntax)
Show/hide line numbers
foc.cpp
00001 00002 #include "foc.h" 00003 00004 //#include "FastMath.h" 00005 //using namespace FastMath; 00006 00007 00008 void abc( float theta, float d, float q, float *a, float *b, float *c){ 00009 ///Phase current amplitude = lengh of dq vector/// 00010 ///i.e. iq = 1, id = 0, peak phase current of 1/// 00011 00012 *a = d*cosf(theta) + q*sinf(theta); 00013 *b = d*cosf((2.0f*PI/3.0f)+theta) + q*sinf((2.0f*PI/3.0f)+theta); 00014 *c = d*cosf((-2.0f*PI/3.0f)+theta) + q*sinf((-2.0f*PI/3.0f)+theta); 00015 } 00016 00017 void dq0(float theta, float a, float b, float c, float *d, float *q){ 00018 ///Phase current amplitude = lengh of dq vector/// 00019 ///i.e. iq = 1, id = 0, peak phase current of 1/// 00020 00021 *d = (2.0f/3.0f)*(a*cosf(theta) + b*cosf((2.0f*PI/3.0f)+theta) + c*cosf((-2.0f*PI/3.0f)+theta)); 00022 *q = (2.0f/3.0f)*(a*sinf(theta) + b*sinf((2.0f*PI/3.0f)+theta) + c*sinf((-2.0f*PI/3.0f)+theta)); 00023 } 00024 00025 void svm(float v_bus, float u, float v, float w, float *dtc_u, float *dtc_v, float *dtc_w){ 00026 ///u,v,w amplitude = v_bus for full modulation depth/// 00027 00028 float v_offset = (fminf3(u, v, w) + fmaxf3(u, v, w))/2.0f; 00029 *dtc_u = fminf(fmaxf(((u - v_offset)*0.5f/v_bus + ((DTC_MAX-DTC_MIN)/2)), DTC_MIN), DTC_MAX); 00030 *dtc_v = fminf(fmaxf(((v - v_offset)*0.5f/v_bus + ((DTC_MAX-DTC_MIN)/2)), DTC_MIN), DTC_MAX); 00031 *dtc_w = fminf(fmaxf(((w - v_offset)*0.5f/v_bus + ((DTC_MAX-DTC_MIN)/2)), DTC_MIN), DTC_MAX); 00032 00033 } 00034 00035 void zero_current(int *offset_1, int *offset_2){ 00036 int adc1_offset = 0; 00037 int adc2_offset = 0; 00038 int n = 1024; 00039 for (int i = 0; i<n; i++){ 00040 ADC1->CR2 |= 0x40000000; 00041 wait(.001); 00042 adc2_offset += ADC2->DR; 00043 adc1_offset += ADC1->DR; 00044 } 00045 *offset_1 = adc1_offset/n; 00046 *offset_2 = adc2_offset/n; 00047 } 00048 00049 void reset_foc(ControllerStruct *controller){ 00050 controller->q_int = 0; 00051 controller->d_int = 0; 00052 } 00053 00054 00055 void commutate(ControllerStruct *controller, GPIOStruct *gpio, float theta){ 00056 00057 controller->loop_count ++; 00058 if(gpio->phasing){ 00059 controller->i_b = I_SCALE*(float)(controller->adc2_raw - controller->adc2_offset); //Calculate phase currents from ADC readings 00060 controller->i_a = I_SCALE*(float)(controller->adc1_raw - controller->adc1_offset); 00061 } 00062 else{ 00063 controller->i_b = I_SCALE*(float)(controller->adc1_raw - controller->adc1_offset); //Calculate phase currents from ADC readings 00064 controller->i_a = I_SCALE*(float)(controller->adc2_raw - controller->adc2_offset); 00065 } 00066 controller->i_c = -controller->i_b - controller->i_a; 00067 00068 00069 dq0(controller->theta_elec, controller->i_a, controller->i_b, controller->i_c, &controller->i_d, &controller->i_q); //dq0 transform on currents 00070 00071 ///Cogging Compensation Lookup/// 00072 //int ind = theta * (128.0f/(2.0f*PI)); 00073 //float cogging_current = controller->cogging[ind]; 00074 //float cogging_current = 1.0f*cos(6*theta); 00075 ///Controller/// 00076 float i_d_error = controller->i_d_ref - controller->i_d; 00077 float i_q_error = controller->i_q_ref - controller->i_q;// + cogging_current; 00078 //float v_d_ff = 2.0f*(2*controller->i_d_ref*R_PHASE); //feed-forward