mn
Embed:
(wiki syntax)
Show/hide line numbers
Thermistor.h
00001 #ifndef THERMISTOR_H 00002 #define THERMISTOR_H 00003 /// 00004 /// @mainpage Thermistor Temperature library 00005 /// 00006 /// This is a Thermistor to Temerature conversion library 00007 /// 00008 /// Much thanks to @Adafruit for this tutorial: 00009 /// https://learn.adafruit.com/thermistor/using-a-thermistor 00010 /// 00011 /// The 100K Thermistor is configured with a 4.7k series resistor 00012 /// tied to vcc (3.3v) like this: 00013 /// 00014 /// +3.3v 00015 /// | 00016 /// \ 00017 /// / 4.7k series resistor 00018 /// \ 00019 /// / 00020 /// | 00021 /// .-----------O To Anlog pin on FRDM board 00022 /// | 00023 /// \ 00024 /// / 00025 /// Thermistor 100k Nominal 00026 /// \ 00027 /// / 00028 /// | 00029 /// --- 00030 /// GND 00031 /// 00032 /// 00033 /// @author Michael J. Ball 00034 /// unix_guru at hotmail.com 00035 /// @unix_guru on Twitter 00036 /// March 2016 00037 /// 00038 /// @code 00039 /// #include "mbed.h" 00040 /// #include "Thermistor.h" 00041 /// 00042 /// Serial pc(USBTX, USBRX); 00043 /// 00044 /// Thermistor bed(PTB10); 00045 /// Thermistor extruder(PTB11); 00046 /// 00047 /// int main() 00048 /// { 00049 /// pc.baud(115200); 00050 /// pc.printf("\r\nThermistor Test - Build " __DATE__ " " __TIME__ "\r\n"); 00051 /// 00052 /// while(1) { 00053 /// pc.printf("Bed Temperature %f *C\r\n",bed.get_temperature()); 00054 /// wait(.5); 00055 /// pc.printf("Extruder Temperature %f *C\r\n",extruder.get_temperature()); 00056 /// wait(.5); 00057 /// } 00058 /// } 00059 00060 /// ... 00061 /// @endcode 00062 /// 00063 /// @license 00064 /// Licensed under the Apache License, Version 2.0 (the "License"); 00065 /// you may not use this file except in compliance with the License. 00066 /// You may obtain a copy of the License at 00067 /// 00068 /// http://www.apache.org/licenses/LICENSE-2.0 00069 /// 00070 /// Unless required by applicable law or agreed to in writing, software 00071 /// distributed under the License is distributed on an "AS IS" BASIS, 00072 /// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00073 /// See the License for the specific language governing permissions and 00074 /// limitations under the License. 00075 /// 00076 00077 00078 #include "mbed.h" 00079 00080 00081 #define THERMISTORNOMINAL 10000 // 100k default 00082 // temp. for nominal resistance (almost always 25 C) 00083 #define TEMPERATURENOMINAL 25 00084 // The beta coefficient of the thermistor (usually 3000-4000) 00085 #define BCOEFFICIENT 3950 00086 // the value of the 'other' resistor 00087 #define SERIESRESISTOR 4700 00088 00089 00090 class Thermistor { 00091 public: 00092 Thermistor(PinName pin); 00093 /** Receives a PinName variable. 00094 * @param pin mbed pin to which the thermistor is connected 00095 */ 00096 00097 00098 float get_temperature(); 00099 /** This is the workhorse routine that calculates the temperature 00100 * using the Steinhart-Hart equation for thermistors 00101 * https://en.wikipedia.org/wiki/Steinhart%E2%80%93Hart_equation 00102 */ 00103 void init(); // Set default Steinhart-Hart values 00104 void set_ThermistorNominal(float thermnom); // Change the thermistors nominal resistance 00105 void set_TemperatureNominal(float tempnom); // Change the thermistors nominal temperature 00106 void set_BCoefficient(float bcoefficient); // Change the thermistors BCoefficient 00107 void set_SeriesResistor(float resistor); // Change the value of the series resistor 00108 00109 private: 00110 AnalogIn _pin; 00111 double ThermistorNominal; 00112 double TemperatureNominal; 00113 double BCoefficient; 00114 double SeriesResistor; 00115 }; 00116 00117 #endif
Generated on Thu Jul 28 2022 14:08:26 by
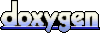