voltage 00079 //float v_q_ff = 2.0f*(2*controller->i_q_ref*R_PHASE + controller->dtheta_elec*WB*0.8165f); 00080 controller->d_int += i_d_error; 00081 controller->q_int += i_q_error; 00082 00083 //v_d_ff = 0; 00084 //v_q_ff = 0; 00085 00086 limit_norm(&controller->d_int, &controller->q_int, V_BUS/(K_Q*KI_Q)); 00087 //controller->d_int = fminf(fmaxf(controller->d_int, -D_INT_LIM), D_INT_LIM); 00088 //controller->q_int = fminf(fmaxf(controller->q_int, -Q_INT_LIM), Q_INT_LIM); 00089 00090 00091 controller->v_d = K_D*i_d_error + K_D*KI_D*controller->d_int;// + v_d_ff; 00092 controller->v_q = K_Q*i_q_error + K_Q*KI_Q*controller->q_int;// + v_q_ff; 00093 00094 //controller->v_d = v_d_ff; 00095 //controller->v_q = v_q_ff; 00096 00097 limit_norm(&controller->v_d, &controller->v_q, controller->v_bus); 00098 00099 abc(controller->theta_elec, controller->v_d, controller->v_q, &controller->v_u, &controller->v_v, &controller->v_w); //inverse dq0 transform on voltages 00100 svm(controller->v_bus, controller->v_u, controller->v_v, controller->v_w, &controller->dtc_u, &controller->dtc_v, &controller->dtc_w); //space vector modulation 00101 00102 //gpio->pwm_u->write(1.0f-controller->dtc_u); //write duty cycles 00103 //gpio->pwm_v->write(1.0f-controller->dtc_v); 00104 //gpio->pwm_w->write(1.0f-controller->dtc_w); 00105 00106 //bing1 = (controller->dtc_u); 00107 //bing2 = (controller->dtc_v); 00108 //bing3 = (controller->dtc_w); 00109 /* 00110 //if(gpio->phasing){ 00111 TIM1->CCR1 = 0x1194*(1.0f-controller->dtc_u); 00112 TIM1->CCR2 = 0x1194*(1.0f-controller->dtc_v); 00113 TIM1->CCR3 = 0x1194*(1.0f-controller->dtc_w); 00114 } 00115 else{ 00116 TIM1->CCR3 = 0x1194*(1.0f-controller->dtc_u); 00117 TIM1->CCR1 = 0x1194*(1.0f-controller->dtc_v); 00118 TIM1->CCR2 = 0x1194*(1.0f-controller->dtc_w); 00119 }*/ 00120 //gpio->pwm_u->write(1.0f - .05f); //write duty cycles 00121 //gpio->pwm_v->write(1.0f - .05f); 00122 //gpio->pwm_w->write(1.0f - .1f); 00123 //TIM1->CCR1 = 0x708*(1.0f-controller->dtc_u); 00124 //TIM1->CCR2 = 0x708*(1.0f-controller->dtc_v); 00125 //TIM1->CCR3 = 0x708*(1.0f-controller->dtc_w); 00126 controller->theta_elec = theta; //For some reason putting this at the front breaks thins 00127 00128 00129 if(controller->loop_count >400){ 00130 //controller->i_q_ref = -controller->i_q_ref; 00131 controller->loop_count = 0; 00132 00133 //printf("%d %f\n\r", ind, cogging_current); 00134 //printf("%f\n\r", controller->theta_elec); 00135 //pc.printf("%f %f %f\n\r", controller->i_a, controller->i_b, controller->i_c); 00136 //pc.printf("%f %f\n\r", controller->i_d, controller->i_q); 00137 //pc.printf("%d %d\n\r", controller->adc1_raw, controller->adc2_raw); 00138 } 00139 } 00140 00141 /* 00142 void zero_encoder(ControllerStruct *controller, GPIOStruct *gpio, ){ 00143 00144 } 00145 */ 00146 00147 void voltageabc( float theta, float d, float q, float *a, float *b, float *c){ 00148 ///Phase current amplitude = lengh of dq vector/// 00149 ///i.e. iq = 1, id = 0, peak phase current of 1/// 00150 00151 *a = sinf(theta); 00152 *b = sinf((2.0f*PI/3.0f)+theta); 00153 *c = sinf((-2.0f*PI/3.0f)+theta); 00154 } 00155 00156 00157 00158 00159 00160 00161
Generated on Tue Jul 12 2022 16:57:53 by
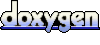